/*-----------------------------------------------
Name: DS18b20 temperature detection LCD display
Forum: www.doflye.net
Written by: shifang
Date: 2009.5
Modification: None
content:
------------------------------------------------*/
#include
#include
#include "18b20.h"
#include "1602.h"
#include "delay.h"
#define KeyPort P3 //Define the key port
bit ReadTempFlag; //define read time flag
void Init_Timer0(void); //Timer initialization
unsigned char KeyScan(void); //keyboard scan
/*------------------------------------------------
Serial communication initialization
------------------------------------------------*/
void UART_Init(void)
{
SCON = 0x50; // SCON: Mode 1, 8-bit UART, receive enabled
TMOD |= 0x20; // TMOD: timer 1, mode 2, 8-bit 重装
TH1 = 0xFD; // TH1: reload value 9600 baud rate crystal 11.0592MHz
TR1 = 1; // TR1: timer 1 is turned on
//EA = 1; //Open the general interrupt
//ES = 1; //Open serial port interrupt
IF=1;
}
/*------------------------------------------------
Main function
------------------------------------------------*/
void main (void)
{
int temp,tempH=50,tempL=1;
float temperature;
unsigned char TempFlag=0;
char displaytemp[16],num; //define the temporary storage array of the display area
LCD_Init(); //Initialize LCD
DelayMs(20); //Delay helps to stabilize
LCD_Clear(); //Clearing
Init_Timer0();
UART_Init();
Lcd_User_Chr(); //Write custom characters
while (1) //main loop
{
num=KeyScan();
switch(num)
{
case 1:if(tempH<127)tempH++;break;
case 2:if(tempH>-55)tempH--;break;
case 3:if(tempL<127)tempL++;break;
case 4:if(tempL>-55)tempL--;break;
default:break;
}
switch(TempFlag)
{
case 0: // Output temperature upper and lower limits
sprintf(displaytemp,"H.%3d L.%3d ",tempH,tempL);
LCD_Write_String(0,1,displaytemp);//Display the second line
break;
case 1:LCD_Write_String(0,1,"over tempH ");break;
case 2:LCD_Write_String(0,1,"under tempL ");break;
default:break;
}
if(ReadTempFlag==1)
{
ReadTempFlag=0;
temp=ReadTemperature();
temperature=temp*0.0625;
temp>>=4;
if(temp>tempH)
TempFlag=1; //Higher than high temperature flag
else if(temp TempFlag=2; //Below low temperature flag else TempFlag=0; //Normal display flag sprintf(displaytemp,"Temp %6.2f ",temperature); //Print temperature value LCD_Write_String(0,0,displaytemp);//Display the first line LCD_Write_Char(13,0,0x01); //Write the upper right corner of the temperature LCD_Write_Char(14,0,'C'); //Write character C } } } /*------------------------------------------------ Timer initialization subroutine ------------------------------------------------*/ void Init_Timer0(void) { TMOD |= 0x01; //Use mode 1, 16-bit timer, use the "|" symbol to avoid being affected when using multiple timers //TH0=0x00; //Give initial value //TL0=0x00; EA=1; //General interrupt is turned on ET0=1; //Timer interrupt is turned on TR0=1; //Timer switch is turned on } /*------------------------------------------------ Timer interrupt subroutine ------------------------------------------------*/ void Timer0_isr(void) interrupt 1 { static unsigned int num; TH0=(65536-2000)/256; //Reassign 2ms TL0=(65536-2000)%256; num++; if(num==400) // { num=0; ReadTempFlag=1; //Read flag position 1 } } /*------------------------------------------------ Key scanning function, returns the scanned key value ------------------------------------------------*/ unsigned char KeyScan(void) { unsigned char keyvalue; if(KeyPort!=0xff) { DelayMs(10); if(KeyPort!=0xff) { keyvalue=KeyPort; while(KeyPort!=0xff); switch(keyvalue) { case 0xfe:return 1;break; case 0xfd:return 2;break; case 0xfb:return 3;break; case 0xf7:return 4;break; case 0xef:return 5;break; case 0xdf:return 6;break; case 0xbf:return 7;break; case 0x7f:return 8;break; default:return 0;break; } } } return 0; }
Previous article:Single chip practical password lock
Next article:Single chip dual color dot matrix display specific graphics
Recommended ReadingLatest update time:2024-11-15 14:56
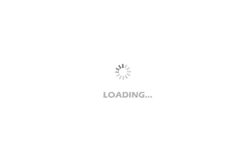
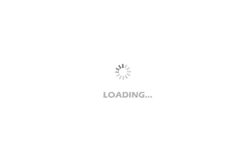
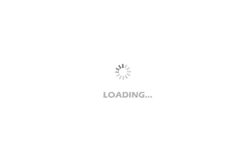
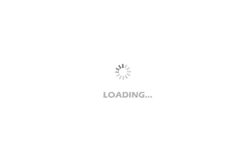
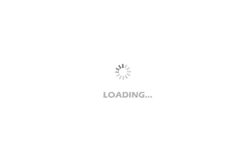
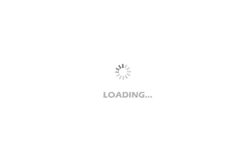
- Popular Resources
- Popular amplifiers
-
Wireless Sensor Network Technology and Applications (Edited by Mou Si, Yin Hong, and Su Xing)
-
Modern Electronic Technology Training Course (Edited by Yao Youfeng)
-
Modern arc welding power supply and its control
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
- Learn ARM development(16)
- Learn ARM development(17)
- Learn ARM development(18)
- Embedded system debugging simulation tool
- A small question that has been bothering me recently has finally been solved~~
- Learn ARM development (1)
- Learn ARM development (2)
- Learn ARM development (4)
- Learn ARM development (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- Analysis of the application of several common contact parts in high-voltage connectors of new energy vehicles
- Wiring harness durability test and contact voltage drop test method
- From probes to power supplies, Tektronix is leading the way in comprehensive innovation in power electronics testing
- From probes to power supplies, Tektronix is leading the way in comprehensive innovation in power electronics testing
- Sn-doped CuO nanostructure-based ethanol gas sensor for real-time drunk driving detection in vehicles
- Design considerations for automotive battery wiring harness
- Do you know all the various motors commonly used in automotive electronics?
- What are the functions of the Internet of Vehicles? What are the uses and benefits of the Internet of Vehicles?
- Power Inverter - A critical safety system for electric vehicles
- Analysis of the information security mechanism of AUTOSAR, the automotive embedded software framework
- Problems in PCB design
- Please share the installation package of modelsim10.4 or other versions
- ATE1133 audio decoding solution, USB sound card solution, TYPE C audio adapter chip solution
- Baobaobao~~~After the Chinese New Year, what new developments are there in the evaluation industry? Hurry up and take a look at the Evaluation Intelligence Bureau~
- [NUCLEO-L552ZE Review] Small thermometer
- STM3L4R5 driver for hts221 and stts751
- Cytech’s award-winning live broadcast: Let you learn about ADI’s digital health biosensor series live!
- Evaluation Weekly Report 20220406: Germany's PHYTEC's i.MX 8M+ AI board and RTT Renesas high-performance CPK-RA6M4 are here
- Is this post of the study club incomplete? The formula part?
- [NXP Rapid IoT Review] + Review the Bluetooth function and learn how to program the application