1. Development board button settings
In addition to the reset button, four buttons are designed on the "STM32-PZ6806L" development board, marked as "UP", "DOWN", "LEFT" and "RIGHT". The circuit is as follows:
According to the circuit connection, one end of the K_UP button is connected to GPIOA_0, and the other end is connected to 3.3V through a 1KΩ resistor. Therefore, when configuring GPIOA_0, the working mode should be set to "pull-down input". When the button is released, it is a low level, and when the button is pressed, it is a high level; one end of the three buttons K_LEFT, K_DOWN and K_RIGHT are connected to GPIOE_2, GPIOE_3 and GPIOE_4 respectively, and the other end is grounded. Therefore, GPIOE_2, GPIOE_3 and GPIOE_4 should be configured as "pull-up input". When the button is released, it is a high level, and when the button is pressed, it is a low level.
2. Basic configuration of the project
This project is designed based on the music player project. When these four keys are pressed, the buzzer will emit different sounds. For the implementation of the music player project, see: Use STM32 to control the passive buzzer to play music (STM32_07)
1. Copy the pMusic project folder and rename the folder to "pKey";
2. Create a new "Key" folder under the "pKey/User" folder;
3. Use "Keil uVision5" to open the project "pMusic" in the "pKey" folder (the project name has not changed);
4. Create "key.h" and "key.c" files and save them in the "pKey/User/Key" folder;
5. Add the "key.c" file to the "User" group of the project;
6. Configure the project and add the ".\User\Key" path to the "Include Paths" in the "C/C++" tab so that the system can find it when other programs include the "key.h" header file.
3. Programming to realize button functions
1. "key.h" header file program
In the header file, define the key GPIO port macro, key pin macro, and macro for reading pin values, and define the declarations of the two functions Key_Init and ReadKey. The contents are as follows:
#ifndef __KEY__H #define __KEY__H #include "system.h" #include "stm32f10x_gpio.h" #define KEY_UP_PORT GPIOA #define KEY_OTHER_PORT GPIOE #define KEY_UP GPIO_Pin_0 #define KEY_LEFT GPIO_Pin_2 #define KEY_DOWN GPIO_Pin_3 #define KEY_RIGHT GPIO_Pin_4 //Use library function to read keystrokes #define K_UP GPIO_ReadInputDataBit(KEY_UP_PORT, KEY_UP) #define K_LEFT GPIO_ReadInputDataBit(KEY_OTHER_PORT, KEY_LEFT) #define K_DOWN GPIO_ReadInputDataBit(KEY_OTHER_PORT, KEY_DOWN) #define K_RIGHT GPIO_ReadInputDataBit(KEY_OTHER_PORT, KEY_RIGHT) void Key_Init(void); u8 ReadKey(u8 mode); #endif |
2. "key.c" program file program
The "Key_Init" function enables GPIOA and GPIOE, configures GPIOA_0 as a pull-down input mode, and configures GPIOE_2, GPIOE_3, and GPIOE_4 as pull-up input modes. The "ReadKey" function implements key scanning, and selects single scan (mode=0, a key press from being pressed to being released) and continuous scan (mode=1, a key press from being pressed to being released can be considered as multiple key presses) through the parameter "mode".
#include "key.h" #include "stm32f10x_Rcc.h" #include "SysTick.h" void Key_Init() { GPIO_InitTypeDef GPIO_mode; // Enable GPIOA and GPIOE clocks RCC_APB2PeriphClockCmd( RCC_APB2Periph_GPIOA | RCC_APB2Periph_GPIOE, ENABLE ); GPIO_mode.GPIO_Mode = GPIO_Mode_IPD; GPIO_mode.GPIO_Pin = KEY_UP; GPIO_mode.GPIO_Speed = GPIO_Speed_50MHz; GPIO_Init(KEY_UP_PORT,&GPIO_mode);
GPIO_mode.GPIO_Mode = GPIO_Mode_IPU; GPIO_mode.GPIO_Pin = KEY_DOWN|KEY_LEFT|KEY_RIGHT; GPIO_mode.GPIO_Speed = GPIO_Speed_50MHz; GPIO_Init(KEY_OTHER_PORT,&GPIO_mode); } /* mode=0--single scan mode=1--continuous scanning */ u8 ReadKey(u8 mode) { static u8 key = 1; if(key==1&&(K_UP==1||K_DOWN==0||K_LEFT==0||K_RIGHT==0)) { delay_ms(10); key = 0; if(K_UP==1) { return 1; } else if(K_DOWN==0) { return 2; } else if(K_LEFT==0) { return 3; } else if(K_RIGHT==0) { return 4; } } else if(K_UP==0&&K_DOWN==1&&K_LEFT==1&&K_RIGHT==1)//按键松开 { key = 1; } if(mode==1) { key = 1; } return 0; } |
3. "main.c" program
In the main function, first initialize SysTick, buzzer IO port, and Key button IO port, then read the buttons repeatedly, and call the Sound function in "beep.c" according to the button value to make the sound. The program is as follows:
#include "beep.h" #include "SysTick.h" #include "key.h" int main() { u8 key, i; u16 tone[] = {0,262,294,330,349}; SysTick_Init(72); BEEP_Init(); Key_Init(); while(1) { key = ReadKey(0); if(key!=0){ for(i=0; i<100;i++) Sound(tone[key]); } } } |
4. Download the hex file to the development board, and press "UP", "DOWN", "LEFT" and "RIGHT" to hear the buzzer emit the sounds of Do, Re, Mi and Fa.
Previous article:Explanation of problems caused by not understanding the STM32 GPIO mode
Next article:About 8 working modes of GPIO in STM32
Recommended ReadingLatest update time:2024-11-16 11:25
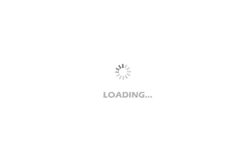
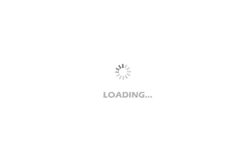
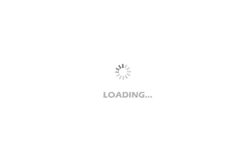
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Microchip Live Today | TA100-VAO performs secure boot and message authentication on CAN FD for ADAS and IVI systems
- 【Paper Sharing】Low-profile Balun Design for Balanced Feeding of Ultra-wideband Antennas
- Regarding MOS tube driver IC, can it only work in PWM mode?
- PCB printed circuit board design technology and practice
- [Top Micro Smart Display Screen Review] 1. Screen interface introduction and power consumption test and serial communication test
- Temperature Problems Solved for You (III) High-Performance Processor Mold Temperature Monitoring
- AD13 This box can't be found
- I wish you all success in the competition and good results!
- [NXP Rapid IoT Review] Week 1: Get familiar with kit resources
- Looking to buy ATSAMC21 series or ATSAME70 series evaluation board