//----------------------------Low priority interrupt entry-----------------------------------
2 void InterruptVectorLow (void) //Low priority interrupt vector function
3 {
4 _asm
5 goto InterruptHandlerLow //Embedded assembly instruction
6 _endasm
7 }
8 #pragma code //This is not redundant, it tells the connector to return to the default code segment. If it is not added, the connector will stupidly put the following code immediately following the above code. The LKR file defines the vector area up to address 0x29, so if this line is not added, an error will usually be reported.
9
10 #pragma interruptlow InterruptHandlerLow //The interruptlow keyword is used here to declare that the InterruptHandlerLow function is a low-priority interrupt service function. After using the keyword, the compiler will automatically generate basic context protection for this function, and the return of this function will be returned using RETFIE.
11
12
13 void InterruptHandlerLow (void)
14 {
15 /* The code of the low-priority service function is written here*/
16 }
The high priority interrupt entry address of the PIC18 series is at 0x0008. The following code places a vector function at this entry address. This vector function contains an embedded assembly GOTO instruction, which GOTOs to the high priority interrupt service function InterruptHandlerHigh.
//----------------------------High priority interrupt entry-----------------------------------
1 #pragma code InterruptVectorHigh = 0x08 //Use the #pragma pseudo-instruction to define a segment named InterruptVectorHigh, and put this segment in the code space starting at address 0x08
2 void InterruptVectorHigh (void) //High priority interrupt vector function
3 {
4 _asm
5 goto InterruptHandlerHigh //Embedded assembly instruction
6 _endasm
7 }
8 #pragma code //Return to the default code segment, the reason is the same as above
9 #pragma interrupt InterruptHandlerHigh
10
11 void InterruptHandlerHigh (void)
12 {
13 /* The code of the high priority service function is written here*/
14
15 if (INTCONbits.TMR0IF)
16 {//check for TMR0 overflow
17 INTCONbits.TMR0IF = 0; //clear interrupt flag
18 ;
19 ;
20 }
21 }
For MPLAB C18, the high-priority and low-priority interrupt vector functions and interrupt service functions can only appear once each, and there cannot be multiple interrupt service functions. If multiple interrupts are of high priority, the high-priority interrupt service function performs corresponding processing by judging their respective interrupt flag bits.
CONbits.IPEN = 1; //Enable interrupt priority
INTCONbits.GIEH = 1; //Enable/disable all high priority interrupts
INTCONbits.GIEL = 1; //Enable/disable all low priority interrupts
//RCONbits.IPEN = 0; //Disable interrupt priority
//INTCONbits.GIE = 1; //Enable/disable all interrupt sources
//INTCONbits.PEIE = 1; //Enable/disable all peripheral interrupt sources
When IPEN=1, the interrupt source uses high priority interrupt GIEH = 1; GIEL = 0;
when the interrupt source uses low priority interrupt GIEH = 1; GIEL = 1;
when IPEN=0, all interrupts jump to 08H (use high priority interrupt vector entry)
GIEH = 1; GIEL = 1;
Previous article:Solution to the large array RAM allocation error of PIC microcontroller
Next article:PIC16F877A Watchdog Timer Experiment
Recommended ReadingLatest update time:2024-11-16 15:31
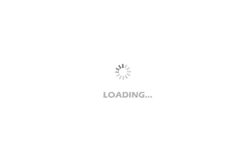
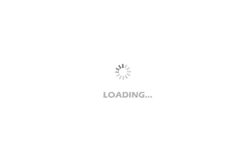
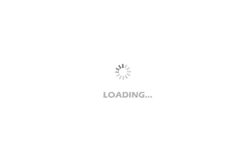
- Popular Resources
- Popular amplifiers
-
Siemens Motion Control Technology and Engineering Applications (Tongxue, edited by Wu Xiaojun)
-
Principles and Applications of Single Chip Microcomputers 3rd Edition (Zhang Yigang)
-
Power Integrated Circuit Technology Theory and Design (Wen Jincai, Chen Keming, Hong Hui, Han Yan)
-
Small Compiler Design Practice (Compiled by Su Mengjin)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- GD32E231C-START Unboxing
- X-NUCLEO-IKS01A3 sensor test based on STM32F401RE development board 5L
- Simulation failed with no results
- Principle of Dialogue Communication
- Voltage jump problem
- 【McQueen Trial】Main functions corresponding to driver pins
- ti dsp (tms320VC5502) + isp1581 usb2.0 high speed data acquisition solution
- Tesla's electric motor
- EEWORLD University ---- The latest version of RTOS training - 15 days to get started with RT-Thread kernel
- [Engineer's Notes] Use 4 "attentions" in PCB wiring to avoid surges!