Introduction:
DMA stands for Direct Memory Access, which means direct memory access.
For example, serial port transmission can be sent with or without DMA. Transmission without DMA requires the real-time participation of the microcontroller, which sends data one by one and monitors it. However, if DMA is used, after setting the starting address, data size and other parameters, a dedicated DMA module will directly send data, and the microcontroller does not need to participate in the transmission process. After the transmission, an interrupt will be generated to inform the microcontroller. It can be seen that using DMA can save microcontroller resources and allow the microcontroller to do more things at the same time.
STM32 has up to 2 DMA controllers (DMA2 only exists in large-capacity products), DMA1 has 7 channels (channel 1 to channel 7). DMA2 has 5 channels (channel 1 to channel 5). Each channel is dedicated to managing requests for memory access from one or more peripherals. There is also an arbitration to coordinate the priority of each DMA request.
Request List:
Request list of 7 channels of DMA1:
DMA2 request queue for 5 channels
CODE:
Use DMA to send serial port.
//dma.c#include "dma.h"DMA_InitTypeDef DMA_InitStructure;u16 DMA1_MEM_LEN;//Save the length of each data transfer by DMA //Configuration of each channel of DMA1//The transmission form here is fixed, which needs to be modified according to different situations//From memory->peripheral mode/8-bit data width/memory increment mode//DMA_CHx:DMA channel CHx//cpar:peripheral address//cmar:memory address//cndtr:data transfer amount void MYDMA_Config(DMA_Channel_TypeDef* DMA_CHx,u32 cpar,u32 cmar,u16 cndtr) { RCC_AHBPeriphClockCmd(RCC_AHBPeriph_DMA1, ENABLE); //Enable DMA transmission DMA_DeInit(DMA_CHx); //Reset DMA channel 1 register to default value DMA1_MEM_LEN=cndtr; DMA_InitStructure.DMA_PeripheralBaseAddr = cpar; //DMA peripheral base address DMA_InitStructure.DMA_MemoryBaseAddr = cmar; //DMA memory base address DMA_InitStructure.DMA_DIR = DMA_DIR_PeripheralDST; //Data transmission direction, read from memory and send to peripherals DMA_InitStructure.DMA_BufferSize = cndtr; //DMA channel DMA buffer size DMA_InitStructure.DMA_PeripheralInc = DMA_PeripheralInc_Disable; //Peripheral address register remains unchanged DMA_InitStructure.DMA_MemoryInc = DMA_MemoryInc_Enable; //Memory address register increment DMA_InitStructure.DMA_PeripheralDataSize = DMA_PeripheralDataSize_Byte; //Data width is 8 bits DMA_InitStructure.DMA_MemoryDataSize = DMA_MemoryDataSize_Byte; //Data width is 8 bits DMA_InitStructure.DMA_Mode = DMA_Mode_Normal; //Work in normal mode DMA_InitStructure.DMA_Priority = DMA_Priority_Medium; //DMA channel x has medium priority DMA_InitStructure.DMA_M2M = DMA_M2M_Disable; //DMA channel x is not set for memory to memory transfer DMA_Init(DMA_CHx, &DMA_InitStructure); //Initialize the registers identified by the DMA channel USART1_Tx_DMA_Channel according to the parameters specified in DMA_InitStruct} // Enable a DMA transfer void MYDMA_Enable(DMA_Channel_TypeDef*DMA_CHx) { DMA_Cmd(DMA_CHx, DISABLE ); //Disable the channel indicated by USART1 TX DMA1 DMA_SetCurrDataCounter(DMA_CHx,DMA1_MEM_LEN); //DMA channel DMA buffer size DMA_Cmd(DMA_CHx, ENABLE); //Enable the channel indicated by USART1 TX DMA1 } main.c #include "led.h"#include "delay.h"#include "key.h"#include "sys.h"#include "lcd.h"#include "usart.h" #include "dma.h"#define SEND_BUF_SIZE 8200 //Send data length, preferably equal to an integer multiple of sizeof(TEXT_TO_SEND)+2.u8 SendBuff[SEND_BUF_SIZE]; //Send data buffer const u8 TEXT_TO_SEND[]={"ALIENTEK