1. Introduction to IWDG:
STM32 has two watchdogs, one is an independent watchdog and the other is a window watchdog. The independent watchdog is known as a pet dog, and the window watchdog is known as a police dog. In this chapter, we mainly analyze the functional block diagram and application of the independent watchdog. In layman's terms, the independent watchdog is a 12-bit down counter. When the value of the counter decreases from a certain value to 0, the system will generate a reset signal, namely IWDG_RESET. If the value of the counter is refreshed before the count is reduced to 0, no reset signal will be generated. This action is what we often call feeding the dog. The watchdog function is powered by the VDD voltage domain and can still work in stop mode and standby mode.
2. Analysis of IWDG functional block diagram
①Independent watchdog clock
The clock of the independent watchdog is provided by an independent RC oscillator LSI. Even if the main clock fails, it is still effective and very independent. The frequency of LSI is generally between 30~60KHZ. There will be a certain drift depending on the temperature and working environment. We generally use 40KHZ, so the timing of the independent watchdog is not necessarily very accurate and is only suitable for occasions with relatively low time accuracy requirements.
②Counter clock
The clock of the down counter is obtained by LSI through an 8-bit prescaler. We can operate the prescaler register IWDG_PR to set the division factor. The division factor can be: [4,8,16,32,64,128,256,256]. The counter clock CK_CNT = 40/ 4*2^PRV. The counter is reduced by one for each counter clock.
③Counter
The counter of the independent watchdog is a 12-bit down counter with a maximum value of 0XFFF. When the counter is reduced to 0, a reset signal: IWDG_RESET is generated to restart the program. If the counter value is refreshed before the counter is reduced to 0, no reset signal will be generated. The action of refreshing the counter value is commonly known as feeding the dog.
④Reload register
The reload register is a 12-bit register that contains the value to be refreshed to the counter. The size of this value determines the overflow time of the independent watchdog. Timeout time Tout = (4*2^prv) / 40 * rlv (s), prv is the value of the prescaler register, and rlv is the value of the reload register.
⑤Key value register
The key value register IWDG_KR can be said to be a control register of the independent watchdog. There are three main control methods. Writing the following three different values to this register has different effects.
Starting the watchdog by writing 0XCCC to the key register is a software startup method. Once the independent watchdog is started, it cannot be turned off and can only be turned off by resetting.
⑥Status register
Only bit 0: PVU and bit 1: RVU are valid in the status register SR. These two bits can only be operated by hardware, not software. RVU: Watchdog counter reload value update. Hardware set to 1 indicates that the reload value update is in progress. After the update is completed, it is cleared to 0 by hardware. PVU: Watchdog prescaler value update. Hardware set to '1' indicates that the prescaler value update is in progress. After the update is completed, it is cleared to 0 by hardware. Therefore, the reload register/prescaler register can only be updated when RVU/PVU is equal to 0.
3. How to use IWDG
Independent watchdogs are generally used to detect and resolve faults caused by programs. For example, the normal running time of a program is 50ms. After running this segment of the program, the watchdog is fed immediately. We set the independent watchdog's timing overflow time to 60ms, which is a little longer than the 50ms of the program we need to monitor. If the watchdog is not fed within 60ms, it means that the program we are monitoring has failed and run away, then a system reset will be generated to allow the program to run again.
4. IWDG overtime experiment
hardware design:
1-IWDG, which is an internal resource and does not require external hardware
2-KEY One
3-LED two, use the RGB lights that come with the development board
experimental design
The timeout period of IWDG is configured to be 1S. If the dog is not fed within 1S, the system will be reset and indicated by the status change of the LED light.
Programming Tips
1- How to configure the timeout of IWDG?
2-How to write a dog feeding function?
3-Where is the appropriate place to feed the dog in the main function?
Configure the IWDG timeout period.
/*
* Set the timeout period of IWDG
* All = prv/40 * rlv(s)
* prv can be [4,8,16,32,64,128,256]
* prv: prescaler value, the value is as follows:
* @arg IWDG_Prescaler_4: IWDG prescaler set to 4
* @arg IWDG_Prescaler_8: IWDG prescaler set to 8
* @arg IWDG_Prescaler_16: IWDG prescaler set to 16
* @arg IWDG_Prescaler_32: IWDG prescaler set to 32
* @arg IWDG_Prescaler_64: IWDG prescaler set to 64
* @arg IWDG_Prescaler_128: IWDG prescaler set to 128
* @arg IWDG_Prescaler_256: IWDG prescaler set to 256
*
* Independent watchdog uses LSI as clock.
* The frequency of LSI is generally between 30~60KHZ, and there will be a certain drift depending on the temperature and working environment.
* Generally, we use 40KHZ, so the timing of the independent watchdog is not necessarily very accurate, and is only suitable for time accuracy.
* For occasions with relatively low requirements.
