For details, please refer to the source code explanation. This program example directly changes the source file in Section 4 to the multi-file programming mode.
(1) Hardware platform:
Based on Zhu Zhaoqi 51 single-chip microcomputer learning board. Change the source file in Section 4 to the multi-file programming mode.
(2) Function: Same as the function in Section 4 above, make an LED flash.
(3) Screenshot preview of Keil multi-file programming:
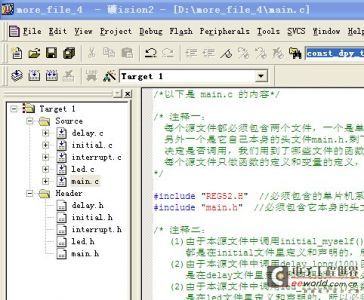

- /*The following is the content of main.h*/
- /* Note 1:
- Each header file is fixed with #ifndef, #define, #endif
- For the template, I suggest using the file name itself plus leading and trailing underscores for the identifier variable _XXX_.
- This flag variable name is used to prevent multiple inclusion errors. Please see Note 2 for detailed explanation.
- Each header file only declares functions and variables, as well as macro definitions for constants and IO ports.
- Function definition and variable definition.
- */
- #ifndef _MAIN_ //The flag variable _MAIN_ is named after its own file name
- #define _MAIN_ //The flag variable _MAIN_ is named after its own file name
- void main(); //This is the function declaration
- #endif
- /* Note 2:
- The above statement
- #ifndef
- #define
- Insert more content...
- #endif
- Similar to treating _MAIN_ as a flag variable
- if(_MAIN_==0) // equivalent to #ifndef _MAIN_
- {
- _MAIN_=1; // equivalent to #define _MAIN_
- Insert more content...
- } //Equivalent to #endif
- The purpose is to assign a flag variable so that the compiler only includes this header file once during compilation to avoid multiple inclusion errors.
- */
- /*------Dividing line--------------------------------------------------*/
- /*The following is the content of main.c*/
- /* Note 1:
- Each source file must contain two files, one is the system header file REG52.H of the microcontroller,
- The other one is its own header file main.h. The rest of the header files depend on the actual situation.
- To decide whether to call, which files' functions, global variables or macro definitions we use, we need to call the corresponding header files.
- Each source file only defines functions and variables, but does not declare functions and variables.
- */
- #include "REG52.H" //Must include the microcontroller system header file
- #include "main.h" //Must include its own header file
- /* Note 2:
- (1) Since the initial_myself() and initial_peripheral() functions are called in this source file, and these two functions
- They are all defined and declared in the initial file, so initial.h must also be included.
- (2) Since the delay_long(100) function is called in this source file, and this function
- It is defined and declared in the delay file, so delay.h must also be included.
- (2) Since the led_flicker() function is called in this source file, and this function
- It is defined and declared in the led file, so led.h must also be included.
- */
- #include "initial.h" //Since the initial_myself() and initial_peripheral() functions are used in this source file, initial.h must also be included
- #include "delay.h" //Because the delay_long(100) function is used in this source file, delay.h must also be included
- #include "led.h" //Since the led_flicker() function is used in this source file, led.h must also be included
- void main() //This is the function definition
- {
- initial_myself();
- delay_long(100);
- initial_peripheral();
- while(1)
- {
- led_flicker();
- }
- }
- /*------Dividing line--------------------------------------------------*/
- /*The following is the content of delay.h*/
- #ifndef _DELAY_ //The flag variable _DELAY_ is named after its own file name
- #define _DELAY_ //The flag variable _DELAY_ is named after its own file name
- void delay_long(unsigned int uiDelaylong); //This is the function declaration. Each function in a source file must be declared in its header file.
- #endif
- /*------Dividing line--------------------------------------------------*/
- /*The following is the content of delay.c*/
- #include "REG52.H" //Must include the microcontroller system header file
- #include "delay.h" //Must include its own header file
- void delay_long(unsigned int uiDelayLong) //This is the function definition
- {
- unsigned int i; //This is the definition of a local variable
- unsigned int j; //This is the definition of a local variable
- for(i=0;i
- {
- for(j=0;j<500;j++)
- {
- ;
- }
- }
- }
- /*------Dividing line--------------------------------------------------*/
- /*The following is the content of initial.h*/
- #ifndef _INITIAL_ //The flag variable _INITIAL_ is named after its own file name
- #define _INITIAL_ //The flag variable _INITIAL_ is named after its own file name
- void initial_myself(); //This is a function declaration. Each function in a source file must be declared in its header file.
- void initial_peripheral(); //This is a function declaration. Each function in a source file must be declared in its header file.
- #endif
- /*------Dividing line--------------------------------------------------*/
- /*The following is the content of initial.c*/
- #include "REG52.H" //Must include the microcontroller system header file
- #include "initial.h" //Must include its own header file
- /* Note 1:
- Since the led_dr statement is used in this source file, and led_dr is a macro definition in the led file, led.h must also be included.
- */
- #include "led.h" //Since the led_dr statement is used in this source file, led.h must also be included
- void initial_myself() //This is the function definition
- {
- TMOD=0x01; //The following can directly use the register keywords TMOD, TH0, TL0, EA, ET0, TR0 because the REG52.H header file is included
- TH0=0xf8;
- TL0=0x2f;
- led_dr=0; //led_dr is defined and declared in the led file
- }
- void initial_peripheral() //This is the function definition
- {
- EA=1;
- ET0=1;
- TR0=1;
- }
- /*------Dividing line--------------------------------------------------*/
- /*The following is the content of interrupt.h*/
- #ifndef _INTERRUPT_ //The flag variable _INTERRUPT_ is named after its own file name
- #define _INTERRUPT_ //The flag variable _INTERRUPT_ is named after its own file name
- void T0_time(); //This is a function declaration. Each function in a source file must be declared in its header file.
