One of the core functions of RTC calibration is void BKP_SetRTCCalibrationValue (uint8_t CalibrationValue) function in library file Stm321f0x_bkp.c. The reference documents related to RTC calibration include AN2604.pdf, AN2821.pdf and AN2821.zip. All three documents can be downloaded from the official website of STM32.
According to the principle described in AN2604.pdf, the calibration value of RTC should be between 0-127. The achievable calibration error corresponds to 0-121ppm. This is equivalent to 0-314s for every 30 days.
A key point to note here is that RTC can only calibrate fast running, not slow running. If the nominal frequency of the watch crystal is 32768Hz, and the possible error range is ±2Hz, the actual frequency will be between 32766Hz-32770Hz. If the internal frequency division coefficient of the RTC is set to 32768, then 32768Hz is a frequency that does not require calibration, and 32768Hz-32770Hz is a frequency that can be calibrated (the maximum calibration capability is about 32772Hz). However, the slow running frequency range of 32766Hz-32768Hz cannot be calibrated. For this reason, in the recommended calibration method, 32766 is used instead of 32768 as the frequency division coefficient. In this way, 32766Hz is a frequency that does not require calibration, and 32766Hz-32770Hz is a frequency range that can be calibrated. The
remaining question is how to measure the error and use it to derive the calibration value. Generally speaking, there are two methods. One is to measure the frequency value of TamperPin and then calculate the ppm error; the other is to actually run for a certain number of days, compare it with the standard clock, first get the number of seconds that run fast every 30 days, and then calculate the ppm error. The
first method is described in detail in AN2604.pdf and AN2821.pdf. AN2821.zip uses timer T2 to automatically measure the frequency value of TamperPin and realizes automatic calibration. Automatic calibration does simplify user operation, but it depends on the accuracy of the 8MHz master clock. Automatic calibration cannot achieve a result with higher accuracy than the 8MHz master clock. Therefore, it is still a foolproof policy to leave a manual calibration interface for users. Even with automatic calibration, manual and automatic functions can be superimposed.
On the other hand, using the first method for calibration requires accurate measurement of the frequency value of TamperPin, such as reaching an accuracy of 511.xxxHz. Ordinary oscilloscopes cannot do this, nor can ordinary frequency meters. Only high-precision frequency meters can do this. Only professionals engaged in measurement have such equipment. As a person engaged in control systems, it is still difficult to use the first method to make a clock with non-measurement accuracy.
Whether it is the first method or the second method, the core is to calculate the ppm error. Let's first look at how the first method calculates the ppm error. Since 32766 is used as the frequency division coefficient, 32766Hz is a reference frequency that does not require calibration. Don't take 32768Hz too seriously. It is nothing now. 32766Hz can be regarded as the new nominal frequency. The frequency of TamperPin should be 32766Hz/64=511.968Hz. This is the reference frequency used repeatedly in the document to calculate the error. According to the example given in the document, if the measured frequency of TamperPin is 511.982Hz, the error is 27.35ppm. The calculation process is (511.982Hz-511.968Hz)/511.968Hz*10^6 = 27.35ppm. The document finally gives the closest calibration value of 28. Note that this is the final calibration value of 28, which is obtained by looking up 27 ppm in the table, not the misunderstanding in some posts that 27.35ppm is approximated to 28ppm.
In fact, the calculation formula for ppm error is: ppm error = deviation/reference value*10 to the sixth power. Based on this, when using the second method, the number of seconds running fast every 30 days is first obtained. The number of seconds that are faster is the deviation, and 30 days is the reference value. So ppm error = number of seconds that are faster every 30 days / (30 days * 24 hours * 3600 seconds) * 10 to the 6th power. This formula can easily explain the "0.65ppm is about 1.7 seconds per month error" mentioned in the document AN2604.pdf. Because: 1.7/(30*24*3600)*10^6 = 0.65ppm. After
calculating the ppm error, you still need to solve the table lookup. Don't pay much attention to the table given in the document. After figuring out where this table comes from, you can use a simple calculation formula instead of looking up the table. AN2604.pdf says that if the calibration value is 1, then when calibrating the RTC, 1 clock pulse is deducted for every 2 to the 20th power clock cycle. This is equivalent to 0.954ppm (1/2^20*10^6 = 0.954). The maximum calibration value is 127, so the maximum slowdown is 121ppm (0.954ppm*127 = 121). So this calibration table is obtained by simple multiplication and division operations. Of course, floating point operations are required to get accurate results.
The following is the RTC calibration program implemented using the second method.
First, two constants are defined. One is PPM_PER_STEP, which is accurate to the precision of 0.9536743ppm that can be represented by floating point numbers. The other is PPM_PER_SEC, which is the ppm error corresponding to one second faster every 30 days, which is accurate to the precision of 0.3858025ppm that can be represented by floating point numbers.
#define PPM_PER_STEP 0.9536743 //10^6/2^20.
#define PPM_PER_SEC 0.3858025 //10^6/(30d*24h*3600s).
Then define the global variable FastSecPer30days. Set through the user menu and pass to the RTC calibration program.
u16 FastSecPer30days = 117; //Menu input. 117 is only used for demonstration.
