The A/D converter supports on-chip sample-and-hold functions and power-down
mode operation.
The touch screen will be in the next article. ADC conversion is relatively simple. Here is a code
- #include "2440addr.h"
- #include "2440lib.h"
- #include "def.h"
- //================================================ =====================
- // Name: ADC_Select(int ch, U32 preScaler)
- //Function: Select the conversion channel and set the converter pre-scaling value
- //Parameters: ch: conversion channel preScaler: pre-scaling value
- //Return value: None
- //================================================ =======================
- void ADC_Select(int ch, U32 preScaler)
- {
- rADCCON=(1<<14)|(preScaler<<6)|(ch<<3);
- }
- //================================================ =======================
- // Name: AD_ENABLE()
- //Function: Start AD conversion by setting enable
- //Parameters: None
- //Return value: converted value
- //================================================ =======================
- int AD_ENABLE(void)
- {
- int i;
- int val=0;
- for(i=0;i<16;i++)
- {
- rADCCON |= 0x1; // Enable ADC conversion
- while(rADCCON & 0x1); // Check if ADC conversion is enabled and cleared
- while(!(rADCCON &0x8000)); //Wait for the conversion to end
- val +=(rADCDAT0 & 0x3ff); //Read the value converted by ADC
- }
- val = val/16; //Calculate ADC conversion value
- return val;
- }
- //================================================ =======================
- // Name: AD_READ()
- //Function: Start AD conversion by reading controller
- //Parameters: None
- //Return value: converted value
- //================================================ =======================
- int AD_READ(void)
- {
- int i;
- int temp,val=0;
- rADCCON |= 0x2; //ADC conversion is started by reading operation
- temp = rADCDAT0 & 0x3ff; //Start ADC
- for(i=0;i<16;i++)
- {
- rADCCON |= 0x1; // Enable ADC conversion
- while(rADCCON & 0x1); // Check if ADC conversion is enabled and cleared
- while(!(rADCCON &0x8000)); //Wait for the conversion to end
- val +=(rADCDAT0 & 0x3ff); //Read the value converted by ADC
- }
- val = val>>4; //Calculate ADC conversion value
- return val;
- }
- void Main(void)
- {
- int temp_val;
- U8 mode;
- SelectFclk(2); //Set the system clock to 400M
- ChangeClockDivider(2, 1); //Set the frequency division to 1:4:8
- CalcBusClk(); //Calculate bus frequency
- rGPHCON &=~((3<<4)|(3<<6));
- rGPHCON |=(2<<4)|(2<<6); //GPH2--TXD[0];GPH3--RXD[0]
- rGPHUP=0x00; //Enable pull-up function
- Uart_Init(0,115200);
- Uart_Select(0);
- while(1)
- {
- ADC_Select(2, 49);
- Uart_Printf("Select ADC Mode: 1.Enable 2.Read\n");
- mode=Uart_Getch();
- Uart_Printf("\n%c\n\n", mode);
- if (mode != '1' && mode != '2')
- {
- Uart_Printf("you select wrong model!\n");
- return;
- }
- switch(mode)
- {
- case '1':
- Uart_Printf("ADC Enable-Convert Mode\n");
- temp_val=AD_ENABLE();
- break;
- case '2':
- Uart_Printf("ADC Read-Convert Mode\n");
- temp_val=AD_READ();
- break;
- }
- Uart_Printf("ADC val = %d\n", temp_val); //Send to the serial port for display
- }
- }
Previous article:S3C2440 LCD Character Display
Next article:S3C2440 drives 4.3-inch TFT screen program
Recommended ReadingLatest update time:2024-11-15 16:21
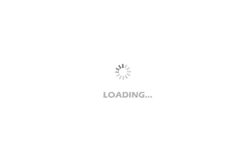
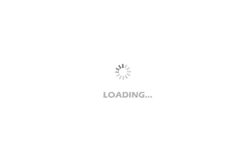
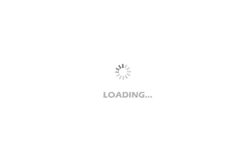
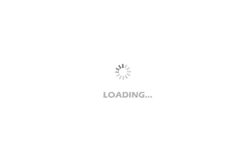
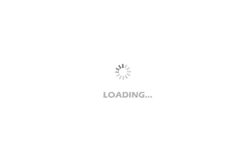
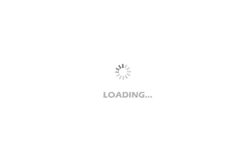
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- Huawei's Strategic Department Director Gai Gang: The cumulative installed base of open source Euler operating system exceeds 10 million sets
- Download from the Internet--ARM Getting Started Notes
- Learn ARM development(22)
- Learn ARM development(21)
- Learn ARM development(20)
- Learn ARM development(19)
- Learn ARM development(14)
- Learn ARM development(15)
- Analysis of the application of several common contact parts in high-voltage connectors of new energy vehicles
- Wiring harness durability test and contact voltage drop test method
- Self-built houses in rural areas VS buying houses in cities
- DSP establishes C environment function c_int00()
- First look at the GD32L233C-START development board
- ESP32-S2 mini Development Board
- Answer questions to win prizes: Azure Sphere IoT solution quiz ranking competition, how long can you dominate the screen?
- Which PCB teaching video is more reliable?
- Application of spectrum analyzer in EMI testing
- Antenna control
- LED backlighting technology
- VKD224B touch chip debugging notes