1. Serial port related registers
USART_SR Status Register
USART_DR Data Register
USART_BRR Baud Rate Register
USART_CR1 Control Register
USART_SR – Status Register:
Status register USART_SR, describes some states of the serial port register:
such as bit 5: read data register is not empty
by reading the value of this bit, determine whether the complete data has been received.
The serial port has received the data and has written it to the USART_DR register.
USART_DR – Data Register:
Data register USART_DR, only bits 0-8 are used, other bits are reserved
Read register: read the register to get the received data value
Write register: write the sent data to the register to send the data
USART_BRR – Baud Rate Register:
The baud rate register USART_BRR only uses the lower 16 bits, and the upper 16 bits are reserved.
0-3 bits [3:0]: the fractional part of the USART divider DIV_Fraction
4-15 bits [15:4]: the integer part of the USART divider DIV_Mantissa
USART_CR1 - Control Register:
USART_BRR baud rate register, set the serial port register enable bit
such as: receive enable, send enable
Second, the calculation method of baud rate
Baud Rate Generator:
As shown in the figure:
The baud rate is generated by the baud rate generator and PCLKx
The value of PCLKx is determined by the serial port itself
The baud rate generator value is determined by configuring the USART_BRR register
The final baud rate is obtained by dividing by 16 through the USARTDIV divider
Baud rate calculation method:
Set the baud rate of serial port 1 to 115200MHz
The clock of serial port 1 comes from PCLK2=72MHz
From the formula:
USARTDIV=72000000/(115200*16)=39.0625
Integer part DIV_Mantissa = 39 = 0x27
Fractional part DIV_Fraction = 16*0,0625 = 1 = 0x01
So setting USART->BRR=0x0271 can set the baud rate of serial port 1 to 115200MHz
3. Serial port operation related library functions
Get status flag function - operate USART_SR register
// Get the status flag
FlagStatus USART_GetFlagStatus(USART_TypeDef* USARTx, uint16_t USART_FLAG);
// Clear the status flag
void USART_ClearFlag(USART_TypeDef* USARTx, uint16_t USART_FLAG);
// Get the interrupt status flag
ITStatus USART_GetITStatus(USART_TypeDef* USARTx, uint16_t USART_IT);
// Clear the interrupt status flag
void USART_ClearITPendingBit(USART_TypeDef* USARTx, uint16_t USART_IT);
Receive and send data function - operate USART_DR register
// Send data to the serial port (send data by writing to the USART_DR register)
void USART_SendData(USART_TypeDef* USARTx, uint16_t Data);
// Receive data (read received data from USART_DR register)
uint16_t USART_ReceiveData(USART_TypeDef* USARTx);
Serial port configuration function
//Serial port initialization: baud rate, data word length, parity check, hardware flow control and transmit and receive enable
void USART_Init(USART_TypeDef* USARTx, USART_InitTypeDef* USART_InitStruct);
// Enable the serial port
void USART_Cmd(USART_TypeDef* USARTx, FunctionalState NewState);
// Enable related interrupts
void USART_ITConfig(USART_TypeDef* USARTx, uint16_t USART_IT, FunctionalState NewState);
4. Serial port hardware connection
PA9-RXD
PA10-TXD
CH340 USB to Serial Port Use USB as a virtual serial port
5. Steps of serial port configuration
1, serial port clock enable, GPIO clock enable
RCC_APB2PeriphClockCmd()
2. Serial port reset
USART_DeInit();
3. GPIO port mode setting
GPIO_Init();
4. Serial port parameter initialization
USART_Init()
5. Enable interrupt and initialize NVIC
NVIC_Init();
USART_ITConfig();
6. Enable the serial port
USART_Cmd();
7. Interrupt function logic
USARTx_IRQHandler();
8.Serial data transmission
void USART_SendData(USART_TypeDef* USARTx, uint16_t Data);
uint16_t USART_ReceiveData(USART_TypeDef* USARTx);
9. Get the serial port transmission status
ITStatus USART_GetITStatus(USART_TypeDef* USARTx, uint16_t USART_IT);
void USART_ClearITPendingBit(USART_TypeDef* USARTx, uint16_t USART_IT);
6. Serial port test program design
Program features:
The computer is connected to the development board via a USB cable, and the development board communicates with the computer via USB to serial port
The computer uses the serial port tool to send data to the microcontroller, and the microcontroller returns the data to the computer after receiving it.