The implemented calibration function is:
void RTC_Calibration(void)
{
float Deviation = 0.0;
u8 CalibStep = 0;
Deviation = FastSecPer30days * PPM_PER_SEC; //Get ppm error
Deviation /= PPM_PER_STEP; //Get the floating point number of the calibration value
CalibStep = (u8)Deviation; //Get the integer number of the calibration value
if(Deviation >= (CalibStep + 0.5))
CalibStep += 1; //Round
if(CalibStep > 127)
CalibStep = 127; //The calibration value should be between 0 and 127
BKP_SetRTCCalibrationValue(CalibStep); //Call library function
}
//End of function RTC_Calibration
Keywords:STM32 RTC
Reference address:About the calibration method of RTC in STM32
According to the principle described in AN2604.pdf, the calibration value of RTC should be between 0-127. The achievable calibration error corresponds to 0-121ppm. This is equivalent to 0-314s for every 30 days.
A key point to note here is that RTC can only calibrate fast running, not slow running. If the nominal frequency of the watch crystal is 32768Hz, and the possible error range is ±2Hz, the actual frequency will be between 32766Hz-32770Hz. If the internal frequency division coefficient of the RTC is set to 32768, then 32768Hz is a frequency that does not require calibration, and 32768Hz-32770Hz is a frequency that can be calibrated (the maximum calibration capability is about 32772Hz). However, the slow running frequency range of 32766Hz-32768Hz cannot be calibrated. For this reason, in the recommended calibration method, 32766 is used instead of 32768 as the frequency division coefficient. In this way, 32766Hz is a frequency that does not require calibration, and 32766Hz-32770Hz is a frequency range that can be calibrated. The
remaining question is how to measure the error and use it to derive the calibration value. Generally speaking, there are two methods. One is to measure the frequency value of TamperPin and then calculate the ppm error; the other is to actually run for a certain number of days, compare it with the standard clock, first get the number of seconds that run fast every 30 days, and then calculate the ppm error. The
first method is described in detail in AN2604.pdf and AN2821.pdf. AN2821.zip uses timer T2 to automatically measure the frequency value of TamperPin and realizes automatic calibration. Automatic calibration does simplify user operation, but it depends on the accuracy of the 8MHz master clock. Automatic calibration cannot achieve a result with higher accuracy than the 8MHz master clock. Therefore, it is still a foolproof policy to leave a manual calibration interface for users. Even with automatic calibration, manual and automatic functions can be superimposed.
On the other hand, using the first method for calibration requires accurate measurement of the frequency value of TamperPin, such as reaching an accuracy of 511.xxxHz. Ordinary oscilloscopes cannot do this, nor can ordinary frequency meters. Only high-precision frequency meters can do this. Only professionals engaged in measurement have such equipment. As a person engaged in control systems, it is still difficult to use the first method to make a clock with non-measurement accuracy.
Whether it is the first method or the second method, the core is to calculate the ppm error. Let's first look at how the first method calculates the ppm error. Since 32766 is used as the frequency division coefficient, 32766Hz is a reference frequency that does not require calibration. Don't take 32768Hz too seriously. It is nothing now. 32766Hz can be regarded as the new nominal frequency. The frequency of TamperPin should be 32766Hz/64=511.968Hz. This is the reference frequency used repeatedly in the document to calculate the error. According to the example given in the document, if the measured frequency of TamperPin is 511.982Hz, the error is 27.35ppm. The calculation process is (511.982Hz-511.968Hz)/511.968Hz*10^6 = 27.35ppm. The document finally gives the closest calibration value of 28. Note that this is the final calibration value of 28, which is obtained by looking up 27 ppm in the table, not the misunderstanding in some posts that 27.35ppm is approximated to 28ppm.
In fact, the calculation formula for ppm error is: ppm error = deviation/reference value*10 to the sixth power. Based on this, when using the second method, the number of seconds running fast every 30 days is first obtained. The number of seconds that are faster is the deviation, and 30 days is the reference value. So ppm error = number of seconds that are faster every 30 days / (30 days * 24 hours * 3600 seconds) * 10 to the 6th power. This formula can easily explain the "0.65ppm is about 1.7 seconds per month error" mentioned in the document AN2604.pdf. Because: 1.7/(30*24*3600)*10^6 = 0.65ppm. After
calculating the ppm error, you still need to solve the table lookup. Don't pay much attention to the table given in the document. After figuring out where this table comes from, you can use a simple calculation formula instead of looking up the table. AN2604.pdf says that if the calibration value is 1, then when calibrating the RTC, 1 clock pulse is deducted for every 2 to the 20th power clock cycle. This is equivalent to 0.954ppm (1/2^20*10^6 = 0.954). The maximum calibration value is 127, so the maximum slowdown is 121ppm (0.954ppm*127 = 121). So this calibration table is obtained by simple multiplication and division operations. Of course, floating point operations are required to get accurate results.
The following is the RTC calibration program implemented using the second method.
