#include "delay.h"
#include "delay.c"
#include
#include
//Fuse configuration***********************************************************
__CONFIG(XT & WDTDIS & PWRTEN & PROTECT);
//Pin definition************************************************************
#define RS RB4 //Select register
#define EN RB5 //Signal enable
//Clock chip********************************************************
#define RST RA0 //Chip enable
#define CLK RA1 //Chip clock
#define DAT RA2 //Chip data
//Display characters********************************************************
unsigned char TopChar[] = {" "}; //Display buffer
unsigned char BotChar[] = {" "}; //
//********************************************************************
//Function name: portInit();
//Input parameters: None
//Output parameters: None
//Function description: Port settings
//Build date: 2008.12.12
//********************************************************************
void PortInit(void)
{
PORTA = 0x00; //Port settings
PORTB = 0x00; //
TRISA = 0x00; //Direction settings
TRISB = 0x00; //
OPTION = 0x00; //pull up to enable
}
//*******************************************************************
//Function name: WriteData(data, rs);
//Input parameter: data to be written, 0 = instruction 1 = data
//Output parameter: None
//Function description: Write 1602LCD
//Build date: 2008.12.12
//*******************************************************************
void WriteData(unsigned char data, unsigned char rs)
{
RS = 0; //Assuming instruction
if (rs & 0x01) RS = 1; //actual data
PORTB = ((PORTB & 0xf0 ) | (data >> 4)); //Write high four bits
EN = 1; //Enable falling edge
EN = 0; //
DelayUs(50); //
PORTB = ((PORTB & 0xf0) | (data & 0x0f)); //Write low four bits
EN = 1; //Enable falling edge
EN = 0; //
DelayUs(50); //
}
//*******************************************************************
//Function name: AddrSite();
//Input parameter: coordinate parameter
//Output parameter: None
//Function description: Set the display address
//Construction date: 2008.12.12
//*******************************************************************
void AddrSite(unsigned char x, unsigned char y)
{
if (y == 0) WriteData((0x80 | x), 0); //First row address
else WriteData((0xc0 | x), 0); //Next row address
}
//*******************************************************************
//Function name: PrintChar(*s, len);
//Input parameters: buffer address, data length
//Output parameters: None
//Function description: string output display
//Construction date: 2008.12.12
//*******************************************************************
void PrintChar(unsigned char *s, unsigned char len)
{
do //Loop sending
{
WriteData(*s, 1); //Display character
s++; //Next character
}
while (--len); //Display a line
}
//*******************************************************************
//Function name: LcdInit();
//Input parameters: None
//Output parameters: None
//Function description: LCD initialization
//Construction date: 2008.12.12
//*******************************************************************
void LcdInit(void)
{
unsigned char i = 3;
RS = 0; //Select command
PORTB = 0x03; //Interface settings
do
{
EN = 1; // Enable falling edge
EN = 0; //
DelayUs(50);
}
while (--i); // Loop three times
PORTB = 0x02; //Four-wire mode
EN = 1; //Enable falling edge
EN = 0; //
DelayUs(50);
WriteData(0x28, 0); //Interface settings
WriteData(0x0c, 0); //Display open
WriteData(0x01, 0); //Display clear
WriteData(0x06, 0); //Address increment
}
//*******************************************************************
//Function name: WriteByte(data);
//Input parameter: data to be written
//Output parameter: None
//Function description: Ds1302 write data
//Build date: 2008.12.12
//*******************************************************************
void WriteByte(unsigned char data)
{
unsigned char i;
TRISA2 = 0; //Output direction
i = 8; //Send eight bits
do
{
DAT = 0; //assumes it is false
if (data & 0x01) DAT = 1; //actually true
CLK = 1; //clock rises
data >>= 1; //shift right one bit
CLK = 0; //clock clears
}
while (--i); //send in a loop
}
//*******************************************************************
//Function name: ReadByte();
//Input parameter: None
//Output parameter: Clock data
//Function description: Ds1302 read data
//Build date: 2008.12.12
//*******************************************************************
unsigned char ReadByte(void)
{
unsigned char i, data;
TRISA2 = 1; //Input direction
i = 8; //Collect eight bits
do
{
data >>= 1; // data right shift
if (DAT) data |= 0x80; //record one bit
CLK = 1; //Clock set
NOP();
CLK = 0; //Clock falls
}
while (--i); //Loop receiving
return data; //return data
}
//***********************************************************************
//Function name: WriteDs1302(addr, code);
//Input parameters: register address, instruction
//Output parameters: None
//Function description: Ds1302 write operation
//Construction date: 2008.12.12
//*******************************************************************
void WriteDs1302(unsigned char addr, unsigned char code)
{
RST = 0; //It can be cleared
CLK = 0; //Clock clears
RST = 1; //Use valid
WriteByte(addr & 0xfe); //Write address
WriteByte(code); //Write data
CLK = 1; //Clock sets
RST = 0; //It can be invalid
}
//***********************************************************************
