#include
#include
#include
#define NOP() _nop_() /* define a null instruction*/
#define _Nop() _nop_() /* define a null instruction*/
sbit SCL=P2^0; //I2C clock
sbit SDA=P2^1; //I2C data
bit ack; /*acknowledgement flag*/
/***********************************************************************
Start bus functionFunction
prototype: void Start_I2c();
Function: Start I2C bus, that is, send I2C start condition.
********************************************************************/
void Start_I2c()
{
SDA=1; /*Send data signal of start condition*/
_Nop();
SCL=1;
_Nop(); /*Start condition establishment time is greater than 4.7us, delay*/
_Nop();
_Nop();
_Nop();
_Nop();
SDA=0; /*Send start signal*/
_Nop(); /*Start condition locking time is greater than 4μs*/
_Nop();
_Nop();
_Nop();
_Nop();
SCL=0; /*Clamp I2C bus, prepare to send or receive data*/
_Nop();
_Nop();
}
/***********************************************************************
End bus function
Function prototype: void Stop_I2c();
Function: End the I2C bus, that is, send the I2C end condition.
********************************************************************/
void Stop_I2c()
{
SDA=0; /*Send the data signal of the end condition*/
_Nop(); /*Send the clock signal of the end condition*/
SCL=1; /*The establishment time of the end condition is greater than 4μs*/
_Nop();
_Nop()
;
_Nop(); _Nop();
_Nop();
SDA=1; /*Send the end signal of the I2C bus*/
_Nop();
_Nop();
_Nop();
_Nop();
}
/*******************************************************************
Byte data sending function
Function prototype: void SendByte(UCHAR c);
Function: Send data c, which can be an address or data. After sending, wait for a response and operate
on this status bit. (No response or non-response makes ack=0)
Sending data is normal, ack=1; ack=0 means that the controlled device has no response or is damaged.
********************************************************************/
void SendByte(unsigned char c)
{
unsigned char BitCnt;
for(BitCnt=0;BitCnt<8;BitCnt++) /*The length of the data to be transmitted is 8 bits*/
{
if((c<
_Nop();
SCL=1; /*Set the clock line high to notify the controlled device to start receiving data bits*/
_Nop();
_Nop(); /*Ensure that the clock high level period is greater than 4μs*/
_Nop(); _Nop
();
_Nop();
SCL=0;
}
_Nop();
_Nop();
SDA=1; /*Release the data line after 8 bits are sent and prepare to receive the response bit*/
_Nop();
_Nop();
SCL=1;
_Nop();
_Nop();
_Nop();
if(SDA==1)ack=0;
else ack=1; /*Judge whether the response signal is received*/
SCL=0;
_Nop();
_Nop();
}
/*******************************************************************
Byte data receiving function
Function prototype: UCHAR RcvByte();
Function: Used to receive data from the slave device and judge the bus error (no response signal is sent).
After sending, please use the response function to respond to the slave device.
********************************************************************/
unsigned char RcvByte()
{
unsigned char retc;
unsigned char BitCnt;
retc=0;
SDA=1; /*Set the data line to input mode*/
for(BitCnt=0;BitCnt<8;BitCnt++)
{
_Nop();
SCL=0; /*Set the clock line to low and prepare to receive data bits*/
_Nop();
_Nop(); /*Clock low level period is greater than 4.7μs*/
_Nop();
_Nop();
_Nop();
SCL=1; /*Set the clock line to high to make the data on the data line valid*/
_Nop();
_Nop();
retc=retc<<1;
if(SDA==1)retc=retc+1; /*Read the data bit, and put the received data bit into retc*/
_Nop();
_Nop();
}
SCL=0;
_Nop();
_Nop();
return(retc);
}
/************************************************************************
Response sub-function
Function prototype: void Ack_I2c(bit a);
Function: The master controller sends a response signal (can be a response or non-response signal, determined by the bit parameter a)
********************************************************************/
void Ack_I2c(bit a)
{
if(a==0)SDA=0; /*Send a response or non-response signal here*/
else SDA=1;
_Nop();
_Nop
(); _Nop();
SCL=1;
_Nop();
_Nop(); /*Clock low level period is greater than 4μs*/
_Nop();
_Nop();
_Nop();
SCL=0; /*Clear the clock line and clamp the I2C bus to continue receiving*/
_Nop();
_Nop();
}
#include
#include
#define PCF8591 0x90 //PCF8591 address
//else IO
sbit LS138A=P2^2;
sbit LS138B=P2^3;
sbit LS138C=P2^4;
//This table is the font of LED, common cathode digital tube 0-9 -
unsigned char code Disp_Tab[] = {0x3f,0x06,0x5b,0x4f,0x66,0x6d,0x7d,0x07,0x7f,0x6f};
unsigned char AD_CHANNEL;
unsigned long xdata LedOut[8];
unsigned int D[32];
/*******************************************************************
