3.2.1 Introduction to STM32 IO ports
3.2.2 Hardware Design
3.2.3 Software Design
3.2.1 Introduction to STM32 IO ports
The IO port of STM32 has been introduced in detail in the previous section, so we will not go into details here. When the IO port of STM32 is used as input, the status of the IO port is read by reading the content of IDR. With this in mind, we can start writing our code.
In this section, we will use the three buttons on the MiniSTM32 board to control the two LEDs on the board. KEY0 controls DS0. Press it once to turn it on, and press it again to turn it off. KEY1 controls DS1, and the effect is the same as KEY0. KEY_2 (KEY_UP) controls DS0 and DS1 at the same time. Press it once to flip their states.
The hardware circuits required for this experiment have been connected on the MiniSTM32 development board. No settings are required. You can directly write the code. The connection of LED has been introduced in the previous section. The key KEY0 on the MiniSTM32 development board is connected to PA13, KEY1 is connected to PA15, and WK_UP (KEY2) is connected to PA0. As shown in the figure below:

Figure 3.2.2.1 Schematic diagram of the connection between buttons and STM32
It should be noted here that KEY0 and KEY1 are low-level valid, while WK_UP is high-level valid, and it is necessary to confirm whether the connection between the WK_UP button and the DS18B20 has been disconnected. Disconnect it first, otherwise the DS18B20 will interfere with the WK_UP button! And KEY0 and KEY1 are connected to the IO ports related to JTAG, so when writing software, you must first disable the JTAG function before you can use these two IO ports as ordinary IO ports. Here is a special note: We not only disabled JTAG but also SWD in the button initialization function, so when using it, please pay attention that once the button initialization function is executed, you will no longer be able to debug the STM32. The only way to recover is to set it to ISP mode (B0 connected to V3.3, B1 connected to GND), and flash other programs (which will not disable SWD/JTAG) to perform hardware debugging (B0 needs to be reconnected to GND).
3.2.3 Software Design
The code design here is still based on the previous one. Open the TEST project in Section 3.1, and then create a KEY folder under the HARDWARE folder to store the KEY-related codes. As shown in the figure below:

Figure 3.2.3.1 Add a new KEY folder under HARDWARE
Then we open the TEST.Uv2 project in the USER folder, press the button to create a new file, and then save it in the HARDWARE->KEY folder, save it as key.c. Enter the following code in the file:
#include
#include "key.h"
#include "delay.h"
//Mini STM32 development board
//Key input driver code
//Atom on point @ALIENTEK
//2010/5/27
//Button initialization function
//PA0.13.15 is set as input
void KEY_Init(void)
{
RCC->APB2ENR|=1<<2; //Enable PORTA clock
RCC->APB2ENR|=1<<0; //Turn on the auxiliary clock
AFIO->MAPR&=0XF8FFFFFF; //Clear [26:24] of MAPR
AFIO->MAPR|=0X04000000; //Disable JTAG
GPIOA->CRL&=0XFFFFFFF0; //PA0 is set as input
GPIOA->CRL|=0X00000008;
GPIOA->CRH&=0X0F0FFFFF; //PA13, 15 are set as input
GPIOA->CRH|=0X80800000;
GPIOA->ODR|=1<<13; //PA13 is pulled up, PA0 is pulled down by default
GPIOA->ODR|=1<<15; //PA15 pull-up
}
//Key processing function
//Return the key value
//0, no button is pressed
//1, KEY0 is pressed
//2, KEY1 is pressed
//3, KEY2 is pressed
//Note that this function has a response priority, KEY0>KEY1>KEY2!!
u8 KEY_Scan(void)
{
static u8 key_up=1;//button press and release flag
if(key_up&&(KEY0==0||KEY1==0||KEY2==1))
{
delay_ms(10); //debounce
key_up=0;
if(KEY0==0)return 1;
else if(KEY1==0)return 2;
else if(KEY2==1)return 3;
}else if(KEY0==1&&KEY1==1&&KEY2==0)key_up=1;
return 0; // No button pressed
}
The code contains two functions, void KEY_Init(void) and u8 KEY_Scan(void). KEY_Init is used to initialize the IO port for key input. It implements the input settings of PA0, PA13, and PA15. The output configuration here is different from the previous section. There is also a JTAG disable setting.
The disabling of JTAG is configured through the MAPR register of AFIO. To configure the multiplexed IO port, the AFIO clock must be enabled first. The clock enable of AFIO is set in the APB2ENR register. The following sentence enables the clock of AFIO:
RCC->APB2ENR|=1<<0; //Turn on the auxiliary clock
After enabling the AFIO clock, we can set the AFIO related registers. Here we need to turn off JTAG, and the register related to the JTAG setting is the AFIO->MAPR register. The description of each bit of this register is as follows:

