main.c
#include
#include "mytype.h"
#include "iic.h"
#include "mma7455.h"
uint8 X,Y,Z;
void main()
{
uint8 i,temp;
EA=1; //Open interrupt
EX1=1; //Open external interrupt INIT1
//Initialize IIC bus
IIC_init();
//Write data
IIC_start();
IIC_write_byte(MMA7455_ADDER_WRITE); //1. Address MMA7455 on IIC bus
IIC_respons();
IIC_write_byte(MMA7455_Mode_Control_Register); //2. Address mode setting register
IIC_respons();
IIC_write_byte(0x05); //3. Write mode register data
IIC_respons();
IIC_stop();
//Write data
IIC_start();
IIC_write_byte(MMA7455_ADDER_WRITE); //1. Address MMA7455 on the IIC bus
IIC_respons();
IIC_write_byte(MMA7455_Mode_Control_Register); //2. Address the range detection setting register
IIC_respons();
IIC_write_byte(MMA7455_2G_Measurement_Mode); //3. Set the range to 2G, detection mode. Write the range detection setting register configuration data
IIC_respons();
IIC_stop();
while(1)
{
//Read X-axis data
IIC_start();
IIC_write_byte(MMA7455_ADDER_WRITE); //1. IIC address addressing
IIC_respons();
IIC_write_byte(MMA7455_READ_X); //2. X-axis data register addressing
IIC_respons();
IIC_start(); //3.
IIC_write_byte(MMA7455_ADDER_READ); //Change read and write direction
IIC_respons();
X=IIC_read_byte(); //4.Read data
IIC_stop();
//Read Y-axis data
IIC_start();
IIC_write_byte(MMA7455_ADDER_WRITE); //1. IIC address addressing
IIC_respons();
IIC_write_byte(MMA7455_READ_Y); //2. X-axis data register addressing
IIC_respons();
IIC_start(); //3.
IIC_write_byte(MMA7455_ADDER_READ); //Change read and write direction
IIC_respons();
Y=IIC_read_byte(); //4.Read data
IIC_stop();
//Read X-axis data
IIC_start();
IIC_write_byte(MMA7455_ADDER_WRITE); //1. IIC address addressing
IIC_respons();
IIC_write_byte(MMA7455_READ_X); //2. X-axis data register addressing
IIC_respons();
IIC_start(); //3.
IIC_write_byte(MMA7455_ADDER_READ); //Change read and write direction
IIC_respons();
Z=IIC_read_byte(); //4.Read data
IIC_stop();
}
}
iic.c
#include
#include
#include "iic.h"
#include "mytype.h"
#define NOP() _nop_()
#define _Nop() _nop_()
void IIC_start()
{
SDA=1;
_Nop();
SCL=1;
_Nop();
_Nop();
_Nop();
_Nop();
_Nop();
SDA=0;
_Nop();
_Nop();
_Nop();
_Nop();
_Nop();
SCL=0;
_Nop();
_Nop();
}
void IIC_stop()
{
SDA=0;
_Nop();
SCL=1;
_Nop();
_Nop();
_Nop();
_Nop();
_Nop();
SDA=1;
_Nop();
_Nop();
_Nop();
_Nop();
_Nop();
_Nop();
_Nop();
_Nop();
}
void IIC_init() //
{
SDA=1;
SCL=1;
}
void IIC_write_byte(int8 adder)
{
uint8 i,temp;
temp=adder;
for(i=0;i<8;i++)
{
temp=temp<<1;
SDA=CY;
SCL=1;
_Nop();
_Nop();
_Nop();
_Nop();
_Nop();
SCL=0;
}
_Nop();
_Nop();
SDA=1;
_Nop();
_Nop();
SCL=1;
_Nop();
_Nop();
_Nop();
}
uint8 IIC_read_byte()
{
uint8 i,j,k;
SCL=0;
_Nop();
_Nop();
_Nop();
_Nop();
_Nop();
_Nop();
_Nop();
_Nop();
_Nop();
_Nop();
SDA=1;
_Nop();
_Nop();
_Nop();
_Nop();
_Nop();
_Nop();
_Nop();
_Nop();
_Nop();
_Nop();
for(i=0;i<8;i++)
{
SCL=1;
_Nop();
_Nop();
_Nop();
j=SDA;
k=(k<<1)|j;
SCL=0;
_Nop();
_Nop();
_Nop();
_Nop();
_Nop();
_Nop();
_Nop();
_Nop();
_Nop();
_Nop();
}
_Nop();
_Nop();
_Nop();
return k;
}
uint8 IIC_respons()
{
_Nop();
_Nop();
_Nop();
_Nop();
_Nop();
_Nop();
_Nop();
_Nop();
if(SDA==1)
{
return 0;
}
SCL=0;
_Nop();
_Nop();
return 1;
