#include
#define uint unsigned int
#define uchar unsigned char
#define input RA3
#define clk RA5
#define cs_led RE0
__CONFIG(0x3B31);
void init();
void delay(uint);
void write_164(uchar);
uint intnum1,intnum2;
void main()
{
heat();
while(1)
{
if(T0IF==1) //Judge whether the interrupt overflow bit overflows. Whether TOIF overflows has nothing to do with whether the total interrupt is enabled.
{
T0IF=0; //need software clear
intnum1++;
if(intnum1==3906)//One second has passed
{
intnum1=0;
intnum2++;
cs_led=0;
if(intnum2==1)
write_164(0xfd);
if(intnum2==2)
{
intnum2=0;
write_164(0xff);
}
}
}
}
}
void init()
{
TRISA=0b11010111;
TRISE=0b11111110;
OPTION=0x08; //Use the internal clock signal, and assign the prescaler to the WDT module, which is equivalent to not setting the prescaler for TM0.
//One clock cycle is one second. When the initial value is not loaded, it overflows after 256 microseconds because it is an 8-bit timer.
}
void delay(uint x)
{
uint a,b;
for(a=x;a>0;a--)
for(b=110;b>0;b--);
}
void write_164(uchar dt)
{
flying i;
for(i=0;i<8;i++)
{
clk=0;
if(dt&0x80)
input=1;
else
input=0;
dt=dt<<1;
clk=1;
}
}
TIM0 query method makes the LED flash for one second, using pre-scaling
#include
#define uint unsigned int
#define uchar unsigned char
#define input RA3
#define clk RA5
#define cs_led RE0
__CONFIG(0x3B31);
void init();
void delay(uint);
void write_164(uchar);
uint intnum1,intnum2;
void main()
{
heat();
while(1)
{
if(T0IF==1) //Judge whether the interrupt overflow bit overflows. Whether TOIF overflows has nothing to do with whether the total interrupt is enabled.
{
T0IF=0; //need software clear
TMR0=61; //Re-initialize the timer.
intnum1++;
if(intnum1==20)//One second has passed
{
intnum1=0;
intnum2++;
cs_led=0;
if(intnum2==1)
write_164(0xfd);
if(intnum2==2)
{
intnum2=0;
write_164(0xff);
}
}
}
}
}
void init()
{
TRISA=0b11010111;
TRISE=0b11111110;
OPTION=0x07; //Use the internal clock signal, the prescaler is assigned to the TIM0 module, and the frequency is divided by 256.
//One clock cycle is one second. When the initial value is not loaded, it overflows after 256 microseconds because it is an 8-bit timer.
TMR0=61; //256*Y=50000, =>Y=195, 256-195=61, so overflow occurs once every 50ms, and overflow occurs 20 times, which equals 1s.
}
void delay(uint x)
{
uint a,b;
for(a=x;a>0;a--)
for(b=110;b>0;b--);
}
void write_164(uchar dt)
{
flying i;
for(i=0;i<8;i++)
{
clk=0;
if(dt&0x80)
input=1;
else
input=0;
dt=dt<<1;
clk=1;
}
}
TIM0 interrupt method makes the LED flash for one second, using pre-scaling
#include
#define uint unsigned int
#define uchar unsigned char
#define input RA3
#define clk RA5
#define cs_led RE0
__CONFIG(0x3B31);
void init();
void delay(uint);
void write_164(uchar);
uint intnum1,intnum2;
void main()
{
heat();
while(1)
{
if(intnum1==2)//One second has passed
{
intnum1=0;
intnum2++;
cs_led=0;
if(intnum2==1)
write_164(0xfd);
if(intnum2==2)
{
intnum2=0;
write_164(0xff);
}
}
}
}
void init()
{
TRISA=0b11010111;
TRISE=0b11111110;
OPTION=0x07; //Use the internal clock signal, the prescaler is assigned to the TIM0 module, and the frequency is divided by 256.
//One clock cycle is one second. When the initial value is not loaded, it overflows after 256 microseconds because it is an 8-bit timer.
INTCON=0xa0; //GIE=1, turn on the general interrupt, T0IE=1, turn on the T0 interrupt, T0IE is the TMR0 overflow interrupt enable bit.
TMR0=61; //256*Y=50000, =>Y=195, 256-195=61, so overflow occurs once every 50ms, and overflow occurs 20 times, which equals 1s.
}
void interrupt time0()
{
T0IF=0; //Since only TMR0 interrupt is enabled, there is no need to check which interrupt it is. The interrupt that comes in must be TMR0 overflow interrupt, so clear the interrupt overflow flag directly.
