#define IMAGE_BUFFER_SIZE 100 //In words After testing, increasing this value did not improve the speed
u32 Image_Buffer1[IMAGE_BUFFER_SIZE]={0};
u32 Image_Buffer2[IMAGE_BUFFER_SIZE]={0};
//OV2640 JPEG mode interface configuration
void OV2640_JpegDcmiInit(void)
{
DCMI_InitTypeDef DCMI_InitStructure;
DMA_InitTypeDef DMA_InitStructure;
NVIC_InitTypeDef NVIC_InitStructure; //NVIC
/*** Configures the DCMI to interface with the OV2640 camera module ***/
/* Enable DCMI clock */
RCC_AHB2PeriphClockCmd(RCC_AHB2Periph_DCMI, ENABLE);
/* DCMI configuration */
DCMI_InitStructure.DCMI_CaptureMode = DCMI_CaptureMode_Continuous; // Single DCMI_CaptureMode_Continuous;
DCMI_InitStructure.DCMI_SynchroMode = DCMI_SynchroMode_Hardware;
DCMI_InitStructure.DCMI_PCKPolarity = DCMI_PCKPolarity_Rising; //
DCMI_InitStructure.DCMI_VSPolarity = DCMI _VSPolarity_Low;
DCMI_InitStructure.DCMI_HSPolarity = DCMI_HSPolarity_Low;
DCMI_InitStructure.DCMI_CaptureRate = DCMI_CaptureRate_All_Frame;
DCMI_InitStructure.DCMI_ExtendedDataMode = DCMI_ExtendedDataMode_8b;
//Initialize DCMI
DCMI_Init(&DCMI_InitStructure);
//Enable J PG
DCMI_JPEGCmd(ENABLE);
/* Configures the DMA2 to transfer Data from DCMI */
/* Enable DMA2 clock */
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_DMA2, ENABLE);
/* DMA2 Stream1 Configuration */
DMA_DeInit(DMA2_Stream1);
DMA_InitStructure.DMA_Channel = DMA_Channel_1; InitStructure.DMA_PeripheralBaseAddr
= DCMI_DR_ADDRESS;
DMA_InitStructure.DMA_Memory0BaseAddr = (uint32_t)&Image_Buffer1;//Transfer to internal array
DMA_InitStructure.DMA_DIR = DMA_DIR_PeripheralToMemory;
DMA_InitStructure.DMA_BufferSize = IMAGE_BUFFER_SIZE; //以字为单位
DMA_InitStructure.DMA_PeripheralInc = DMA_PeripheralInc_Disable;
DMA_InitStructure.DMA_MemoryInc = DMA_MemoryInc_Enable;//使能增长模式
DMA_InitStructure.DMA_PeripheralDataSize = DMA_PeripheralDataSize_Word;
DMA_InitStructure.DMA_MemoryDataSize = DMA_MemoryDataSize_Word;//DMA_MemoryDataSize_HalfWord;
DMA_InitStructure.DMA_Mode = DMA_Mode_Circular;
DMA_InitStructure.DMA_Priority = DMA_Priority_High;
DMA_InitStructure.DMA_FIFOMode = DMA_FIFOMode_Enable;
DMA_InitStructure.DMA_FIFOThreshold = DMA_FIFOThreshold_Full;
DMA_InitStructure.DMA_MemoryBurst = DMA_MemoryBurst_Single;
DMA_InitStructure.DMA_PeripheralBurst = DMA_PeripheralBurst_Single;
/*DMA double buffer mode*///Double buffer mode
DMA_DoubleBufferModeConfig(DMA2_Stream1,(uint32_t)&Image_Buffer2,DMA_Memory_0);//DMA_Memory_0 is transferred first
DMA_DoubleBufferModeCmd(DMA2_Stream1,ENABLE);
DMA_Init(DMA2_Stream1, &DMA_InitStructure); //Initialize DMA
Dma_FreeBuf_Ok = 0;//No data is ready at this time
// DMA_Cmd(DMA2_Stream1,ENABLE);//Start DMA2_Stream1
//Interrupt enable
NVIC_PriorityGroupConfig(NVIC_PriorityGroup_0);
NVIC_InitStructure.NVIC_IRQChannel = DCMI_IRQn;
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 1;
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 1;
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStructure);
