environment:
Host: XP
Development environment: MDK4.23
MCU:STM32F103CBT6
illustrate:
Collect AD through pins PA1 and PA2. Collect AD 10 times for each channel.
#include "ad_driver.h"
//Global variables
//AD sampling storage space
__IO uint16_t ADCConvertedValue[20];
//function
//Initialize AD
void init_ad(void)
{
ADC_InitTypeDef ADC_InitStructure;
DMA_InitTypeDef DMA_InitStructure;
GPIO_InitTypeDef GPIO_InitStructure;
//---------------------Charge AD initialization--------------------
//Start DMA clock
RCC_AHBPeriphClockCmd(RCC_AHBPeriph_DMA1, ENABLE);
//Start ADC1 clock
RCC_APB2PeriphClockCmd(RCC_APB2Periph_ADC1, ENABLE);
//Sampling pin settings
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA,ENABLE);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_1 | GPIO_Pin_2;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AIN;
GPIO_Init(GPIOA, &GPIO_InitStructure);
//DMA1 channel 1 configuration
DMA_DeInit(DMA1_Channel1);
//Peripheral address
DMA_InitStructure.DMA_PeripheralBaseAddr = ADC1_DR_ADDRESS;
//Memory address
DMA_InitStructure.DMA_MemoryBaseAddr = (uint32_t)ADCConvertedValue;
//dma transmission direction is one-way
DMA_InitStructure.DMA_DIR = DMA_DIR_PeripheralSRC;
//Set the length of the DMA buffer during transmission
DMA_InitStructure.DMA_BufferSize = 20;
//Set the DMA peripheral increment mode, a peripheral
DMA_InitStructure.DMA_PeripheralInc = DMA_PeripheralInc_Disable;
//Set DMA memory increment mode
DMA_InitStructure.DMA_MemoryInc = DMA_MemoryInc_Enable;
//Peripheral data word length
DMA_InitStructure.DMA_PeripheralDataSize = DMA_PeripheralDataSize_HalfWord;
//Memory data word length
DMA_InitStructure.DMA_MemoryDataSize = DMA_MemoryDataSize_HalfWord;
//Set DMA transfer mode: continuous loop mode
DMA_InitStructure.DMA_Mode = DMA_Mode_Circular;
//Set the DMA priority level
DMA_InitStructure.DMA_Priority = DMA_Priority_High;
//Set the variables in the two DMA memories to access each other
DMA_InitStructure.DMA_M2M = DMA_M2M_Disable;
DMA_Init(DMA1_Channel1, &DMA_InitStructure);
// Enable channel 1
DMA_Cmd(DMA1_Channel1, ENABLE);
//ADC1 configuration
//Independent working mode
ADC_InitStructure.ADC_Mode = ADC_Mode_Independent;
//scanning method
ADC_InitStructure.ADC_ScanConvMode = ENABLE;
//Continuous conversion
ADC_InitStructure.ADC_ContinuousConvMode = ENABLE;
//External trigger disabled
ADC_InitStructure.ADC_ExternalTrigConv = ADC_ExternalTrigConv_None;
//Right align the data
ADC_InitStructure.ADC_DataAlign = ADC_DataAlign_Right;
//Number of channels to convert
ADC_InitStructure.ADC_NbrOfChannel = 2;
ADC_Init(ADC1, &ADC_InitStructure);
//Rule mode channel configuration
ADC_RegularChannelConfig(ADC1, ADC_Channel_1 , 1, ADC_SampleTime_239Cycles5);
ADC_RegularChannelConfig(ADC1, ADC_Channel_2 , 2, ADC_SampleTime_239Cycles5);
// Enable DMA of ADC1
ADC_DMACmd(ADC1, ENABLE);
// Enable ADC1
ADC_Cmd(ADC1, ENABLE);
// Enable ADC1 reset calibration register
ADC_ResetCalibration(ADC1);
// Check if the calibration register is reset
while(ADC_GetResetCalibrationStatus(ADC1));
//Start calibration
ADC_StartCalibration(ADC1);
//Check if calibration is complete
while(ADC_GetCalibrationStatus(ADC1));
//Start software conversion of ADC1
ADC_SoftwareStartConvCmd(ADC1, ENABLE);
}
//Get charging voltage
float voltage_charge(void)
{
uint8_t i = 0;
uint16_t sum = 0;
float v = 0;
//Get the average value of charging voltage for 10 times
for (i = 0;i < 10;i++)
{
sum += ADCConvertedValue[i * 2];
}
sum /= 10;
//Convert to voltage value
v = 0.002991 * sum;
return v;
}