Note: Take serial port 1 as an example
7. Analysis of serial port test program implementation
1. Enable GPIO clock
The sending and receiving pins of serial port 1 are PA9 and PA10
So we need to enable the clock of GPIOA and serial port 1
The clock source of serial port 1 and GPIOx is APB2
So use the RCC_APB2PeriphClockCmd function to initialize
stm32f10x_rcc.c found the RCC_APB2PeriphClockCmd function source code:
/**
* @brief Enables or disables the High Speed APB (APB2) peripheral clock.
* @param RCC_APB2Periph: specifies the APB2 peripheral to gates its clock.
* This parameter can be any combination of the following values:
* @arg RCC_APB2Periph_AFIO, RCC_APB2Periph_GPIOA, RCC_APB2Periph_GPIOB,
* RCC_APB2Periph_GPIOC, RCC_APB2Periph_GPIOD, RCC_APB2Periph_GPIOE,
* RCC_APB2Periph_GPIOF, RCC_APB2Periph_GPIOG, RCC_APB2Periph_ADC1,
* RCC_APB2Periph_ADC2, RCC_APB2Periph_TIM1, RCC_APB2Periph_SPI1,
* RCC_APB2Periph_TIM8, RCC_APB2Periph_USART1, RCC_APB2Periph_ADC3,
* RCC_APB2Periph_TIM15, RCC_APB2Periph_TIM16, RCC_APB2Periph_TIM17,
* RCC_APB2Periph_TIM9, RCC_APB2Periph_TIM10, RCC_APB2Periph_TIM11
* @param NewState: new state of the specified peripheral clock.
* This parameter can be: ENABLE or DISABLE.
* @retval None
*/
void RCC_APB2PeriphClockCmd(uint32_t RCC_APB2Periph, FunctionalState NewState)
{
/* Check the parameters */
assert_param(IS_RCC_APB2_PERIPH(RCC_APB2Periph));
assert_param(IS_FUNCTIONAL_STATE(NewState));
if (NewState != DISABLE)
{
RCC->APB2ENR |= RCC_APB2Periph;
}
else
{
RCC->APB2ENR &= ~RCC_APB2Periph;
}
}
Stm32f10x_rcc.h finds the IS_RCC_APB2_PERIPH function declaration:
/** @defgroup APB2_peripheral
* @{
*/
#define RCC_APB2Periph_AFIO ((uint32_t)0x00000001)
#define RCC_APB2Periph_GPIOA ((uint32_t)0x00000004)
#define RCC_APB2Periph_GPIOB ((uint32_t)0x00000008)
#define RCC_APB2Periph_GPIOC ((uint32_t)0x00000010)
#define RCC_APB2Periph_GPIOD ((uint32_t)0x00000020)
#define RCC_APB2Periph_GPIOE ((uint32_t)0x00000040)
#define RCC_APB2Periph_GPIOF ((uint32_t)0x00000080)
#define RCC_APB2Periph_GPIOG ((uint32_t)0x00000100)
#define RCC_APB2Periph_ADC1 ((uint32_t)0x00000200)
#define RCC_APB2Periph_ADC2 ((uint32_t)0x00000400)
#define RCC_APB2Periph_TIM1 ((uint32_t)0x00000800)
#define RCC_APB2Periph_SPI1 ((uint32_t)0x00001000)
#define RCC_APB2Periph_TIM8 ((uint32_t)0x00002000)
#define RCC_APB2Periph_USART1 ((uint32_t)0x00004000)
#define RCC_APB2Periph_ADC3 ((uint32_t)0x00008000)
#define RCC_APB2Periph_TIM15 ((uint32_t)0x00010000)
#define RCC_APB2Periph_TIM16 ((uint32_t)0x00020000)
#define RCC_APB2Periph_TIM17 ((uint32_t)0x00040000)
#define RCC_APB2Periph_TIM9 ((uint32_t)0x00080000)
#define RCC_APB2Periph_TIM10 ((uint32_t)0x00100000)
#define RCC_APB2Periph_TIM11 ((uint32_t)0x00200000)
#define IS_RCC_APB2_PERIPH(PERIPH) ((((PERIPH) & 0xFFC00002) == 0x00) && ((PERIPH) != 0x00))
From the parameter definition, it is verified that the clock enable of GPIOA-GPIOG and serial port 1 (USART1) is controlled by RCC_APB2PeriphClockCmd()
So the code to enable GPIOA and serial port 1 clock is:
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE); //Enable GPIOA clock source
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1, ENABLE); //Enable serial port 1 clock source
2. Initialize the working mode of GPIOA
Determine the working mode configuration of the serial port 1 pin by looking up the STM32 Chinese reference manual:
