;**** Design of single-chip bell ringer************************************************
;* Number: LRDZAVR0100
;* Title: 32-point bell ringer routine
;* File name: timecontr.asm
;* Version: 1.0
;* Start date: 02.06.13
;* Target MCU: AT90S8515
;* Technical support: http://www.dianz.cn
;* Hardware structure: PA0-5 corresponds to the bits of 6 LED digital display tubes, PC0-7 corresponds to the segments of each LED digital display tube, and PB4-7 is the keyboard.
;* Software description: After power-on, the clock automatically runs from 00.00.00 (hour/minute/second). In the running state, press PB4 (set
key) for more than 3 seconds to cycle through the adjustment time/operating parameters, then press PB5 to confirm the selected operation, and after entering the adjustment operation,
;* press PB5 to select the parameter to be adjusted, and press PB6 (increase key) to complete the parameter adjustment operation. Press PB7 to enter the time control operation, PB1 lights up,
;* press PB7 again to stop the time control operation, and the running time is echoed. When the timing is up, PB0 lights up.
;****************************************************
.device AT90S8515
.include "8515def.inc"
.equ DISBUF = $0060 ;Display buffer start address.equ
TIMEDIS = $0066 ;Clock hour, minute, second storage unit.equ
XSDBZ = $0073 ;Decimal point flag.equ
DISTBL = $0f00 ;Display character encoding table start address.equ
TIMECTR = $0010 ;32-segment time control table first address (EEPRON)
.equ TIMECBZ = $0080 ;Control time up flag.cseg
.org
$0000
rjmp RESET
.org $0007
rjmp TIM0_OVF
;**** Single-chip bell design main program********************************************************
.def cntms =r9
.def hour =r10
.def minute =r11
.def second =r12
.def EEdwr =r16
.def EEawr =r17
.def EEawrh =r18
.def EEdrd =r16
.def EEard =r17
.def EEardh =r18
.def temp =r20
.def temp1 =r21
.def temp2 =r22
.def temp3 =r23
.def temp4 =r24
.def TIM0INT =r19
.org $0020
RESET: ldi temp,low(ramend) ;set stack pointer
out spl,temp
ldi temp,high(ramend)
out sph,temp
ldi temp,0b11111111 ;set input and output status of each port line
out ddra,temp
ldi temp,0b00000011
out ddrb,temp
ldi temp,0b11111111
out ddrc,temp
ldi zl,TIMECBZ
clr zh
clr temp
st y,temp
clr r9 ;clear each working register
clr r10
clr r11 clr
r12 clr
r13
clr r14
clr r15
clr r16
clr r17
clr r18
clr r19
clr r25
ldi temp,$5
out tccr0,temp ;T0 set ck/1024 frequency division
ldi temp,256-195
out tcnt0,temp ;install T0 time constant
res1: ldi temp,$02 ;enable T0 interrupt and time
out timsk,temp
sei ;open interrupt
res2: clr r6
clr r7
sbi PORTb,1
sbi PORTB,0
res3: ldi temp,$af
res4: rcall colodis ;clock running time display
dec temp
brne res4
sbis pinb,7 ;key scan
rjmp PB7M
sbis pinb,4
rjmp BP4M ;go to parameter setting
rjmp res3
;****《System Function Program》
.org $0080 ; define the first address of the system function program storage area
;****32-point bell ringer working procedure********************************************************
PB7M: cbi PORTb,1 ;set the running indicator light on
clr r25
clr r7
GNCX11:ldi r17,TIMECTR ;the first control point EEPRON address
clr r18
lsl r25
lsl r25
add r17,r25 ;get the control point address in EEPRON (Aj=A0+4*j), A=(r17), j=(r25)
lsr r25
lsr r25
rcall EERead
cpi r16,0
breq GNCX12 ;r16=(r17)=0 means “ON”
inc r25
cpi r25,31
brmi GNCX11
rjmp GNCX16
GNCX12:inc r17
rcall EERead
cp r16,r10
brne GNCX13
inc r17
rcall EERead
cp r16,r11
brne GNCX14
inc r17
rcall EERead
mov r7,r16
inc r7
cbi PORTb,0
rjmp GNCX15
GNCX13:dec r17
inc r25
rjmp GNCX11
GNCX14:dec r17
dec r17
inc r25
rjmp GNCX11
GNCX15:cpi TIM0INT,1
brne GNCX17
clr TIM0INT
dec r7
brne GNCX17
clr r7
sbi PORTb,0
rjmp GNCX16
GNCX17:rcall colodis
sbic pinb,7
rjmp GNCX15
rjmp res2
GNCX16:ldi temp,$5f
GNCX18:rcall colodis
dec temp
brne GNCX18
sbic pinb,7
rjmp GNCX11
rjmp res2
;****MCU bell ringer parameter setting
BP4M: ldi temp3,$ff
ldi temp4,$f
BP4M1: nop
rcall colodis
sbic pinb,4
rjmp res1
dec temp3 ;PB4 is pressed
brne BP4M1
inc temp3
dec temp4
brne BP4M1
ldi temp3,$ff ;After more than 3 seconds, enter
ldi temp4,$f
BP4M2: clr yh
ldi yl,low(DISBUF) ;Set display cloc.
