HC_SR04 is a widely used ultrasonic ranging module. The module diagram is as follows
The module has four pins, namely VCC GND TRIG ECHO, where VCC GND is the power supply pin
TRIG is the ranging trigger pin, ECHO is the ranging input pin
The drive mode of this module is
The control port sends a high level of more than 10US, and then you can wait for the high level output at the receiving port. Once there is an output, you can start the timer. When this port becomes a low level, you can read the timer value, which is the time of this distance measurement, and then you can calculate the distance. By continuously measuring in this way, you can reach the value of your mobile measurement.
The module works as follows
(1) Use IO to trigger the ranging and provide a high-level signal of at least 10us;
(2) The module automatically sends 8 40khz square waves and automatically detects whether there is a signal return;
(3) When a signal is returned, a high level is output through IO. The duration of the high level is the time from the ultrasonic wave being emitted to the ultrasonic wave being returned.
(4 Calculate the test distance Test distance = (high level time * sound speed (340M/S))/2;
According to the working principle, we can choose two modes to drive
1. Use the interrupt + timer method, define ECHO as both the rising and falling edges can trigger interrupts. After trig is triggered, echo high level will interrupt and turn on the timer, echo low level will turn off the timer and count the timer count value
2. Use normal IO+timer mode, wait for echo response after triggering, turn on the timer when responding, and turn off the timer until echo returns to low, and get the time
1: This module should not be connected with power on. If it is to be connected with power on, connect the Gnd end of the module first. Otherwise, it will affect
Modules work.
2: When measuring distance, the area of the object to be measured should be no less than 0.5 square meters and should be as flat as possible. Otherwise, the test results will be affected.
The detailed driver code is as follows
Hcsr04.c
#include "hcsr04.h"
#define HCSR04_PORT GPIOB
#define HCSR04_CLK RCC_APB2Periph_GPIOB
#define HCSR04_TRIG GPIO_Pin_5
#define HCSR04_ECHO GPIO_Pin_6
#define TRIG_Send PBout(5)
#define ECHO_Reci PBin(6)
u16 msHcCount = 0; //ms count
void Hcsr04Init(u32 NVIC_PriorityGroup,u32 PreemptionPriority,u32 SubPriority)
{
u32 NVICtemp = 0; //Variables for NVIC controller
TIM_TimeBaseInitTypeDef TIM_TimeBaseStructure; //Generate a structure for timer settings
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(HCSR04_CLK, ENABLE);
NVICtemp = NVIC_EncodePriority(NVIC_PriorityGroup, PreemptionPriority, SubPriority); //Interrupt priority variable decoding
//IO initialization
GPIO_InitStructure.GPIO_Pin =HCSR04_TRIG; //Send level pin
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP; //Push-pull output
GPIO_Init(HCSR04_PORT, &GPIO_InitStructure);
GPIO_ResetBits(HCSR04_PORT,HCSR04_TRIG);
GPIO_InitStructure.GPIO_Pin = HCSR04_ECHO; //Return level pin
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPD; //Pull-down input
GPIO_Init(HCSR04_PORT, &GPIO_InitStructure);
//Timer initialization using basic timer TIM6
RCC_APB1PeriphClockCmd(RCC_APB1Periph_TIM6, ENABLE); //Enable the corresponding RCC clock
//Configure the timer infrastructure structure
TIM_TimeBaseStructure.TIM_Period = 1000; //Set the value of the auto-reload register period to load the activity at the next update event. Count to 1000 for 1ms