As shown in the figure:
Serial port 1 receiving and sending pin configuration
The transmitting end PA9 is configured as push-pull multiplex output
The receiving end PA10 is configured as floating input or pull-up input
Code:
GPIO_InitTypeDef GPIO_InitStrue;
//Sender PA9 configuration
GPIO_InitStrue.GPIO_Pin=GPIO_Pin_9; //Transmitter-TXD
GPIO_InitStrue.GPIO_Mode=GPIO_Mode_AF_PP; //Push-pull output
GPIO_InitStrue.GPIO_Speed=GPIO_Speed_10MHz;
GPIO_Init(GPIOA, &GPIO_InitStrue);
//Receiver PA10 configuration
GPIO_InitStrue.GPIO_Pin=GPIO_Pin_10; //Receiver-RXD
GPIO_InitStrue.GPIO_Mode=GPIO_Mode_IN_FLOATING; //Floating input
GPIO_InitStrue.GPIO_Speed=GPIO_Speed_10MHz;
GPIO_Init(GPIOA, &GPIO_InitStrue);
3.Serial port initialization
Find the USART_Init function declaration in the stm32f10x_usart.h header file:
void USART_Init(USART_TypeDef* USARTx, USART_InitTypeDef* USART_InitStruct);
stm32f10x_usart.h finds the USART_InitTypeDef structure declaration
/**
* @brief USART Init Structure definition
*/
typedef struct
{
uint32_t USART_BaudRate; //Set the baud rate
uint16_t USART_WordLength; // word length 8 or 9 (stop bits)
uint16_t USART_StopBits; // Stop bit
uint16_t USART_Parity; // Parity check
uint16_t USART_Mode; // Enable sending and receiving
uint16_t USART_HardwareFlowControl; // Hardware flow control
} USART_InitTypeDef;
USART_HardwareFlowControl - Hardware flow parameter validity verification
/** @defgroup USART_Hardware_Flow_Control
* @{
*/
#define USART_HardwareFlowControl_None ((uint16_t)0x0000)
#define USART_HardwareFlowControl_RTS ((uint16_t)0x0100)
#define USART_HardwareFlowControl_CTS ((uint16_t)0x0200)
#define USART_HardwareFlowControl_RTS_CTS ((uint16_t)0x0300)
#define IS_USART_HARDWARE_FLOW_CONTROL(CONTROL)\
(((CONTROL) == USART_HardwareFlowControl_None) || \
((CONTROL) == USART_HardwareFlowControl_RTS) || \
((CONTROL) == USART_HardwareFlowControl_CTS) || \
((CONTROL) == USART_HardwareFlowControl_RTS_CTS))
USART_Mode - Enable parameter validity verification
/** @defgroup USART_Mode
* @{
*/
#define USART_Mode_Rx ((uint16_t)0x0004) #define USART_Mode_Tx ((uint16_t)0x0008) #define IS_USART_MODE(MODE) ((((MODE) & (uint16_t)0xFFF3) == 0x00) && ((MODE) != (uint16_t)0x00))
USART_Parity - Parity parameter validity
/** @defgroup USART_Parity
* @{
*/
#define USART_Parity_No ((uint16_t)0x0000) #define USART_Parity_Even ((uint16_t)0x0400) #define USART_Parity_Odd ((uint16_t)0x0600) #define IS_USART_PARITY(PARITY) (((PARITY) == USART_Parity_No) || \ ((PARITY) == USART_Parity_Even) || \ ((PARITY) == USART_Parity_Odd))
USART_StopBits - Stop bits parameter validity
/** @defgroup USART_Stop_Bits
* @{
*/
#define USART_StopBits_1 ((uint16_t)0x0000)
#define USART_StopBits_0_5 ((uint16_t)0x1000)
#define USART_StopBits_2 ((uint16_t)0x2000)
#define USART_StopBits_1_5 ((uint16_t)0x3000)
#define IS_USART_STOPBITS(STOPBITS) (((STOPBITS) == USART_StopBits_1) || \
((STOPBITS) == USART_StopBits_0_5) || \
((STOPBITS) == USART_StopBits_2) || \
((STOPBITS) == USART_StopBits_1_5))
USART_WordLength - word length parameter validity
/** @defgroup USART_Word_Length
* @{
*/
#define USART_WordLength_8b ((uint16_t)0x0000) #define USART_WordLength_9b ((uint16_t)0x1000) #define IS_USART_WORD_LENGTH(LENGTH) (((LENGTH) == USART_WordLength_8b) || \ ((LENGTH) == USART_WordLength_9b))
Serial port initialization code:
