STM32 Study Notes (VI) ----TIM (to be supplemented)
1. Enable TIM clock
RCC_APB1PeriphClockCmd(RCC_APB1Periph_TIM*,ENABLE);
2. Basic Settings
TIM_TimeBaseStructure.TIM_Period count value
TIM_TimeBaseStructure.TIM_Prescaler pre-divided frequency, this value + 1 is the divisor of the frequency division
TIM_TimeBaseStructure.TIM_ClockDivision = 0 The clock factor is to be further explained
TIM_TimeBaseStructure.TIM_RepetitionCounter = 0 To be further explained
TIM_TimeBaseStructure.TIM_CounterMode = TIM_CounterMode_Up Count up
TIM_CounterMode_Dowm Count down
TIM_CounterMode_CenterAligned1 Center alignment 1
TIM_CounterMode_CenterAligned2 Center alignment 2
TIM_CounterMode_CenterAligned3 Center alignment 3
TIM_TimeBaseInit(TIM2, &TIM_TimeBaseStructure);
3. Channel settings
----------------------------------------------------------------------------------------------
Output Compare & PWM Channels
TIM_OCInitStructure.TIM_OCMode = TIM_OCMode_Timing Output comparison timing mode (output pin freeze is invalid)
TIM_OCMode_Active Output compare active mode (set the output pin to a valid level when matching, and force the output to a high level when the count value is the same as the compare/capture register value)
TIM_OCMode_Inactive; Output comparison inactive mode (set the output pin to an invalid level when matching, and force the output to a low level when the count value is the same as the compare/capture register value)
TIM_OCMode_Toggle Output compare trigger mode (toggle. When the count value is the same as the compare/capture register value, the output pin level is toggled)
When TIM_OCMode_PWM1 counts up, when TIMx_CNT < TIMx_CCR*, the output level is valid, otherwise it is invalid
When counting down, when TIMx_CNT > TIMx_CCR*, the output level is invalid, otherwise it is valid
TIM_OCMode_PWM2 is opposite to PWM1 mode
TIM_OCInitStructure.TIM_OutputState = TIM_OutputState_Disable Disable OC* output
TIM_OutputState_Enable turns on OC* output to the corresponding pin
TIM_OCInitStructure.TIM_OutputNState = TIM_OutputNState_Disable Complementary output enable. Disable OC*N output
TIM_OutputNState_Enable Complementary output enable. Turn on OC*N output to the corresponding pin
TIM_OCInitStructure.TIM_Pulse compare/PWM channel value
TIM_OCInitStructure.TIM_OCPolarity = TIM_OCPolarity_High; The polarity is positive
TIM_OCPolarity_Low must be negative
TIM_OCInitStructure.TIM_OCNPolarity = TIM_OCNPolarity_High; polarity is positive
TIM_OCNPolarity_Low is always negative
TIM_OCInitStructure.TIM_OCIdleState = TIM_OCIdleState_Set When MOE=0, if OC*N is implemented, OC*=1 after the dead zone
TIM_OCIdleState_Reset When MOE=0, if OC*N is implemented, OC*=0 after the dead zone
TIM_OCInitStructure.TIM_OCNIdleState = TIM_OCIdleNState_Set When MOE=0, OC*N=1 after dead zone
TIM_OCIdleNState_Reset When MOE=0, OC*N=0 after dead zone
TIM_OC1Init(TIM2, &TIM_OCInitStructure);
TIM_OC1PreloadConfig(TIM2, TIM_OCPreload_Disable); Disable OC1 reload, that is, the number of TIM*_CCR* takes effect immediately after being written, otherwise it will be loaded into the register after the next update event arrives
TIM_CtrlPWMOutputs(TIM1,ENABLE); If PWM mode is used, this sentence must not be omitted.