//Get the discharge voltage
float voltage_discharge(void)
{
uint8_t i = 0;
uint16_t sum = 0;
float v = 0;
//Get the average value of charging voltage for 10 times
for (i = 0;i < 10;i++)
{
sum += ADCConvertedValue[i * 2 + 1];
}
sum /= 10;
//Convert to voltage value
v = 0.002991 * sum;
return v;
}
Previous article:Understanding of dma in stm32
Next article:Summary of STM32 peripheral DMA usage
Recommended ReadingLatest update time:2024-11-23 10:29
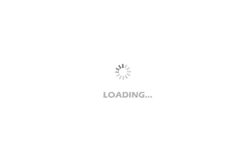
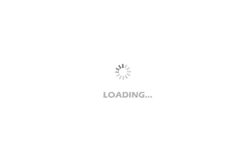
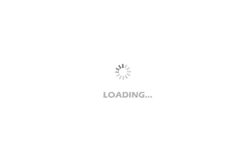
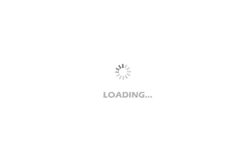
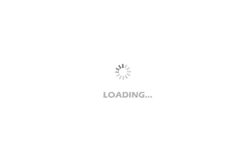
- Popular Resources
- Popular amplifiers
- Naxin Micro and Xinxian jointly launched the NS800RT series of real-time control MCUs
- How to learn embedded systems based on ARM platform
- Summary of jffs2_scan_eraseblock issues
- Application of SPCOMM Control in Serial Communication of Delphi7.0
- Using TComm component to realize serial communication in Delphi environment
- Bar chart code for embedded development practices
- Embedded Development Learning (10)
- Embedded Development Learning (8)
- Embedded Development Learning (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Intel promotes AI with multi-dimensional efforts in technology, application, and ecology
- ChinaJoy Qualcomm Snapdragon Theme Pavilion takes you to experience the new changes in digital entertainment in the 5G era
- Infineon's latest generation IGBT technology platform enables precise control of speed and position
- Two test methods for LED lighting life
- Don't Let Lightning Induced Surges Scare You
- Application of brushless motor controller ML4425/4426
- Easy identification of LED power supply quality
- World's first integrated photovoltaic solar system completed in Israel
- Sliding window mean filter for avr microcontroller AD conversion
- What does call mean in the detailed explanation of ABB robot programming instructions?
- STMicroelectronics discloses its 2027-2028 financial model and path to achieve its 2030 goals
- 2024 China Automotive Charging and Battery Swapping Ecosystem Conference held in Taiyuan
- State-owned enterprises team up to invest in solid-state battery giant
- The evolution of electronic and electrical architecture is accelerating
- The first! National Automotive Chip Quality Inspection Center established
- BYD releases self-developed automotive chip using 4nm process, with a running score of up to 1.15 million
- GEODNET launches GEO-PULSE, a car GPS navigation device
- Should Chinese car companies develop their own high-computing chips?
- Infineon and Siemens combine embedded automotive software platform with microcontrollers to provide the necessary functions for next-generation SDVs
- Continental launches invisible biometric sensor display to monitor passengers' vital signs
- Mid-Autumn Festival Gift-TI-36X Pro Scientific Calculator
- Reset mode of TMS320C6678
- The PWM module of the MSP430G2 microcontroller controls the brightness of the LED indicator
- Who is holding back Moore's Law?
- Novel single-electrode touch switch circuit
- FPGA-Based Video Games
- Summary of DSP boot mode / boot loader / power-on sequence / online upgrade and other issues
- 【Silicon Labs BG22-EK4108A Bluetooth Development Evaluation】 III. Modify the example project to achieve dual-channel PWM output
- Does anyone have the routine for balancing a ball? It's a ping-pong ball sliding on a round flat surface and never falling off.
- micropython update: 2020.5