USART_InitTypeDef USART_InitStrue;
USART_InitStrue.USART_BaudRate=115200; //Set baud rate - 115200MHz
USART_InitStrue.USART_HardwareFlowControl=USART_HardwareFlowControl_None; //Hardware flow control - not used
USART_InitStrue.USART_Mode=USART_Mode_Rx| USART_Mode_Tx; //Enable setting - both sending and receiving are enabled
USART_InitStrue.USART_Parity=USART_Parity_No; //Parity check - do not use parity check
USART_InitStrue.USART_StopBits=USART_StopBits_1; //Stop bit - one stop bit
USART_InitStrue.USART_WordLength=USART_WordLength_8b //Word length - 8 bits
USART_Init(USART1, &USART_InitStrue);
4. Enable serial port 1:
Find the USART_Cmd function definition in the stm32f10x_usart.h header file
void USART_Cmd(USART_TypeDef* USARTx, FunctionalState NewState);
USART_Cmd(USART1, ENABLE);
5. Since interrupts are used, you must first configure the interrupt priority grouping - in the main function
Find the NVIC_PriorityGroupConfig function declaration in the misc.h header file:
void NVIC_PriorityGroupConfig(uint32_t NVIC_PriorityGroup);
1
1
Find the NVIC_PriorityGroupConfig function implementation in misc.c:
void NVIC_PriorityGroupConfig(uint32_t NVIC_PriorityGroup)
{
/* Check the parameters */
assert_param(IS_NVIC_PRIORITY_GROUP(NVIC_PriorityGroup));
/* Set the PRIGROUP[10:8] bits according to NVIC_PriorityGroup value */
SCB->AIRCR = AIRCR_VECTKEY_MASK | NVIC_PriorityGroup;
}
Check the validity of the parameter IS_NVIC_PRIORITY_GROUP - misc.h
#define NVIC_PriorityGroup_0 ((uint32_t)0x700) /*!< 0 bits for pre-emption priority
4 bits for subpriority */
#define NVIC_PriorityGroup_1 ((uint32_t)0x600) /*!< 1 bits for pre-emption priority
3 bits for subpriority */
#define NVIC_PriorityGroup_2 ((uint32_t)0x500) /*!< 2 bits for pre-emption priority
2 bits for subpriority */
#define NVIC_PriorityGroup_3 ((uint32_t)0x400) /*!< 3 bits for pre-emption priority
1 bits for subpriority */
#define NVIC_PriorityGroup_4 ((uint32_t)0x300) /*!< 4 bits for pre-emption priority
0 bits for subpriority */
#define IS_NVIC_PRIORITY_GROUP(GROUP) (((GROUP) == NVIC_PriorityGroup_0) || \
((GROUP) == NVIC_PriorityGroup_1) || \
((GROUP) == NVIC_PriorityGroup_2) || \
((GROUP) == NVIC_PriorityGroup_3) || \
((GROUP) == NVIC_PriorityGroup_4))
Interrupt group configuration code:
//Configure the interrupt group to 2, that is, 2-bit preemption priority and 2-bit response priority
NVIC_PriorityGroupConfig(NVIC_PriorityGroup_2)
6. Enable receive interrupt
Find the USART_ITConfig function declaration in the stm32f10x_usart.h header file:
void USART_ITConfig(USART_TypeDef* USARTx, uint16_t USART_IT, FunctionalState NewState);
Find the USART_ITConfig function implementation in stm32f10x_usart.c:
void USART_ITConfig(USART_TypeDef* USARTx, uint16_t USART_IT, FunctionalState NewState)
{
uint32_t usartreg = 0x00, itpos = 0x00, itmask = 0x00;
uint32_t usartxbase = 0x00;
/* Check the parameters */
assert_param(IS_USART_ALL_PERIPH(USARTx));
assert_param(IS_USART_CONFIG_IT(USART_IT));
assert_param(IS_FUNCTIONAL_STATE(NewState));
/* The CTS interrupt is not available for UART4 and UART5 */
if (USART_IT == USART_IT_CTS)
{
assert_param(IS_USART_123_PERIPH(USARTx));
}
usartxbase = (uint32_t)USARTx;
/* Get the USART register index */
usartreg = (((uint8_t)USART_IT) >> 0x05);
/* Get the interrupt position */
itpos = USART_IT & IT_Mask;
itmask = (((uint32_t)0x01) << itpos);