WarShip STM32F1 DMA Serial Port Experiment"}; int main(void) { u16 i; u8 t=0; u8 j,mask=0; float pro=0;//Progress delay_init(); //delay function initialization NVIC_PriorityGroupConfig(NVIC_PriorityGroup_2); //Set the interrupt priority group to group 2: 2-bit preemption priority, 2-bit response priority uart_init(115200); //Serial port initialized to 115200 LED_Init(); //Initialize the hardware interface connected to the LED LCD_Init(); //Initialize LCD KEY_Init(); //Key initialization MYDMA_Config(DMA1_Channel4,(u32)&USART1->DR,(u32)SendBuff,SEND_BUF_SIZE);//DMA1 channel 4, peripheral is set to serial port 1, memory is SendBuff, length is SEND_BUF_SIZE. POINT_COLOR=RED; //Set the font to red LCD_ShowString(30,50,200,16,16,"WarShip STM32"); LCD_ShowString(30,70,200,16,16,"DMA TEST"); LCD_ShowString(30,90,200,16,16,"ATOM@ALIENTEK"); LCD_ShowString(30,110,200,16,16,"2015/1/15"); LCD_ShowString(30,130,200,16,16,"KEY0:Start"); //Display prompt information j=sizeof(TEXT_TO_SEND); for(i=0;i=j)//Add line break { if(mask) { SendBuff[i]=0x0a; t=0; }else { SendBuff[i]=0x0d; mask++; } } else //Copy TEXT_TO_SEND statement { mask=0; SendBuff[i]=TEXT_TO_SEND[t]; t++; } } POINT_COLOR=BLUE; //Set the font to blue i=0; while(1) { t=KEY_Scan(0); if(t==KEY0_PRES)//KEY0 is pressed { LCD_ShowString(30,150,200,16,16,"Start Transimit...."); LCD_ShowString(30,170,200,16,16," %");//Display the percent sign printf("\r\nDMA DATA:\r\n"); USART_DMACmd(USART1,USART_DMAReq_Tx,ENABLE); //Enable DMA transmission of serial port 1 MYDMA_Enable(DMA1_Channel4); //Start a DMA transfer! //Wait for DMA transfer to complete, now we can do something else, turn on the light //In actual applications, other tasks can be performed during data transmission while(1) { if(DMA_GetFlagStatus(DMA1_FLAG_TC4)!=RESET) //Judge whether the transmission of channel 4 is completed { DMA_ClearFlag(DMA1_FLAG_TC4); //Clear channel 4 transfer completion flag break; } pro=DMA_GetCurrDataCounter(DMA1_Channel4); //Get the current amount of data remaining pro=1-pro/SEND_BUF_SIZE; //get the percentage pro*=100; //Expand 100 times LCD_ShowNum(30,170,pro,3,16); } LCD_ShowNum(30,170,100,3,16); //Display 100% LCD_ShowString(30,150,200,16,16,"Transimit Finished!");//Prompt that the transmission is completed } i++; delay_ms(10); if(i==20) { LED0=!LED0; //Prompt that the system is running i=0; } } }
Previous article:STM32 Series Chapter 22 - I2C
Next article:STM32 Series Chapter 20 - DAC
Recommended ReadingLatest update time:2024-11-16 13:39
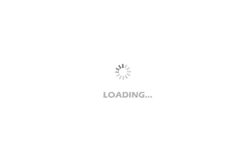
- Popular Resources
- Popular amplifiers
-
Microcomputer Principles and Interface Technology 3rd Edition (Zhou Mingde, Zhang Xiaoxia, Lan Fangpeng)
-
Automotive Electronics S32K Series Microcontrollers: Based on ARM Cortex-M4F Core
-
LPC1700 Chapter 31 General Purpose DMA
-
ARM Cortex-M4+Wi-Fi MCU Application Guide (Embedded Technology and Application Series) (Guo Shujun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- 【Recruitment】Full-time and part-time RF engineers
- What is the difference between the voltage follower circuit of the transistor and the operational amplifier?
- How can I detect whether there is 220V input with the lowest power consumption?
- TI K2 Heterogeneous SoC (66AK&663x) Software Development Difficulties and Software Solutions
- This is a simplified circuit for measuring insulation resistance using the unbalanced bridge method.
- CircuitPython goals for 2021
- DIY a miniaturized ultrasound device
- MPS Mall Big Offer Experience Season】 Unboxing
- Review summary: Free review - Topmicro smart display module
- Has anyone researched tinyos and nesc?