First, two constants are defined. One is PPM_PER_STEP, which is accurate to the precision of 0.9536743ppm that can be represented by floating point numbers. The other is PPM_PER_SEC, which is the ppm error corresponding to one second faster every 30 days, which is accurate to the precision of 0.3858025ppm that can be represented by floating point numbers.
#define PPM_PER_STEP
#define PPM_PER_SEC
Then define the global variable FastSecPer30days. Set through the user menu and pass to the RTC calibration program.
u16 FastSecPer30days = 117; //Menu input. 117 is only used for demonstration.
The implemented calibration function is:
void RTC_Calibration(void)
{
}
//End of function RTC_Calibration
Previous article:STM32Fxxx - Clock
Next article:STM32F101xx and STM32F103xx RTC calibration
Recommended ReadingLatest update time:2024-11-17 08:50
STM32 clock tree notes
1 STM32 has five clock sources: HSI, HSE, LSI, LSE, PLL
1.1 HSI: high-speed internal clock, RC oscillator, frequency is 8MHz, clock accuracy is poor, can be used as a backup clock source (clock safety system CSS).
1.2 HSE: high-speed external clock, can connect external crystal/ceramic resonator (4MHz~16MHz) or ex
[Microcontroller]
STM32 medical rehabilitation robot arm control system
Abstract: Medical rehabilitation robot is a new type of robot that has appeared in recent years. Its main function is to help patients complete various motor function recovery training. To this end, it is proposed to control the operation of the robot arm by controlling the brushless DC motor through the STM32 micro
[Medical Electronics]
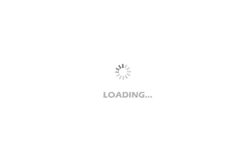
STM32 learning: the three main parts of STM32F4XX
Three major modules: power supply, IO port and clock. 1. First, let's take a look at the power supply. Here is the power supply block diagram From the above we can see that the power supply provides power to three key parts inside. The first is to power the ADC, which needs no further explanation. The second is the ba
[Microcontroller]
STM32 learning Flash write operation & watchdog feeding
I encountered such a problem during debugging these two days. When I was writing data to the flash, the probability of system reset was much higher, and the reset flag was obtained, which was a watchdog reset. However, the interrupt priority and preemption priority used by the timer for feeding the watchdog were the h
[Microcontroller]
Question about the clock source of STM32 common timer (TIM2-7)
【question】 The maximum bus clock of APB1 of STM32F103 is 1/2 of the AHB bus clock, which is 36MHz at most. When using ST's library function (v2.0), the clock frequency of TIM2 (normal timer) is 72MHz. I don't know why? 【problem analysis】 There are up to 8 timers in STM32, of which TIM1 and TIM8 are advanced timers tha
[Microcontroller]
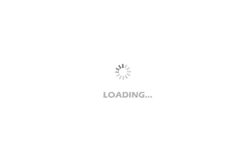
STM32 - Hardware IIC slave communication
Preface: According to the information on the Internet, most netizens said that there is a bug in the hardware IIC of STM32, which is easy to get stuck when reading and writing. I also got stuck when debugging, but I finally debugged it bit by bit and it worked. This article will record some of my experience in debug
[Microcontroller]
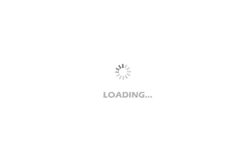
Deep thinking on the serial port of STM32 microcontroller
In fact, when learning to use a single-chip microcomputer, everyone often thinks it is simple and passes it quickly, but there are actually some things that are worth thinking about. When I wrote programs before, I usually sent data, so I just called the rewritten printf() function. But this project uses NRF full-dupl
[Microcontroller]
Research on power quality detection technology based on STM32
0 Introduction
In recent years, with the popularization of modern industrial equipment and civil electrical equipment, power users have higher and higher requirements for power supply quality. In particular, a large number of nonlinear power loads are used in daily life and industrial production, making the
[Microcontroller]
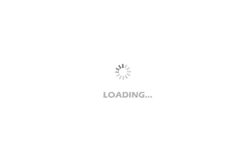
- Popular Resources
- Popular amplifiers
Recommended Content
Latest Microcontroller Articles
He Limin Column
Microcontroller and Embedded Systems Bible
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
MoreSelected Circuit Diagrams
MorePopular Articles
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
MoreDaily News
- New breakthrough! Ultra-fast memory accelerates Intel Xeon 6-core processors
- New breakthrough! Ultra-fast memory accelerates Intel Xeon 6-core processors
- Consolidating vRAN sites onto a single server helps operators reduce total cost of ownership
- Consolidating vRAN sites onto a single server helps operators reduce total cost of ownership
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
Guess you like
- Limited time cash win: Are the new and best-selling products from “e-selection” coming?
- What does it mean that private 5G can solve some enterprise problems that Wi-Fi cannot?
- The most detailed analysis of the working principle of Bluetooth positioning technology
- I would like to ask for advice on the RAM allocation problem of MSP430.
- stm32429 board, RGB display, self-built small font library to display Chinese characters
- Help with 485 enable analysis
- Understanding of time domain and frequency domain
- China's IC design industry
- How to add MCU model in iar5.1? What files need to be added?
- China's RFID frequency band regulations