By controlling LED lights, you can become familiar with STM32's memory structure, address mapping, clock tree, library files, library utilization methods, and development project steps, and establish STM32 development ideas.
This is the first project I have built. Since it involves a wide range of areas and the knowledge points are scattered, I will make a summary here.
1) To control the LED light, you need to use the GPIO peripheral.
GPIO (General-Purpose I/O): I/O pins can be set by software to various functions, such as input or output. Controlling the LED light is achieved by controlling the level of the I/O pins of the STM32 chip.
2) Understand the functions of the GPIO peripheral and how to use it.
GPIO pins are divided into different groups: GPIOA, GPIOB, ..., GPIOG. Each group of ports is divided into 16 different pins from 0 to 15. For different chip models, the number of port groups and pins is different.
Function View Reference Manual
3) Get the address mapping of GPIO and know the bus APB2 on which it is mounted.
4) Understand the encapsulation of registers in the ST official library.
5) Understand the clock tree and check the clock source of GPIOC, which is PCLK2.
6) Include the used header files stm32f10x_gpio.h and stm32f10x_rcc.h in the stm32f10x_conf.h file.
7) Add led.c and led.h user files based on the project template.
8) Write the driver initialization function LED_GPIO_Config().
9) Turn on the peripheral GPIOC clock and analyze whether the default clock frequency of Sysclk=71MHz configured by the SystemInit() function meets the project requirements.
10) Define and fill the initialization structure GPIO_InitStructure according to the control requirements, and write appropriate parameters to the corresponding structure members.
11) Call the initialization function GPIO_Init() to initialize GPIOC.
12) Write the corresponding led.h header file.
13) Write the main application.
14) Debug the program and complete.
The project I built:
/*led.h*/
#ifndef __LED_H
#define __LED_H
#include "stm32f10x.h"
#define ON 0
#define OFF 1
#define LED1(a) if(a) \
GPIO_SetBits(GPIOD,GPIO_Pin_3);\
else \
GPIO_ResetBits(GPIOD,GPIO_Pin_3)
#define LED2(a) if(a) \
GPIO_SetBits(GPIOD,GPIO_Pin_6);\
else \
GPIO_ResetBits(GPIOD,GPIO_Pin_6)
#define LED3(a) if(a) \
GPIO_SetBits(GPIOB,GPIO_Pin_5);\
else \
GPIO_ResetBits(GPIOB,GPIO_Pin_5)
void LED_GPIO_Config(void);
#endif /*__LED_H*/
/*led.c*/
#include "led.h"
/*Configure the I/O port used by the LED*/
void LED_GPIO_Config(void)
{
/*Define a GPIO_InitTypeDef type structure*/
GPIO_InitTypeDef GPIO_InitStructure;
/*Turn on the peripheral clock of GPIOC*/
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB,ENABLE);
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOD,ENABLE);
/*Select the GPIO pin to control*/
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_3 | GPIO_Pin_6 | GPIO_Pin_5;
/*Set pin mode to general push-pull output*/
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
/*Set the pin rate to 50MHz*/
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
/*Call library function to initialize GPIOB, GPIOD*/
GPIO_Init(GPIOB,&GPIO_InitStructure);
GPIO_Init(GPIOD,&GPIO_InitStructure);
/*Turn off all LED lights*/
GPIO_SetBits(GPIOB,GPIO_Pin_5); //Pins 3, 6, and 5 output high level and turn off the three LED lights
GPIO_SetBits(GPIOD,GPIO_Pin_3|GPIO_Pin_6);
}
/*main.c*/
#include "stm32f10x.h"
#include "led.h"
void Delay(__IO u32 nCount);
int main (void)
{
SystemInit() ; //Set the system clock SYSCLK
LED_GPIO_Config();
while(1)
{
LED1(ON); //light up
Delay(0x0FFFEF);
LED1(OFF);
LED2(ON);
Delay(0x0FFFEF);
LED2(OFF);
LED3(ON);
Delay(0x0FFFEF);
LED3(OFF);
}
The above code runs in the STM32 class. The following is a recommended example for comparison to find out your own shortcomings.
