The radio.h file is as follows:
#ifndef _RADIO_H_
#define _RADIO_H_
uint8 radio_read[5]; //define two five-byte arrays to store memory space for writing control registers and reading data
uint8 radio_write[5]={0x00,0x00,0xd0,0x17,0x00};
//uint16 pll; //14-bit PLL value is stored in one word
static unsigned long frequency; //Radio frequency save variable
/*-----------------------------------------------------------------------------------------------------------------------------------
Write register 1
msb lsb
MUTE SM PLL13 PLL12 PLL11 PLL10 PLL09 PLL08
1: Mute 1: Search mode PLL13->PLL8
0: Normal 0: Non-searching Preset or search for radio station frequency value high 6 bits
--------------------------------------------------------------------------------------------------------------------*/
/*--------------------------------------------------------------------------------------------------------------------
Write register 2
msb lsb
PLL7 PLL6 PLL5 PLL4 PLL3 PLL2 PLL1 PLL0
PLL7->PLL0
preset or search for radio station frequency low 8 bits
--------------------------------------------------------------------------------------------------------------------*/
/*--------------------------------------------------------------------------------------------------------------------
Write register 3
msb lsb
SUD SSL1 SSL0 HLSI MS ML MR SWP1
1: Upward search 0 0: No search 1: Local oscillator is higher than the radio station 1: Mono 1: Left channel muted 1: Right channel mute Programmable output port settings
0: Downward search 0 1: ADC is 5 stop 225KHz 0: Stereo 0: Right channel normal 0: Right channel normal 1: SWPOR1 high
1 0: ADC is 7 stop 0: Local oscillator is lower than the radio station 0: SWPOR1 low
1 1: ADC is 10 stop 225KHz
------------------------------------------------------------------------------------------------------------------------------------*/
/*--------------------------------------------------------------------------------------------------------------------
Write register 4
msb lsb
SWP2 STBY BL XTAL SMUTE HHC SNC SI
Programmable output port 1: Standby 1: 76~91MHz 1: Crystal oscillator 32768Hz 1: Software mute on 1: High level cutting on 1: Stereo noise cancellation on
1: SWPOR2 high 0: Normal 0: 87.5~108MHz 0: 13MHz 0: Software mute off 0: High level cutting off 0: Stereo noise cancellation off
0: SWPOR2 low
------------------------------------------------------------------------------------------------------------------------------------*/
/*--------------------------------------------------------------------------------------------------------------------
Write register 5
msb lsb
PLLREF DTC - - - - - -
1: De-emphasis time 75uS
0: De-emphasis time 50uS
--------------------------------------------------------------------------------------------------------------------*/
/*---------------------------------------------------------
Function function: Conversion of radio frequency to PLL
Call form: Convert_PLL();
Parameters:
Return value:
Modification time:
Remarks: HLSI is 1, high local oscillator state, XTAL=1,32.768KHz, PLLREF=0
---------------------------------------------------------*/
void Convert_PLL(void)
{
uint16 pll;
uint8 pll1, pll2; //high and low pll values are temporarily stored in variables
pll=((frequency+225000)*4)/32768; //The unit is Hz
pll1=pll/256;
pll2=pll%256;
radio_write[0]=pll1;
radio_write[1]=pll2;
}
/*---------------------------------------------------------
Function: Initialize RADIO module
Call form: Radio_Init();
Parameters:
Return value:
Modify time:
Remarks: Convert PLL value, write control word
---------------------------------------------------------*/
void Radio_Init(void)
{
//frequency=93400000; //Initial radio frequency 93.4MHz
//radio_write[0]=0x00;
//radio_write[1]=0x00;
//radio_write[2]=0xf0;
radio_write[3]=0x40;
//radio_write[4]=0x00;
//Convert_PLL();
frequency=93400000;
SendString(RADIO_ADDRESS,radio_write,5);
}
/*--------------------------------------------------------------------------------------------------------------------
