1. Input/output port GPIO programming
1--(01), one-digit digital tube static display (realized by 74HC595)
1. Pin connection module
First, let me introduce the relevant pins of LPC2106~~
Features: Independent pin configuration is possible
Application: The purpose of the pin connection module is to configure the pins to the required functions (this chapter mainly introduces the GPIO function~~ Others will be introduced in the following chapters~~)
Description: The pin connection module enables selected pins to have more than one function. Configuration registers control multiplexers to connect pins to on-chip peripherals. Peripherals must be connected to the appropriate pins before activation and any related read-only enables. Any enabled peripheral function is considered invalid if it is not mapped to the corresponding pin.
Register Description:
The pin connection module consists of two registers:
Pin function register 0: (PINSEL0)
The PINSEL0 register controls the function of the pin according to the settings in the table below.
The direction control bit in the IODIR register is only valid when the pin is selected as GPIO function (which is what this chapter will describe). For other functions, the direction is automatically controlled.
Pin function register 1: (PINSEL1)
The PINSEL1 register sets the function of the control pin according to the following table.
The direction control bit in the IODIR register is only valid when the pin selects the GPIO function. For other functions, the direction is automatically controlled.
When DBGSEL is pulled low during reset, the function control of pins P0.17-P0.31 is valid. (I really don't know this~~ )
Pin function register value:
The PINSEL register controls the function of the device pins. See the figure below.
Each pair of register bits corresponds to a specific device pin.
The direction control bit of the IODIR register is valid only when the pin is selected as the GPIO function.
The direction of other functions is controlled automatically.
Each derivative typically has a different pinout, so each pin may have a different function.
2. GPIO
characteristic:
1) Single bit direction control
2) Individually control the setting and clearing of outputs
3) All I/O ports default to input after reset
application:
1) General I/O port
2) Driving LED or other indicators
3) Driving off-chip devices
4) Detect digital input
Pin Description:
Register Description:
GPIO contains 4 registers, as shown in the following table:
GPIO pin value register IOPIN:
GPIO output set register IOSET:
GPIO output clear register:
GPIO direction register:
Okay, that's it~~
Then here is today’s experiment:
Static display of a digital tube
I'm dizzy~~ I just can't adjust it well with IAR for ARM~~
shit~~
Then I switched to Keil~~
Waste a lot of my time~~
If I had known this earlier, I would have used Keil~~
I have to debug IAR again later~~
~~
Okay, post the picture~~
Then there is the procedure~~
MDK1_1.c (main program first, you know)
//------------------------------------------------------------------------------
//LED digital tube display
//Connect with 74HC595 through I/O analog synchronous serial interface, control 74HC595 to drive LED digital tube display
//------------------------------------------------------------------------------
#include"lpc210x.h"
typedef unsigned long uint32;
typedef unsigned char uchar;
#define SPI_IO 0x00000150 //SPI interface I/O setting word
uchar const seg[]={0xc0,0xf9,0xa4,0xb0,0x99,0x92,0x82,0xf8,
0x80,0x90,0x88,0x83,0xc6,0xa1,0x86,0x8e};
//------------------------------------------------------------------------------
//Delay function
void delay(uint32 z)
{
uint32 i;
for(;z>0;z--)
for(i=0;i<50000;i++);
}
//------------------------------------------------------------------------------
//main
int main()
{
uchar i;
PINSEL0=0X00000000;
PINSEL1=0X00000000; //Set the left and right pins to connect to GPIO
IODIR=SPI_IO; //Set the SPI control port to output~~Since this is simulated, you need to set the direction bit yourselfwhile
(1)
{
for(i=0;i<16;i++)
{
HC595_send_data(seg[i]);
delay(1);
}
}
}
74HC595.c
//------------------------------------------------------------------------------
//74HC595 simulates SPI communication for easy calling
#include "lpc210x.h"
typedef unsigned long uint32;
typedef unsigned char uchar;
#define SPI_CS 0x00000100 //P0.8 simulates chip select
#define SPI_DA 0x00000040 //P0.6 simulates data transmission port
#define SPI_CLK 0x00000010 //P0.4 simulates CLK
//------------------------------------------------------------------------------
//Send a byte function to 74HC595 (when sending data, the high bit is in front)
//Let's give a brief introduction to 74HC595:
//74HC595 has an 8-bit shift register and a memory, and a three-state output function.
//The shift register and memory are clocked separately.
//Data is input to the shift register at the rising edge of SH_CP and input to the storage register at the rising edge of ST_CP.
//If the two clocks are connected together, the shift register is always one pulse earlier than the storage register (usually it is not used this way~~).
//The shift register has a serial shift input (DS), a serial output (Q7'), and an asynchronous low-level reset.
//The storage register has a parallel 8-bit bus output with three states. When OE is enabled (low level), the data of the storage register is output to the bus.
void HC595_send_data(uchar dat)
{
uchar i;
IOCLR=SPI_CS; //SPI_CS=0
for(i=0;i<8;i++) //Simulate SPI~~
{
IOCLR=SPI_CLK; //SPI_CLK=0
if((dat&0x80)!=0) //Set the output value of SPI_DA
IOSET=SPI_DA; //To send from the highest bit, when the highest bit is 1, set SPI_DA
else
IOCLR=SPI_DA; //When the highest bit is 0, clear SPI_DA
dat<<=1; //dat is cyclically shifted left by one bit
IOSET=SPI_CLK; //SPI_CLK is 1, a pulse rising edge, the data is moved into the shift register
}
IOSET=SPI_CS; //SPI_CS=1, input to the storage register, because the chip select is always low, the displayed data is directly output
}
Previous article:ARM7 MCU (Learning) - (I) Input/output port GPIO programming - 02
Next article:ARM microcontroller (learning) - my first ARM7 microcontroller program
Recommended ReadingLatest update time:2024-11-15 16:46
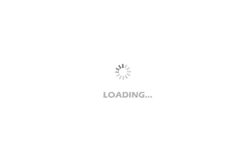
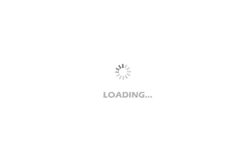
- Popular Resources
- Popular amplifiers
-
Wireless Sensor Network Technology and Applications (Edited by Mou Si, Yin Hong, and Su Xing)
-
Modern Electronic Technology Training Course (Edited by Yao Youfeng)
-
Modern arc welding power supply and its control
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- Huawei's Strategic Department Director Gai Gang: The cumulative installed base of open source Euler operating system exceeds 10 million sets
- Download from the Internet--ARM Getting Started Notes
- Learn ARM development(22)
- Learn ARM development(21)
- Learn ARM development(20)
- Learn ARM development(19)
- Learn ARM development(14)
- Learn ARM development(15)
- Analysis of the application of several common contact parts in high-voltage connectors of new energy vehicles
- Wiring harness durability test and contact voltage drop test method
- [Synopsys IP Resources] MIPI IP opens the security barrier of smart cars and perceives a clearer future
- The software timer of nrf51822 just doesn't work
- Can stm32f407zget use sd card and emmc at the same time?
- IIC Design Based on FPGA.pdf
- Domestic encryption chip recommendation, similar to ATECC508A with ECC encryption
- Application of high voltage amplifier based on piezoelectric ceramics in damage identification of dual block sleeper and ballast bed interface
- Electronic Engineering - Oscilloscope Learning and Using Tutorial Sharing
- 【Beetle ESP32-C3】5. Enable two UARTs (Arduino)
- Thank you for having you +EEWORLD
- [Runhe Neptune Review] Burning firmware with SecureCRT and Upgrade Tools respectively