if (usartreg == 0x01) /* The IT is in CR1 register */
{
usartxbase += 0x0C;
}
else if (usartreg == 0x02) /* The IT is in CR2 register */
{
usartxbase += 0x10;
}
else /* The IT is in CR3 register */
{
usartxbase += 0x14;
}
if (NewState != DISABLE)
{
*(__IO uint32_t*)usartxbase |= itmask;
}
else
{
*(__IO uint32_t*)usartxbase &= ~itmask;
}
}
The validity check of the second parameter USART_IT is IS_USART_CONFIG_IT:
Find the IS_USART_CONFIG_IT declaration in the stm32f10x_usart.h header file:
#define USART_IT_PE ((uint16_t)0x0028)
#define USART_IT_TXE ((uint16_t)0x0727)
#define USART_IT_TC ((uint16_t)0x0626)
#define USART_IT_RXNE ((uint16_t)0x0525)
#define USART_IT_IDLE ((uint16_t)0x0424)
#define USART_IT_LBD ((uint16_t)0x0846)
#define USART_IT_CTS ((uint16_t)0x096A)
#define USART_IT_ERR ((uint16_t)0x0060)
#define USART_IT_ORE ((uint16_t)0x0360)
#define USART_IT_NE ((uint16_t)0x0260)
#define USART_IT_FE ((uint16_t)0x0160)
#define IS_USART_CONFIG_IT(IT) (((IT) == USART_IT_PE) || ((IT) == USART_IT_TXE) || \
((IT) == USART_IT_TC) || ((IT) == USART_IT_RXNE) || \
((IT) == USART_IT_IDLE) || ((IT) == USART_IT_LBD) || \
((IT) == USART_IT_CTS) || ((IT) == USART_IT_ERR))
Serial port 1 initialization code:
//Open the receive interrupt of serial port 1. This interrupt will be triggered when serial port 1 receives data.
USART_ITConfig(USART1, USART_IT_RXNE, ENABLE);
6. Setting interrupt priority
Initialize NVIC, set interrupt preemption priority and response priority
Reference:NVIC
NVIC_InitTypeDef NVIC_InitTypeStrue;
NVIC_InitTypeStrue.NVIC_IRQChannel=USART1_IRQn; // Which channel - stm32f10x.h top-level header file contains parameter definitions
NVIC_InitTypeStrue.NVIC_IRQChannelCmd=ENABLE; //Whether to enable the interrupt channel - enable
NVIC_InitTypeStrue.NVIC_IRQChannelPreemptionPriority=1; // Preemption priority
NVIC_InitTypeStrue.NVIC_IRQChannelSubPriority=1; // Response priority, sub-priority
NVIC_Init(&NVIC_InitTypeStrue);
7. Write interrupt service function
Format: USARTx_IRQHandler();
Find the interrupt service function of serial port 123 in the startup file startup_stm32f10x_hd.s
DCD USART1_IRQHandler ; USART1
DCD USART2_IRQHandler ; USART2
DCD USART3_IRQHandler ; USART3
Interrupt service function code
void USART1_IRQHandler(void){
u8 res;
// Determine the interrupt type
//Parameter 1: which serial port Parameter 2: interrupt type
if(USART_GetITStatus(USART1, USART_IT_RXNE)){//Receive data interrupt
res = USART_ReceiveData(USART1); //Read the data received by serial port 1
//Postback
USART_SendData(USART1, res);
}
}
8. Complete code of serial port program
Create a new main.c function in the USER folder:
#include "stm32f10x.h"
// Main function
int main(void)
{
// Set interrupt priority group bit 2 - 2 bits preempt 2 bits corresponding
NVIC_PriorityGroupConfig(NVIC_PriorityGroup_2);
// Call function to initialize USART1 related pin configuration
My_USART1_Init();
while(1);
}
//Serial port initialization function
void My_USART1_Init(void)
{
GPIO_InitTypeDef GPIO_InitStrue;
USART_InitTypeDef USART_InitStrue;
NVIC_InitTypeDef NVIC_InitStrue;
// 1, enable GPIOA, USART1 clock
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA,ENABLE);
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1,ENABLE);
// 2, set PGIO working mode - PA9 PA10 multiplexed as serial port 1
GPIO_InitStrue.GPIO_Mode=GPIO_Mode_AF_PP;
