/* Clock chip driver */
/***********************************************************************/
#include "msp430x41x.h"
#include "delay.h"
#include "iodefine.h"
#include "all_variable.h"
/*****************************************************************/
/* Internal function declaration */
/********************************************************************/
void S35390A_SDA_HIGH(void); //SDA pin outputs high level
void S35390A_SDA_LOW(void); //SDA pin outputs low level
void S35390A_SCL_HIGH(void); //SCK pin outputs high level
void S35390A_SCL_LOW(void); //SDA pin outputs low level
void S35390A_START(void); //Start S35390
void S35390A_STOP(void); //Stop S35390
unsigned char S35390A_GETACK(void); //Get ACK signalvoid
S35390A_SETACK(void); //Output ACK signalvoid
S35390A_SETNCK(void); //Output NACK signalunsigned
char S35390A_RECEIVE_BYTE(void); //Receive a byte of data from S35390void
S35390A_SEND_BYTE(unsigned char senddata); //Send a byte of data to S35390extern
void DELAYeightNOP(void);
extern void DELAYthreeNOP(void);
extern void SetS35390ATime(void);
extern void readS35390ATime(void);
unsigned char S35390A_WRITE(unsigned char opt,unsigned char char count);//write data to S35390A
unsigned char S35390A_READ(unsigned char opt,unsigned char count); //read data from S35390A
unsigned char S35390A_SWAP_BYTE(unsigned char swdata); //byte head and tail swap
unsigned char S35390A_CACULATE_WEEK(unsigned char year,unsigned char month,unsigned char date);//calculate the week by year, month and day
void S35390A_INIT(void);//S35390A initialization
/************************************************************
Function: S35390A_SDA_HIGH(void)
Description: SDA pin outputs high level
Input: none
Output: none
Return: none
**********************************************************/
void S35390A_SDA_HIGH(void)
{
S35390A_SDA_DIR|=S35390A_SDA; // set SDA as output pin
S35390A_SDA_OUT|=S35390A_SDA; //set SDA pin high
_NOP();
return;
}
/**********************************************************
Function: S35390A_SDA_low(void)
Description: SDA脚输出低电平
Input: none
output: none
Return: none
**********************************************************/
void S35390A_SDA_LOW(void)
{
S35390A_SDA_DIR|=S35390A_SDA; //set SDA as output pin
S35390A_SDA_OUT&=~S35390A_SDA; //set SDA pin low
_NOP();
return;
}
/**********************************************************
Function: S35390A_SCL_HIGH(void)
Description: SCK脚输出高电平
Input: none
output: none
Return: none
**********************************************************/
void S35390A_SCL_HIGH(void)
{
S35390A_SCL_DIR|=S35390A_SCL; // set SCK as output pin
S35390A_SCL_OUT|=S35390A_SCL; //set SCK pin high
_NOP();
return;
}
/**********************************************************
Function: S35390A_SCL_low(void)
Description: SDA脚输出低电平
Input: none
output: none
Return: none
**********************************************************/
void S35390A_SCL_LOW(void)
{
S35390A_SCL_DIR|=S35390A_SCL; //set SCK as output pin
S35390A_SCL_OUT&=~S35390A_SCL; //set SCK pin low
_NOP();
return;
}
/**********************************************************
Function: S35390A_RECEIVE_BYTE(void)
Description: 从S35390接收一个字节数据
Input: none
output: none
Return: none
**********************************************************/
unsigned char S35390A_RECEIVE_BYTE(void)
{
unsigned char S35390A_buf=0;
unsigned char w;
S35390A_SDA_DIR &= ~S35390A_SDA; //输入方式
//S35390A_SDA_OUT|=1<
for(w=0; w<8; w++)
{
S35390A_SCL_HIGH();
if((S35390A_SDA_IN & S35390A_SDA))
{
S35390A_buf |= (1<
S35390A_SCL_LOW();
DELAYeightNOP();
}
return(S35390A_buf);
}
/**********************************************************
Function: S35390A_SEND_BYTE(void)
Description: 向S35390发送一个字节数据
Input: none
output: none
Return: none
**********************************************************/
void S35390A_SEND_BYTE(unsigned char senddata)
{
unsigned char w;
for(w=0;w<8;w++)
{
if(senddata&0x80)
{
S35390A_SDA_HIGH();
}
else
{
S35390A_SDA_LOW();
}
S35390A_SCL_HIGH();
DELAYthreeNOP();
senddata<<=1;
S35390A_SCL_LOW();
DELAYeightNOP();
}
}
/**********************************************************
Function: S35390A_START(void)
