{
CAN_InitTypeDef CAN_InitStructure;
CAN_FilterInitTypeDef CAN_FilterInitStructure;
CAN_DeInit();
CAN_StructInit(&CAN_InitStructure);
/* CAN cell init */
CAN_InitStructure.CAN_TTCM=DISABLE;//Disable time triggered communication mode
CAN_InitStructure.CAN_ABOM=DISABLE;
CAN_InitStructure.CAN_AWUM=DISABLE;
CAN_InitStructure.CAN_NART=DISABLE;//CAN message is sent only once, regardless of the result of the transmission (success, error or arbitration loss)
CAN_InitStructure.CAN_RFLM=DISABLE;
CAN_InitStructure.CAN_TXFP=DISABLE;
CAN_InitStructure.CAN_Mode=CAN_Mode_Normal;
//CAN_Mode_LoopBack
//CAN_Mode_Normal
CAN_InitStructure.CAN_SJW=CAN_SJW_1tq;
CAN_InitStructure.CAN_BS1=CAN_BS1_5tq;//1--16
CAN_InitStructure.CAN_BS2=CAN_BS2_2tq;//1--8
CAN_InitStructure.CAN_Prescaler=2;
CAN_Init(&CAN_InitStructure);
#ifdef can_id_filter
/* CAN filter init */
CAN_FilterInitStructure.CAN _FilterNumber=0;//Select filter 0
CAN_FilterInitStructure.CAN_FilterMode=CAN_FilterMode_IdMask;//Specify that the filter is set to identifier mask mode
CAN_FilterInitStructure.CAN_FilterScale=CAN_FilterScale_32bit;//Give the filter bit width 32 bits
CAN_FilterInitStructure.CAN_FilterIdHigh=slave_id<<5;//Filter identifier
CAN_FilterInitStructure.CAN_FilterIdLow=0x0000;//
CAN_FilterInitStructure.CAN_FilterMaskIdHigh=0xffff;//Filter mask identifier
CAN_FilterInitStructure.CAN_FilterMaskIdLow=0xfffc;
CAN_FilterInitStructure.CAN_FilterFIFOAssignment=0;//Select FIFO0
CAN_FilterInitStructure.CAN_FilterActivation=ENABLE;//Enable filterCAN_FilterInit
(&CAN_FilterInitStructure);//Enter initialization function
#else
/* CAN filter init */
CAN_FilterInitStructure.CAN_FilterNumber=0;//Select filter 0
CAN_FilterInitStructure.CAN_FilterMode=CAN_FilterMode_IdMask;//Specify that the filter is set to identifier mask modeCAN_FilterInitStructure.CAN_FilterScale
=CAN_FilterScale_32bit;//Give the filter a bit width of 32
bitsCAN_FilterInitStructure.CAN_FilterIdHigh=0x0000;//Filter identifierCAN_FilterInitStructure.CAN_FilterIdLow
=0x0000;//
CAN_FilterInitStructure.CAN_FilterMaskIdHigh=0x0000; //Filter mask identifier
CAN_FilterInitStructure.CAN_FilterMaskIdLow=0x0000;
CAN_ITConfig(CAN_IT_FMP0, ENABLE); //Enable the specified CAN interrupt
}
{
/* Enable CAN reset state */
RCC_APB1PeriphResetCmd(RCC_APB1Periph_CAN, ENABLE);
/* Release CAN from reset state */
RCC_APB1PeriphResetCmd(RCC_APB1Periph_CAN, DISABLE);
}
{
u8 TransmitMailbox=0;
CanTxMsg TxMessage;
if(pTransmitBuf -> LEN > 8)
{
return 1;
}
/* transmit */
TxMessage.StdId=pTransmitBuf ->StdId;//Used to set the standard identifier (0-0x7ff, 11 bits)
TxMessage.ExtId=pTransmitBuf ->ExtId;
TxMessage.RTR= pTransmitBuf ->RTR;//Set the RTR bit to the data frame
TxMessage.IDE= pTransmitBuf ->IDE;//Identifier extension bit, standard frame
TxMessage.DLC= pTransmitBuf ->LEN;//Set the data length
//According to the value of the DLC field, copy the valid data to the transmit data register
memcpy(TxMessage.Data, pTransmitBuf ->BUF,pTransmitBuf ->LEN);
TransmitMailbox = CAN_Transmit(&TxMessage);
// TxMessage.Data[1]=(data & 0xff00)>>8;
return 1;
}
{
//Clear can receive buffer
CanRxMsg RxMessage;
RxMessage.StdId=0x00;
RxMessage.ExtId=0x00;
RxMessage.IDE=0;
RxMessage.DLC=0;
