1. Memory format (word alignment):
The Arm architecture treats memory as a linear combination of bytes starting from address zero. The first stored word (32-bit) data is placed from byte zero to byte three, and the second stored word data is placed from byte four to byte seven, arranged one by one. As a 32-bit microprocessor, the maximum addressing space supported by the Arm architecture is 4GB.
Storage format
1. Big-endian format: high byte at low address, low byte at high address;
2. Little-endian format: high byte at high address, low byte at low address;
Instruction length:
The instruction length of the Arm microprocessor is 32 bits, and can also be 16 bits (in thumb mode). The Arm microprocessor supports three data types: byte (8 bits), halfword (16 bits), and word (32 bits). Among them, words need to be aligned to 4 bytes, and halfwords need to be aligned to 2 bytes.
Note: The so-called instruction length is the length of a complete instruction, not just the length of the three letters mov.
2. The ARM system CPU has two working states
1. ARM state: the processor executes 32-bit word-aligned ARM instructions;
2. Thumb state: The processor executes 16-bit, half-word aligned Thumb instructions;
During the program execution, the two states can be switched accordingly. The change of the processor working state does not affect the processor's working mode and the contents of the corresponding registers.
CPU is powered on in ARM state
3. The ARM system CPU has the following 7 working modes:
1. User mode (Usr): used for normal program execution;
2. Fast interrupt mode (FIQ): used for high-speed data transmission;
3. External interrupt mode (IRQ): used for normal interrupt processing;
4. Management mode (svc): protection mode used by the operating system;
5. Data access termination mode (abt): This mode is entered when data or instruction prefetching is terminated. It can be used for virtual storage and storage protection.
6. System mode (sys): runs privileged operating system tasks;
7. Undefined instruction abort mode (und): This mode is entered when an undefined instruction is executed and can be used to support hardware;
ARM Context-A* architecture has 8 modes
The on-chip registers referred to here are the registers inside the CPU, while the registers that control GPIO belong to peripherals.
Reference: http://infocenter.arm.com/help/index.jsp Corresponding series of documents on the arm official website
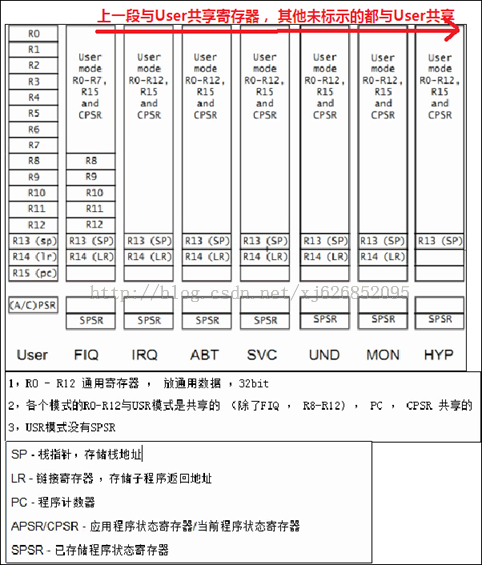
There are two ways to switch the working mode of Arm:
Passive switching: some exceptions or interrupts are generated when the arm is running to automatically switch modes
Active switching: through software changes, that is, the software sets the register to perform arm mode switching, because the arm's working mode can be switched by assigning values to the corresponding registers.
Tips: When the processor runs in user mode, certain protected system resources cannot be accessed.
Except for the user mode, the other six working modes are privileged modes;
The other five modes in the privileged mode except the system mode are called exception modes;
Most programs run in user mode;
Entering privileged mode is to handle interrupts, exceptions, or access protected system resources;
4. Register
ARM has 31 general 32-bit registers and 6 program status registers, which are divided into 7 groups. Some registers are shared by all working modes, and some registers are specific to each working mode.
R13 - Stack pointer register, used to save the stack pointer;
R14 - Program connection register. When the BL subroutine call instruction is executed, R14 gets the backup of R15. When an interrupt or exception occurs, R14 saves the return value of R15.
R15——Program counter;
The fast interrupt mode has 7 backup registers R8-R14, which makes it unnecessary to save any registers when entering the fast interrupt mode to execute a large part of the program;
Other privileged modes have two independent copies of registers, R13 and R14, so that each mode can have its own stack pointer and connection registers;
5. Current Program Status Register (CPSR)
The meaning of each bit in CPSR is as follows:
T bit: 1——CPU is in Thumb state, 0——CPU is in ARM state;
I, F (interrupt disable bit): 1-disable interrupt, 0-enable interrupt;
Working mode bits: These bits can be changed to switch modes;
6. Program Status Save Register (SPSR)
When switching to a privileged mode, the SPSR saves the CPSR value of the previous working mode, so that when returning to the previous working mode, the value of the SPSR can be restored to the CPSR;
7. Mode Switching
When an exception occurs and the CPU enters the corresponding exception mode, the following tasks are automatically completed by the CPU:
1. Save the address of the next instruction to be executed in the previous working mode in R14 of the exception mode;
2. The value of CPSR is transferred to the SPSR of the abnormal mode;
3. Set the working mode of CPSR to the working mode corresponding to the abnormal mode;
4. Set the PC value to the address of this exception mode in the exception vector table, that is, jump to execute the corresponding instruction in the exception vector table;
When returning from an abnormal working mode to the previous working mode, the software needs to complete the following tasks:
1. Subtract an appropriate value (4 or 8) from R14 in the exception mode and assign it to the PC register;
2. Assign the value of the exception mode SPSR to the CPSR;
ARM has seven exceptions. When an exception occurs, the ARM core will automatically execute the instructions in the Vector Table.