- /* Note 1:
- To declare an external global variable, you must add the extern keyword. At the same time, you must not assign an initial value when declaring a global variable, for example:
- extern unsigned int uiTimeCnt=0; This is absolutely wrong.
- */
- extern unsigned int uiTimeCnt; //This is a global variable declaration, and cannot be assigned an initial value
- #endif
- /*------Dividing line--------------------------------------------------*/
- /*The following is the content of interrupt.c*/
- #include "REG52.H" //Must include the microcontroller system header file
- #include "interrupt.h" //Must include its own header file
- unsigned int uiTimeCnt=0; //This is the definition of a global variable, which can be assigned an initial value
- void T0_time() interrupt 1 //This is the function definition
- {
- TF0=0; //The following can directly use the register keywords TF0, TR0, TH0, TL0 because the REG52.H header file is included
- TR0=0;
- if(uiTimeCnt<0xffff)
- {
- uiTimeCnt++;
- }
- TH0=0xf8;
- TL0=0x2f;
- TR0=1;
- }
- /*------Dividing line--------------------------------------------------*/
- /*The following is the content of led.h*/
- #ifndef _LED_ //The flag variable _LED_ is named after its own file name
- #define _LED_ //The flag variable _LED_ is named after its own file name
- #define const_time_level 200 //Macro definitions are placed in header files
- /* Note 1:
- The macro definition of the IO port is also placed in the header file.
- If it is a PIC microcontroller, the following IO port definition is equivalent to the macro definition #define led_dr LATBbits.LATB4 and other statements
- */
- sbit led_dr=P3^5; //If it is a PIC microcontroller, it is equivalent to the macro definition #define led_dr LATBbits.LATB4 and other statements
- void led_flicker(); //This is the function declaration. Each function in a source file must be declared in its header file.
- /* Comment 3:
- When declaring a global variable, you must add the extern keyword. At the same time, you must not assign an initial value when declaring a global variable, for example:
- extern unsigned char ucLedStep=0; This is absolutely wrong.
- */
- extern unsigned char ucLedStep; //This is the declaration of a global variable
- #endif
- /*------Dividing line--------------------------------------------------*/
- /*The following is the content of led.c*/
- #include "REG52.H" //Must include the microcontroller system header file
- #include "led.h" //Must include its own header file
- /* Note 1:
- Since the uiTimeCnt global variable is used in this source file, and uiTimeCnt is declared and defined in the interrupt file,
- So interrupt.h must also be included
- */
- #include "interrupt.h" //Must include its own header file
- unsigned char ucLedStep=0; //This is the definition of a global variable, which can be assigned an initial value
- void led_flicker() //This is the function definition
- {
- switch(ucLedStep)
- {
- case 0:
- if(uiTimeCnt>=const_time_level)
- {
- ET0=0; //The following can directly use the ET0 register keyword because the REG52.H header file is included
- uiTimeCnt=0; //The uiTimeCnt variable is declared and defined in the interrupt file, so interrupt.h must also be included
- ET0=1;
- led_dr=1; //This IO port definition has been defined in the led.h header file
- ucLedStep=1; //Switch to the next step
- }
- break;
- case 1:
- if(uiTimeCnt>=const_time_level)
- {
- ET0=0;
- uiTimeCnt=0;
- ET0=1;
- led_dr=0;
- ucLedStep=0; //Return to the previous step
- }
- break;
- }
- }
- /*------Dividing line--------------------------------------------------*/
Previous article:Section 67: Using external interrupts to simulate serial port data transmission and reception
Next article:Section 69: Using the static keyword can reduce the use of global variables
- Popular Resources
- Popular amplifiers
- Learn ARM development(16)
- Learn ARM development(17)
- Learn ARM development(18)
- Embedded system debugging simulation tool
- A small question that has been bothering me recently has finally been solved~~
- Learn ARM development (1)
- Learn ARM development (2)
- Learn ARM development (4)
- Learn ARM development (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- Pickering Launches New Future-Proof PXIe Single-Slot Controller for High-Performance Test and Measurement Applications
- Apple faces class action lawsuit from 40 million UK iCloud users, faces $27.6 billion in claims
- Apple faces class action lawsuit from 40 million UK iCloud users, faces $27.6 billion in claims
- The US asked TSMC to restrict the export of high-end chips, and the Ministry of Commerce responded
- The US asked TSMC to restrict the export of high-end chips, and the Ministry of Commerce responded
- ASML predicts that its revenue in 2030 will exceed 457 billion yuan! Gross profit margin 56-60%
- Detailed explanation of intelligent car body perception system
- How to solve the problem that the servo drive is not enabled
- Why does the servo drive not power on?
- What point should I connect to when the servo is turned on?
- Microchip Live at 10:00 today: MCU programming is no longer difficult, use MPLAB Code Configurator (MCC) to achieve rapid development
- Application of TMS320DM642 in ATP Technology of Mobile Platform
- [ufun Learning] Unboxing Instructions: What are the preparations before formal learning?
- Raspberry Pi PICO and Firefly Jar
- Today is 520. As boys majoring in science and engineering, how do you surprise your significant other?
- A batch of new Bluetooth speakers are sent for free, and you only need to pay the ten yuan shipping fee
- BearPi HM Nano Introduction
- TI - MCU - MSP430 User Guide
- Class-D amplifier TAS5731M power-on timing analysis
- Award-winning review: Pingtouge RISC-V low-power board - RVB2601