Read Register 1
MSB LSB
RF BLF PLL13 PLL12 PLL11 PLL10 PLL9 PLL8
1: Radio station found 1: Band reached PLL13->PLL8
or search reached 0: Band not reached Search or preset radio station frequency high 6 bits
0: No radio station found
------------------------------------------------------------------------------------------------------------------------------------*/
/*--------------------------------------------------------------------------------------------------------------------
Read Register 2
MSB LSB
PLL7 PLL6 PLL5 PLL4 PLL3 PLL2 PLL1 PLL0
PLL7->PLL0
Search or preset radio station frequency low 8 bits
--------------------------------------------------------------------------------------------------------------------*/
/*--------------------------------------------------------------------------------------------------------------------
Read Register 3
MSB LSB
STERE0 IF6 IF5 IF4 IF3 IF2 IF1 IF0
1: Stereo IF6->IF0
0: Mono IF counter result
------------------------------------------------------------------------------------------------------------------------------------*/
/*--------------------------------------------------------------------------------------------------------------------
Read register 4
msb lsb
LEV3 LEV2 LEV1 LEV0 CI3 CI2 CI1 CI0
LEV3->LEV0 CI3->CI0
ADC output level Chip identification (all 0)
------------------------------------------------------------------------------------------------------------------------------------*/
/*--------------------------------------------------------------------------------------------------------------------
Read register 5
, all reserved bits
--------------------------------------------------------------------------------------------------------------------*/
void Convert_Frequency()
{
frequency=radio_read[0]&0x3f;
frequency<<=8;
frequency|=radio_read[1];
frequency=frequency*8192-225000;
}
void Radio_Ing(void)
{
static bit k=0;
bit i=0;
uint8 n=0;
uint16 j;
if(!k)
{
k=1;
radio_write[3]=0x17;
}[page]
Convert_PLL();
SendString(RADIO_ADDRESS,radio_write,5);
Display_8x16(0,0,frequency%100000000/10000000);
Display_8x16(8,0,frequency%10000000/1000000);
Display_8x16(16,0,10);
Display_8x16(24,0,frequency%1000000/100000);
/*ReceiveString(RADIO_ADDRESS,radio_read,5);
Display_8x16(0,0,(radio_read[3]&0x80)>>7);
Display_8x16(8,0,(radio_read[3]&0x40)>>6);
Display_8x16(16,0,(radio_read[3]&0x20)>>5);
Display_8x16(24,0,(radio_read[3]&0x10)>>4);*/
for(j=0;j<500;j++)
Display();
while(1)
{
if(ir_value==0x16||ir_value==0x0c||ir_value==0x18||ir_value==0x5e||ir_value==0x08||ir_value==0x1c||ir_value==0x5a||ir_value==0x42||ir_value==0x52||ir_value==0x4a)
{
while(1)
{
if(!i)
{
i=1;
ClearBuf();
//Display_8x16(16,0,10);
}
//if(n==4) frequency*=10;
if(ir_value==0x16)
{ir_value=0xff;Display_8x16(8*n,0,0);if(!n)frequency=0;n++;}
else if(ir_value==0x0c)
{ir_value=0xff;Display_8x16(8*n,0,1);if(!n)frequency=100000000;else if(n==1)frequency+=10000000;else if(n==2) frequency+=1000000;else if(n==3)frequency+=100000;n++;}
else if(ir_value==0x18)
{ir_value=0xff;Display_8x16(8*n,0,2);if(!n)frequency=2*100000000;else if(n==1)frequency+=2*10000000;else if(n==2) frequency+=2*1000000;else if(n==3)frequency+=2*100000;n++;}
else if(ir_value==0x5e)
{ir_value=0xff;Display_8x16(8*n,0,3);if(!n)frequency=3*100000000;else if(n==1)frequency+=3*10000000;else if(n==2) frequency+=3*1000000;else if(n==3)frequency+=3*100000;n++;}
else if(ir_value==0x08)
{ir_value=0xff;Display_8x16(8*n,0,4);if(!n)frequency=4*100000000;else if(n==1)frequency+=4*10000000;else if(n==2) frequency+=4*1000000;else if(n==3)frequency+=4*100000;n++;}
else if(ir_value==0x1c)
{ir_value=0xff;Display_8x16(8*n,0,5);if(!n)frequency=5*100000000;else if(n==1)frequency+=5*10000000;else if(n==2) frequency+=5*1000000;else if(n==3)frequency+=5*100000;n++;}
else if(ir_value==0x5a)
{ir_value=0xff;Display_8x16(8*n,0,6);if(!n)frequency=6*100000000;else if(n==1)frequency+=6*10000000;else if(n==2) frequency+=6*1000000;else if(n==3)frequency+=6*100000;n++;}
else if(ir_value==0x42)
