SPI is the abbreviation of Serial Peripheral Interface, which literally means serial peripheral interface. SPI is a synchronous serial communication method launched by Motorola. It is a four-wire synchronous bus. Because of its powerful hardware functions, the software related to SPI is quite simple, which allows the MCU to have more time to handle other matters. It should be noted here that patents are still very important in the electronics industry. Therefore, some other manufacturers renamed the SPI communication protocol to avoid high patent fees, but the hardware processing method is the same, just with a different name, such as SSI communication in Texas Instruments microcontrollers.
The commonly used SPI communication method is the standard four-wire system, as shown in the following circuit diagram:

MISO: Master input/slave output pin. This pin sends data in slave mode and receives data in master mode.
MOSI: Master output/slave input pin. This pin sends data in master mode and receives data in slave mode.
SCK: serial port clock, output of the master device and input of the slave device
NSS: Slave Select. This is an optional pin used to select the master/slave device.
The MOSI pins are connected to each other, and the MISO pins are connected to each other. In this way, data is transferred serially between the master and the slave (MSB first). Communication is always initiated by the master device. The master device sends data to the slave device through the MOSI pin, and the slave device returns the data through the MISO pin. This means that the data output and data input of full-duplex communication are synchronized with the same clock signal; the clock signal is provided by the master device through the SCK pin.
The more complicated part is the slave select (NSS) pin, which has two modes: software NSS mode and hardware NSS mode.
Software NSS mode: In this mode, the pin is used as a normal GPIO. Its input/output function is the same as that of GPIO. We often use this mode when operating external devices through STM32.
In hardware NSS mode: This mode is divided into two cases: Case 1, NSS output is enabled: When STM32 works as the master SPI and NSS output is enabled, the NSS pin is pulled low, and all NSS pins are connected to the NSS pin of the master SPI and configured as hardware NSS SPI devices, which will automatically become slave SPI devices; Case 2, NSS output is turned off: allowing operation in a multi-master environment.
Now that we have finished talking about the hardware connection, let me introduce the clock line and signal line.
When learning digital logic circuits, we have all heard teachers talk about data latching methods, such as rising edge latching, etc. Our SPI communication method handles data latching methods very flexibly in hardware, and provides four different data transmission modes through the configuration of two parameters, as shown in the figure below:


From the above figure, we can see that when CPHA is set high, the data is locked at the second clock edge; when CPHA is cleared, the data is locked at the first clock edge. When the CPOL parameter is set high, the data is locked at the falling edge of the clock signal, and the clock line idle state is always high. Conversely, the data is locked at the rising edge of the clock signal, and the idle state is always low.
For the data transmission process, the frame format can also be modified. For example, you can choose the MSB mode (the most significant bit is sent first) or the LSB mode (the least significant bit is sent first). You can also choose to insert a CRC check, etc. These advanced applications will not be explained in detail here due to the limited space of this article.
Next, let's take a look at the SPI hardware standard through the STM32 microcontroller's initialization process for the SPI peripheral.
void SPI_init(void)
{
RCC_APB2PeriphClockCmd(sFLASH_CS_GPIO_CLK | sFLASH_SPI_MOSI_GPIO_CLK | sFLASH_SPI_MISO_GPIO_CLK |
sFLASH_SPI_SCK_GPIO_CLK, ENABLE);
/*!< Configure the SPI peripheral clock and enable it*/
RCC_APB2PeriphClockCmd(sFLASH_SPI_CLK, ENABLE);
/*!< Configure SCK pin*/
GPIO_InitStructure.GPIO_Pin = sFLASH_SPI_SCK_PIN;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP; //This is set according to the specific application, for example, it can be configured as open-drain output
GPIO_Init(sFLASH_SPI_SCK_GPIO_PORT, &GPIO_InitStructure);
/*!< Configure MOSI pin*/
GPIO_InitStructure.GPIO_Pin = sFLASH_SPI_MOSI_PIN;
GPIO_Init(sFLASH_SPI_MOSI_GPIO_PORT, &GPIO_InitStructure);
/*!< Configure MISO pin */
GPIO_InitStructure.GPIO_Pin = sFLASH_SPI_MISO_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(sFLASH_SPI_MISO_GPIO_PORT, &GPIO_InitStructure);
/*!< Configure NSS pin as GPIO output*/
GPIO_InitStructure.GPIO_Pin = sFLASH_CS_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_Init(sFLASH_CS_GPIO_PORT, &GPIO_InitStructure);
/*!< SPI configuration*/
SPI_InitStructure.SPI_Direction = SPI_Direction_2Lines_FullDuplex; //Two data lines, bidirectional full duplex and half duplex
SPI_InitStructure.SPI_Mode = SPI_Mode_Master; //
SPI_InitStructure.SPI_DataSize = SPI_DataSize_8b; //
SPI_InitStructure.SPI_CPOL = SPI_CPOL_High; //CPOL is set high, the clock line is always high when idle, and the falling edge latches data
SPI_InitStructure.SPI_CPHA = SPI_CPHA_2Edge; //CPHA is set high, the second clock edge latches data
SPI_InitStructure.SPI_NSS = SPI_NSS_Soft; //Slave pin is software configuration mode
SPI_InitStructure.SPI_BaudRatePrescaler = SPI_BaudRatePrescaler_4; //SPI clock frequency is divided by 4
SPI_InitStructure.SPI_FirstBit = SPI_FirstBit_MSB; //MSB highest bit is sent first
SPI_InitStructure.SPI_CRCPolynomial = 7; //CRC check formula selects item 7
SPI_Init(sFLASH_SPI, &SPI_InitStructure);
/*!< Enable SPI */
SPI_Cmd(sFLASH_SPI, ENABLE);
}
The source code above is a demo example of ST company operating SPI flash. We will use the hardware operation of 74HC595 chip to configure and initialize the SPI peripheral.
Let's first look at the hardware operation timing diagram of 74HC595:

From the above figure, we can see that the clock line (SH_CP) is always low in the idle state, and the data is latched on the rising edge of the first clock edge. Therefore, we need to modify the two parameters of the above configuration initialization to the following:
SPI_InitStructure.SPI_CPOL = SPI_CPOL_Low; //CPOL is set high, the clock line is always low when idle, and the rising and falling edges latch data
SPI_InitStructure.SPI_CPHA = SPI_CPHA_1Edge; //CPHA is cleared, the first clock edge latches data
The other parameters do not need to be modified. The above source code has been tested by connecting STM32F103 and 8 74HC595 chips in series. The complete engineering source code of the example can be found and downloaded from the Electronic Products World Forum.
The standard four-wire SPI communication not only saves us precious microcontroller pins, but its standardized hardware protocol also provides great convenience for our embedded software programming. Rich peripheral device support, such as SPI flash storage, SPI interface SD card reader, SPI interface network communication module are already very popular. It can be seen that the application of peripheral SPI communication has become one of the necessary skills for an engineer.
Previous article:12-bit 4-20mA loop-powered thermocouple measurement system using Cortex-M3
Next article:Remote testing system of oil well liquid level based on embedded cloud technology
Recommended ReadingLatest update time:2024-11-23 11:02
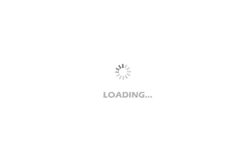
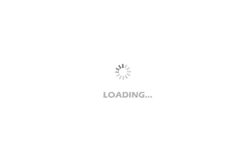
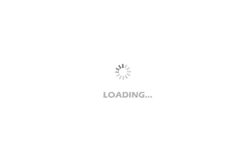
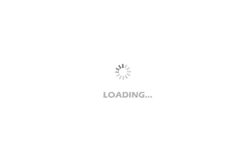
- Naxin Micro and Xinxian jointly launched the NS800RT series of real-time control MCUs
- How to learn embedded systems based on ARM platform
- Summary of jffs2_scan_eraseblock issues
- Application of SPCOMM Control in Serial Communication of Delphi7.0
- Using TComm component to realize serial communication in Delphi environment
- Bar chart code for embedded development practices
- Embedded Development Learning (10)
- Embedded Development Learning (8)
- Embedded Development Learning (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Intel promotes AI with multi-dimensional efforts in technology, application, and ecology
- ChinaJoy Qualcomm Snapdragon Theme Pavilion takes you to experience the new changes in digital entertainment in the 5G era
- Infineon's latest generation IGBT technology platform enables precise control of speed and position
- Two test methods for LED lighting life
- Don't Let Lightning Induced Surges Scare You
- Application of brushless motor controller ML4425/4426
- Easy identification of LED power supply quality
- World's first integrated photovoltaic solar system completed in Israel
- Sliding window mean filter for avr microcontroller AD conversion
- What does call mean in the detailed explanation of ABB robot programming instructions?
- STMicroelectronics discloses its 2027-2028 financial model and path to achieve its 2030 goals
- 2024 China Automotive Charging and Battery Swapping Ecosystem Conference held in Taiyuan
- State-owned enterprises team up to invest in solid-state battery giant
- The evolution of electronic and electrical architecture is accelerating
- The first! National Automotive Chip Quality Inspection Center established
- BYD releases self-developed automotive chip using 4nm process, with a running score of up to 1.15 million
- GEODNET launches GEO-PULSE, a car GPS navigation device
- Should Chinese car companies develop their own high-computing chips?
- Infineon and Siemens combine embedded automotive software platform with microcontrollers to provide the necessary functions for next-generation SDVs
- Continental launches invisible biometric sensor display to monitor passengers' vital signs
- EEWORLD University Hall----Live Replay: Protecting Clean Water Sources-ADI Water Quality Monitoring Solutions
- Share: How to convert C program from floating point to fixed point
- MicroPython major historical versions
- Hello, second half of 2020
- SignalTap II integrated assign statement problem
- Basic knowledge of 5G standards
- How to Reduce Waveform Noise When Measuring with an Oscilloscope
- What do the 8 defense zones represent in the building intercom system?
- [Live Review] ROHM DCDC Converter Design Seminar
- Overview of air conditioning automatic control