File name: DAC_10k
Function: Use the internal DAC module of msp430f169 to generate a 10kHz sine signal through the transmission of the DMA module . This program takes
100 points
in one cycle
**********************************************************************/
#include
static int Sin_tab[100] = {
1638,1740,1843,1944,2045,2143,2240,2335,2426,2515,2600,2681,2758,2831,
2899,2962,3020,3072 ,3119,3160,3195,3224,3246,3262,3272,3272,3263,3247,
3224,3196,3161,3120,3074,3021,2964,2901,2833,2760,2683,2602,2517,2429,
2337,2243,2146,2047,1947,1845,1743,1640,1537,1435,1333,1233,1134,1037,
943,851,762,677,596,519,446,378,314,256,204,157,116 ,81,52,29,13,3,0,2,
12,28,50, 78,113,154,200,252,310,373,440,513,590,671,756,756,844,936,
1030,1127,1225,1326,1427,1529};
void INIT_XT2();
void main(void)
{
WDTCTL = WDTPW + WDTHOLD; // Stop watchdog
INIT_XT2();
P5SEL=0XFF;
ADC12CTL0 = REFON; // Reference voltage is internal 2.5v
DMA0SA = (int) Sin_tab; / / Source address register
DMA0DA = DAC12_0DAT_; // Destination address register
DMA0SZ = 100; // Number of basic units of transfer
DMACTL0 = DMA0TSEL_5; // DAC12IFG flag of DAC12_0CTL
DMA0CTL = DMADT_4 + DMASRCINCR_3 + DMAEN; // DMADT_4: repeated block Transmission mode Rpt, inc src, word-word
DAC12_0CTL = DAC12LSEL_2 + DAC12IR + DAC12AMP_5 + DAC12IFG + DAC12ENC; // Config
// **force first interrupt**
CCTL1 = OUTMOD_3; // Set/reset
CCR1 = 1; // PWM Duty Cycle
CCR0 = 8-1; // ~1kHz Clock period
TACTL = TASSEL_2 + MC_1; // SMCLK, up-mode
_BIS_SR(LPM0_bits); // Enter LPM0
}
void INIT_XT2()
{
unsigned char i;
BCSCTL1&=~XT2OFF;//Turn on XT2 oscillator
BCSCTL2|=SELM_2+SELS;//SELM_2: Select XT2 as the clock source of MCLK SELS: Select XT2 as the clock source of SMCLK
do
{
IFG1&=~OFIFG;//Clear oscillation error flag
for(i=0;i<100;i++);
_NOP();
}
while((IFG1&OFIFG)!=0);
IFG1&=~OFIFG;
}
==================================================================================
/******************************************************************************
* File: main.c
* Description: MSP430F5438 development board ADC12 conversion experiment
* Set breakpoints to observe the voltage value in voltage
* Change the variable resistor R25 to observe the change of the voltage value in voltage
* Compiler: IAR Embedded Workbench IDE for MSP430 v5.10
*******************************************************************************/
#include
#include "GPIO.h"
#include "BoardConfig.h"
#include "Clock.h"
/**********************************************************************************
*Macro definition area
**************************************************************************/
#define Num_of_Results 8 //Maximum value of data storage
/**************************************************************************
*Function declaration area
**********************************************************************/
void ADC_init(void);
float ADC_opera(void);
/**********************************************************************************
*Variable definition area
**************************************************************************/
volatile unsigned int results[Num_of_Results]; //Store ADC12 sampling conversion results
static unsigned char index = 0;
float voltage; // Store voltage value operation results
/**********************************System main function****************************************/
int main( void )
{
WDTCTL=WDTPW+WDTHOLD;
BoardConfig(0xEF); // Board resource configuration
Init_CLK(); // Initialize system clock
WDTCTL = WDT_ADLY_1_9;
SFRIE1 |= WDTIE; // WDT interrupt enable
ADC_init(); // ADC12 conversion initialization
_EINT(); // Open global interrupt
while(1)
{
if(index==Num_of_Results-1)
voltage=ADC_opera();
_NOP();
LPM1;
}
}
/**************************************************************************************
* Function name: void ADC_init(void)
* Function: ADC12 initialization
* Parameters: None
* Return value: None
********************************************************************************/
void ADC_init(void)
{
P6SEL |= 0x01; // Open channel 1