{ir_value=0xff;Display_8x16(8*n,0,7);if(!n)frequency=7*100000000;else if(n==1)frequency+=7*10000000;else if(n==2) frequency+=7*1000000;else if(n==3)frequency+=7*100000;n++;}
else if(ir_value==0x52)
{ir_value=0xff;Display_8x16(8*n,0,8);if(!n)frequency=8*100000000;else if(n==1)frequency+=8*10000000;else if(n==2) frequency+=8*1000000;else if(n==3)frequency+=8*100000;n++;}
else if(ir_value==0x4a)
{ir_value=0xff;Display_8x16(8*n,0,9);if(!n)frequency=9*100000000;else if(n==1)frequency+=9*10000000;else if(n==2) frequency+=9*1000000;else if(n==3)frequency+=9*100000;n++;}
else if(ir_value==0x43)
{ir_value=0xff;Convert_PLL();SendString(RADIO_ADDRESS,radio_write,5);n=0;i=0;break;}
//if(n==2)
//n++;
for(j=0;j<100;j++)
Display();
}
}
else if(ir_value==0x15)
{
ir_value=0xff;//清除
frequency=frequency+100000;
Convert_PLL();
SendString(RADIO_ADDRESS,radio_write,5);
break;
}
else if(ir_value==0x07)
{
ir_value=0xff;
frequency=frequency-100000;
Convert_PLL();
SendString(RADIO_ADDRESS,radio_write,5);
break;
}
else if(ir_value==0x44||ir_value==0x40)//自动搜台
{
while(1)
{
if(ir_value==0x40)//向上自动搜台
{frequency+=100000;if(frequency>108000000)frequency=78000000;}
else {frequency-=100000;if(frequency<78000000)frequency=108000000;}
Convert_PLL();
SendString(RADIO_ADDRESS,radio_write,5);
if(frequency>108000000||frequency<78000000)break;
ReceiveString(RADIO_ADDRESS,radio_read,5);
Convert_Frequency();
if(((radio_read[2]&0x7f<0x3E)&(radio_read[2]&0x7f>0x31)|(radio_read[3]>>4)>5|(radio_read[0]&0x80)))
break;
else if(ir_value==0x43){break;}
Display_8x16(0,0,frequency%100000000/10000000);
Display_8x16(8,0,frequency%10000000/1000000);
Display_8x16(16,0,10);
Display_8x16(24,0,frequency%1000000/100000);
Display();
}
ir_value=0xff;
break;
}
else if(ir_value==0x46)//待机or正常
{
if(radio_write[3]&0x40)
radio_write[3]&=0xbf;
else radio_write[3]|=0x40;
ir_value=0xff;
//i=0;
break;
}
else if(ir_value==0x43||matrix.S==FIRST)
{
ir_value=0xff;
// i=0;
k=0;
//Radio_Init();不待机即可后台运行
matrix.S=FIRST;
break;
}
}
}
void Radio_Key(void)
{
if(!KEY_ENTER)
{
DelayMs(SCAN_DELAY);
if(!KEY_ENTER)
{
while(!KEY_ENTER)
;
matrix.S=FIRST;
}
}
}
/*
void Radio_Read1()
{
ReceiveString(0xc0,radio_read,5);
Convert_Frequency();
if(radio_read[0]&0x80)
temp[6]=duanma[1];
else temp[6]=duanma[0];
if(radio_read[0]&0x40)
{
temp[7]=duanma[1];
}
else temp[7]=duanma[0];
temp[0]=duanma[frequency/100];
temp[1]=duanma[frequency%100/10];
temp[2]=duanma[frequency%10];
}
*/
#endif
Previous article:Program to realize multi-machine communication between single chip microcomputer and PC
Next article:Homemade Logic Analyzer Using STC12C5A60S2
Recommended ReadingLatest update time:2024-11-16 13:24
![[51 microcontroller STC89C52] Timer (interrupt) controls LED](https://6.eewimg.cn/news/statics/images/loading.gif)
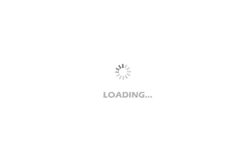
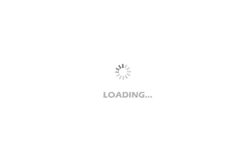
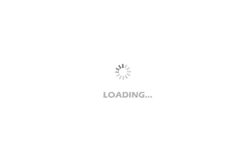
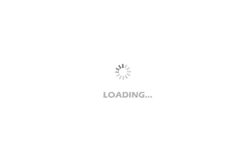
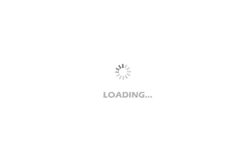
- Popular Resources
- Popular amplifiers
-
Wireless Sensor Network Technology and Applications (Edited by Mou Si, Yin Hong, and Su Xing)
-
Modern Electronic Technology Training Course (Edited by Yao Youfeng)
-
Modern arc welding power supply and its control
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- 【i.MX6ULL】Driver Development 5——Device Tree Principle and Lighting LED
- Calculation of wireless communication distance
- 【TI recommended course】#What is I2C design tool? #
- STM32 low power mode
- I saw a power supply circuit, but I don't know what power supply chip it uses. I want to introduce a similar product. It has a screen print of A9Y93 and an output voltage of 3.3V.
- What is the difference between TMS320C6416 and TMS320C6416T?
- [McQueen Trial] The second post is delayed - Adjusting the basic input and output peripherals on the car
- Thank you for having you + all the people and things that helped me
- Excellent electronic design and product design materials 1
- [GD32L233C-START Review] Part 1: Any surprises after unboxing?