The interaction between the microcontroller and peripherals is carried out through the I/O port. Each I/O port has three operation registers:
1. TRISx ——— Data direction register
Used to control the direction of the I/O pin, that is, to control whether PORTx is input or output.
2. PORTx ——— Port register
Used to latch output data. When reading PORTx, the device reads the I/O pin level directly (not the latched value).
3. LATx ——— Output data latch
Writing to the port is writing to this latch (LATx). The data latch can also be read and written directly. If the peripheral does not use the pin and the TRISx bit configures the pin as an output, the data in the latch is output to the pin.
In the reset state, the reset value of TRISx is 0xff, that is, the values of the 8 bits (D0 ~ D7) of the TRISx register are all 1. At this time, the corresponding PORTx pin is defined as input, and the corresponding output driver is in high impedance state. When set to 0, it means that the corresponding pin is defined as output.
It should be noted here that writing PORT is writing LAT, but reading PORT is different from reading LAT. Reading PORT reads the state of the pin, whether the pin is set as an input pin or an output pin. Reading LAT, on the other hand, gets the stored value of the output data latch. The value obtained by reading LAT may be different from the value obtained by reading PORT.
In Microchip C18, the three operation registers of the I/O port can be operated bit by bit or byte by byte.
For example, the direction register of port B is represented by TRISB (or DDRB), and a certain bit is represented by TRISBbits.TRISB0 (or DDRB bits.RB0). Bytes are represented by TRISB (or DDRB).
For example, the PORT register of port B is represented by PORTB, and a certain bit is represented by PORTBbits.RB0. The byte is represented by PORTB.
For example, the output data latch of port B is represented by LATB, a certain bit is represented by LATBbits. LATB0. Bytes are represented by LATB.
After the chip is reset, the value of the LATx (PORTx) latch is random. In order to eliminate the possibility of glitches on the I/O pin level, when initializing the port, first initialize the PORT data latch (LAT or PORT register) and then initialize the data direction register TRIS.
The following example illustrates a specific application. In the figure below, PIC18F4550, power supply, crystal oscillator and light-emitting diodes form the simplest 8-bit microcontroller system, which requires lighting up 8 light-emitting diodes at the same time.
First, we can choose the bitwise operation method to implement it. It is not difficult to see that the bitwise operation does not actually realize the simultaneous lighting of the 8 LEDs connected to PORTB. It is just that the delay effect of the LEDs covering up the fact of sequential lighting makes the final effect reach the simultaneous lighting. The following is the implementation code of the bitwise operation method.
#include
void main(void)
{
PORTBbits.RB0=1;
TRISBbits.TRISB0=0; //Turn on the 1st LED
PORTBbits.RB1=1;
TRISBbits.TRISB1=0; //Turn on the 2nd LED
PORTBbits.RB2=1;
TRISBbits.TRISB2=0; //Turn on the 3rd LED
PORTBbits.RB3=1;
TRISBbits.TRISB3=0; //Turn on the 4th LED
PORTBbits.RB4=1;
TRISBbits.TRISB4=0; //Turn on the 5th LED
PORTBbits.RB5=1;
TRISBbits.TRISB5=0; //Turn on the 6th LED
PORTBbits.RB6=1;
TRISBbits.TRISB6=0; //Turn on the 7th LED
PORTBbits.RB7=1;
TRISBbits.TRISB7=0; //Light up the 8th LED
while(1);
}
Secondly, it can be implemented by byte operation. The code is much simpler than bit operation, and it can truly achieve the requirement of lighting up at the same time. The following is the implementation code of byte operation.
#include
void main(void)
{
PORTB=0xff;
TRISB=0x00; //Light up 8 LEDs
while(1);
}
Previous article:Use of PIC Timer
Next article:Four reasons to choose PIC microcontroller
Recommended ReadingLatest update time:2024-11-16 13:34
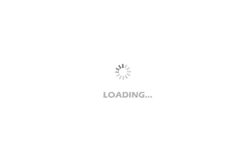
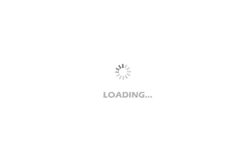
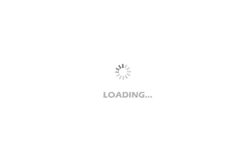
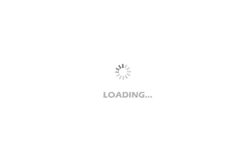
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- CPLD chip EPM240T100C5
- [RVB2601 Creative Application Development] Four SGP30 environmental sensors can take eCO2 and TVOC
- TWS headset charging case schematic diagram 1
- Design of cell image acquisition system based on DSP and FPGA technology
- Raspberry Pi Pico W
- Linear optocoupler isolation voltage problem
- 【ESP32-C3-DevKitM-1】ESP32-C3 connects to MQTT server
- MATLAB APP Designer serial port debugging tool writing
- [Zero-knowledge ESP8266 tutorial] Quick start 1-Let your development board say hello to the world
- EEWORLD University Hall----Engineering is smarter, industrial design is more powerful: TI robot system