#include "STC12C5620AD.H" #include "EEPROM.H" #include "Channel_Handle.H" /********************** Declare external variables**************************/ /********************** EEPROM storage radio PLL****************************/ void EEPROM_Save_PLL(uchar Index,uint Udata) { uchar Temp_H; uchar Temp_L; //PLL conversion buffer uchar EEPROM_H; uchar EEPROM_L; //EEPROM read cache Temp_H=Udata>>8; Temp_L=Udata%256; switch (Index) { case 1: EEPROM_H=EEPROM_Read_Byte(0x00,0x02); //temporarily store the high bit of PLL of radio station N0.8 EEPROM_L=EEPROM_Read_Byte(0x00,0x03); //temporarily store the high bit of PLL of radio station N0.8 EEPROM_Earse(0x00,0x00); //Erase sector 1 EEPROM_Write_Byte(Temp_H,0x00,0x00); //Write to the high bit of PLL of N0.1 radio station EEPROM_Write_Byte(Temp_L,0x00,0x01); //Write to the low bit of PLL of N0.1 radio station EEPROM_Write_Byte(EEPROM_H,0x00,0x02); //Write to N0.8 radio PLL high bit EEPROM_Write_Byte(EEPROM_L,0x00,0x03); //Write N0.8 radio PLL low bit EEPROM_Earse(0x0e,0x00); //Erase sector 8 EEPROM_Write_Byte(Index,0x0e,0x00); //Write the radio station number break; case 2: EEPROM_H=EEPROM_Read_Byte(0x02,0x02); //temporarily store the high bit of the N0.9 radio station PLL EEPROM_L=EEPROM_Read_Byte(0x02,0x03); //temporarily store the high bit of the N0.9 radio station PLL EEPROM_Earse(0x02,0x00); //Erase sector 2 EEPROM_Write_Byte(Temp_H,0x02,0x00); //Write to the high bit of PLL of radio station N0.2 EEPROM_Write_Byte(Temp_L,0x02,0x01); //Write to the low bit of PLL of radio station N0.2 EEPROM_Write_Byte(EEPROM_H,0x02,0x02); //Write N0.9 radio PLL high bit EEPROM_Write_Byte(EEPROM_L,0x02,0x03); //Write N0.9 radio PLL low bit EEPROM_Earse(0x0e,0x00); //Erase sector 8 EEPROM_Write_Byte(Index,0x0e,0x00); //Write the radio station number break; case 3: EEPROM_H=EEPROM_Read_Byte(0x04,0x02); //temporarily store the high bit of the N0.10 radio station PLL EEPROM_L=EEPROM_Read_Byte(0x04,0x03); //temporarily store the high bit of the N0.10 radio station PLL EEPROM_Earse(0x04,0x00); //Erase sector 3 EEPROM_Write_Byte(Temp_H,0x04,0x00); //Write to the high bit of PLL of N0.3 radio station EEPROM_Write_Byte(Temp_L,0x04,0x01); //Write to the low bit of PLL of radio station N0.3 EEPROM_Write_Byte(EEPROM_H,0x04,0x02); //Write N0.10 radio PLL high bit EEPROM_Write_Byte(EEPROM_L,0x04,0x03); //Write N0.10 radio PLL low bit EEPROM_Earse(0x0e,0x00); //Erase sector 8 EEPROM_Write_Byte(Index,0x0e,0x00); //Write the radio station number break; case 4: EEPROM_H=EEPROM_Read_Byte(0x06,0x02); //temporarily store the high bit of PLL of radio station N0.11 EEPROM_L=EEPROM_Read_Byte(0x06,0x03); //temporarily store the high bit of the N0.11 radio station PLL EEPROM_Earse(0x06,0x00); //Erase the 4th sector EEPROM_Write_Byte(Temp_H,0x06,0x00); //Write to the high bit of PLL of radio station N0.4 EEPROM_Write_Byte(Temp_L,0x06,0x01); //Write to the low bit of PLL of radio station N0.4 EEPROM_Write_Byte(EEPROM_H,0x06,0x02); //Write N0.11 radio PLL high bit EEPROM_Write_Byte(EEPROM_L,0x06,0x03); //Write N0.11 