RxMessage.FMI=0;//CAN filter master control register
memset( &RxMessage.Data[0],0,8);
//can bus receive data function
CAN_Receive(CAN_FIFO0, &RxMessage);
//Write the received data to modbus register 1
//modbus_regester[1]=(RxMessage.Data[0]<<8)|(RxMessage.Data[1]);
// if((RxMessage.StdId==slave_id) && (RxMessage.ExtId==0x00) && (RxMessage.IDE==CAN_ID_STD))
{
//Set the can receive flag to 1, indicating that a can message has been received from the card
flag_can_recv=0;
memcpy(can_rx_data,&RxMessage.Data,RxMessage.DLC);
flag_can_recv=1;
}
Filtering on extended data frames:
CAN_FilterInitStructure.CAN_FilterNumber = 0;
CAN_FilterInitStructure.CAN_FilterMode = CAN_FilterMode_IdMask;
CAN_FilterInitStructure.CAN_FilterScale = CAN_FilterScale_32bit;
CAN_FilterInitStructure.CAN_FilterIdHigh = (((u32)CAN_ID<<3)&0xFFFF0000)>>16;
CAN_FilterInitStructure.CAN_FilterIdLow= (((u32)CAN_ID<<3)|CAN_ID_EXT|CAN_RTR_DATA)&0xFFFF;
CAN_FilterInitStructure.CAN_FilterMaskIdHigh = 0xFFFF;
CAN_FilterInitStructure.CAN_FilterMaskIdLow = 0xFFFF;
Filtering on standard data frames:
CAN_FilterInitStructure.CAN_FilterIdHigh= (((u32)CAN_ID0<<21)&0xFFFF0000)>>16;
CAN_FilterInitStructure.CAN_FilterIdLow=(((u32)CAN_ID0<<21)|CAN_ID_STD|CAN_RTR_DATA)&0xFFFF;
CAN_FilterInitStructure.CAN_FilterMaskIdHigh = 0xFFFF;
CAN_FilterInitStructure.CAN_FilterMaskIdLow = 0xFFFF;
If you want to filter standard remote frames, then:
Just need to
CAN_FilterInitStructure.CAN_FilterIdLow=(((u32)CAN_ID0<<21)|CAN_ID_STD|CAN_RTR_DATA)&0xFFFF; 改成:
CAN_FilterInitStructure.CAN_FilterIdLow=(((u32)CAN_ID0<<21)|CAN_ID_STD|CAN_RTR_REMOTE)&0xFFFF;
5. CAN bus frame format
Data frame format:
Remote frame format:
6. Logic level of CAN bus
The physical connection of the CAN bus has two wires: CANH and CANL, which are output in differential form.
(Sometimes there is a ground wire, used as a shielding wire)
The high level of the CAN bus is 3.5v, indicating logic 0
The low level of the CAN bus is 1.5V, indicating logic 1
7. Calculation of CAN bus baud rate
The CAN clock is RCC_APB1PeriphClock. Please note that the CAN clock frequency
CAN baud rate = RCC_APB1PeriphClock/CAN_SJW CAN_BS1 CAN_BS2/CAN_Prescaler;
if the CAN clock is 8M, CAN_SJW = 1, CAN_BS1 = 8, CAN_BS2 = 7, CAN_Prescaler = 2
, then the baud rate is = 8M/(1 8 7)/2=250K
8. Arbitration mechanism of CAN bus
Priority is determined by arbitration:
(1) If at the same time, a standard format message and an extended format message seize the bus at the same time, and their basic IDs are the same, then the node sending the standard format message will succeed in the PK. This is because the extended format is followed by the SRR bit and the IDE bit after the basic ID, and these two bits are recessive. In the standard format, these two bits correspond to the RTR and r1, where RTR can be either a recessive bit or a dominant bit, but r1 must be a dominant bit. According to the arbitration rules, the standard frame will definitely win at this time.
(2) Similarly, if a data frame with the same format and ID competes with a remote frame for bus control at the same time, the data frame will win because RTR explicitly indicates a data frame and implicitly indicates a remote frame.