*
* rlv: reload register value, value range: 0-0XFFF
* Function call example:
* IWDG_Config(IWDG_Prescaler_64, 625); // IWDG 1s timeout overflow
* (64/40)*625 = 1s
*/
void IWDG_Config(uint8_t prv ,uint16_t rlv)
{
// Enable the prescaler register PR and reload register RLR to be writable
IWDG_WriteAccessCmd( IWDG_WriteAccess_Enable );
// Set the prescaler value
IWDG_SetPrescaler( prv );
// Set the reload register value
IWDG_SetReload( rlv );
// Put the value of the reload register into the counter
IWDG_ReloadCounter();
// Enable IWDG
IWDG_Enable();
}
Feed the dog function:
// Feed the dog
void IWDG_Feed(void)
{
// Put the value of the reload register into the counter, feed the dog, and prevent IWDG from resetting
// When the counter value reaches 0, the system will be reset
IWDG_ReloadCounter();
}
#include "stm32f4xx.h"
#include "./led/bsp_led.h"
#include "./key/bsp_key.h"
#include "./iwdg/bsp_iwdg.h"
static void Delay(__IO u32 nCount);
int main(void)
{
/* LED port initialization */
LED_GPIO_Config();
Delay(0X8FFFFF);
/* Check if it is an independent watchdog reset */
if (RCC_GetFlagStatus(RCC_FLAG_IWDGRST) != RESET)
{
/* Independent watchdog reset */
/* Turn on red light*/
LED_RED;
/* Clear flag */
RCC_ClearFlag();
/* If you don't feed the dog, it will keep resetting, plus the previous delay, you will see the red light flashing
If you feed the dog within 1 second, the green light will remain on*/
}
else
{
/*Not an independent watchdog reset (maybe a power-on reset or manual button reset, etc.) */
/* Turn on blue light*/
LED_BLUE;
}
/*Initialize buttons*/
Key_GPIO_Config();
// IWDG 1s timeout overflow
IWDG_Config(IWDG_Prescaler_64 ,625);
//The while part is the code we need to write in the project. This part of the program can be monitored by an independent watchdog
//If we know the execution time of this part of the code, for example, 500ms, then we can set the independent watchdog
//The overflow time is 600ms, a little more than 500ms. If the program to be monitored does not run away and executes normally, then
//After the execution is completed, the program for feeding the dog will be executed. If the program runs away, the program will time out and cannot reach the point where the dog is fed.
// program, then the system will be reset. But it is not ruled out that the program ran away and ran back, just feeding the dog,
//A lucky coincidence. So if you want a more accurate monitoring program, you can use a window watchdog. The window watchdog requires
//Feed the dog within the specified window time.
while(1)
{
if( Key_Scan(KEY1_GPIO_PORT,KEY1_PIN) == KEY_ON )
{
// Feed the dog. If you don't feed the dog, the system will reset. After reset, the red light will turn on. If
// If you feed the dog on time, the green light will turn on
IWDG_Feed();
// After feeding the dog, the green light turns on
LED_GREEN;
}
}
}
static void Delay(__IO uint32_t nCount) //Simple delay function
{
for(; nCount != 0; nCount--);
}
/*********************************************END OF FILE**********************/
The program first checks whether it is an independent watchdog reset. If it is an independent watchdog reset, the red light will be on. If the watchdog is not fed, it will keep resetting. With the previous delay, you will see the red light flashing. If the watchdog is fed within 1S, the green light will continue to be on.
Previous article:STM32 AD DMA mode
Next article:STM32 IWDG dog feeding time calculation
Recommended ReadingLatest update time:2024-11-16 04:50
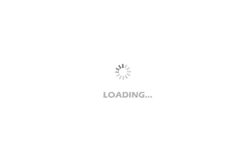
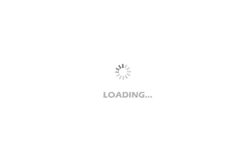
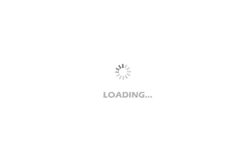
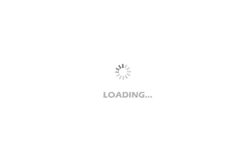
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Using STM32F107 as TCP client, connecting to the host computer TCP server program
- What is feedback? What is a feedback circuit? What does feedback do?
- EEWORLD University Hall--Detailed explanation of algorithms Stanford University classic video course
- The differences between the road voltage method, dynamic voltage method, and CEDV compensated discharge termination voltage method, as well as the specific measurement principles
- Dielectric strength test/voltage withstand test and ESD static test
- A silicon microphone was soldered with flying wires
- [Newbie Question] Basic Circuit Questions
- EEWORLD University Hall----Embedded System Theory and Technology Wuhan University of Science and Technology
- Report the problem, EE forum administrator come in and take a look!
- Please help me find out how to modify this circuit.