radio PLL low bit EEPROM_Earse(0x0e,0x00); //Erase sector 8 EEPROM_Write_Byte(Index,0x0e,0x00); //Write the radio station number break; case 5: EEPROM_H=EEPROM_Read_Byte(0x08,0x02); //temporarily store the high bit of the N0.12 radio station PLL EEPROM_L=EEPROM_Read_Byte(0x08,0x03); //temporarily store the high bit of the N0.12 radio station PLL EEPROM_Earse(0x08,0x00); //Erase sector 5 EEPROM_Write_Byte(Temp_H,0x08,0x00); //Write to the high bit of PLL of N0.5 radio station EEPROM_Write_Byte(Temp_L,0x08,0x01); //Write to the low bit of PLL of N0.5 radio station EEPROM_Write_Byte(EEPROM_H,0x08,0x02); //Write N0.12 radio PLL high bit EEPROM_Write_Byte(EEPROM_L,0x08,0x03); //Write N0.12 radio PLL low bit EEPROM_Earse(0x0e,0x00); //Erase sector 8 EEPROM_Write_Byte(Index,0x0e,0x00); //Write the radio station number break; case 6: EEPROM_H=EEPROM_Read_Byte(0x0a,0x02); //temporarily store the high bit of the N0.13 radio station PLL EEPROM_L=EEPROM_Read_Byte(0x0a,0x03); //temporarily store the high bit of PLL of radio station N0.13 EEPROM_Earse(0x0a,0x00); //Erase sector 6 EEPROM_Write_Byte(Temp_H,0x0a,0x00); //Write to the high bit of PLL of N0.6 radio station EEPROM_Write_Byte(Temp_L,0x0a,0x01); //Write to the low bit of PLL of N0.6 radio station EEPROM_Write_Byte(EEPROM_H,0x0a,0x02); //Write N0.13 radio PLL high bit EEPROM_Write_Byte(EEPROM_L,0x0a,0x03); //Write N0.13 radio PLL low bit EEPROM_Earse(0x0e,0x00); //Erase sector 8 EEPROM_Write_Byte(Index,0x0e,0x00); //Write the radio station number break; case 7: EEPROM_H=EEPROM_Read_Byte(0x0c,0x02); //temporarily store the high bit of the N0.14 radio station PLL EEPROM_L=EEPROM_Read_Byte(0x0c,0x03); //temporarily store the high bit of the N0.14 radio station PLL EEPROM_Earse(0x0c,0x00); //Erase sector 7 EEPROM_Write_Byte(Temp_H,0x0c,0x00); //Write to the high bit of the N0.7 radio PLL EEPROM_Write_Byte(Temp_L,0x0c,0x01); //Write to the low bit of PLL of N0.7 radio EEPROM_Write_Byte(EEPROM_H,0x0c,0x02); //Write N0.14 radio PLL high bit EEPROM_Write_Byte(EEPROM_L,0x0c,0x03); //Write N0.14 radio PLL low bit EEPROM_Earse(0x0e,0x00); //Erase sector 8 EEPROM_Write_Byte(Index,0x0e,0x00); //Write the radio station number break; case 8: EEPROM_H=EEPROM_Read_Byte(0x00,0x00); //temporarily store the high bit of PLL of radio station N0.1 EEPROM_L=EEPROM_Read_Byte(0x00,0x01); //temporarily store the high bit of PLL of radio station N0.1 EEPROM_Earse(0x00,0x00); //Erase sector 1 EEPROM_Write_Byte(Temp_H,0x00,0x02); //Write to the high bit of the N0.8 radio PLL EEPROM_Write_Byte(Temp_L,0x00,0x03); //Write to the low bit of PLL of N0.8 radio EEPROM_Write_Byte(EEPROM_H,0x00,0x00); //Write N0.1 radio PLL high bit EEPROM_Write_Byte(EEPROM_L,0x00,0x01); //Write N0.1 radio PLL low bit EEPROM_Earse(0x0e,0x00); //Erase sector 8 EEPROM_Write_Byte(Index,0x0e,0x00); //Write the radio station number break; case 9: EEPROM_H=EEPROM_Read_Byte(0x02,0x00); //temporarily store the high bit of PLL of radio station N0.2 EEPROM_L=EEPROM_Read_Byte(0x02,0x01); //temporarily store the high bit of PLL of radio