----------------------------------------------------------------------------------------------
Input capture channel
TIM_ICInitStructure.TIM_Channel = TIM_Channel_1
TIM_Channel_2
TIM_Channel_3
TIM_Channel_4
TIM_ICInitStructure.TIM_ICPolarity = TIM_ICPolarity_Rising Input/capture rising edge is valid
TIM_ICPolarity_Falling Input/capture falling edge is valid
TIM_ICInitStructure.TIM_ICSelection = TIM_ICSelection_DirectTI IC* input pin selection, different definitions for IC1/IC2
TIM_ICSelection_IndirectTI
TIM_ICSelection_TRC
TIM_ICInitStructure.TIM_ICPrescaler = TIM_ICPSC_DIV1 In input mode, each edge on the capture port triggers a capture
In TIM_ICPSC_DIV2 input mode, every 2 events trigger a capture
TIM_ICPSC_DIV4 In input mode, every 4 events trigger a capture
In TIM_ICPSC_DIV8 input mode, every 8 events trigger a capture
TIM_ICInitStructure.TIM_ICFilter = Capture sampling frequency, see TIM*_CCMR->IC*F description for details
----------------------------------------------------------------------------------------------
Dead zone setting
TIM_BDTRInitStructure.TIM_OSSRState = TIM_OSSRState_Enable
TIM_OSSRState_Disable
TIM_BDTRInitStructure.TIM_OSSRIState = TIM_OSSRIState_Enable
TIM_OSSRIState_Disable
TIM_BDTRInitStructure.TIM_LOCKLevel = TIM_LOCKLevel_OFF
TIM_LOCKLevel_1
TIM_LOCKLevel_2
TIM_LOCKLevel_3
TIM_BDTRInitStructure.TIM_DeadTime = adjust the dead zone size here 0-0xff
TIM_BDTRInitStructure.TIM_Break = TIM_Break_Enable
TIM_Break_Disable
TIM_BDTRInitStructure.TIM_BreakPolarity = TIM_BreakPolarity_Low
TIM_BreakPolarity_High
TIM_BDTRInitStructure.TIM_AutomaticOutput= TIM_AutomaticOutput_Enable
TIM_AutomaticOutPut_Disable
4. Placement interruption
5. Enable TIM
----------------------------------------------------------------------------------------------------
example:
TIM_TimeBaseInitTypeDef TIM_TimeBaseStructure;
TIM_OCInitTypeDef TIM_OCInitStructure;
u16 CCR1_Val = 60000;
u16 CCR2_Val = 40000;
u16 CCR3_Val = 20000;
u16 CCR4_Val = 10000;
/* TIM2 clock enable */
RCC_APB1PeriphClockCmd(RCC_APB1Periph_TIM2, ENABLE);
/* Basic Settings*/
TIM_TimeBaseStructure.TIM_Period = 65535; //Count value
TIM_TimeBaseStructure.TIM_Prescaler = 7200-1; //Pre-division, this value + 1 is the divisor of the division
TIM_TimeBaseStructure.TIM_ClockDivision = 0x0; //
TIM_TimeBaseStructure.TIM_CounterMode = TIM_CounterMode_Up; //Count up
TIM_TimeBaseInit(TIM2, &TIM_TimeBaseStructure);
/* Compare channel 1 */
TIM_OCInitStructure.TIM_OCMode = TIM_OCMode_Inactive; //Output comparison inactive mode
TIM_OCInitStructure.TIM_Pulse = CCR1_Val;
TIM_OCInitStructure.TIM_OCPolarity = TIM_OCPolarity_High; //Polarity is positive
TIM_OC1Init(TIM2, &TIM_OCInitStructure);
TIM_OC1PreloadConfig(TIM2, TIM_OCPreload_Disable); //Disable OC1 reload. Actually, you can omit this sentence because the default is that all 4 channels are not reloaded.
/*Compare channel 2 */
TIM_OCInitStructure.TIM_Pulse = CCR2_Val;
TIM_OC2Init(TIM2, &TIM_OCInitStructure);
TIM_OC2PreloadConfig(TIM2, TIM_OCPreload_Disable);
/* Compare channel 3 */
TIM_OCInitStructure.TIM_Pulse = CCR3_Val;
TIM_OC3Init(TIM2, &TIM_OCInitStructure);
TIM_OC3PreloadConfig(TIM2, TIM_OCPreload_Disable);
/* Compare channel 4 */
TIM_OCInitStructure.TIM_Pulse = CCR4_Val;
TIM_OC4Init(TIM2, &TIM_OCInitStructure);
TIM_OC4PreloadConfig(TIM2, TIM_OCPreload_Disable);
/* Enable preloading */
TIM_ARRPreloadConfig(TIM2, ENABLE);
/* Clear all interrupt bits beforehand */
TIM_ClearITPendingBit(TIM2, TIM_IT_CC1 | TIM_IT_CC2 | TIM_IT_CC3 | TIM_IT_CC4|TIM_IT_Update);
/* Configure interrupts for all 4 channels and overflow*/
TIM_ITConfig(TIM2, TIM_IT_CC1 | TIM_IT_CC2 | TIM_IT_CC3 | TIM_IT_CC4|TIM_IT_Update, ENABLE);
/* Allow TIM2 to start counting */
TIM_Cmd(TIM2, ENABLE);
Previous article:STM32+FATFS file system continuously writes content to the same txt file
Next article:32DMA parameter setting in stm
Recommended ReadingLatest update time:2024-11-16 22:41
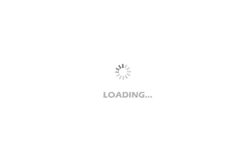
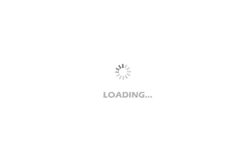
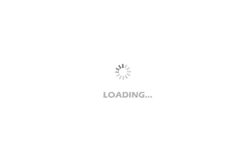
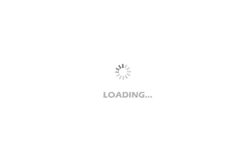
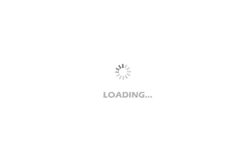
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- 【Silicon Labs Development Kit Review】+PG22 Hardware Resources
- "Play Board" + Shared Bicycle Control Panel-Hardware Modification
- MSP430fr6989 serial port DMA sending experimental routine
- Live FAQ|Microchip's Trusted Platform for CryptoAuthentication Series
- 【Qinheng Trial】7. TouchKey
- Former Chairman explains the new PCIe5.0 specification. Tektronix invites you to watch and win prizes
- Today I thought of the "collection economy", office workers are very busy
- In adjustment
- RISC-V MCU Development (Part 14): Help and Feedback
- Don’t get lost in power supply design by reading “Selected Basic Knowledge of Power Supply Design” which can be downloaded for free!