ADC12CTL0 = ADC12ON+ADC12SHT0_8+ADC12MSC;
ADC12CTL1 = ADC12SHP+ADC12CONSEQ_2;
ADC12IE = 0x01;
ADC12CTL0 |= ADC12ENC;
ADC12CTL0 |= ADC12SC;
}
/******************************************************************************
* Function name: void ADC_opera(void)
* Function: Convert the data sampled by ADC into a decimal number
* Parameter: None
* Return value: None
************************************************************************************/
float ADC_opera(void)
{
unsigned long temp=0;
float voltage;
for(unsigned char i=0;i
temp +=results[i];
}
voltage=((temp/Num_of_Results)*3.3/4095);
return voltage;
}
/******************************************************************************
* Function Name: __interrupt void ADC12ISR (void)
* Function: ADC12 interrupt service function
* Parameter: None
* Return Value: None
********************************************************************************/
#pragma vector=ADC12_VECTOR
__interrupt void ADC12ISR (void)
{
switch(__even_in_range(ADC12IV,34))
{
case 6: // ADC12IFG0
results[index] = ADC12MEM0; //
index++; //
if (index == 8)
{
index = 0;
}
default: break;
}
LPM1_EXIT;
}
-----------------------------------------------------------------------------------------------------------------
This picture helped me a lot in my learning process. If you don’t understand the program, you can take a look at these, maybe you will gain something. I am studying integrated circuit design and I find it very helpful.
Here are some of my own test programs. Don't underestimate these few lines of programs, but I tested them N times.
#include "msp430x14x.h"
void InitADC12();
unsigned char wait;
int main( void )
{
// Stop watchdog timer to prevent time out reset
WDTCTL = WDTPW + WDTHOLD;
InitADC12();
return 0;
}
void InitADC12()
{
//_DINT();
ADC12CTL0 &= ~ENC; //ADC12CTL0 and 1 can only be set when ENC is reset!!! This is very important!!!
ADC12CTL0 = MSC + REFON + REF2_5V + SHT0_15 + SHT1_15;
/*MSC: Multiple sampling conversion bit, only valid for sequence sampling or multiple multiple conversions.
0: The sampling timer needs the rising edge of the SHI signal to trigger
1: The first time the sampling timer SHI signal is triggered, the subsequent conversions will start immediately after the last conversion. */
ADC12CTL1 |= SHP + CONSEQ_0;
/*SHP: 1: The sampling signal comes from the sampling timer 0: The sampling signal comes from the sampling input signal
CONSEQ: 0: Single channel single conversion 1: Sequential channel word conversion 2: Single channel multiple conversion 3: Sequential channel multiple conversion
CSTARTADD_x(0~15):Conversion starting position*/
ADC12MCTL0 = EOS + SREF_0 + INCH_7;
/* EOS: sequence end bit
SREF: Reference voltage selection
INCH: Analog input channel selection*/
ADC12IE |= 0x0001; //Open the corresponding analog channel interrupt, and trigger an interrupt after the conversion is completed
_UNITE();
//ADC12CTL0 |= ADC12ON + ENC; //Modify!!! // This is wrong
ADC12CTL0 |= ADC12ON;
ADC12CTL0 |= ENC;
wait = 0; //Variables can be defined here to indicate whether the conversion is completed
ADC12CTL0 |= ENC+ADC12SC; //Conversion start If you want to start the conversion at other times, you can put this sentence somewhere else.
while(wait==0) ;
}
#pragma vector=ADC_VECTOR
__interrupt void ADC()
{int result[7];
wait=1;
result[0] = ADC12MEM0;
/*unsigned char q0;
int *pmem=ADC12MEM;
//The conversion is complete.
for(q0=0;q0<16;q0++)
{
AdMem[q0]= *pmem;
pmem++;
}*/
}
Let's briefly introduce the difficult-to-understand aspects of this module.
1. MSP430 can use internal and external reference voltages, internal 1.5 or 2.5, external 0~3.3, which can be set through registers . The maximum input range of the sampling voltage is 0~3.3, that is, AVss~AVcc. Negative voltage cannot be detected. If negative voltage needs to be detected, an op amp can be used to bias the voltage into a positive voltage and then detect it.
2. Using external AVss~AVcc as the reference voltage is not stable enough, but has high accuracy. Using internal voltage is more stable.