station N0.2 EEPROM_Earse(0x02,0x00); //Erase sector 2 EEPROM_Write_Byte(Temp_H,0x02,0x02); //Write to the high bit of the N0.9 radio station PLL EEPROM_Write_Byte(Temp_L,0x02,0x03); //Write to the low bit of PLL of N0.9 radio station EEPROM_Write_Byte(EEPROM_H,0x02,0x00); //Write to N0.2 radio PLL high bit EEPROM_Write_Byte(EEPROM_L,0x02,0x01); //Write N0.2 radio PLL low bit EEPROM_Earse(0x0e,0x00); //Erase sector 8 EEPROM_Write_Byte(Index,0x0e,0x00); //Write the radio station number break; case 10: EEPROM_H=EEPROM_Read_Byte(0x04,0x00); //temporarily store the high bit of PLL of radio station N0.3 EEPROM_L=EEPROM_Read_Byte(0x04,0x01); //temporarily store the high bit of the N0.3 radio station PLL EEPROM_Earse(0x04,0x00); //Erase sector 3 EEPROM_Write_Byte(Temp_H,0x04,0x02); //Write N0.10 radio PLL high bit EEPROM_Write_Byte(Temp_L,0x04,0x03); //Write N0.10 radio PLL low bit EEPROM_Write_Byte(EEPROM_H,0x04,0x00); //Write N0.3 radio PLL high bit EEPROM_Write_Byte(EEPROM_L,0x04,0x01); //Write N0.3 radio PLL low bit EEPROM_Earse(0x0e,0x00); //Erase sector 8 EEPROM_Write_Byte(Index,0x0e,0x00); //Write the radio station number break; case 11: EEPROM_H=EEPROM_Read_Byte(0x06,0x00); //temporarily store the high bit of PLL of radio station N0.4 EEPROM_L=EEPROM_Read_Byte(0x06,0x01); //temporarily store the high bit of PLL of radio station N0.4 EEPROM_Earse(0x06,0x00); //Erase the 4th sector EEPROM_Write_Byte(Temp_H,0x06,0x02); //Write to the high bit of the N0.11 radio station PLL EEPROM_Write_Byte(Temp_L,0x06,0x03); //Write N0.11 radio PLL low bit EEPROM_Write_Byte(EEPROM_H,0x06,0x00); //Write to N0.4 radio PLL high bit EEPROM_Write_Byte(EEPROM_L,0x06,0x01); //Write N0.4 radio PLL low bit EEPROM_Earse(0x0e,0x00); //Erase sector 8 EEPROM_Write_Byte(Index,0x0e,0x00); //Write the radio station number break; case 12: EEPROM_H=EEPROM_Read_Byte(0x08,0x00); //temporarily store the high bit of PLL of radio station N0.5 EEPROM_L=EEPROM_Read_Byte(0x08,0x01); //temporarily store the high bit of PLL of radio station N0.5 EEPROM_Earse(0x08,0x00); //Erase sector 5 EEPROM_Write_Byte(Temp_H,0x08,0x02); //Write N0.12 radio PLL high bit EEPROM_Write_Byte(Temp_L,0x08,0x03); //Write to the low bit of the N0.12 radio station PLL EEPROM_Write_Byte(EEPROM_H,0x08,0x00); //Write to the high bit of PLL of N0.5 radio station EEPROM_Write_Byte(EEPROM_L,0x08,0x01); //Write the low bit of PLL of N0.5 radio EEPROM_Earse(0x0e,0x00); //Erase sector 8 EEPROM_Write_Byte(Index,0x0e,0x00); //Write the radio station number break; case 13: EEPROM_H=EEPROM_Read_Byte(0x0a,0x00); //temporarily store the high bit of PLL of radio station N0.6 EEPROM_L=EEPROM_Read_Byte(0x0a,0x01); //temporarily store the high bit of PLL of radio station N0.6 EEPROM_Earse(0x0a,0x00); //Erase sector 6 EEPROM_Write_Byte(Temp_H,0x0a,0x02); //Write to the high bit of the N0.13 radio station PLL EEPROM_Write_Byte(Temp_L,0x0a,0x03); //Write to the low bit of PLL of radio station N0.13 EEPROM_Write_Byte(EEPROM_H,0x0a,0x00); //Write N0.6 radio PLL high bit EEPROM_Write_Byte(EEPROM_L,0x0a,0x01); //Write