1. For the single-chip microcomputer that can set the system clock, the delay program uniformly sets the system clock frequency to the lowest first, and then writes the delay
function according to this lowest clock frequency. The advantages of this are: first, the delay under different system clocks is unified; second, power consumption is reduced.
2. For the single-chip microcomputer system, different external clocks have different corresponding times for the delay program. For ease of use, add the pre-compilation instructions #ifdef/#else/#
endif. In this way, if a system clock is pre-defined, the corresponding delay parameters are selected for compilation, and the main body of the delay program remains unchanged, but there is
a delay parameter in it, and the corresponding parameters are selected according to the pre-definition.
2. For loop processing:
Use for(i=XX,i>0,i--) instead of for(i=0;i
3. Operation of peripherals: You can operate the peripherals as a file. For example, you can treat the LCD as a file. To print strings
or , you can use fprintf() (51's C standard library does not support it, but you can write a file operation library yourself). As long as it is an input and output
device, try to operate it with file operations.
4. Use of standard library functions:
1. Standard input and output library (stdio.h):
When a character string mixed with variable numbers (for example, send the nihao character string, and then send the value of the variable i) is sent to the LCD display or to the serial port, the format output function printf("nihao%d",i) can be used to
send port; but it is not easy to display on the LCD. Usually, a function of converting numbers to strings is written,
and then the original string is sent first, and then the string after digital conversion is sent. This is too troublesome. You can use the sprintf() function in the standard input and output library to complete it. Its
prototype is: sprintf(char *buffer, const char *format, …), *buffer is the buffer to which the string and variable are written. You can
use an array or a pointer. The subsequent format is the same as the printf() function; the corresponding function is the sscanf() function, which reads a string from the buffer
, converts it to the corresponding type, and then assigns it to the specified variable.
For example:
#include
void PrintToLcd(unsigned char *str)
{
...
}
void main()
{
unsigned char *p;
unsigned char i = 50;
sprintf(p,"nihao%d",i);
PrintToLcd(p);
}
2. String library (string.h)
string concatenation (addition) char *strcat(char *dest,const char *stc)
connects src to the end of the dest string and returns a pointer to dest
String comparison int strcmp(char *str1,char *str2)
Return value: less than 0: str1
String copy 1 char *strcpy(char *dest,const char *src)
Result Copy the contents of src into dest, the contents of the two strings are the same, and return a pointer to dest
String copy 2 char *strdup(const char *src)
src: source string to be copied, return value: pointer to the copied string
String reversal char *strrev(char *s);
returns a pointer to the reversed string
3. Type conversion (math.h; stdlib.h)
String to double precision (similar to StrToDouble in C++Builder) double atof(char *str)
String to integer (similar to StrToInt) int atoi(char *str)
String to long integer long atol(char *str)
Floating point number to string char *ecvt(double value,int ndigit,int*dec,int *sign)
char *fcvt(double value,int ndigit,int*dec,int *sign)
Input parameters: value: floating point number to be converted, ndigit: length of the converted string
Output parameters: dec: decimal point position, sign: sign
Returns the converted string pointer
Integer to string char *itoa(int value,char *string,int radix)
Input parameters: value: number to be converted, radix: Converted binary
output parameter: string: The converted string
returns a pointer to string.
Long integer to string conversion char *ltoa(long value,char *string,int radix)
Previous article:MCU C language program (key pressing and debounce)
Next article:STC12C5A60S2 MCU Dual Serial Port Communication
Recommended ReadingLatest update time:2024-11-16 18:05
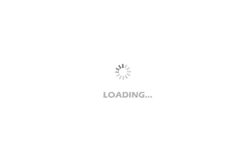
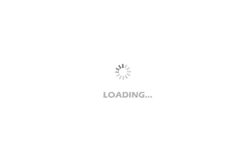
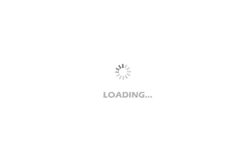
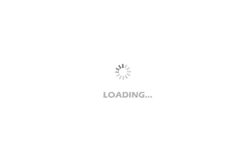
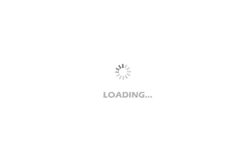
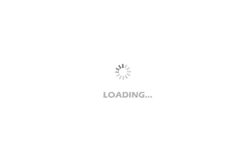
- Popular Resources
- Popular amplifiers
-
Wireless Sensor Network Technology and Applications (Edited by Mou Si, Yin Hong, and Su Xing)
-
Modern Electronic Technology Training Course (Edited by Yao Youfeng)
-
Modern arc welding power supply and its control
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- 5G's new Ultra-high Bands
- Switching Power Supply Study Group
- Is the Tsinghua virtual student singing a real person AI face replacement? The R&D team responds again!
- Sand built on gold - Qorvo announces acquisition of Cavendish Kinetics
- Can multiple ADCs simultaneously acquiring a signal improve system performance?
- Study of TMS320F28335 ADC module
- C8051F340 delay issue
- About Industrial Control System Development
- Everspin MRAM optimizes system energy consumption
- The impact of 5g wireless network on the development of e-sports market