3. The global interrupt _EINT() must be enabled in the program;
ADC12
********************************************************** /
#define__ MSP430_HAS_ADC12__ /* define to indicate the module is */
#define ADC12CTL0_(0x01A0u) /* ADC12 control 0 */
DEFW(ADC12CTL0, ADC12CTL0_)
#define ADC12CTL1_(0x01A2u) /* ADC12 control 1 */
DEFW(ADC12CTL1, ADC12CTL1_)
#define ADC12IFG_(0x01A4u) /* ADC12 interrupt flag */
DEFW(ADC12IFG, ADC12IFG_)
#define ADC12IE_(0x01A6u) /* ADC12 interrupt enable */
DEFW(ADC12IE, ADC12IE_)
#define ADC12IV_(0x01A8u) /* ADC12 interrupt vector word */
DEFW(ADC12IV, ADC12IV_)
#define ADC12MEM_(0x0140u) /* ADC12 conversion memory */
#ifndef __IAR_SYSTEMS_ICC__
#define ADC12MEM(ADC12MEM_) /* ADC12 conversion memory (Assembly) */
#else
#define ADC12MEM((int *)ADC12MEM_) /* ADC12 conversion memory ( C) */
#ENDIF
#define ADC12MEM0_(0x0140u) /* ADC12 conversion memory 0 */
defw(ADC12MEM0, ADC12MEM0_)
#define ADC12MEM1_(0x0142u) /* ADC12 conversion memory 1 */
defw(ADC12MEM1, ADC12MEM1_)
#define ADC12MEM2_(0x0144u) /* ADC12 conversion memory 2 */
defw(ADC12MEM2, ADC12MEM2_)
#define ADC12MEM3_(0x0146u) /* ADC12 conversion memory 3 */
DEFW(ADC12MEM3, ADC12MEM3_)
#define ADC12MEM4_(0x0148u) /* ADC12 conversion memory 4 */
DEFW(ADC12MEM4, ADC12MEM4_)
#define ADC12MEM5_(0x014Au) /* ADC12 conversion memory 5 */
DEFW(ADC12MEM5, ADC12MEM5_)
#define ADC12MEM6_(0x014Cu) /* ADC12 conversion memory 6 */
DEFW(ADC12MEM6, ADC12MEM6_)
#define ADC12MEM7_(0x014Eu) /* ADC12 conversion memory 7 */
DEFW(ADC12MEM7, ADC12MEM7_)
#define ADC12MEM8_(0x0150u) /* ADC12 conversion memory 8 */ DEFW
(ADC12MEM8, ADC12MEM8_)
#define ADC12MEM9_ (0x0152u) /* ADC12 conversion memory 9 */
DEFW (ADC12MEM9, ADC12MEM9_)
#define ADC12MEM10_ (0x0154u) /* ADC12 conversion memory 10 */
DEFW (ADC12MEM10, ADC12MEM10_)
#define ADC12MEM11_ (0x0156u) /* ADC12 conversion memory 11 */
DEFW (ADC12MEM11, ADC12MEM11_)
#define ADC12MEM12_ (0x0158u) /* ADC12 conversion memory 12 */
DEFW (ADC12MEM12, ADC12MEM12_)
#define ADC12MEM13_ (0x015Au) /* ADC12 conversion memory 13 */
DEFW (ADC12MEM13, ADC12MEM13_)
#define ADC12MEM14_ (0x015Cu) /* ADC12 conversion memory 14 */
DEFW (ADC12MEM14, ADC12MEM14_)
#define ADC12MEM15_ (0x015Eu) /* ADC12 conversion memory 15 */
DEFW (ADC12MEM15, ADC12MEM15_)
#define ADC12MCTL_(0x0080u) /* ADC12 memory control */
#ifndef __IAR_SYSTEMS_ICC__
#define ADC12MCTL(ADC12MCTL_) /* ADC12 memory control (for assembly) */
#else
#define ADC12MCTL((char *)ADC12MCTL_) /* ADC12 memory control (for C) */
#ENDIF
#define ADC12MCTL0_(0x0080u) /* ADC12 memory control 0 */
defc(ADC12MCTL0, ADC12MCTL0_)
#define ADC12MCTL1_(0x0081u) /* ADC12 memory control 1 */
defc(ADC12MCTL1, ADC12MCTL1_)
#define ADC12MCTL2_(0x0082u) /* ADC12 memory control 2 */
DEFC (ADC12MCTL2, ADC12MCTL2_)
#define ADC12MCTL3_ (0x0083u) /* ADC12 memory control 3 */
DEFC (ADC12MCTL3, ADC12MCTL3_)
#define ADC12MCTL4_ (0x0084u) /* ADC12 memory control 4 */
DEFC (ADC12MCTL4, ADC12MCTL4_)
#define ADC12MCTL5_ (0x0085u) /* ADC12 memory control 5 */
DEFC (ADC12MCTL5, ADC12MCTL5_)
#define ADC12MCTL6_ (0x0086u) /* ADC12 memory control 6 */
