The topic of heap and stack has become a monthly topic for programmers, and most of the discussions are based on the OS layer.
So, what is the distribution of the heap and stack in a bare-bones MCU? Here is an excerpt from the Internet:
When you first take over STM32, you only write one
int main()
{
while(1);
}
BUILD://Program Size: Code=340 RO-data=252 RW-data=0 ZI-data=1632
After compiling, you will find that such a program has used more than 1600 RAM. If it is on a 51 microcontroller, you will be very sad. Where did the more than 1600 RAM go?
Analyzing the map, you will find that it is occupied by the heap and stack. In the startup_stm32f10x_md.s file, the above definition is in the first few lines.
Now you understand.
Stack_Size EQU 0x00000400
Heap_Size EQU 0x00000200
The following references to online information to understand the difference between the heap and the stack
(1) Stack: It is automatically allocated and released by the compiler to store function parameter values, local variable values, etc. Its operation is similar to
A stack in a data structure .
(2) Heap: Generally allocated and released by the programmer. If the programmer does not release it, it may be reclaimed by the operating system when the program ends .
The method is similar to the linked list in data structure.
(3) Global area (static area): Global variables and static variables are stored together. Initialized global variables and static variables are stored together.
Variables are in one area, and uninitialized global variables and uninitialized static variables are in another adjacent area.
The system is automatically released.
(4) Text constant area: Constant character strings are stored here.
(5) Program code area: stores the binary code of the function body.
For example:
int a=0; //global initialization area
char *p1; //global uninitialized area
main()
{
int b; //stack
char s[]="abc"; //stack
char *p3= "1234567"; // Flash in the text constant area
static int c =0 ; //static initialization area
p1= (char *)malloc(10); //heap area
strcpy(p1,"123456"); //"123456" is placed in the constant area
}
So the difference between the heap and the stack:
The stack space is automatically allocated/released by the operating system, and the heap space is manually allocated/released.
The stack has limited space, and the heap is a large free storage area.
Program compile time and function memory allocation are all done on the stack, and parameter passing when calling functions while the program is running is also done on the stack.
-------------------------------------------------- -------------------------------------------------- --
1. Heap and stack size
Define the size in startup_stm32f2xx.s
Stack_Size EQU 0x00000400
AREA STACK, NOINIT, READWRITE, ALIGN=3
Stack_Mem SPACE Stack_Size
__initial_sp
; Heap Configuration
; Heap Size (in Bytes) <0x0-0xFFFFFFFF:8>
;
Heap_Size EQU 0x00000200
AREA HEAP, NOINIT, READWRITE, ALIGN=3
__heap_base
2. Heap and stack locations
From the MAP file, we can know
HEAP 0x200106f8 Section 512 startup_stm32f2xx.o(HEAP)
STACK 0x200108f8 Section 1024 startup_stm32f2xx.o(STACK)
__heap_base 0x200106f8 Data 0 startup_stm32f2xx.o(HEAP)
__heap_limit 0x200108f8 Data 0 startup_stm32f2xx.o(HEAP)
__initial_sp 0x20010cf8 Data 0 startup_stm32f2xx.o(STACK)
Obviously, from the Cortex-m3 documentation, __initial_sp is the stack pointer, which is the first 4 bytes of the 0x8000000 address of FLASH (it is automatically generated by the compiler based on the stack size)
Obviously the heap and stack are adjacent.
3. Heap and stack space allocation
Stack: Expanding to lower addresses
Heap: Expanding to higher addresses
Obviously, if you define variables in sequence
The memory address of the stack variable defined first is larger than the memory address of the stack variable defined later.
The memory address of the heap variable defined first is smaller than the memory address of the heap variable defined later
4. Heap and stack variables
Stack: Temporary variables, which are automatically released when exiting the scope
Heap: malloc variables, released by free function
In addition: Stack overflow, compilation will not prompt, need to pay attention
-------------------------------------------------- -------------------------------------------------- --
If HEAP is used, the HEAP size must be set.
