S3C2440 has 2 USB host interfaces and 1 USB device interface. This article describes the USB device interface.
1 USB classification and the difference between host interface and device interface
1.1USB2.0 is divided into the following three categories according to speed
High-speed USB2.0: Theoretical speed is 480Mbps, corresponding to the previous USB2.0;
Full-speed USB2.0: Theoretical speed is 12Mbps, which is the previous USB1.1;
Low-speed USB2.0: Theoretical speed is 1.5Mbps, which is generally used for external devices such as mouse and keyboard that do not require high speed.
1.2 Differences between low-speed USB and full-speed USB hardware device interfaces
USB determines the insertion of the device through the status of the D- and D+ signals, as shown in the figure below. If D+ is connected to a pull-up resistor, it is a full-speed device, and if D- is connected to a pull-down resistor, it is a low-speed device.
1.3 Differences between MINI2440 USB device interfaces
The Mini2440 development board has only one USB host interface and one USB device interface, both of which are USB full-speed interfaces. The D+ of the Mini2440 USB device is controlled by GPC5. If GPC5 outputs a high level, D+ is equivalent to being connected to +5V through a pull-up resistor, and the device is enabled; if GPC5 outputs a low level, D+ is equivalent to being connected to GND through a pull-down resistor, and the device is disabled.
2 Analysis of the firmware enumeration process of the S3C2440USB device
2.1 S3C2440 USB device endpoints
2440 has 5 endpoints, EP0, EP1, EP2, EP3, EP4.
EP0 is used for USB device enumeration, and the transmission mode is control mode. EP1 to EP4 are used for data transmission. There are two transmission directions of endpoints, IN and OUT, which are configured by IN_CSR2_REG.
The endpoint's transmission mode, bulk, and interrupt are also configured by IN_CSR2_REG.
As can be seen from the figure above, BIT6 is used to configure the batch mode, and BIT5 is used to configure the transmission direction.
2.2 S3C2440 USB Program Analysis
The entire USB enumeration process is actually very simple. First, initialize the USB clock and set it to 48MHz; then enable GPC5 to indicate a full-speed device, then set USBD1 as a USB device and disable USB suspend. Then call UsbdMain(); to perform necessary initialization, and you are ready.
When we plug the USB port of 2440 into the host computer, a USB device reset interrupt will be generated. We perform some initialization in the device reset interrupt, and the entire enumeration enters a state machine, step by step until the enumeration is completed.
// Initialize USB device clock 48MHZ
ChangeUPllValue(0x38,2,2); // UCLK=48Mhz
// GPC5 is enabled, the output is high, indicating a full-speed device
rGPCCON &= ~(3<<10);
rGPCCON |= (1<<10); // output
rGPCUP |= (1<<5); // pullup disable
rGPCDAT |= (1<<5); // ourtput
rMISCCR=rMISCCR&~(1<<3); // USBD1 is set as device (not host)
rMISCCR=rMISCCR&~(1<<13); // Set USBD1 to normal mode (not suspend mode)
UsbdMain();
while(1)
{
delay();
}
Let's look at the definition of the function UsbdMain
void UsbdMain(void)
{
InitDescriptorTable(); // Initialize device descriptor
ConfigUsbd(); // USB configuration
PrepareEp1Fifo(); // Initialize endpoint 1
}
Let's look at the function ConfigUsbd
void ConfigUsbd(void)
{
ReconfigUsbd(); // Reconfigure USB
pISR_USBD =(unsigned)IsrUsbd; //Install interrupt function
ClearPending(BIT_USBD); // Clear USB interrupt pending flag
rINTMSK&=~(BIT_USBD); // Enable USB interrupt
}
void ReconfigUsbd(void)
{
// *** End point information ***
// EP0: control
// EP1: bulk in end point
// EP2: not used
// EP3: bulk out end point
// EP4: not used
// Disable the device from entering suspend mode (normal mode)
rPWR_REG=PWR_REG_DEFAULT_VALUE;
rINDEX_REG=0;
//EP0 max packit size = 8 maximum data packet
rMAXP_REG=FIFO_SIZE_8;
//EP0:clear OUT_PKT_RDY & SETUP_END
rEP0_CSR=EP0_SERVICED_OUT_PKT_RDY|EP0_SERVICED_SETUP_END;
rINDEX_REG=1;
#if (EP1_PKT_SIZE==32)
//EP1:max packit size = 32
rMAXP_REG=FIFO_SIZE_32;
#else
//EP1:max packit size = 64
rMAXP_REG=FIFO_SIZE_64;
#endif
rIN_CSR1_REG=EPI_FIFO_FLUSH|EPI_CDT;
//IN mode, IN_DMA_INT=masked, endpoint direction is IN, bulk transfer mode, interrupt disabled.
