This involves a very important register, the clock configuration register: RCC_CFGR
1 #if defined (STM32F10X_LD_VL) || (defined STM32F10X_MD_VL) || (defined STM32F10X_HD_VL)
2 /* #define SYSCLK_FREQ_HSE HSE_VALUE */
3 #define SYSCLK_FREQ_24MHz 24000000
4 #else
5 /* #define SYSCLK_FREQ_HSE HSE_VALUE */
6 /* #define SYSCLK_FREQ_24MHz 24000000 */
7 /* #define SYSCLK_FREQ_36MHz 36000000 */
8 /* #define SYSCLK_FREQ_48MHz 48000000 */
9 /* #define SYSCLK_FREQ_56MHz 56000000 */
10 #define SYSCLK_FREQ_72MHz 72000000
11 #endif
1 /**
2 * @brief Setup the microcontroller system
3 * Initialize the Embedded Flash Interface, the PLL and update the
4 * SystemCoreClock variable.
5 * @note This function should be used only after reset.
6 * @param None
7 * @retval None
8 */
9 void SystemInit (void)
10 {
11 /* Reset the RCC clock configuration to the default reset state(for debug purpose) */
12 /* Set HSION bit */
13 RCC->CR |= (uint32_t)0x00000001;
14
15 /* Reset SW, HPRE, PPRE1, PPRE2, ADCPRE and MCO bits */
16 #ifndef STM32F10X_CL
17 RCC->CFGR &= (uint32_t)0xF8FF0000;
18 #else
19 RCC->CFGR &= (uint32_t)0xF0FF0000;
20 #endif /* STM32F10X_CL */
21
22 /* Reset HSEON, CSSON and PLLON bits */
23 RCC->CR &= (uint32_t)0xFEF6FFFF;
24
25 /* Reset HSEBYP bit */
26 RCC->CR &= (uint32_t)0xFFFBFFFF;
27
28 /* Reset PLLSRC, PLLXTPRE, PLLMUL and USBPRE/OTGFSPRE bits */
29 RCC->CFGR &= (uint32_t)0xFF80FFFF;
30
31 #ifdef STM32F10X_CL
32 /* Reset PLL2ON and PLL3ON bits */
33 RCC->CR &= (uint32_t)0xEBFFFFFF;
34
35 /* Disable all interrupts and clear pending bits */
36 RCC->CIR = 0x00FF0000;
37
38 /* Reset CFGR2 register */
39 RCC->CFGR2 = 0x00000000;
40 #elif defined (STM32F10X_LD_VL) || defined (STM32F10X_MD_VL) || (defined STM32F10X_HD_VL)
41 /* Disable all interrupts and clear pending bits */
42 RCC->CIR = 0x009F0000;
43
44 /* Reset CFGR2 register */
45 RCC->CFGR2 = 0x00000000;
46 #else
47 /* Disable all interrupts and clear pending bits */
48 RCC->CIR = 0x009F0000;
49 #endif /* STM32F10X_CL */
50
51 #if defined (STM32F10X_HD) || (defined STM32F10X_XL) || (defined STM32F10X_HD_VL)
52 #ifdef DATA_IN_ExtSRAM
53 SystemInit_ExtMemCtl();
54 #endif /* DATA_IN_ExtSRAM */
55 #endif
56
57 /* Configure the System clock frequency, HCLK, PCLK2 and PCLK1 prescalers */
58 /* Configure the Flash Latency cycles and enable prefetch buffer */
59 SetSysClock();
60
61 #ifdef VECT_TAB_SRAM
62 SCB->VTOR = SRAM_BASE | VECT_TAB_OFFSET; /* Vector Table Relocation in Internal SRAM. */
63 #else
64 SCB->VTOR = FLASH_BASE | VECT_TAB_OFFSET; /* Vector Table Relocation in Internal FLASH. */
65 #endif
66 }
/**
* @brief Configures the System clock frequency, HCLK, PCLK2 and PCLK1 prescalers.
* @param None
* @retval None
*/
static void SetSysClock(void)
{
#ifdef SYSCLK_FREQ_HSE
SetSysClockToHSE();
#elif defined SYSCLK_FREQ_24MHz
SetSysClockTo24();
#elif defined SYSCLK_FREQ_36MHz
SetSysClockTo36();
#elif defined SYSCLK_FREQ_48MHz
SetSysClockTo48();
#elif defined SYSCLK_FREQ_56MHz
SetSysClockTo56();
#elif defined SYSCLK_FREQ_72MHz
SetSysClockTo72();
#endif
Set the bit parameters of the RCC_CFGR register to match the external crystal oscillator to obtain a 72M system clock.
1 /**
2 * @brief Sets System clock frequency to 72MHz and configure HCLK, PCLK2
3 * and PCLK1 prescalers.
4 * @note This function should be used only after reset.