DEFC (ADC12MCTL6, ADC12MCTL6_)
#define ADC12MCTL7_ (0x0087u) /* ADC12 memory control 7 */
DEFC (ADC12MCTL7, ADC12MCTL7_)
#define ADC12MCTL8_ (0x0088u) /* ADC12 memory control 8 */
DEFC (ADC12MCTL8, ADC12MCTL8_)
#define ADC12MCTL9_ (0x0089u) /* ADC12 memory control 9 */
DEFC (ADC12MCTL9, ADC12MCTL9_)
#define ADC12MCTL10_ (0x008Au) /* ADC12 memory control 10 */
DEFC (ADC12MCTL10, ADC12MCTL10_)
#define ADC12MCTL11_ (0x008Bu) /* ADC12 memory control 11 */
DEFC (ADC12MCTL11, ADC12MCTL11_)
#define ADC12MCTL12_ (0x008Cu) /* ADC12 memory control 12 */
DEFC (ADC12MCTL12, ADC12MCTL12_)
#define ADC12MCTL13_ (0x008Du) /* ADC12 memory control 13 */
DEFC (ADC12MCTL13, ADC12MCTL13_)
#define ADC12MCTL14_ (0x008Eu) /* ADC12 memory control 14 */
DEFC (ADC12MCTL14, ADC12MCTL14_)
#define ADC12MCTL15_ (0x008Fu) /* ADC12 memory control 15 */
DEFC (ADC12MCTL15, ADC12MCTL15_)
/* ADC12CTL0 */
#define ADC12SC (0x001) /* ADC12 start conversion */
#define ENC (0x002) /* ADC12 enable conversion */
#define ADC12TOVIE (0x004) /* ADC12 timer overflow interrupt enable */
#define ADC12OVIE (0x008) /* ADC12 overflow interrupt enable */
#define ADC12ON (0x010) /* ADC12 on/enable */
#define REFON (0x020) /* ADC12 reference /
#define REF2_5V (0x040) /* ADC12 reference 0:1.5 V / 1:2.5 V */
#define MSC (0x080) /* ADC12 multi-SampleConversion */
#define SHT00 (0x0100u) /* ADC12 Sample Hold 0 Select 0 */
#define SHT01 (0x0200u) /* ADC12 Sample Hold 0 Select 1 */
#define SHT02 (0x0400u) /* ADC12 Sample Hold 0 Select 2 */
#define SHT03 (0x0800u) /* ADC12 Sample Hold 0 Select 3 */
#define SHT10 (0x1000u) /* ADC12 Sample Hold 0 Select 0 */
#define SHT11 (0x2000u) /* ADC12 Sample Hold 1 Select 1 */
#define SHT12 (0x4000u) /* ADC12 Sample Hold 2 Select 2 */
#define SHT13 (0x8000u) /* ADC12 Sample Hold 3 Select 3 */
#define MSH (0x0800u)
#define SHT0_0 (0 * 0x100u) /* ADC12 Sample Hold 0 Select Bit: 0 */
#define SHT0_1 (1 * 0x100u) /* ADC12 Sample Hold 0 Select Bit: 1 */
#define SHT0_2 (2 * 0x100u) /* ADC12 Sample Hold 0 Select Bit: 2 */
#define SHT0_3 (3 * 0x100u) /* ADC12 Sample Hold 0 Select Bit: 3 */
#define SHT0_4 (4 * 0x100u) /* ADC12 Sample Hold 0 Select Bit: 4 */
#define SHT0_5 (5 * 0x100u) /* ADC12 Sample Hold 0 Select Bit: 5 */
#define SHT0_6 (6 * 0x100u) /* ADC12 Sample Hold 0 Select Bit: 6 */
#define SHT0_7 (7 * 0x100u) /* ADC12 sample hold 0 select bit: 7 */
#define SHT0_8 (8 * 0x100u) /* ADC12 sample hold 0 select bit: 8 */
#define SHT0_9 (9 * 0x100u) /* ADC12 sample hold 0 select bit: 9 */
#define SHT0_10 (10 * 0x100u) /* ADC12 sample hold 0 select bit: 10 */
#define SHT0_11 (11 * 0x100u) /* ADC12 sample hold 0 select bit: 11 */
#define SHT0_12 (12 * 0x100u) /* ADC12 sample hold 0 select bit: 12 */
#define SHT0_13 (13 * 0x100u) /* ADC12 sample hold 0 select bit:
Previous article:Power consumption problem of Songhan MCU 8p2501
Next article:Screen snow program implemented by linked list
Recommended ReadingLatest update time:2024-11-23 10:51
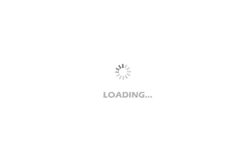
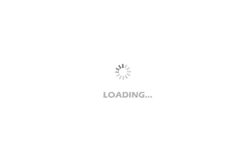
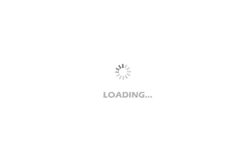
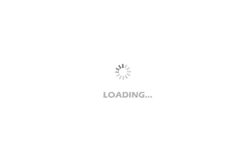
- Popular Resources