}
iic.h
#ifndef __IIC_H_
#define __IIC_H_
#include
sbit SDA = P2^1; //PIN 13 of MA7455 is data
sbit SCL = P2^0; //PIN 14 of MA7455 is clock
void IIC_delay();
void IIC_init();
void IIC_start();
void IIC_stop();
void IIC_write_byte(uint8);
uint8 IIC_read_byte();
uint8 IIC_respons();
mma7455.c
#include
#include
#include "iic.h"
#include"mma7455.h"
#define NOP() _nop_()
#define _Nop() _nop_()
void IIC_adder_write_byte(int8 adder,int8 rw)
{
uint8 i,temp;
temp=adder;
temp=temp<<1; //Shift left one bit from device address,
temp=temp|rw; //Read and write one bit later
IIC_delay();
for(i=0;i<8;i++)
{
temp=temp<<1;
SDA=CY;
SCL=1;
_Nop();
_Nop();
_Nop();
_Nop();
_Nop();
SCL=0;
}
_Nop();
_Nop();
SDA=1;
_Nop();
_Nop();
SCL=1;
_Nop();
_Nop();
_Nop();
}
mma7455.h
#ifndef __MMA7455_H__
#define __MMA7455_H__
#define MMA7455_ADDER_WRITE 0x3A //0x1D shift left, add a write bit 0 at the end, get 0x3A
#define MMA7455_ADDER_READ 0x3B //0x1D shift left, add a read bit 1 at the end, get 0x3B
#define MMA7455_Mode_Control_Register 0x16
#define MMA7455_WHOAMI 0X0F
#define MMA7455_2G_Measurement_Mode 0x05
#define MMA7455_READ_X 0x06 //Read 8bits X-axis data address
#define MMA7455_READ_Y 0x07 //Read 8bits Y-axis data address
#define MMA7455_READ_Z 0x08 //Read 8bits Y-axis data address
void IIC_adder_write_byte(int8,int8);
mytype.h
#ifndef _MY_TYPE_H__
#define _MY_TPYE_H__
#define uint8 unsigned char
#define uint16 unsigned short int
#define uint32 unsigned long int
#define uint64 unsigned long long int
#define int8 signed char
#define int16 signed short int
#define int32 signed long int
#define int64 signed long long int
#endif
Previous article:DMA demo for STM32, USART
Next article:Solution to unsuccessful stm32 serial communication
Recommended ReadingLatest update time:2024-11-16 23:35
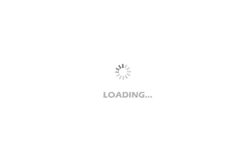
- Popular Resources
- Popular amplifiers
-
Principles and Applications of Single Chip Microcomputers 3rd Edition (Zhang Yigang)
-
Traffic Light Control System Based on Fuzzy Control (Single-Chip Microcomputer Implementation)
-
Principles and Applications of Single Chip Microcomputers (Second Edition) (Wanlong)
-
MCU Principles and C51 Programming Tutorial (2nd Edition)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Matters needing attention in the production of high-frequency microwave boards
- eMMC Summary (Forwarded)
- [ESP32-Korvo Review] 07 Compile the first project hello world
- MCU restart freeze problem help
- How to use an op amp to control the FB feedback pin of an adjustable power supply?
- National Technology N32G032 Motor Control Board Hardware Usage Guide
- What is a Class D power amplifier?
- CBB capacitors and monolithic capacitors have better frequency characteristics, small dielectric loss, wide response range, high insulation impedance, and no polarity.
- Recommend a mall for purchasing electronic components: free shipping for new customers’ first order, and also provide services such as sample application and technical support!
- Zhengzhou Customs destroyed 20,000 counterfeit Texas Instruments integrated circuits. Have you ever come into contact with counterfeit and shoddy products at work?