CAN_InitStructure.CAN_ABOM=DISABLE;
CAN_InitStructure.CAN_AWUM=DISABLE;
CAN_InitStructure.CAN_NART=DISABLE;//CAN message is only sent once, regardless of the result of the transmission (success, error or arbitration loss)
CAN_InitStructure.CAN_RFLM=DISABLE;
CAN_InitStructure.CAN_TXFP=DISABLE;
CAN_InitStructure.CAN_Mode=CAN_Mode_Normal;
//CAN_Mode_LoopBack
//CAN_Mode_Normal
CAN_InitStructure.CAN_SJW=CAN_SJW_1tq;
CAN_InitStructure.CAN_BS1=CAN_BS1_5tq;//1--16
CAN_InitStructure.CAN_BS2=CAN_BS2_2tq;//1--8
CAN_InitStructure.CAN_Prescaler=2;
CAN_Init(&CAN_InitStructure);
CAN_FilterInitStructure.CAN_FilterNumber=0;//Select filter 0
CAN_FilterInitStructure.CAN_FilterMode=CAN_FilterMode_IdMask;//Specify that the filter is set to identifier mask mode
CAN_FilterInitStructure.CAN_FilterScale=CAN_FilterScale_32bit;//Give the filter a bit width of 32 bits
CAN_FilterInitStructure.CAN_FilterIdHigh = (((u32)slave_id<<3)&0xFFFF0000)>>16;
CAN_FilterInitStructure.CAN_FilterIdLow = (((u32)slave_id<<3)|CAN_ID_EXT|CAN_RTR_DATA)&0xFFFF;
CAN_FilterInitStructure.CAN_FilterMaskIdHigh = 0xFFFF;
CAN_FilterInitStructure.CAN_FilterMaskIdLow = 0xFFFF;
(Note: standard frame data frame, standard remote frame and extended remote frame are all filtered)
2. Filter the extended remote frame: (only receive the extended remote frame)
CAN_FilterInitStructure.CAN_FilterIdHigh = (((u32)slave_id<<3)&0xFFFF0000)>>16;
CAN_FilterInitStructure.CAN_FilterIdLow = (((u32)slave_id<<3)|CAN_ID_EXT|CAN_RTR_REMOTE)&0xFFFF;
CAN_FilterInitStructure.CAN_FilterMaskIdHigh = 0xFFFF;
CAN_FilterInit Structure.CAN_FilterMaskIdLow = 0xFFFF;
CAN_FilterInitStructure.CAN_FilterIdHigh = (((u32)slave_id<<21)&0xffff0000)>>16;
CAN_FilterInitStructure.CAN_FilterIdLow = (((u32)slave_id<<21)|CAN_ID_STD|CAN_RTR_REMOTE)&0xffff;
CAN_FilterInitStructure.CAN_FilterMaskIdHigh = 0xFFFF;
CAN_FilterInitStructure.CAN_FilterMaskIdLow = 0xFFFF;
4. Filter standard data frames: (only receive standard data frames)
CAN_FilterInitStructure.CAN_FilterIdHigh = (((u32)slave_id<<21)&0xffff0000)>>16;
CAN_FilterInitStructure.CAN_FilterIdLow = (((u32)slave_id<<21)|CAN_ID_STD|CAN_RTR_DATA)&0xffff;
CAN_FilterInitStructure.CAN_FilterMaskIdHigh = 0xFFFF;
CAN_FilterInitStructure.CAN_FilterMaskIdLow = 0xFFFF;
CAN_FilterInitStructure.CAN_FilterIdHigh = (((u32)slave_id<<3)&0xFFFF0000)>>16;
CAN_FilterInitStructure.CAN_FilterIdLow = (((u32)slave_id<<3)|CAN_ID_EXT)&0xFFFF;
CAN_FilterInitStructure.CAN_FilterMaskIdHigh = 0xFFFF;
CAN_FilterInitStructure.CAN_FilterMaskIdLow = 0xFFFC;
6. Filter standard frames: (standard frames will not be filtered out)
CAN_FilterInitStructure.CAN_FilterIdHigh = (((u32)slave_id<<21)&0xffff0000)>>16;
CAN_FilterInitStructure.CAN_FilterIdLow = (((u32)slave_id<<21)|CAN_ID_STD)&0xffff;
CAN_FilterInitStructure.CAN_FilterMaskIdHigh = 0xFFFF;
CAN_FilterInitStructure.CAN_FilterMaskIdLow = 0xFFFC;
Note: slave_id is the ID number to be filtered.
Previous article:Thermocouple Measurement System Based on Cortex-M3 Microcontroller
Next article:STM32 programming software simulates IIC to read and write 24C02 memory
Recommended ReadingLatest update time:2024-11-16 22:55
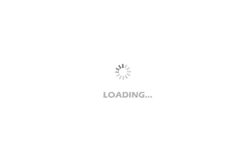
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- The best articles of Bluetooth in the past 10 years
- Program to replace Matlab results with EXCEL formulas
- C2000 ±0.1° Accurate Discrete Resolver Front-End Reference Design
- EEWORLD University Hall----Live Replay: ADI MEMS Sensors Open a New Era of Conditional State Monitoring
- Easily solve design challenges with MSP430 MCUs
- TI Logistics Robot CPU Board
- I would like to ask which one has better performance, thank you for your recommendation
- TI supports Bluetooth technology for high-precision body temperature measurement flexible PCB reference design
- Problems of charging and discharging dual capacitors in peak detectors
- EK140P Linux-4.1.15 Test Manual