GPIO_InitStrue.GPIO_Pin=GPIO_Pin_9;
GPIO_InitStrue.GPIO_Speed=GPIO_Speed_10MHz;
GPIO_Init(GPIOA,&GPIO_InitStrue);
GPIO_InitStrue.GPIO_Mode=GPIO_Mode_IN_FLOATING;
GPIO_InitStrue.GPIO_Pin=GPIO_Pin_10;
GPIO_InitStrue.GPIO_Speed=GPIO_Speed_10MHz;
GPIO_Init(GPIOA,&GPIO_InitStrue);
// 3, initialization configuration of serial port 1
USART_InitStrue.USART_BaudRate=115200;
USART_InitStrue.USART_HardwareFlowControl=USART_HardwareFlowControl_None;
USART_InitStrue.USART_Mode=USART_Mode_Tx|USART_Mode_Rx;
USART_InitStrue.USART_Parity=USART_Parity_No;
USART_InitStrue.USART_StopBits=USART_StopBits_1;
USART_InitStrue.USART_WordLength=USART_WordLength_8b;
USART_Init(USART1,&USART_InitStrue);
// 4, open serial port 1
USART_Cmd(USART1,ENABLE);
// 5, enable serial port 1 interrupt - receive data complete interrupt
USART_ITConfig(USART1,USART_IT_RXNE,ENABLE);
// 6, set interrupt priority - set interrupt priority group in main function
NVIC_InitStrue.NVIC_IRQChannel=USART1_IRQn;
NVIC_InitStrue.NVIC_IRQChannelCmd=ENABLE;
NVIC_InitStrue.NVIC_IRQChannelPreemptionPriority=1;
NVIC_InitStrue.NVIC_IRQChannelSubPriority=1;
NVIC_Init(&NVIC_InitStrue);
}
//Interrupt service function
void USART1_IRQHandler(void)
{
u8 res;
if(USART_GetITStatus(USART1,USART_IT_RXNE))//Received data
{
res= USART_ReceiveData(USART1); // Get the data received by serial port 1
USART_SendData(USART1,res); //Send data through serial port 1
}
}
The above code implements:
The computer sends data to the microcontroller through the serial port assistant
The microcontroller receives data and enters the receiving data serial port interrupt
Read the received data in the DR register
The received data is then written back to the computer through the serial port
(function () {(function () {('pre.prettyprint code').each(function () { var lines = (this).text().split(′\n′).length;var(this).text().split(′\n′).length;varnumbering = $('
').addClass('pre-numbering').hide(); (this).addClass(′has−numbering′).parent().append((this).addClass(′has−numbering′).parent().append(numbering); for (i = 1; i
Previous article:Several common reasons for serious abnormalities of stm32 functions
Next article:STM32 MDK common errors and solutions
Recommended ReadingLatest update time:2024-11-16 18:06
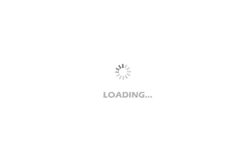
![[STM32 motor square wave] Record 2——NVIC interrupt basic settings](https://6.eewimg.cn/news/statics/images/loading.gif)
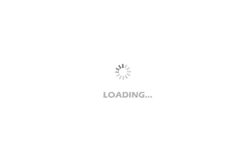
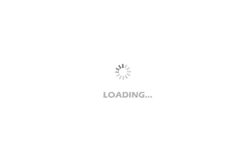
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- It's Friday. Let's do a little reasoning
- Why should rectifier filter capacitors use high frequency and low resistance?
- Counting TI's star products in T-BOX: linear regulators | Section 5: TPS7B7701-Q1: Safeguarding automotive applications
- GD32E230C development board download error
- About the steps of converting .brd to .PCB
- In the LLC circuit, when the operating frequency fs is less than the resonant frequency fr, there is a problem with the secondary rectifier diode voltage waveform.
- bq40z50 series thermistor resistance coefficient calculator
- How should a fast charging decoy be evaluated?
- The hierarchical wordline structure can improve the SRAM read and write speed and reduce the circuit dynamic power consumption
- Bluehive Bluetooth headset charging case disassembly