N0.6 radio PLL low bit EEPROM_Earse(0x0e,0x00); //Erase sector 8 EEPROM_Write_Byte(Index,0x0e,0x00); //Write the radio station number break; case 14: EEPROM_H=EEPROM_Read_Byte(0x0c,0x00); //temporarily store the high bit of PLL of radio station N0.7 EEPROM_L=EEPROM_Read_Byte(0x0c,0x01); //temporarily store the high bit of PLL of radio station N0.7 EEPROM_Earse(0x0c,0x00); //Erase sector 7 EEPROM_Write_Byte(Temp_H,0x0c,0x02); //Write N0.14 radio PLL high bit EEPROM_Write_Byte(Temp_L,0x0c,0x03); //Write to the low bit of PLL of radio station N0.14 EEPROM_Write_Byte(EEPROM_H,0x0c,0x00); //Write N0.7 radio PLL high bit EEPROM_Write_Byte(EEPROM_L,0x0c,0x01); //Write N0.7 radio PLL low bit EEPROM_Earse(0x0e,0x00); //Erase sector 8 EEPROM_Write_Byte(Index,0x0e,0x00); //Write the radio station number break; case 15: EEPROM_Earse(0x0e,0x00); //Erase sector 8 EEPROM_Write_Byte(Index,0x0e,0x00); //Write the radio station number EEPROM_Write_Byte(Temp_H,0x0e,0x02); //Write to the high bit of the N0.15 radio station PLL EEPROM_Write_Byte(Temp_L,0x0e,0x03); //Write to the low bit of PLL of N0.15 radio break; default: break; } } /********************** EEPROM reads radio PLL****************************/ uint EEPROM_Get_PLL(uchar Index) { uint PLL_Temp; uchar Temp_H; uchar Temp_L; switch (Index) { case 1: //NO.1 radio station PLL Temp_H=EEPROM_Read_Byte(0x00,0x00); //Get PLL high bit Temp_L=EEPROM_Read_Byte(0x00,0x01); //Get PLL high bit PLL_Temp=Temp_H<<8|Temp_L; break; case 2: //NO.2 radio station PLL Temp_H=EEPROM_Read_Byte(0x02,0x00); //Get PLL high bit Temp_L=EEPROM_Read_Byte(0x02,0x01); //Get PLL high bit PLL_Temp=Temp_H<<8|Temp_L; break; case 3: //NO.3 radio station PLL Temp_H=EEPROM_Read_Byte(0x04,0x00); //Get PLL high bit Temp_L=EEPROM_Read_Byte(0x04,0x01); //Get PLL high bit PLL_Temp=Temp_H<<8|Temp_L; break; case 4: //NO.4 radio station PLL Temp_H=EEPROM_Read_Byte(0x06,0x00); //Get PLL high bit Temp_L=EEPROM_Read_Byte(0x06,0x01); //Get PLL high bit PLL_Temp=Temp_H<<8|Temp_L; break; case 5: //NO.5 radio station PLL Temp_H=EEPROM_Read_Byte(0x08,0x00); //Get PLL high bit Temp_L=EEPROM_Read_Byte(0x08,0x01); //Get PLL high bit PLL_Temp=Temp_H<<8|Temp_L; break; case 6: //NO.6 radio station PLL Temp_H=EEPROM_Read_Byte(0x0a,0x00); //Get PLL high bit Temp_L=EEPROM_Read_Byte(0x0a,0x01); //Get PLL high bit PLL_Temp=Temp_H<<8|Temp_L; case 7: //NO.7 radio station PLL Temp_H=EEPROM_Read_Byte(0x0c,0x00); //Get PLL high bit Temp_L=EEPROM_Read_Byte(0x0c,0x01); //Get PLL high bit PLL_Temp=Temp_H<<8|Temp_L; break; case 8: //NO.8 radio station PLL Temp_H=EEPROM_Read_Byte(0x00,0x02); //Get PLL high bit Temp_L=EEPROM_Read_Byte(0x00,0x03); //Get PLL high bit PLL_Temp=Temp_H<<8|Temp_L; break; case 9: //NO.9 radio station PLL Temp_H=EEPROM_Read_Byte(0x02,0x02); //Get PLL high bit Temp_L=EEPROM_Read_Byte(0x02,0x03); //Get PLL high bit PLL_Temp=Temp_H<<8|Temp_L; break; case 10: //NO.10 radio station PLL Temp_H=EEPROM_Read_Byte(0x04,0x02); //Get PLL high bit Temp_L=EEPROM_Read_Byte(0x04,0x03); //Get PLL high bit PLL_Temp=Temp_H<<8|Temp_L; break; case 