5 * @param None
6 * @retval None
7 */
8 static void SetSysClockTo72(void)
9 {
10 __IO uint32_t StartUpCounter = 0, HSEStatus = 0;
11
12 /* SYSCLK, HCLK, PCLK2 and PCLK1 configuration ---------------------------*/
13 /* Enable HSE */
14 RCC->CR |= ((uint32_t)RCC_CR_HSEON);
15
16 /* Wait till HSE is ready and if Time out is reached exit */
17 do
18 {
19 HSEStatus = RCC->CR & RCC_CR_HSERDY;
20 StartUpCounter++;
21 } while((HSEStatus == 0) && (StartUpCounter != HSE_STARTUP_TIMEOUT));
22
23 if ((RCC->CR & RCC_CR_HSERDY) != RESET)
24 {
25 HSEStatus = (uint32_t)0x01;
26 }
27 else
28 {
29 HSEStatus = (uint32_t)0x00;
30 }
31
32 if (HSEStatus == (uint32_t)0x01)
33 {
34 /* Enable Prefetch Buffer */
35 FLASH->ACR |= FLASH_ACR_PRFTBE;
36
37 /* Flash 2 wait state */
38 FLASH->ACR &= (uint32_t)((uint32_t)~FLASH_ACR_LATENCY);
39 FLASH->ACR |= (uint32_t)FLASH_ACR_LATENCY_2;
40
41
42 /* HCLK = SYSCLK */
43 RCC->CFGR |= (uint32_t)RCC_CFGR_HPRE_DIV1; //AHB one frequency division
44
45 /* PCLK2 = HCLK */
46 RCC->CFGR |= (uint32_t)RCC_CFGR_PPRE2_DIV1;
47
48 /* PCLK1 = HCLK */
49 RCC->CFGR |= (uint32_t)RCC_CFGR_PPRE1_DIV2;
50
51 #ifdef STM32F10X_CL
52 /* Configure PLLs ------------------------------------------------------*/
53 /* PLL2 configuration: PLL2CLK = (HSE / 5) * 8 = 40 MHz */
54 /* PREDIV1 configuration: PREDIV1CLK = PLL2 / 5 = 8 MHz */
55
56 RCC->CFGR2 &= (uint32_t)~(RCC_CFGR2_PREDIV2 | RCC_CFGR2_PLL2MUL |
57 RCC_CFGR2_PREDIV1 | RCC_CFGR2_PREDIV1SRC);
58 RCC->CFGR2 |= (uint32_t)(RCC_CFGR2_PREDIV2_DIV5 | RCC_CFGR2_PLL2MUL8 |
59 RCC_CFGR2_PREDIV1SRC_PLL2 | RCC_CFGR2_PREDIV1_DIV5);
60
61 /* Enable PLL2 */
62 RCC->CR |= RCC_CR_PLL2ON;
63 /* Wait till PLL2 is ready */
64 while((RCC->CR & RCC_CR_PLL2RDY) == 0)
65 {
66 }
67
68
69 /* PLL configuration: PLLCLK = PREDIV1 * 9 = 72 MHz */
70 RCC->CFGR &= (uint32_t)~(RCC_CFGR_PLLXTPRE | RCC_CFGR_PLLSRC | RCC_CFGR_PLLMULL);
71 RCC->CFGR |= (uint32_t)(RCC_CFGR_PLLXTPRE_PREDIV1 | RCC_CFGR_PLLSRC_PREDIV1 |
72 RCC_CFGR_PLLMULL9);
73 #else
74 /* PLL configuration: PLLCLK = HSE * 9 = 72 MHz */
75 RCC->CFGR &= (uint32_t)((uint32_t)~(RCC_CFGR_PLLSRC | RCC_CFGR_PLLXTPRE |
76 RCC_CFGR_PLLMULL));
77 RCC->CFGR |= (uint32_t)(RCC_CFGR_PLLSRC_HSE | RCC_CFGR_PLLMULL9);
78 #endif /* STM32F10X_CL */
79
80 /* Enable PLL */
81 RCC->CR |= RCC_CR_PLLON;
82
83 /* Wait till PLL is ready */
84 while((RCC->CR & RCC_CR_PLLRDY) == 0)
85 {
86 }
87
88 /* Select PLL as system clock source */
89 RCC->CFGR &= (uint32_t)((uint32_t)~(RCC_CFGR_SW));
90 RCC->CFGR |= (uint32_t)RCC_CFGR_SW_PLL;
91
92 /* Wait till PLL is used as system clock source */
93 while ((RCC->CFGR & (uint32_t)RCC_CFGR_SWS) != (uint32_t)0x08)
94 {
95 }
96 }
97 else
98 { /* If HSE fails to start-up, the application will have wrong clock
99 configuration. User can add here some code to deal with this error */
100 }
101 }
Previous article:STM32 Hardware IIC
Next article:Bit-band operation in STM32
Recommended ReadingLatest update time:2024-11-16 17:52
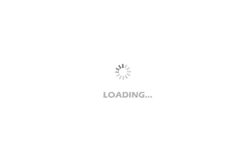
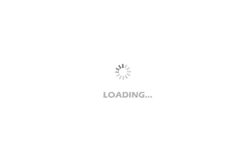
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- First picture of the start-up - high voltage motor drive
- [RVB2601 Creative Application Development] VI. Sound Playback Test of RVB2601
- MicroPython adds instructions for pyboard.py
- SD/MMC SPI mode command set
- DS90UB954 debugging problem help
- Microsecond latency, wireless communication solution
- Use STM32 Universal Bootloader to make OTA easier
- MLCC Noise Howling and Countermeasures
- Design of brushless DC motor driver based on hardware FOC TMC4671
- [ART-Pi Review] VI: Review of ATT-Pi's onboard Flash file system and FTP functions in RTT Studio environment