rIN_CSR2_REG=EPI_MODE_IN|EPI_IN_DMA_INT_MASK|EPI_BULK;
rOUT_CSR1_REG=EPO_CDT;
rOUT_CSR2_REG=EPO_BULK|EPO_OUT_DMA_INT_MASK;
rINDEX_REG=2;
//EP2:max packit size = 64
rMAXP_REG=FIFO_SIZE_64;
rIN_CSR1_REG=EPI_FIFO_FLUSH|EPI_CDT|EPI_BULK;
//IN mode, IN_DMA_INT=masked
rIN_CSR2_REG=EPI_MODE_IN|EPI_IN_DMA_INT_MASK;
rOUT_CSR1_REG=EPO_CDT;
rOUT_CSR2_REG=EPO_BULK|EPO_OUT_DMA_INT_MASK;
rINDEX_REG=3;
#if (EP3_PKT_SIZE==32)
//EP3:max packit size = 32
rMAXP_REG=FIFO_SIZE_32;
#else
//EP3:max packit size = 64
rMAXP_REG=FIFO_SIZE_64;
#endif
//OUT mode, IN_DMA_INT=masked
rIN_CSR1_REG=EPI_FIFO_FLUSH|EPI_CDT|EPI_BULK;
rIN_CSR2_REG=EPI_MODE_OUT|EPI_IN_DMA_INT_MASK;
rOUT_CSR1_REG=EPO_CDT;
//clear OUT_PKT_RDY, data_toggle_bit.
//The data toggle bit should be cleared when initialization.
rOUT_CSR2_REG=EPO_BULK|EPO_OUT_DMA_INT_MASK;
rINDEX_REG=4;
rMAXP_REG=FIFO_SIZE_64; //EP4:max packit size = 64
rIN_CSR1_REG=EPI_FIFO_FLUSH|EPI_CDT|EPI_BULK;
//OUT mode, IN_DMA_INT=masked
rIN_CSR2_REG=EPI_MODE_OUT|EPI_IN_DMA_INT_MASK;
//clear OUT_PKT_RDY, data_toggle_bit.
rOUT_CSR1_REG=EPO_CDT;
//The data toggle bit should be cleared when initialization.
rOUT_CSR2_REG=EPO_BULK|EPO_OUT_DMA_INT_MASK;
// Clear the interrupt flag
rEP_INT_REG=EP0_INT|EP1_INT|EP2_INT|EP3_INT|EP4_INT;
//Clear all usbd pending bits
rUSB_INT_REG=RESET_INT|SUSPEND_INT|RESUME_INT;
//EP0,1,3 & reset interrupt are enabled
//Interrupt enable register
rEP_INT_EN_REG=EP0_INT|EP1_INT|EP3_INT;
//USB reset interrupt enable
rUSB_INT_EN_REG=RESET_INT;
// Set endpoint 0 status to initialize
ep0State=EP0_STATE_INIT;
}
void IsrUsbd(void)
{
U8 usbdIntpnd,epIntpnd;
U8 saveIndexReg=rINDEX_REG;
usbdIntpnd=rUSB_INT_REG; // Read USB interrupt register
epIntpnd=rEP_INT_REG; // Read breakpoint interrupt register
//DbgPrintf( "[INT:EP_I=%x,USBI=%x]",epIntpnd,usbIntpnd );
if(usbdIntpnd&SUSPEND_INT)
{
rUSB_INT_REG=SUSPEND_INT;
DbgPrintf( " } if(usbdIntpnd&RESUME_INT) { rUSB_INT_REG=RESUME_INT; DbgPrintf(" } if(usbdIntpnd&RESET_INT) // Receive reset signal, reconfigure USB device configuration { DbgPrintf( " // Clear the interrupt flag rUSB_INT_REG = RESET_INT; //RESET_INT should be cleared after ResetUsbd(). //ResetUsbd(); ReconfigUsbd(); Test(); //PrepareEp1Fifo(); } if(epIntpnd&EP0_INT) { rEP_INT_REG=EP0_INT; Ep0Handler(); } if(epIntpnd&EP1_INT) { rEP_INT_REG=EP1_INT; Ep1Handler(); } if(epIntpnd&EP2_INT) { rEP_INT_REG=EP2_INT; DbgPrintf("<2:TBD]"); //not implemented yet //Ep2Handler(); } if(epIntpnd&EP3_INT) { rEP_INT_REG=EP3_INT; Ep3Handler(); } if(epIntpnd&EP4_INT) { rEP_INT_REG=EP4_INT; DbgPrintf("<4:TBD]"); //not implemented yet //Ep4Handler(); } ClearPending(BIT_USBD); rINDEX_REG=saveIndexReg; } void Test() { U8 in_csr1; rINDEX_REG=1; in_csr1=rIN_CSR1_REG; SET_EP1_IN_PKT_READY(); }