TIM_TimeBaseStructure.TIM_Prescaler = (72-1); //Set the prescaler value used as the TIMx clock frequency divisor 1M counting frequency 1US counting
TIM_TimeBaseStructure.TIM_CounterMode = TIM_CounterMode_Up; //TIM up counting mode
TIM_TimeBaseInit(TIM6, &TIM_TimeBaseStructure); //Initialize the time base unit of TIMx according to the parameters specified in TIM_TimeBaseInitStruct
TIM_ClearFlag(TIM6, TIM_FLAG_Update); //Clear the update interrupt to avoid an interrupt being generated immediately after the interrupt is enabled
TIM_ITConfig(TIM6,TIM_IT_Update,ENABLE); //Enable timer update interrupt
NVIC_SetPriority(TIM6_IRQn,NVICtemp); //Set interrupt priority
NVIC_EnableIRQ(TIM6_IRQn); //Enable the corresponding interrupt
}
static void OpenTimerForHc() //Open the timer
{
TIM_SetCounter(TIM6,0); //Clear count
msHcCount = 0;
TIM_Cmd(TIM6, ENABLE); //Enable TIMx peripherals
}
static void CloseTimerForHc() //Close the timer
{
TIM_Cmd(TIM6, DISABLE); //Enable TIMx peripherals
}
//Timer 6 interrupt service routine
void TIM6_IRQHandler(void) //TIM3 interrupt
{
if (TIM_GetITStatus(TIM6, TIM_IT_Update) != RESET) //Check whether TIM3 update interrupt occurs
{
TIM_ClearITPendingBit(TIM6, TIM_IT_Update ); //Clear TIMx update interrupt flag
msHcCount++;
}
}
u32 GetEchoTimer(void)
{
u32 t = 0;
t = msHcCount*1000; //Get MS
t += TIM_GetCounter(TIM6); //Get US
return t;
}
//Get ultrasonic distance measurement data once. There needs to be a period of time between two distance measurements to cut off the echo signal.
void Hcsr04GetLength(u32* length)
{
u32 t = 0;
float lengthTemp;
TRIG_Send = 1; //Send port high level output
DelayUs(10);
TRIG_Send = 0;
while(ECHO_Reci == 0); //Wait for the receiving port to output high level
OpenTimerForHc(); //Open the timer
while(ECHO_Reci == 1);
CloseTimerForHc(); //Close the timer
t = GetEchoTimer(); //Get time, resolution is 1US
lengthTemp = ((float)t/58);//cm
*length = (u32)lengthTemp;
}
Hcsr04.h
#ifndef __HCSR04_H
#define __HCSR04_H
#include "stm32f10x.h"
#include "delay.h"
#include "ioremap.h"
#include "common.h"
//Ultrasonic module initialization
void Hcsr04Init(u32 NVIC_PriorityGroup,u32 PreemptionPriority,u32 SubPriority);
//Ultrasonic module once distance acquisition
//Return 0 Success
//Return 1 if failed
void Hcsr04GetLength(u32* length);
#endif
Previous article:RDA5820 radio chip driver
Next article:USB custom HID device implementation-STM32
Recommended ReadingLatest update time:2024-11-16 17:47
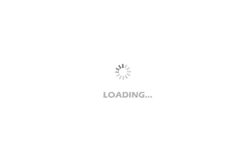
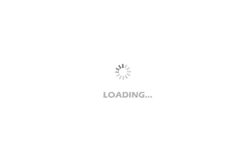
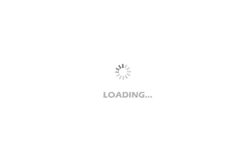
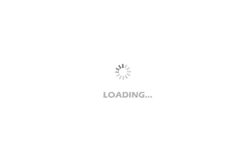
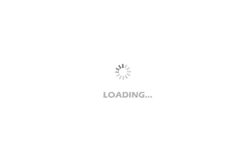
- Popular Resources
- Popular amplifiers
-
Siemens Motion Control Technology and Engineering Applications (Tongxue, edited by Wu Xiaojun)
-
Modern Product Design Guide
-
Modern arc welding power supply and its control
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- A rather strange op amp, take a look
- Undergraduate entrance examination
- Bill of Materials Problem
- Direct Memory Access (DMA) Controller - TMS320VC5509A
- A must-read for beginners! Experts explain the PCB return path for high-speed circuits
- Two major power outages in five days! The Taiwan authorities have asked TSMC to relocate or suspend some production lines
- PADS PCB 3D component library
- Frequency division of analog signals
- C2000-GNAG Operation and Use
- [ESK32-360 Review] 6. Hello! Hello! Hello! Hello!