Description: I2C communication start flag
Input: none
Output: none
Return: none
**********************************************************/
void S35390A_START(void)
{
S35390A_SDA_HIGH(); //High
S35390A_SCL_HIGH(); //High
DELAYthreeNOP();
S35390A_SDA_LOW(); //Low
DELAYthreeNOP();
S35390A_SCL_LOW(); //Low
DELAYeightNOP();
}
/**********************************************************
Function: S35390A_STOP(void)
Description: I2C communication end flag
Input: none
Output: none
Return: none
**********************************************************/
void S35390A_STOP(void)
{
S35390A_SDA_LOW(); //Low
S35390A_SCL_HIGH(); //High
DELAYthreeNOP();
S35390A_SDA_HIGH(); //High
DELAYeightNOP();
//S35390A_SCL_LOW(); //Low
//DELAYeightNOP();
}
/**********************************************************
Function: S35390A_GETACK(void)
Description: 获得ACK信号
Input: none
output: none
Return: temp
**********************************************************/
unsigned char S35390A_GETACK(void)
{
unsigned char w=0;
unsigned char z=100;
_NOP();
_NOP();
S35390A_SCL_LOW();
S35390A_SDA_DIR&=~S35390A_SDA; //SDA input
S35390A_SCL_OUT|=S35390A_SCL;
_NOP();
wait:
w=((S35390A_SDA_IN&S35390A_SDA));
if((w!=0)&&((z--)!=0))
{
goto wait;
}
S35390A_SCL_LOW();
DELAYeightNOP();
return(w);
}
/**********************************************************
Function: S35390A_SETACK(void)
Description: 输出ACK信号
Input: none
output: none
Return: none
**********************************************************/
void S35390A_SETACK(void)
{
S35390A_SCL_LOW();
DELAYeightNOP();
S35390A_SDA_LOW();
DELAYthreeNOP();
S35390A_SCL_HIGH();
DELAYthreeNOP();
S35390A_SCL_LOW();
DELAYeightNOP();
}
/**********************************************************
Function: S35390A_SETNCK(void)
Description: 输出NCK信号
Input: none
output: none
Return: none
**********************************************************/
void S35390A_SETNCK(void)
{
S35390A_SCL_LOW();
DELAYeightNOP();
S35390A_SDA_HIGH();
DELAYthreeNOP();
S35390A_SCL_HIGH();
DELAYthreeNOP();
S35390A_SCL_LOW();
DELAYeightNOP();
}
/**********************************************************
Function: S35390A_WRITE(unsigned char opt,unsigned char adr,unsigned char count)
Description: Write data to S35390A
Input: opt: device command, count: number of bytes to write data
Output: none
Return: 0 or 1
**********************************************************/
unsigned char S35390A_WRITE(unsigned char opt,unsigned char count)
{
unsigned char s_temp=0;
unsigned char ws;
S35390A_START(); //Start the bus
S35390A_SEND_BYTE(opt); //Send device command byte
s_temp=S35390A_GETACK(); //Receive response signal
if((s_temp & S35390A_SDA))
{
S35390A_STOP();
return(0);
}
for(ws=0;ws
s_temp=s35390a[ws];
S35390A_SEND_BYTE(s_temp);
s_temp=S35390A_GETACK(); //Receive response signalif
((s_temp & S35390A_SDA))
{
S35390A_STOP();
return(0);
}
}
S35390A_STOP(); //Stop the busreturn
(1);
}
/**********************************************************
Function: S35390A_READ(unsigned char opt,unsigned char adr,unsigned char count)
Description: Read data from S35390A
Input: none
Output: none
Return: none
**********************************************************/
unsigned char S35390A_READ(unsigned char opt,unsigned char count)
{
unsigned char s_temp=0;
unsigned char ws;
S35390A_START(); //Start the bus
S35390A_SEND_BYTE(opt); //Send device command byte
s_temp=S35390A_GETACK(); //Receive response signal
if((s_temp & S35390A_SDA))
{
S35390A_STOP();
return(0);
}
for(ws=0;ws
s35390a[ws] = S35390A_RECEIVE_BYTE();
if(ws==(count-1))
{
S35390A_SETNCK(); //Do not send a response
}
else
{
S35390A_SETACK(); //Send a response
}
}
S35390A_STOP(); //Stop the busreturn
(1);
}
/**********************************************************
Function: S35390A_SWAP_BYTE(unsigned char swdata)
Description: 字节首尾位交换
Input: none
output: none
Return: none
**********************************************************/
unsigned char S35390A_SWAP_BYTE(unsigned char swdata)
{
unsigned char swtemp = swdata;
swtemp = ((swtemp & 0x55) << 1) | ((swtemp & 0xaa) >> 1); //相邻两位对换
swtemp = ((swtemp & 0x33) << 2) | ((swtemp & 0xcc) >> 2); //