Previous article:Analysis of s3c2440 startup process
Next article:Talk about the comparison with S3C2440
Recommended ReadingLatest update time:2024-11-15 14:36
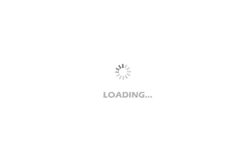
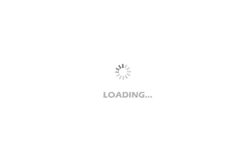
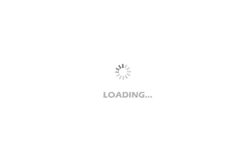
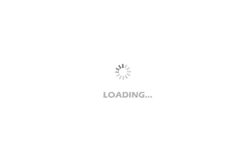
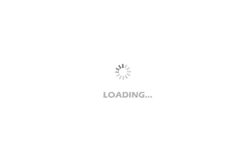
- Popular Resources
- Popular amplifiers
- Learn ARM development(16)
- Learn ARM development(17)
- Learn ARM development(18)
- Embedded system debugging simulation tool
- A small question that has been bothering me recently has finally been solved~~
- Learn ARM development (1)
- Learn ARM development (2)
- Learn ARM development (4)
- Learn ARM development (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- Analysis of the application of several common contact parts in high-voltage connectors of new energy vehicles
- Wiring harness durability test and contact voltage drop test method
- From probes to power supplies, Tektronix is leading the way in comprehensive innovation in power electronics testing
- From probes to power supplies, Tektronix is leading the way in comprehensive innovation in power electronics testing
- Sn-doped CuO nanostructure-based ethanol gas sensor for real-time drunk driving detection in vehicles
- Design considerations for automotive battery wiring harness
- Do you know all the various motors commonly used in automotive electronics?
- What are the functions of the Internet of Vehicles? What are the uses and benefits of the Internet of Vehicles?
- Power Inverter - A critical safety system for electric vehicles
- Analysis of the information security mechanism of AUTOSAR, the automotive embedded software framework
- 【RT-Thread software package application works】Multi-function weather clock
- [Erha Image Recognition Artificial Intelligence Vision Sensor] 6. Identify motors and control their operation
- ST60 millimeter wave water medium data transmission test report——part1
- 【GD32E503 Evaluation】 ADC Experiment (Continued)
- STM32 core Arduino board-ZeroKnow mini board with small size
- Programming examples to learn DSP timer and interrupt system
- TI Battery Tester Reference Design for High Current Applications
- [ESK32-360 Review] + TFT screen display function and expansion (1)
- [NXP Rapid IoT Review] Part 2: Detailed tutorial of online IDE - Open Rapid IoT to see the weather...
- 【GD32L233C-START Review】3. Implementation of USB keyboard