TMR0=61;
intnum1++;
}
void delay(uint x)
{
uint a,b;
for(a=x;a>0;a--)
for(b=110;b>0;b--);
}
void write_164(uchar dt)
{
flying i;
for(i=0;i<8;i++)
{
clk=0;
if(dt&0x80)
input=1;
else
input=0;
dt=dt<<1;
clk=1;
}
}
The TMR1 interrupt method and the TIM0 interrupt method make the LED flash for one second without setting the prescaler.
#include
#define uint unsigned int
#define uchar unsigned char
#define input RA3
#define clk RA5
#define cs_led RE0
__CONFIG(0x3B31);
void init();
void delay(uint);
void write_164(uchar);
uint intnum1,intnum2;
void main()
{
heat();
while(1)
{
if(intnum1==20)//One second has passed
{
intnum1=0;
intnum2++;
cs_led=0;
if(intnum2==1)
write_164(0xfd);
if(intnum2==2)
{
intnum2=0;
write_164(0xff);
}
}
}
}
void init()
{
TRISA=0b11010111;
TRISE=0b11111110;
INTCON=0xc0; //GIE=1, open the general interrupt, open the first peripheral interrupt
PIE1=0x01; // Enable the interrupt of timer 1
TMR1L=(65536-50000)%256;
TMR1H=(65536-50000)/256; //Enter an interrupt, which is 50ms,
T1CON=0x01; //Do not set pre-scaling, turn off the crystal enable control bit of timer 1, synchronize with the external clock, select the internal clock, enable timer 1,
}
void interrupt time1()
{
TMR1IF=0; // Clear the interrupt overflow flag.
TMR1L=(65536-50000)%256;
TMR1H=(65536-50000)/256;
intnum1++;
}
void delay(uint x)
{
uint a,b;
for(a=x;a>0;a--)
for(b=110;b>0;b--);
}
void write_164(uchar dt)
{
flying i;
for(i=0;i<8;i++)
{
clk=0;
if(dt&0x80)
input=1;
else
input=0;
dt=dt<<1;
clk=1;
}
}
TMR1 interrupt method TIM0 interrupt method makes LED flash for 400ms and sets pre-scaling
#include
#define uint unsigned int
#define uchar unsigned char
#define input RA3
#define clk RA5
#define cs_led RE0
__CONFIG(0x3B31);
void init();
void delay(uint);
void write_164(uchar);
uint intnum1,intnum2;
void main()
{
heat();
while(1)
{
/* if(intnum1==20)//One second has passed
{
intnum1=0;
intnum2++;
cs_led=0;
if(intnum2==1)
write_164(0xfd);
if(intnum2==2)
{
intnum2=0;
write_164(0xff);
}
}*/
}
}
void init()
{
TRISA=0b11010111;
TRISE=0b11111110;
INTCON=0xc0; //GIE=1, open the general interrupt, open the first peripheral interrupt
PIE1=0x01; // Enable the interrupt of timer 1
TMR1L=(65536-50000)%256;
TMR1H=(65536-50000)/256; //If the pre-scaling frequency is not set, the interruption time is 50ms. Now set the pre-scaling frequency to 8 times, and the interruption time is 400ms.
T1CON=0x31; //Set 8 times pre-scaling, turn off the crystal enable control bit of timer 1, synchronize with the external clock, select the internal clock, enable timer 1,
}
void interrupt time1()
{
TMR1IF=0; // Clear the interrupt overflow flag.