/*example*/
#include"stm32f10x.h"
#define ON 1
#define OFF 0
#define DELAY_TIME 0x3FFFFF
enum
{
LED1 = 0,
LED2,
LED3,
MAX_LED,
};
typedef struct led_gpio_s
{
int num; /* LED number*/
GPIO_TypeDef *group; /* Which group is the GPIO used by the LED: GPIOB or GPIOD */
uint16_t pin; /* Which pin in the GPIO group is used by the LED: GPIO_Pin_x */
} led_gpio_t;
led_gpio_t leds_gpio[MAX_LED] =
{
{LED1, GPIOB, GPIO_Pin_5}, /* GPB5 for LED1 */
{LED2, GPIOD, GPIO_Pin_6}, /* GPD6 for LED2 */
{LED3, GPIOD, GPIO_Pin_3}, /* LED3 use GPD3 */
};
void init_led_gpio(void)
{
int i;
GPIO_InitTypeDef GPIO_InitStructure;
/* Enable the clock of PB and PD group GPIO*/
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB | RCC_APB2Periph_GPIOD , ENABLE);
/*Set PB5(LED1), PD6(LED2), PD3(LED3) to GPIO output push-to-disable mode, port line flip speed is 50MHz */
for(i=0; i { /*Set PB5 (LED1) to GPIO output push-to-disable mode, port line flip speed is 50MHz */ GPIO_InitStructure.GPIO_Pin = leds_gpio[i].pin; GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP; GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz; GPIO_Init(leds_gpio[i].group, &GPIO_InitStructure); } } void turn_led(int which, int cmd) { if(which<0 || which> MAX_LED ) return; if(OFF == cmd) GPIO_ResetBits(leds_gpio[which].group, leds_gpio[which].pin); else GPIO_SetBits(leds_gpio[which].group, leds_gpio[which].pin); } void Delay(__IO uint32_t nCount) { for(; nCount != 0; nCount--) ; } int main(void) { /* Initialize system clock */ SystemInit(); /* Initialize the GPIO pins of each LED*/ init_led_gpio(); while(1) { /* Turn on LED1 and turn off LED2 and LED3*/ turn_led(LED1, ON); turn_led(LED2, OFF); turn_led(LED3, OFF); Delay(DELAY_TIME); /* Turn on LED2 and turn off LED1 and LED3*/ turn_led(LED2, ON); turn_led(LED1, OFF); turn_led(LED3, OFF); Delay(DELAY_TIME); /* Turn on LED3 and turn off LED1 and LED2*/ turn_led(LED3, ON); turn_led(LED2, OFF); turn_led(LED1, OFF); Delay(DELAY_TIME); } }
Previous article:STM32F103C8T6-LED lighting program
Next article:STM32F407 serial port usage collection (USART1, USART2, USART3, USART6)
Recommended ReadingLatest update time:2024-11-15 16:46
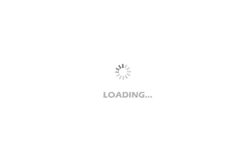
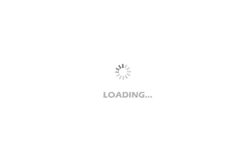
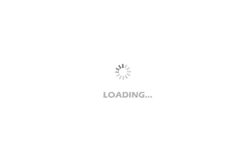
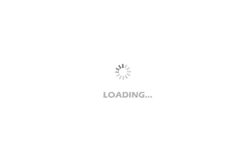
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- Huawei's Strategic Department Director Gai Gang: The cumulative installed base of open source Euler operating system exceeds 10 million sets
- Download from the Internet--ARM Getting Started Notes
- Learn ARM development(22)
- Learn ARM development(21)
- Learn ARM development(20)
- Learn ARM development(19)
- Learn ARM development(14)
- Learn ARM development(15)
- Analysis of the application of several common contact parts in high-voltage connectors of new energy vehicles
- Wiring harness durability test and contact voltage drop test method
- IoT platform architecture design
- Tantalum is easy to break down
- Very detailed filter basics
- EEWORLD University Hall----Live Replay: How to solve the challenge of precision timing in ADAS systems
- When will it end? ST is out of stock and it is hard to find a domestic alternative, but the domestic ones are also out of stock...
- [Mil MYC-JX8MPQ Review] 3. GPIO Application Programming
- 【GD32F307E-START】+connected to UASRT interface power supply
- 【Laser target detector】I2C and SPI
- MOS tube knowledge
- PCB technology: PROTEL's metal slot method