test.c includes 3 header files
#include "stm32f10x_lib.h" some library functions of stm32
#include "sys.h" system clock initialization and some delay functions
#include "led.h" running light related programs
================================================== =======================
First look at stm32f10x_lib.h
It also includes a header file #include "stm32f10x_map.h"
Next is something like
#ifdef _ADC
#include "stm32f10x_adc.h"
#endif
#ifdef _BKP
#include "stm32f10x_bkp.h"
#endif
#ifdef _CAN
#include "stm32f10x_can.h"
#endif
........
#ifdef _GPIO If _GPIO is defined, include GPIO related header files. These macros are defined in stm32f10x_conf.h
#include "stm32f10x_gpio.h"
#endif
.....
Look at this header file stm32f10x_map.h
Also includes several header files
#include "stm32f10x_conf.h" similar to #define _GPIOA macro definition
#include "stm32f10x_type.h" similar to some data types of typedef signed long s32;
#include "cortexm3_macro.h"
stm32f10x_map.h defines
typedef struct
{
vu32 CRL;
vu32 CRH;
vu32 IDR;
vu32 ODR;
vu32 BSRR;
vu32 BRR;
vu32 LCKR;
} GPIO_TypeDef;
similar structure
......
#define PERIPH_BASE ((u32)0x40000000)
#define APB2PERIPH_BASE (PERIPH_BASE + 0x10000)
#define GPIOC_BASE (APB2PERIPH_BASE + 0x1000)
Then comes the important part
#ifdef _GPIOC
#define GPIOC ((GPIO_TypeDef *) GPIOC_BASE)
#endif
Convert the memory type starting from GPIOC_BASE to a structure of type GPIO_TypeDef
In led.h, call GPIOC->ODR=(GPIOC->ODR&~LED0)|(x ? LED0:0)
The address assignment of 0x40011000 + 12 completes the assignment of the corresponding register.
================================================== =================
Let's take a look at the simple register assignment in sys.h. It's easy to understand by referring to the manual.
//us delay function
void delay_us(unsigned int us)
{
u8 n;
while(us--)for(n=0;n
//ms delay function
void delay_ms(unsigned int ms)
{
while(ms--)delay_us(1000);
}
//Reset all clock registers
void RCC_DeInit(void)
{
RCC->APB2RSTR = 0x00000000; //Peripheral reset
RCC->APB1RSTR = 0x00000000;
RCC->AHBENR = 0x00000014; //Flash clock, flash clock enable. DMA clock off
RCC->APB2ENR = 0x00000000; //Peripheral clock off.
RCC->APB1ENR = 0x00000000;
RCC->CR |= 0x00000001; //Enable internal high-speed clock HSION
RCC->CFGR &= 0xF8FF0000; //Reset SW[1:0], HPRE[3:0], PPRE1[2:0], PPRE2[2:0], ADCPRE[1:0], MCO[2:0]
RCC->CR &= 0xFEF6FFFF; //Reset HSEON, CSSON, PLLON
RCC->CR &= 0xFFFBFFFF; //Reset HSEBYP
RCC->CFGR &= 0xFF80FFFF; //Reset PLLSRC, PLLXTPRE, PLLMUL[3:0] and USBPRE
RCC->CIR = 0x00000000; //Disable all interrupts
}
//External 8M, then get 72M system clock
void Stm32_Clock_Init(void)
{
unsigned char temp=0;
u8 timeout=0;
RCC_DeInit();
RCC->CR|=0x00010000; //External high speed clock enable HSEON
timeout=0;
while(!(RCC->CR>>17)&&timeout<200)timeout++;//Wait for external clock to be ready
//0-24M wait 0; 24-48M wait 1; 48-72M wait 2; (very important!)
