Summary:
This article mainly introduces the SPI interface of STM32, cubeMX software configuration of SPI interface and analysis of SPI related codes.
Introduction to STM32 SPI:
(1) SPI protocol [Serial Peripheral Interface]
Serial Peripheral Interface is a high-speed full-duplex communication bus, mainly used for communication between MCU and FLASH\ADC\LCD and other modules.
(2) SPI signal line
SPI contains 4 buses in total.
SS (Slave Select): Chip select signal line. When multiple SPI devices are connected to the MCU, the chip select signal line of each device is connected to a separate pin of the MCU, while the other SCK, MOSI, and MISO lines are for multiple devices to be connected in parallel to the same SPI bus, and low level is valid.
SCK (Serial Clock): Clock signal line, generated by the main communication device. Different devices support different clock frequencies. For example, the maximum SPI clock frequency of STM32 is f PCLK /2.
MOSI (Master Output Slave Input): Master device output / slave device input pin. The data of the host is output from this signal line, and the data of the slave is read from this signal line, that is, the direction of the data on this line is from the host to the slave.
MISO (Master Input Slave Output): Master input/slave output pin. The host reads data from this signal line, and the slave outputs data from this signal line, that is, the direction of data on this line is from slave to host.
The following figure shows the communication between the master device and multiple slave devices. SCK, MOSI, and MISO are connected together, and NSS is connected to different IO pins for control. If the master device wants to communicate with the slave device, it must first pull down the NSS pin of the corresponding slave device to enable it. In the default state, IO1, IO2, and IO3 are all high levels. When the master device communicates with slave device 1, pull down the IO1 pin to enable slave device 1. Slave devices 2 and 3 are not enabled and do not respond.
(3) SPI characteristics
A single transfer can be selected as 8 or 16 bits.
Baud rate prescaler factor (maximum fPCLK/2).
The clock polarity (CPOL) and phase (CPHA) are programmable.
The data transmission order is programmable, MSB first or LSB first.
Note: MSB (Most Significant Bit) is the "most significant bit" and LSB (Least Significant Bit) is the "least significant bit".
Dedicated transmit and receive flags that can trigger interrupts.
DMA can be used for data transfer operations.
The figure below is the SPI framework diagram of STM32.
As shown in the figure above, the signal received by the MISO data line is processed by the shift register and the data is transferred to the receive buffer, and then this data can be read from the receive buffer by our software.
When we want to send data, we write the data into the send buffer, and the hardware will process it using the shift register and output it to the MOSI data line.
The SCK clock signal is generated by the baud rate generator, and we can control the baud rate it outputs through the baud rate control bit (BR).
The control register CR1 is in charge of the main control circuit, and the protocol settings (clock polarity, phase, etc.) of the STM32 SPI module are set by it. The control register CR2 is used to set various interrupt enables.
Finally, the NSS pin plays the role of the SS chip select signal line in the SPI protocol. If we configure the NSS pin as hardware automatic control, the SPI module can automatically determine whether it can become the SPI master or automatically enter the SPI slave mode. But in fact, we use some GPIO pins controlled by software as SS signals more often, and this GPIO pin can be selected at will.
(4) SPI clock timing
SPI has four operating modes depending on the clock polarity (CPOL) and phase (CPHA).
Clock polarity (CPOL) defines the clock idle state level:
CPOL=0 means the clock is at a low level when it is idle
CPOL=1 means the clock is high when idle
The clock phase (CPHA) defines when the data is collected.
CPHA=0: Data sampling is performed on the first transition edge (rising edge or falling edge) of the clock.
CPHA=1: Data sampling is performed on the second transition edge (rising or falling edge) of the clock.
cubeMX software configuration SPI:
The following is an introduction to the cubeMX software configuration method for the STM32L152 SPI interface.
(1) Open the software, select the corresponding chip, and configure the clock source;
(2) Select SPI1 as full-duplex and hardware NSS as closed, as shown below:
(3) After checking, PA5, PA6, and PA7 are as shown below. When PA4 is configured as a normal IO port, gpio_output
(4) Select the default parameter configuration for SPI1, as shown in the figure below
(5) Generate code and save it.
Analysis of SPI functions of HAL library:
The following is a detailed analysis of the generated SPI functions and function calls.
SPI_HandleTypeDef hspi1; //SPI structure class definition, let's look at the declaration inside the structure.