ARM's seven exceptions and their offsets in the Vector Table:
abnormal |
model |
Vector table offset |
Reset |
SVC |
+0x00 |
Undefined instruction |
UND |
+0x04 |
Software Interrupt (SWI) |
SVC |
+0x08 |
Prefetch Abort |
ABT |
+0x0c |
Data Termination |
ABT |
+0x10 |
Unassigned |
-- |
+0x14 |
IRQ |
IRQ |
+0x18 |
FIQ |
FIQ |
+0x1c |
The address of the Vector Table of ARM versions below V4 is 0x00000000. The address of the Vector Table of versions above V4 can be selected between 0x00000000 and 0xFFFF0000.
In the ARM architecture, interrupts are a type of exception. Taking interrupts as an example, when an interrupt is received, the ARM core first saves the CPSR and PC registers in the current mode to the SPSR and LR registers in the exception mode, and then sets the PC register value of the target mode to address 0x00000018 (or 0xFFFF0018), and finally switches to the target mode, that is, the IRQ mode. The first instruction executed after switching to the target mode is the instruction at address 0x00000018 (or 0xFFFF0018). This is generally a jump instruction, which is used to jump to the interrupt processing function. Other exception handling methods are similar.
S3C6410 has a 32KB IROM (internel ROM) with a program embedded in it. The beginning of the program is a Vector Table. When booting in IROM mode, IROM is mapped to 0x00000000, so the first instruction executed is the Reset exception jump instruction in the Vector Table (the first instruction is taken at address 0 when the ARM core is powered on). We can use the exception vector table of this IROM to implement interrupt processing under u-boot.
Just after power-on, the interrupt is initialized so that S3C6410 can receive and process interrupts. After an interrupt occurs, the exception jump in 0x00000018 is executed first, and then the interrupt processing function in IROM is executed. This processing function is very simple: the value at 0x0C001FF8 (which falls within the SRAM address range) is assigned to PC. Therefore, we can store the entry address of our own interrupt processing function at 0x0C001FF8, and when an interrupt occurs, our interrupt processing function will be automatically called. The implementation method of other exceptions is similar, except that 0x0C001FF8 needs to be changed to another value. The specific value needs to be analyzed in the code in IROM.
The disassembled code of S3C6410 IROM can be searched on the Internet, or you can extract and disassemble it yourself.
Previous article:ARM processor program status register (CPSR, SPSR) access instructions
Next article:Registers in different processor modes
Recommended ReadingLatest update time:2024-11-15 16:25
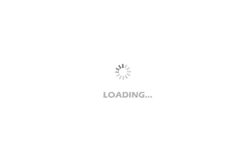
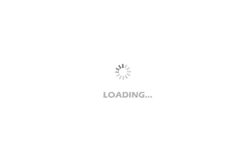
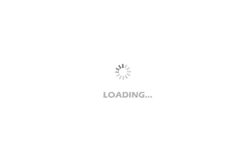
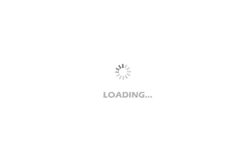
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- Huawei's Strategic Department Director Gai Gang: The cumulative installed base of open source Euler operating system exceeds 10 million sets
- Download from the Internet--ARM Getting Started Notes
- Learn ARM development(22)
- Learn ARM development(21)
- Learn ARM development(20)
- Learn ARM development(19)
- Learn ARM development(14)
- Learn ARM development(15)
- Analysis of the application of several common contact parts in high-voltage connectors of new energy vehicles
- Wiring harness durability test and contact voltage drop test method
- The world's first integrated 5G baseband processor Kirin 990 5G was released today
- [CH579M-R1] + Help: Simulating I2C to read data failed (solved)
- CC2530 RF part use - to achieve point-to-point transmission and reception
- Cut the charging cable TI wireless charging solution to unlock a variety of applications
- Bicycle modification series: headlights
- [Qinheng RISC-V core CH582] I2C lights up the OLED screen
- Area Occupancy Detection Reference Design for mmWave Sensors
- Multi-axis drone airbag
- I bought an old power supply, and this method of cleaning dust is the most effective
- MSP430 MCU Development Record (27)