FLASH->ACR|=0x32; //FLASH 2 delay cycles
RCC->CFGR|=0X001D2400;//APB1/2=DIV2;AHB=DIV1;PLL=9*CLK;HSE as PLL clock sourceRCC-
>CR|=0x01000000; //PLLON
timeout=0;
while(!(RCC->CR>>25)&&timeout<200)timeout++;//wait for PLL lock
RCC->CFGR|=0x00000002; //PLL as system clock
while(temp!=0x02&&timeout<200) //Wait for PLL to be set as system clock successfully
{
temp=RCC->CFGR>>2;
timeout++;
temp&=0x03;
}
}
================================================== ============================
led.h
#define LED0 (1<<10)// PC10
#define LED1 (1<<11)// PC11
#define LED2 (1<<12)// PC12
#define LED3 (1<<2) // PD2
#define LED0_SET(x) GPIOC->ODR=(GPIOC->ODR&~LED0)|(x ? LED0:0)
#define LED1_SET(x) GPIOC->ODR=(GPIOC->ODR&~LED1)|(x ? LED1: 0)
#define LED2_SET(x) GPIOC->ODR=(GPIOC->ODR&~LED2)|(x ? LED2:0) #define LED3_SET(x)
GPIOD->ODR=(GPIOD->ODR&~LED3)|( x ? LED3:0)
//LED IO initialization
void led_init(void)
{
RCC->APB2ENR|=1<<4; //Enable PORTC clock
GPIOC->CRH&=0XFFF000FF;
GPIOC->CRH|=0X00033300; //PC.10/11/12 push-pull output
GPIOC->ODR|= 0X1C00; //PC.10/11/12 output high
RCC->APB2ENR|=1<<5; //Enable PORTD clock
GPIOD->CRL&=0XFFFFF0FF;
GPIOD->CRL|=0X300; //PD.2 Push-pull output
GPIOD->ODR|=1<<2; //PD.2 output high
}
Previous article:STM32 Advanced Timer-PWM Simple Use
Next article:SPI debugging-74HC595 digital tube control experiment
Recommended ReadingLatest update time:2024-11-22 20:48
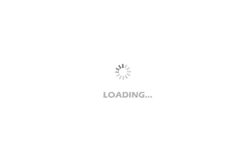
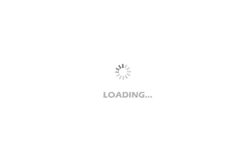
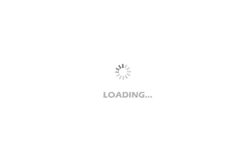
- Popular Resources
- Popular amplifiers
- Naxin Micro and Xinxian jointly launched the NS800RT series of real-time control MCUs
- How to learn embedded systems based on ARM platform
- Summary of jffs2_scan_eraseblock issues
- Application of SPCOMM Control in Serial Communication of Delphi7.0
- Using TComm component to realize serial communication in Delphi environment
- Bar chart code for embedded development practices
- Embedded Development Learning (10)
- Embedded Development Learning (8)
- Embedded Development Learning (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Intel promotes AI with multi-dimensional efforts in technology, application, and ecology
- ChinaJoy Qualcomm Snapdragon Theme Pavilion takes you to experience the new changes in digital entertainment in the 5G era
- Infineon's latest generation IGBT technology platform enables precise control of speed and position
- Two test methods for LED lighting life
- Don't Let Lightning Induced Surges Scare You
- Application of brushless motor controller ML4425/4426
- Easy identification of LED power supply quality
- World's first integrated photovoltaic solar system completed in Israel
- Sliding window mean filter for avr microcontroller AD conversion
- What does call mean in the detailed explanation of ABB robot programming instructions?
- STMicroelectronics discloses its 2027-2028 financial model and path to achieve its 2030 goals
- 2024 China Automotive Charging and Battery Swapping Ecosystem Conference held in Taiyuan
- State-owned enterprises team up to invest in solid-state battery giant
- The evolution of electronic and electrical architecture is accelerating
- The first! National Automotive Chip Quality Inspection Center established
- BYD releases self-developed automotive chip using 4nm process, with a running score of up to 1.15 million
- GEODNET launches GEO-PULSE, a car GPS navigation device
- Should Chinese car companies develop their own high-computing chips?
- Infineon and Siemens combine embedded automotive software platform with microcontrollers to provide the necessary functions for next-generation SDVs
- Continental launches invisible biometric sensor display to monitor passengers' vital signs
- Microwave RF filter classification and performance indicators
- Exploring embedded development with the Rust programming language - based on ESP32-S3
- 200W inverter power supply using TL494
- Ultra-wideband technology helps smart cities: positioning in the future is so simple
- To output such a carrier waveform, how to modulate GPIO17 and GPIO24? What frequency, pulse width, and duty cycle should be given? Software...
- Add USB to any device you want
- A51 MCU Programmer
- Recalling the teachers who taught me
- Answer the questions to win prizes | TDK special reports are waiting for you (Issue 3)
- Questions about the 2x in-phase proportional operational amplifier composed of OPA380