swtemp = ((swtemp & 0x0f) << 4) | ((swtemp & 0xf0) >> 4);
return(swtemp);
}
/**********************************************************
Function: S35390A_CACULATE_WEEK(unsigned char year,unsigned char month,unsigned char data)
Description: 通过年月日计算星期
Input: year,month,date
output: none
Return: week
**********************************************************/
unsigned char S35390A_CACULATE_WEEK(unsigned char year,unsigned char month,unsigned char date)
{
unsigned char wtemp;
if(month==1)
{
year-=1;
month=13;
}
else
{
if(month==2)
{
year-=1;
month=14;
}
}
wtemp=year+year/4+13*((month+1)/5)+date-36;
wtemp%=7;
return(wtemp);
}
/**********************************************************
Function: S35390A_INIT(void)
Description: S35390A initialization
Input: none
Output: none
Return: week
**********************************************************/
void S35390A_INIT(void)
{
S35390A_SDA_DIR |= S35390A_SDA; //Output
S35390A_SCL_DIR |= S35390A_SCL;
S35390A_INT1_DIR &= ~S35390A_INT1; //Input
// S35390A_INT2_DIR &= ~(1<
S35390A_SCL_OUT |= S35390A_SCL;
S35390A_INT1_OUT |=S35390A_INT1;
// S35390A_INT2_OUT |= 1<
S35390A_WRITE(0x62, 0x01); // Clear alarm 1 interrupt enable, make INT1 pin output high level
Delay_Nms(5); // Delay
if(S35390A_READ(0x61,0x01))
{
if((s35390a[0]&0xc0)!=0)
{
s35390a[0]=0xc0;
S35390A_WRITE(0x60,0x01);
}
}
if(S35390A_READ(0x63,0x01))
{
if((s35390a[0]&0x80)!=0)
{
s35390a[0]=0x0c;
S35390A_WRITE(0x60,0x01);
}
}
Delay_Nms(5);
s35390a[0]=0x40; //Set to 24-hour system
S35390A_WRITE(0x60,0x01);
Delay_Nms(5);
s35390a[0]=0x20; //Set alarm 1 interrupt enable
S35390A_WRITE(0x62,0x01); //Set alarm 1 interrupt enable
s35390a[0] =0;
s35390a[1] = S35390A_SWAP_BYTE((0x08<<4)|0x00); //Time;
s35390a[2] =0;
S35390A_WRITE(0x68,0x03); //The interrupt time is 0 o'clock every day
S35390A_INT1_DIR &= ~S35390A_INT1; //Set to input mode
S35390A_INT1_OUT |= S35390A_INT1; //Set to high level
P1IE|=S35390A_INT1; //Interrupt enable
P1IES|=S35390A_INT1; //Interrupt is generated on the falling edge
}
void SetS35390ATime(void)
{
s35390a[0] = S35390A_SWAP_BYTE(twdata[7]); //Year
s35390a[1] = S35390A_SWAP_BYTE(twdata[6]%32); //Month
s35390a[2] = S35390A_SWAP_BYTE(twdata[4]%64); //day
s35390a[3] = 0x00; //week is not used and is 00
s35390a[4] = S35390A_SWAP_BYTE(twdata[3]%64); //hour
s35390a[5] = S35390A_SWAP_BYTE(twdata[2]%128); //minute
s35390a[6] = 0x00; //second is 00
S35390A_WRITE(0x64,0x07); //write time and date
}
void readS35390ATime(void)
{
S35390A_READ(0x65,0x07); //Read time
Delay_Nms(5);
trdata[6]=s35390a[0]; //Year
trdata[5]=s35390a[1]%32; //Month
months=trdata[5];
trdata[3]=s35390a[2]%64; //Day
trdata[2]=s35390a[4]%64; //Hour
trdata[1]=s35390a[5]%128; //Minute
}
Previous article:Comparison of interrupt function writing methods
Next article:430 MCU programming problems and wiring methods
Recommended ReadingLatest update time:2024-11-16 20:45
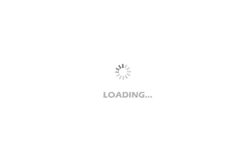
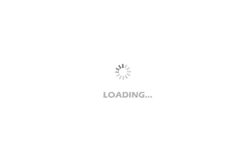
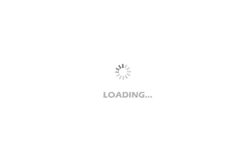
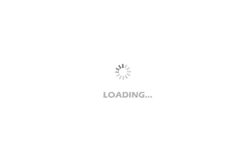
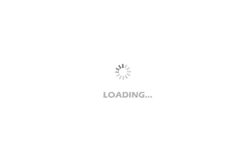
- Popular Resources
- Popular amplifiers
-
Siemens Motion Control Technology and Engineering Applications (Tongxue, edited by Wu Xiaojun)
-
Modern Product Design Guide
-
Modern arc welding power supply and its control
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Where to go during the May Day holiday?
- The 5G era is within reach! Qorvo RF Fusion has been successfully applied to many new smartphone designs
- I want to make a game console recently, but in order to prevent others from copying it, what is the best way to do it?
- The firmware development kit has been upgraded, ONSemiconductor.BDK.1.18.0.pack
- Linux builds RVB2601 development environment
- Wi-Fi 6 and Wi-Fi 6E: The key to the Internet of Things
- Two boards can be cascaded, but not placed on the same layout.
- [ATmega4809 Curiosity Nano Review] How to use RTC
- EEWORLD University Hall----Set up UCD3138 for JTAG communication
- Can the silkscreen on the board be deleted if there is insufficient space? What are the key files required for patch?