TMR1L=(65536-50000)%256;
TMR1H=(65536-50000)/256;
//intnum1++;
intnum2++;
cs_led=0;
if(intnum2==1)
write_164(0xfd);
if(intnum2==2)
{
intnum2=0;
write_164(0xff);
}
}
void delay(uint x)
{
uint a,b;
for(a=x;a>0;a--)
for(b=110;b>0;b--);
}
void write_164(uchar dt)
{
flying i;
for(i=0;i<8;i++)
{
clk=0;
if(dt&0x80)
input=1;
else
input=0;
dt=dt<<1;
clk=1;
}
}
TMR2 pre-scaling and post-scaling
#include
#define uint unsigned int
#define uchar unsigned char
#define input RA3
#define clk RA5
#define cs_led RE0
__CONFIG(0x3B31);
void init();
void delay(uint);
void write_164(uchar);
uint intnum1,intnum2;
void main()
{
heat();
while(1)
{
if(intnum1==1000) //Originally, when the pre-scaling frequency was 1:1, it was 200ms. Now the pre-scaling frequency is 4. So it is 200*4 ms. Since the post-scaling frequency is 1:2, it is 200*4*2 ms
{
intnum1=0;
intnum2++;
cs_led=0;
if(intnum2==1)
write_164(0xfd);
if(intnum2==2)
{
intnum2=0;
write_164(0xff);
}
}
}
}
void init()
{
TRISA=0b11010111;
TRISE=0b11111110;
INTCON=0xc0; //GIE=1, open the general interrupt, open the first peripheral interrupt peripheral function module interrupt
PIE1=0x02; // Enable the interrupt of timer 2
TMR2=56;
T2CON = 0x0d; // Pre-scaling 1:4, enable tmr2 count enable/disable control bit, pre-scaling 1:4 post-scaling 1:2,
}
void interrupt time1()
{
TMR2IF=0; // Clear the interrupt overflow flag.
TMR2=56;
intnum1++;
}
void delay(uint x)
{
uint a,b;
for(a=x;a>0;a--)
for(b=110;b>0;b--);
}
void write_164(uchar dt)
{
flying i;
for(i=0;i<8;i++)
{
clk=0;
if(dt&0x80)
input=1;
else
input=0;
dt=dt<<1;
clk=1;
}
}
TMR2 Prescaler and Postscaler Period Register
#include
#define uint unsigned int
#define uchar unsigned char
#define input RA3
#define clk RA5
#define cs_led RE0
__CONFIG(0x3B31);
void init();
void delay(uint);
void write_164(uchar);
uint intnum1,intnum2;
void main()
{
heat();
while(1)
{
if(intnum1==1000) //Originally, when the pre-scaling frequency was 1:1, 100 ms had arrived. Now the pre-scaling frequency is 4, so 100*4 ms has arrived. Since the post-scaling frequency is 1:2, it is 100*4*2 ms
{
intnum1=0;
intnum2++;
cs_led=0;
if(intnum2==1)
write_164(0xfd);
if(intnum2==2)
{
intnum2=0;
write_164(0xff);
}
}
}
}
void init()
{
TRISA=0b11010111;
TRISE=0b11111110;
INTCON=0xc0; //GIE=1, open the general interrupt, open the first peripheral interrupt peripheral function module interrupt
PIE1=0x02; // Enable the interrupt of timer 2
TMR2=0;
PR2=100; //Period register
T2CON = 0x0d; // Pre-scaling 1:4, enable tmr2 count enable/disable control bit, pre-scaling 1:4 post-scaling 1:2,
}
void interrupt time1()
{
TMR2IF=0; // Clear the interrupt overflow flag.
//TMR2=56;
intnum1++;
}
void delay(uint x)
{
uint a,b;
for(a=x;a>0;a--)
for(b=110;b>0;b--);
}
void write_164(uchar dt)
{
flying i;
for(i=0;i<8;i++)
{
clk=0;
if(dt&0x80)
input=1;
else
input=0;
dt=dt<<1;
clk=1;
}
}
Previous article:Application of PIC interrupt (Part 2)
Next article:PIC 18XXX PORT LAT
Recommended ReadingLatest update time:2024-11-16 15:57
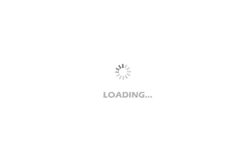
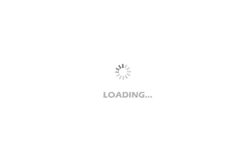
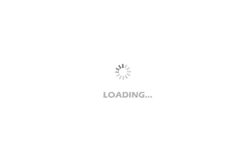
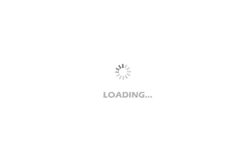
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Kubo MS6908 perfect PIN2PIN replacement MP6908
- Research and FPGA Design of Image Scaling Algorithm
- Decoding Analysis and Simulation of 315Mhz and 433Mhz Wireless Remote Control Signals
- 【AT32WB415 Review】Bluetooth Communication and Test 2
- [Dry Goods] Analysis of Commonly Used Formulas in Power Supply Design
- Official announcement: "Keysight Thanksgiving Month", where hundreds of instruments are given away every year, is here again!
- 28335 development board problem
- 【Project source code】CRC32 calculation based on FPGA
- Download and get a gift | TE pressure sensor solutions
- ST sensor driver package, official C-Driver-MEMS