The following is an analysis of the SPI initialization function:
void HAL_SPI_MspInit(SPI_HandleTypeDef* hspi)
{
GPIO_InitTypeDef GPIO_InitStruct;
if(hspi->Instance==SPI1)
{
/* USER CODE BEGIN SPI1_MspInit 0 */
/* USER CODE END SPI1_MspInit 0 */
/* Peripheral clock enable */
__HAL_RCC_SPI1_CLK_ENABLE(); //Enable SPI1 clock
/**SPI1 GPIO Configuration
PA5 ------> SPI1_SCK
PA6 ------> SPI1_MISO
PA7 ------> SPI1_MOSI
*/
GPIO_InitStruct.Pin = GPIO_PIN_5|GPIO_PIN_6|GPIO_PIN_7;
GPIO_InitStruct.Mode = GPIO_MODE_AF_PP;
GPIO_InitStruct.Pull = GPIO_NOPULL;
GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH;
GPIO_InitStruct.Alternate = GPIO_AF5_SPI1;
HAL_GPIO_Init(GPIOA, &GPIO_InitStruct); //Configure SPI data line and clock line
/* USER CODE BEGIN SPI1_MspInit 1 */
/* USER CODE END SPI1_MspInit 1 */
}
static void MX_SPI1_Init(void)
{
hspi1.Instance = SPI1;
hspi1.Init.Mode = SPI_MODE_MASTER; //Master mode
hspi1.Init.Direction = SPI_DIRECTION_2LINES; //Full duplex
hspi1.Init.DataSize = SPI_DATASIZE_8BIT; //The data bit is 8 bits
hspi1.Init.CLKPolarity = SPI_POLARITY_LOW;//CPOL=0,low
hspi1.Init.CLKPhase = SPI_PHASE_1EDGE; //CPHA is the first change edge of the data line
hspi1.Init.NSS = SPI_NSS_SOFT; //Software controls NSS
hspi1.Init.BaudRatePrescaler = SPI_BAUDRATEPRESCALER_2;//2 frequency division, 32M/2=16MHz
hspi1.Init.FirstBit = SPI_FIRSTBIT_MSB; //The highest bit is sent first
hspi1.Init.TIMode = SPI_TIMODE_DISABLE; //TIMODE mode disabled
hspi1.Init.CRCCalculation = SPI_CRCCALCULATION_DISABLE;//CRC is closed
hspi1.Init.CRCPolynomial = 10; //Default value, invalid
if (HAL_SPI_Init(&hspi1) != HAL_OK) // Initialization
{
_Error_Handler(__FILE__, __LINE__);
}
}
The following two functions are mainly called to send and receive data using the SPI interface:
HAL_StatusTypeDef HAL_SPI_Transmit(SPI_HandleTypeDef *hspi, uint8_t *pData, uint16_t Size, uint32_t Timeout); //Send data
HAL_StatusTypeDef HAL_SPI_Receive(SPI_HandleTypeDef *hspi, uint8_t *pData, uint16_t Size, uint32_t Timeout); //Receive data
Previous article:Detailed explanation of time.h file in STM32
Next article:STM32 - Power consumption cannot be reduced after entering STOP mode
Recommended ReadingLatest update time:2024-11-16 13:57
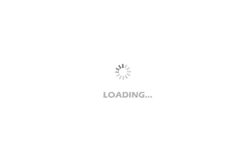
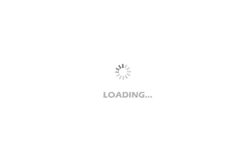
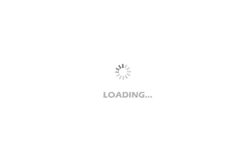
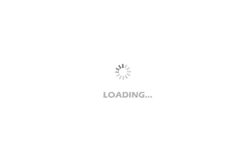
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- 【New Year's Taste Competition】+ Spring Festival Customs
- ESD electrostatic protection classic book - "ESD Secrets: Electrostatic Protection Principles and Typical Applications"
- [TI star product limited time purchase] + AWR683ISK run demo
- Fancy PCB Exhibition (1)
- Why is it said that 90% of EMC is designed? (Long article)
- Recommended postgraduate schools
- Share CC2541 Bluetooth learning about ADC
- MSP430 LCD5110 driver programming example
- NUCLEO F446RE meets X-NUCLEO-IKS01A3
- A huge reward! Looking for someone who can crack the RSA2048 and factory protocol in the ECU!