Figure 3.2.3.2 Description of each bit of AFIO->MAPR register
Among them, SWJ_CFG[2:0] (AFIO->MAPR[26:24]) is the configuration register bit related to JTAG. The specific settings of these bits and their corresponding descriptions are as follows:

Figure 3.2.3.2 SWJ_CFG bit segment setting relationship
Here we disable all JTAG and SW ports and set AFIO->MAPR [26:24] to 100, as follows:
AFIO->MAPR&=0XF8FFFFFF; //Clear [26:24] of MAPR
AFIO->MAPR|=0X04000000; //Disable JTAG
When setting the [26:24] bits of MAPR, we first clear these bits and then set them. This will not affect the previous setting of AFIO->MAPR. Please pay attention to this point when configuring registers in the future, otherwise, the previous configuration may become invalid! [page]
After disabling JTAG, we configure PA0, PA13, and PA15 as inputs. The settings are similar to the output configuration in 3.1 and will not be introduced here.
The KEY_Scan function is used to scan whether there are any keys pressed on these three IO ports. This KEY_Scan function scans a key. After the key is pressed, it must be released to trigger it a second time, otherwise it will not respond to the key. The advantage of this is that it can prevent multiple triggers from pressing once, but the disadvantage is that it is not suitable when a long press is required. At the same time, it is important to note that the key scan of this function has a priority, the highest priority is KEY0, the second priority is KEY1, and the last is KEY2. This function has a return value. If a key is pressed, it returns a non-zero value. If not or the key is incorrect, it returns 0. Please refer to the KEY_Scan code for specific implementation.
Save the key.c code, and then create a key.h file in the same way and save it in the KEY folder. Enter the following code in key.h:
#ifndef __KEY_H
#define __KEY_H
#include "sys.h"
//Mini STM32 development board
//Key input driver code
//Atom on point @ALIENTEK
//2010/5/27
#define KEY0 PAin(13) //PA13
#define KEY1 PAin(15) //PA15
#define KEY2 PAin(0) //PA0 WK_UP
void KEY_Init(void); //IO initialization
u8 KEY_Scan(void); //Key scanning function
#endif
The key to this code is the three macro definitions:
#define KEY0 PAin(13) //PA13
#define KEY1 PAin(15) //PA15
#define KEY2 PAin(0) //PA0 WK_UP
Here we use bit-banding operation to read 1 bit of an IO port. As with output, we also have another way to implement the function of the above code, as follows:
#define KEY0 (1<<13) //KEY0 PA13
#define KEY1 (1<<15) //KEY1 PA15
#define KEY2 (1<<0) //KEY2 PA0
#define KEY0_GET() ((GPIOA->IDR&(KEY0))?1:0) //Read key 0
#define KEY1_GET() ((GPIOA->IDR&(KEY1))?1:0) //Read key 1
#define KEY2_GET() ((GPIOA->IDR&(KEY2))?1:0) //Read key 2
The first method is simpler, looks clearer, and is more convenient to modify. In subsequent examples, we generally use the first method to implement input port reading. The second method is suitable for porting between different compilers because it does not rely on other codes. You can decide which one to choose based on your own preferences.
Save key.h as well. Next, we add key.c to the HARDWARE group. This time we add a new .c file by double-clicking it. Double-click HARDWARE, find key.c, and add it to HARDWARE, as shown below:

Figure 3.2.3.3 Add key.c to the HARDWARE group
You can see that there is an additional key.c file in the HARDWARE folder. Then use the old method to add the path where the key.h header file is located to the project. Return to the main interface and write the following code in test.c:
#include
#include "sys.h"
#include "usart.h"
#include "delay.h"
#include "led.h"
#include "key.h"
//Mini STM32 development board example code 2
//Key input experiment
//Atom on point @ALIENTEK
//2010.5.27
int main(void)
{
u8 t;
Stm32_Clock_Init(9); //System clock settings
delay_init(72); //delay initialization
LED_Init(); //Initialize the hardware interface connected to the LED
KEY_Init(); //Initialize the hardware interface connected to the key
while(1)
{
t=KEY_Scan(); //Get the key value
if(t)
{
switch(t)
{
case 1:
LED0=!LED0;
break;
case 2:
LED1=!LED1;
break;
case 3:
LED0=!LED0;
LED1=!LED1;
break;
}
}
}
}
Note that you must add the KEY folder to the header file include path, otherwise an error will be reported during compilation. This implementation code is relatively simple, just to implement the functions described in the previous introduction.
Then press to compile the project and the result is as shown below:

Figure 3.2.3.4 Compilation results
It can be seen that there are no errors or warnings. From the compilation information, we can see that the FLASH size occupied by our code is: 1792 bytes (1524+268), and the SRAM size used is: 520 bytes.
Here we explain the meaning of several data in the compilation results:
Code: Indicates the size of the FLASH (FLASH) occupied by the program.
RO-data: represents program-defined constants (FLASH).
RW-data: indicates initialized global variables (SRAM)
ZI-data: indicates uninitialized global variables (SRAM)
With this you can know the size of the flash and sram you are currently using, so it is important to note that the size of the program is not the size of the .hex file.
Next, we will perform software simulation to verify whether there are any errors, and then download it to Mini STM32 to see the actual running results.
Previous article:Alientek SMT32 development board marquee experiment
Next article:ADS_LPC2103 development board SPI 4-bit digital tube test experiment
Recommended ReadingLatest update time:2024-11-15 10:54
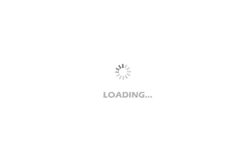
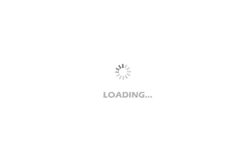
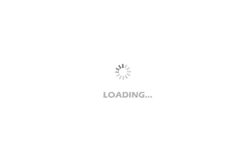
- Popular Resources
- Popular amplifiers
- Learn ARM development(16)
- Learn ARM development(17)
- Learn ARM development(18)
- Embedded system debugging simulation tool
- A small question that has been bothering me recently has finally been solved~~
- Learn ARM development (1)
- Learn ARM development (2)
- Learn ARM development (4)
- Learn ARM development (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- ASML predicts that its revenue in 2030 will exceed 457 billion yuan! Gross profit margin 56-60%
- Detailed explanation of intelligent car body perception system
- How to solve the problem that the servo drive is not enabled
- Why does the servo drive not power on?
- What point should I connect to when the servo is turned on?
- How to turn on the internal enable of Panasonic servo drive?
- What is the rigidity setting of Panasonic servo drive?
- How to change the inertia ratio of Panasonic servo drive
- What is the inertia ratio of the servo motor?
- Is it better for the motor to have a large or small moment of inertia?
- Learn about the future of Wi-Fi 6 / 6E through the Wi-Fi Alliance
- WS2410 high performance and low power consumption 2.4G SOC chip
- Does anyone know what circuit this is and what function it has?
- Two new TI boards
- How to observe the PWM output of DSPF2812 through graph in CCS?
- Improve the clarity of the R329 development board camera
- Analysis of the three most commonly used communication protocols in single-chip microcomputer systems
- MSP-EXP430F5529LP Development Board 001-GPIO
- Chip type and model
- Several modes of Bluetooth devices