//Function name: ReadDs1302(addr);
//Input parameter: register address
//Output parameter: function data
//Function description: Ds1302 read operation
//Build date: 2008.12.12
//*******************************************************************
unsigned char ReadDs1302(unsigned addr)
{
unsigned char code;
RST = 0; //Clear
CLK = 0; //Clear clock
RST = 1; //Use valid
WriteByte(addr | 0x01); //Write address
code = ReadByte(); //Read data
CLK = 1; //Set clock
RST = 0; //Fail
return code; //Return data
}
//*******************************************************************
//Function name: TimeCahr();
//Input parameter: None
//Output parameter: None
//Function description: Clock conversion character
//Construction date: 2008.12.12
//*******************************************************************
void TimeCahr(void)
{
unsigned char data;
data = ReadDs1302(0x8c); //Year data
TopChar[2] = 0x30 + (data >> 4); //Year tens digit
TopChar[3] = 0x30 + (data & 0x0f); //Year ones digit
data = ReadDs1302(0x88); //Month data
TopChar[5] = 0x30 + (data >> 4); //Month tens digit
TopChar[6] = 0x30 + (data & 0x0f); //Month units digit
data = ReadDs1302(0x86); //Day data
TopChar[8] = 0x30 + (data >> 4); //Day tens digit
TopChar[9] = 0x30 + (data & 0x0f); //Day units digit
data = ReadDs1302(0x84); //Clock data
BotChar[0] = 0x30 + (data >> 4); //Hour tens digit
BotChar[1] = 0x30 + (data & 0x0f); //Hour units digit
data = ReadDs1302(0x82); //Minutes data
BotChar[3] = 0x30 + (data >> 4); //Minutes Tens digit
BotChar[4] = 0x30 + (data & 0x0f); //Minutes Units
digit data = ReadDs1302(0x80); //Seconds data
BotChar[6] = 0x30 + (data >> 4); //Seconds Tens digit
BotChar[7] = 0x30 + (data & 0x0f); //Seconds Units digit
}
//***********************************************************************
//Function name: Picture();
//Input parameters: None
//Output parameters: None
//Function description: Display picture
//Construction date: 2008.12.12
//*******************************************************************
void Picture(void)
{
TopChar[0] = '2'; //Default year
TopChar[1] = '0';
TopChar[4] = '/'; //Date label
TopChar[7] = '/';
BotChar[2] = ':'; //Clock label
BotChar[5] = ':';
}
//*******************************************************************
//Function name: Ds1302Init();
//Input parameter: None
//Output parameter: None
//Function description: Clock chip setting
//Build date: 2008.12.12
//*******************************************************************
void Ds1302Init(void)
{
unsigned char code;
code = ReadDs1302(0x80); //Read oscillator
if (code & 0x80) //Whether to stop vibration
{
WriteDs1302(0x8e, 0x00); //Allow writing
WriteDs1302(0x8c, 0x08); //Default date
WriteDs1302(0x88, 0x01);
WriteDs1302(0x86, 0x01);
WriteDs1302(0x84, 0x00); //Default time
WriteDs1302(0x82, 0x00);
WriteDs1302(0x80, 0x00);
WriteDs1302(0x8e, 0x80); //Prohibit writing
}
code = ReadDs1302(0x90); //Charging status
if (code != 0xab) //Settings do not match
{
WriteDs1302(0x8e, 0x00); //Allow
writingWriteDs1302(0x90, 0xab); //Charging
settingWriteDs1302(0x8e, 0x80); //Prohibit writing
}
}
//***********************************************************************
//Function name: main();
//Input parameters: None
//Output parameters: None
//Function description: Main program
//Construction date: 2008.12.12
//*******************************************************************
void main(void)
{
unsigned char count, i;
PortInit(); //Pin setting
LcdInit(); //LCD setting
Ds1302Init(); //Clock setting
TimeCahr(); //Time conversion
Picture(); //Display label
while (1)
{
AddrSite(0, 0); //Coordinate setting
PrintChar(TopChar, 16); //Send character
AddrSite(0, 1); //Coordinate setting
PrintChar(BotChar, 16); //Send character
DelayMs(100); //Update cycle
TimeCahr(); //Update time
}
}
Previous article:PIC key LCD+DS1302+AD program (C program)
Next article:PIC16F877A serial port transmission
Recommended ReadingLatest update time:2024-11-16 16:00
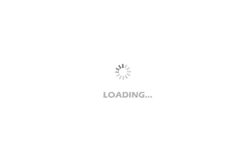
- Popular Resources
- Popular amplifiers
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
-
Principles and Applications of Single Chip Microcomputers and C51 Programming (3rd Edition) (Xie Weicheng, Yang Jiaguo)
-
Teach you to learn 51 single chip microcomputer-C language version (Second Edition) (Song Xuefeng)
-
ATmega16 MCU C language programming classic example (Chen Zhongping)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Basic Op Amp Configuration
- I need help with the schematic diagram or other information of USR-GM3P, or a communication module similar to it.
- [FreeRTOS check-in second stop is open] Stack - the key to task switching, closing time is August 17
- TI-PMLK Boost Experiment Board for TPS55340 and LM5122
- [SC8905 EVM Review] Get the Nanxin Power Board
- 【GD32450I-EVAL】SPI transceiver and touch chip XPT2046 driver and pen interrupt
- Reading Notes on Operational Amplifier Parameter Analysis and LTspice Application Simulation Part 2 - Error Estimation
- 【Beijing】Recruiting embedded (video direction) R&D engineers
- UART interface algorithm transplantation encryption chip debugging skills - communication debugging
- Free sharing video - introduction to common electronic components such as resistors, capacitors and inductors------------The key is free