- Popular amplifiers
-
MSP430 series single chip microcomputer system engineering design and practice
-
oled multi-chip calling program
-
Microcontroller Principles and Applications Tutorial (2nd Edition) (Zhang Yuanliang)
-
Getting Started and Improving MSP430 Microcontrollers - National Undergraduate Electronic Design Competition Training Course
- Naxin Micro and Xinxian jointly launched the NS800RT series of real-time control MCUs
- How to learn embedded systems based on ARM platform
- Summary of jffs2_scan_eraseblock issues
- Application of SPCOMM Control in Serial Communication of Delphi7.0
- Using TComm component to realize serial communication in Delphi environment
- Bar chart code for embedded development practices
- Embedded Development Learning (10)
- Embedded Development Learning (8)
- Embedded Development Learning (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Intel promotes AI with multi-dimensional efforts in technology, application, and ecology
- ChinaJoy Qualcomm Snapdragon Theme Pavilion takes you to experience the new changes in digital entertainment in the 5G era
- Infineon's latest generation IGBT technology platform enables precise control of speed and position
- Two test methods for LED lighting life
- Don't Let Lightning Induced Surges Scare You
- Application of brushless motor controller ML4425/4426
- Easy identification of LED power supply quality
- World's first integrated photovoltaic solar system completed in Israel
- Sliding window mean filter for avr microcontroller AD conversion
- What does call mean in the detailed explanation of ABB robot programming instructions?
- STMicroelectronics discloses its 2027-2028 financial model and path to achieve its 2030 goals
- 2024 China Automotive Charging and Battery Swapping Ecosystem Conference held in Taiyuan
- State-owned enterprises team up to invest in solid-state battery giant
- The evolution of electronic and electrical architecture is accelerating
- The first! National Automotive Chip Quality Inspection Center established
- BYD releases self-developed automotive chip using 4nm process, with a running score of up to 1.15 million
- GEODNET launches GEO-PULSE, a car GPS navigation device
- Should Chinese car companies develop their own high-computing chips?
- Infineon and Siemens combine embedded automotive software platform with microcontrollers to provide the necessary functions for next-generation SDVs
- Continental launches invisible biometric sensor display to monitor passengers' vital signs
- I downloaded a source code from the Internet. Please help me find out what IDE this project was developed in. Thanks
- X-NUCLEO-IKS01A3 MakeCode Driver (Extension) Collection
- This strange circuit
- EEWORLD University ---- test
- What is ModBus? What are the differences and connections with RS485 protocol?
- Is this speed too fast? I have never experienced it properly.
- Application Differences between HT8691 and HT8691R
- Better
- SimpleLink MCU code migration guide: CC1310 from VQFN48 (7×7) to VQFN32 (5×5) code migration process reference
- Welcome the new moderator of the "201420208012" eSports