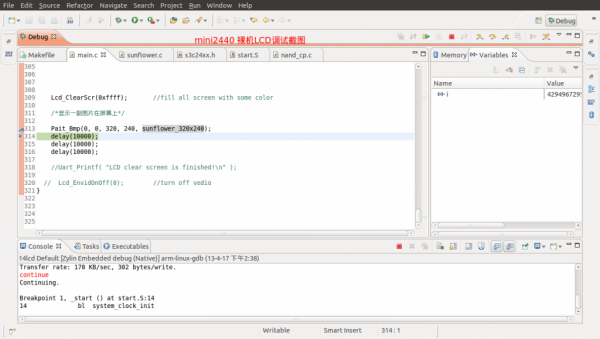
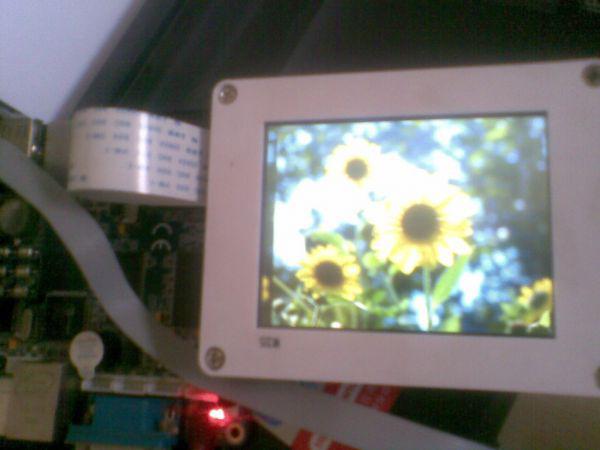
Previous article:Eclipse development and debugging of ARM bare metal programs (Part 7) SD card reading and writing
Next article:Developing and debugging ARM bare metal programs in Eclipse (V) MMU debugging
Recommended ReadingLatest update time:2024-11-16 19:58
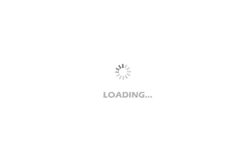
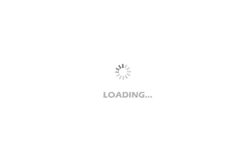
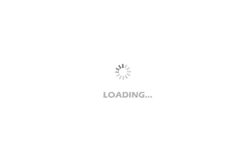
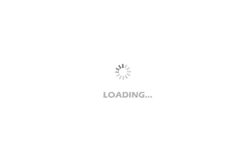
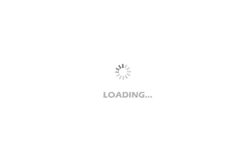
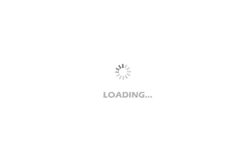
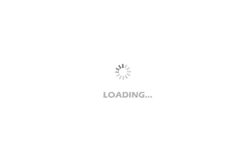
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- [Chuanglong TLA40i-EVM development board] +01. Unboxing (zmj)
- Can the 66AK2L06 SoC enable miniaturization of test and measurement equipment?
- Does ThreadX open source have a big impact on other RTOS?
- Relay switching contacts do not open
- Ten key points about GaN
- QCA9531+QCA9887 Series Embedded Dual-Band AP Module Selection Reference
- Taking the route of "surrounding the city from the countryside"? What do you think about Hongmeng system? ? ?
- [RTT & Renesas high performance CPK-RA6M4] 4. SPI drive OLED evaluation
- How can I quantify the change in resistance value due to the heat generated by the circuit?
- How to calculate the power dissipation of a power supply solution?