DCMI_ITConfig(DCMI_IT_FRAME,ENABLE); //Interrupt enable
NVIC_InitStructure.NVIC_IRQChannel = DMA2_Stream1_IRQn;
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 1;
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 4;
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStructure);
DMA_ITConfig(DMA2_Stream1,DMA_IT_TC,ENABLE);
}
//DMA transfer completed interrupt
void DMA2_Stream1_IRQHandler(void)
{
DMA_InitTypeDef DMA_InitStructure;
if(DMA_GetITStatus(DMA2_Stream1,DMA_IT_TCIF1) != RESET)
{
DMA_ClearITPendingBit(DMA2_Stream1,DMA_IT_TCIF1);
Dma_FreeBuf_Ok = 1;//There is data ready
}
}
//Main loop
while(1)
{
//uip_polling();
if(Dma_FreeBuf_Ok == 1)//DMA has a free buffer
{
usart_sendJPEGdata();
Dma_FreeBuf_Ok = 0;
}
//Serial port transmission
void usart_sendJPEGdata(void)
{
u32 i;
if(DMA_GetCurrentMemoryTarget(DMA2_Stream1) == 1)
{
for(i=0;i
USART_SendData(USART2,Image_Buffer1);
while(USART_GetFlagStatus(USART2,USART_FLAG_TXE)==RESET);
USART_SendData(USART2,Image_Buffer1>>8);
while(USART_GetFlagStatus(USART2,USART_FLAG_TXE)==RESET);
USART_SendData(USART2,I mage_Buffer1>>16);
while(USART_GetFlagStatus(USART2,USART_FLAG_TXE)==RESET);
USART_SendData(USART2,Image_Buffer1>>24);
while(USART_GetFlagStatus(USART2,USART_FLAG_TXE)==RESET);
}
}
else
{
for(i=0;i
USART_SendData(USART2, Image_Buffer2);
while(USART_GetFlagStatus(USART2,USART_FLAG_TXE)==RESET);
USART_SendData(USART2,Image_Buffer2>>8);
while(USART_GetFlagStatus(USART2,USART_FLAG_TXE)==RESET);
USART_SendData(USART2,Image_Buffer2>>16);
while(USART_GetFlagStatus(USART2,USART_FLAG_TXE)==RESET);
USART_SendData(USART2,Image_Buffer2>>24);
while(USART_GetFlagStatus(USART2,USART_FLAG_TXE)==RESET);
}
}
}
Previous article:STM32 uses two ways to receive variable-length data using serial port IDLE interrupt
Next article:Problem of missing bytes when using STM32 serial port to send data in DMA mode in RS485 communication
Recommended ReadingLatest update time:2024-11-16 11:51
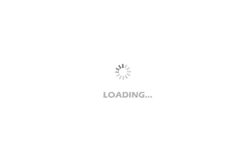
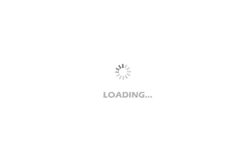
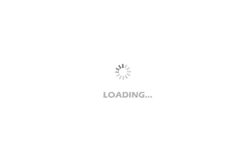
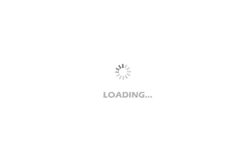
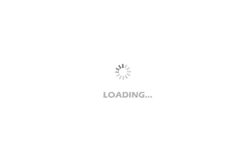
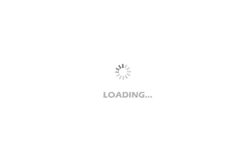
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Algorithm Engineer Recruitment-Beijing-This post is valid for a long time
- There is a problem with MicroPython's official online test function
- What is the difference between PPG and ECG, which can draw electrocardiograms?
- RISC-V IDE MounRive Studio V182 Updates (Part 2): Workspace Switching
- EEWORLD University----[High Precision Laboratory] Motor Drive: Brushless DC Motor Driver
- [National Technology N32G457 Review] RTC + Thermometer
- Are managers and engineers enemies?
- Contactless low-power card reader solution, domestic RC522 focuses on solving the problem of LPCD
- ESP32-S2-Saola-1 Development Board
- Balancing Car and Motor PID Series Video Tutorial