11: //NO.11 radio station PLL Temp_H=EEPROM_Read_Byte(0x06,0x02); //Get PLL high bit Temp_L=EEPROM_Read_Byte(0x06,0x03); //Get PLL high bit PLL_Temp=Temp_H<<8|Temp_L; break; case 12: //NO.12 radio station PLL Temp_H=EEPROM_Read_Byte(0x08,0x02); //Get PLL high bit Temp_L=EEPROM_Read_Byte(0x08,0x03); //Get PLL high bit PLL_Temp=Temp_H<<8|Temp_L; break; case 13: //NO.13 radio station PLL Temp_H=EEPROM_Read_Byte(0x0a,0x02); //Get PLL high bit Temp_L=EEPROM_Read_Byte(0x0a,0x03); //Get PLL high bit PLL_Temp=Temp_H<<8|Temp_L; break; case 14: //NO.14 radio station PLL Temp_H=EEPROM_Read_Byte(0x0c,0x02); //Get PLL high bit Temp_L=EEPROM_Read_Byte(0x0c,0x03); //Get PLL high bit PLL_Temp=Temp_H<<8|Temp_L; break; case 15: //NO.15 radio station PLL Temp_H=EEPROM_Read_Byte(0x0e,0x02); //Get PLL high bit Temp_L=EEPROM_Read_Byte(0x0e,0x03); //Get PLL high bit PLL_Temp=Temp_H<<8|Temp_L; break; default: break; } return PLL_Temp; } /********************** EEPROM reads the last serial number*************************/ uchar EEPROM_Get_Index() { uchar Index; Index=EEPROM_Read_Byte(0x0e,0x00); return Index; } /********************** EEPROM writes the last serial number*************************/ void EEPROM_Write_Index(uchar Index) { EEPROM_Earse(0x0e,0x00); // Erase sector 8 first EEPROM_Write_Byte(Index,0x0e,0x00); //Write the radio station number }
Previous article:Some points to note when programming 24C02 single chip microcomputer
Next article:What is RAM? What is ROM? What are its main functions?
Recommended ReadingLatest update time:2024-11-16 13:28
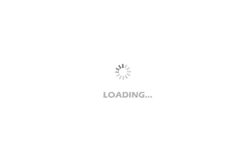
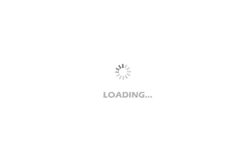
![[51 MCU Quick Start Guide] 4.1: I2C and AT24C02 (EEPROM) cross-page continuous reading and writing](https://6.eewimg.cn/news/statics/images/loading.gif)
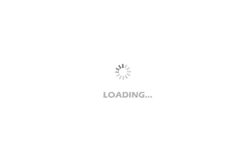
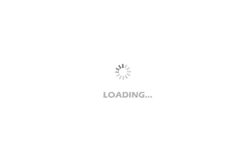
- Popular Resources
- Popular amplifiers
-
Principles and Applications of Single Chip Microcomputers and C51 Programming (3rd Edition) (Xie Weicheng, Yang Jiaguo)
-
FPGA Principle and Structure (Zhao Qian)
-
ADS2008 RF Circuit Design and Simulation Examples (Edited by Xu Xingfu)
-
High-frequency circuit design and production (by Yuichi Ichikawa, Masaru Aoki, and Shengpeng Zhuo)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- MSP430 capture device is simple and practical
- Colorful wallet programmed with CircuitPython
- The storage and downloading of time values sent by customers require sum verification or CRC verification
- EPWM CMPA register configuration problem
- Allegro's latest solution for 48v system
- EEWORLD University Hall----Live Replay: Microchip Security Series 12 - PolarFire? SoC FPGA Secure Boot
- "Praise my country": Let's gather together and talk about the useful domestic microcontrollers
- Ling Embedded Talent Recruitment is in full swing! Don’t run~ It’s you~
- A correct schematic does not necessarily produce a correct PCB design
- "Operational Amplifier Parameter Analysis and LTspice Application Simulation" 5, Chapter 3, 4, 5 Sample Reading