DAC conversion, conversion function
***************************************************************/
bit DACconversion(unsigned char sla,unsigned char c, unsigned char Val)
{
Start_I2c(); //Start the bus
SendByte(sla); //Send device address
if(ack==0)return(0);
SendByte(c); //Send control byte
if(ack==0)return(0);
SendByte(Val); //Send DAC value
if(ack==0)return(0);
Stop_I2c(); //End the bus
return(1);
}
/*******************************************************************
ADC sends byte [command] data function
***************************************************************/
bit ISendByte(unsigned char sla,unsigned char c)
{
Start_I2c(); //Start the bus
SendByte(sla); //Send device address
if(ack==0)return(0);
SendByte(c); //Send data
if(ack==0)return(0);
Stop_I2c(); //End the bus
return(1);
}
/***********************************************************************
ADC read byte data function
***************************************************************/
unsigned char IRcvByte(unsigned char sla)
{ unsigned char c;
Start_I2c(); //Start the bus
SendByte(sla+1); //Send the device address. The reason for adding one to the address is that the last bit of the address word is 0 for writing and 1 for reading
if(ack==0)return(0);
c=RcvByte(); //Read data 0
Ack_I2c(1); //Send non-acknowledge bit
Stop_I2c(); //End bus
return(c);
}
//******************************************************************/
main()
{ char i,j;
while(1)
{/********The following AD-DA processing****************/
switch(AD_CHANNEL)
{
case 0: ISendByte(PCF8591,0x41);
D[0]=IRcvByte(PCF8591)*2; //ADC0 analog-to-digital conversion 1, the reason for multiplying by 2 is to convert the result 00~0xff into 0~510, and divide by 100 to get the actual sampling value
break;
case 1: ISendByte(PCF8591,0x42);
D[1]=IRcvByte(PCF8591)*2; //ADC1 analog-to-digital conversion 2
break;
case 2: ISendByte(PCF8591,0x43);
D[2]=IRcvByte(PCF8591)*2; //ADC2 analog-to-digital conversion 3
break;
case 3: ISendByte(PCF8591,0x40);
D[3]=IRcvByte(PCF8591)*2; //ADC3 analog-to-digital conversion 4
break;
case 4: DACconversion(PCF8591,0x40, D[4]/4); //DAC digital-to-analog conversion
break;
}
// D[4]=400; //Digital--->>Analog output
D[4]=D[3];
if(++AD_CHANNEL>4) AD_CHANNEL=0;
/********The following will send the AD value to the LED digital tube for display*************/
LedOut[0]=Disp_Tab[D[1]%10000/1000];
LedOut[1]=Disp_Tab[D[1]%1000/100];
LedOut[2]=Disp_Tab[D[1]%100/10]|0x80;
LedOut[3]=Disp_Tab[D[1]%10];
LedOut[4]=Disp_Tab[D[0]%10000/1000];
LedOut[5]=Disp_Tab[D[0]%1000/100];
LedOut[6]=Disp_Tab[D[0]%100/10]|0x80;
LedOut[7]=Disp_Tab[D[0]%10];
for( i=0; i<8; i++)
{ P1 = LedOut[i];
switch(i) //Use switch statement to control 138 decoder. You can also use table lookup. Students can try to modify it by themselves
{
case 0:LS138A=0; LS138B=0; LS138C=0; break;
case 1:LS138A=1; LS138B=0; LS138C=0; break;
case 2:LS138A=0; LS138B=1; LS138C=0; break;
case 3:LS138A=1; LS138B=1; LS138C=0; break;
case 4:LS138A=0; LS138B=0; LS138C=1; break;
case 5:LS138A=1; LS138B=0; LS138C=1; break;
case 6:LS138A=0; LS138B=1; LS138C=1; break;
case 7:LS138A=1; LS138B=1; LS138C=1; break;
}
for (j = 0 ; j<90 ;j++) { ;} //Scan interval time
}
P1 = 0;
}
}
Previous article:Comparison between 51 MCU interruption process and main program calling subroutine process
Next article:Implementing Fuzzy Control PID Program in Single Chip Microcomputer
Recommended ReadingLatest update time:2024-11-16 15:46
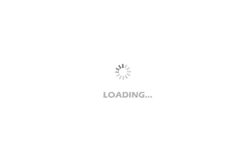
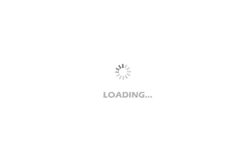
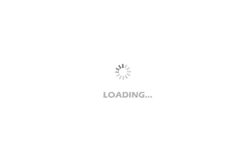
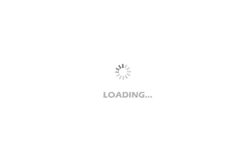
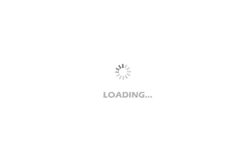
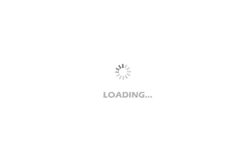
- Popular Resources
- Popular amplifiers
-
Siemens Motion Control Technology and Engineering Applications (Tongxue, edited by Wu Xiaojun)
-
Modern Product Design Guide
-
Modern arc welding power supply and its control
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- The 5 yuan proofing board is back
- About the history, reasons and principles of PFC power factor correction
- A highlight of UCOS compared with ordinary mission structures!!
- Implementation of the sharpening algorithm on DSP
- [NXP Rapid IoT Review] Rapid IoT Studio with Examples
- What does IAR do before main?
- Solution of DC power supply soft start circuit
- 【Complain and Get Rewarded】Complain about the forum news alerts.
- E-book download | "ADI Renewable Energy—Energy Storage Solutions"
- Download and comment to win double gifts | PI invites you to disassemble Xiaomi's latest 2-in-1 power bank with littleshrimp