If it is STACK, it can be set to 0, which will not affect the program operation.
IAR STM8 defines STACK, which is a one-byte area allocated at the end of RAM as a stack reserved area.
When the program static variables, global variables, or heap conflict with the reserved stack area, the compiler will report an error when connecting.
You can set STACK to 0, which will not affect the operation. (It will affect debugging, and debugging will report a stack overflow warning).
In fact, there is no need to do this.
For general programs, setting STACK (within the allowed range) does not affect the actual RAM size used by the program.
(You can test it, set STACK to a certain amount, and the compiled HEX files are the same),
the program still uses RAM according to its original state. Setting STACK to 0 does not really reduce RAM usage.
It just deceives the compiler and makes the program appear to use less RAM on the surface.
Setting a certain size STACK does not really use more RAM, but just allows the compiler to help you
check whether it can ensure that the RAM of that size is not occupied and can be used as a stack.
The above is only for IAR STM8.
-------------------------------------------------- -------------------------------------------------- --
From the above web excerpt, it can be seen that the heap and stack of the MCU are allocated in RAM, which may be internal or external, and can be read and written;
Stack: stores temporary variables of a function, i.e. local variables. When a function returns, it may be used by other functions at any time. Therefore, the stack is a storage area that is used in turn in a time-sharing manner.
The Stack_Size defined in the compiler is to limit the range of local data activities of a function. If the range is exceeded, it may run away, that is, the stack overflows;
Stack_Size does not affect Hex, nor does it affect how Hex runs. It just prompts an error during debugging. Stack overflow also occurs when it exceeds the national boundary.
Activities, as long as the foreigners have no objection, you can continue to play. If the foreigners don't let you play, you will die, or everyone will die (fighting each other). Some people write
The microcontroller code defines a large array int buf[8192] in the function. If the stack is less than 8192, it will die miserably.
Heap: stores global variables, which are theoretically accessible to all functions. Some global variables have initial values, but these values are not stored in RAM.
It is stored in Hex, downloaded to Flash, and moved to it by code (assembler code generated by the compiler) when powered on. Some people are very "domineering" and occupy a large area when powered on.
Large RAM (Heap_Size) is owned by the user (malloc_init), and others can only borrow it from the user's manager (malloc), and must exchange it after use (free).
Once a "domineering" person appears, Heap_Size must be defined in the compiler, otherwise it is useless to borrow from his housekeeper.
In short: the heap and stack are stored in RAM, and the amount of each depends on the function requirements, but the total value of the two cannot exceed the actual RAM size of the microcontroller hardware, otherwise it can only
Go play in the sea (drowned) or build your own boat and play (expand RAM).
Previous article:Some questions about using STM32-PB3 pins
Next article:STM32 stack size details and variable storage location
Recommended ReadingLatest update time:2024-11-16 16:01
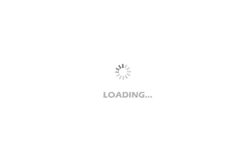
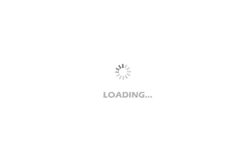
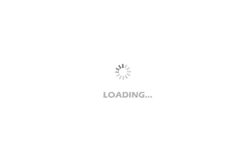
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Please help! The ADC sampling fluctuation in stm32h743 is very large, and the 16-bit resolution exceeds the 8-bit fluctuation
- Apply for ufun learning board, introductory & deep learning simulation, engineering skills
- I downloaded a source code from the Internet. Please help me find out what IDE this project was developed in. Thanks
- X-NUCLEO-IKS01A3 MakeCode Driver (Extension) Collection
- This strange circuit
- EEWORLD University ---- test
- What is ModBus? What are the differences and connections with RS485 protocol?
- Is this speed too fast? I have never experienced it properly.
- Application Differences between HT8691 and HT8691R
- SimpleLink MCU code migration guide: CC1310 from VQFN48 (7×7) to VQFN32 (5×5) code migration process reference