ldi temp,25
st y+,temp
st y+,temp
ldi temp,18
st y+,temp
ldi temp,19
st y+,temp
ldi temp,20
st y+,temp
ldi temp,18
st y,temp
ldi zl,low(XSDBZ)
clr zh
ldi temp,0b00100000 ; b0-5 corresponds to the decimal point of the LED from left to right 1-6, set "1" at the decimal point. [page]
st z,temp
rcall display
sbis pinb,5
rjmp cloc ;Enter time setting
dec temp3
brne BP4M2
inc temp3
dec temp4
brne BP4M2
BP4M3: ldi temp3,$ff
ldi temp4,$f
BP4M4: clr yh
ldi yl,low(DISBUF) ;Set display labor.
ldi temp,25
st y+,temp
ldi temp,19
st y+,temp
ldi temp,10
st y+,temp
ldi temp,11
st y+,temp
ldi temp,20
st y+,temp
ldi temp,22
st y,temp
ldi zl,low(XSDBZ)
clr zh
ldi temp,0b00100000 ;b0-5 correspond to the 1-6th decimal point of LED from left to right, set "1" at this decimal point.
st z,temp
rcall display
sbis pinb,5
rjmp GNCS1 ;Enter working parameter setting
dec temp3
brne BP4M4
inc temp3
dec temp4
brne BP4M4
sbic pinb,4
rjmp BP4M
rjmp res1
;****Clock adjustment************************************************
cloc: clr temp
out timsk,temp ;Do not allow T0 interrupt
cli ;Disable interrupt
rcall colodis
mov temp,r10
clr temp4
ldi temp1,20
ldi temp3,$80
cloc3: ldi yl,low(DISBUF)
add yl,temp4
sbic pinb,5 ;Select hours, minutes and seconds
rjmp cloc4
dec temp1
brne cloc4
ldi temp1,80
inc temp4
inc temp4
add yl,temp4
cpi yl,$68
brmi cloc4
clr temp4
ldi yl,low(DISBUF)
cloc4: nop
sbic pinb,6 ; adjust the hour, minute and second values
rjmp cloc8
dec temp3
brne cloc8
ldi temp3,$20
cloc5: cpi yl,$60
brne cloc6
inc r10
mov temp,r10
cpi temp,24
brmi cloc8
clr temp
clr r10
cloc6: cpi yl,$62
brne cloc7
inc r11
mov temp,r11
cpi temp,60
brmi cloc8
clr temp
clr r11
cloc7: cpi yl,$64
brne cloc8
inc r12
mov temp,r12
cpi temp,60
brmi cloc8
clr temp
clr r12
cloc8: cpi yl,$60
brne cloc9
ldi zl,low(XSDBZ)
clr zh
ldi temp,0b00000010 ;b0-5 corresponds to the 1-6th decimal point of the LED from left to right, set "1" at this decimal point.
st z,temp
mov r16,r10
rjmp cloc11
cloc9: cpi yl,$62
brne cloc10
ldi zl,low(XSDBZ)
clr zh
ldi temp,0b00001000 ;b0-5 corresponds to the 1-6th decimal point of the LED from left to right, set "1" at this decimal point.
st z,temp
mov r16,r11
cloc10: cpi yl,$64
brne cloc11
ldi zl,low(XSDBZ)
clr zh
ldi temp,0b00100000 ; b0-5 corresponds to the 1-6th decimal point of LED from left to right, set "1" at the decimal point.
st z,temp
mov r16,r12
cloc11: rcall bto
st y+,r3
st y,r4
rcall display
sbis pinb,4 ; exit
rjmp cloc12
rjmp cloc3
cloc12: ldi temp,$02
out timsk,temp ; allow T0 interrupt
sei ; open interrupt
rjmp res1
; ****32-point bell working parameter adjustment *************************************************************
GNCS1: clr r25 ;first control point
clr r22 ;r22 is 0 to display the switch page, 1 to display the h-xx page, 2 to display the m-xx page, and 3 to display the c-xx page
ldi zl,low(XSDBZ)
clr zh
ldi r19,0b00000010
st z,r19
gncs: ldi r17,TIMECTR ;first control point EEPRON address
clr r18
ldi yl,low(DISBUF) ;set the display buffer start address
clr yh
lsl r25
lsl r25
add r17,r25 ;get the control point address in EEPRON (Aj=A0+4*j), A=(r17), j=(r25)
lsr r25
lsr r25
mov r16,r25
rcall bto
st y+,r3
st y+,r4
cpi r22,0
breq kgymxs
cpi r22,1
breq hymxs
cpi r22,2
breq mymxs
cpi r22,3
breq cymxs
clr r22
rjmp gncs
kgymxs: rcall EERead
cpi r16,0
brne offxs
ldi r23,27 ;display xx.--on
st y+,r23
st y+,r2 3
ldi r23,20
st y+,r23
ldi r23,26
st y,r23
rjmp gzcsxs
offxs: ldi r23,27 ;display xx.-off
st y+,r23
ldi r23,20
st y+,r23
ldi r23,15
st y+,r23
st y,r23
rjmp gzcsxs
hymxs: ldi r23,28 ;display xx.h-aa
st y+,r23
ldi r23,27
st y+,r23
inc r17
rcall EERead
rcall bto
st y+,r3
st y+,r4
dec r17
rjmp gzcsxs
mymxs: ldi r23,26 ;display xx.n-aa
st y+,r23
ldi r23,27
st y+ ,r23
inc r17
inc r17
rcall EERead
rcall bto
st y+,r3
st y+,r4
dec r17
dec r17
rjmp gzcsxs
cymxs: ldi r23,18 ;show xx.c-aa
st y+,r23
ldi r23,27
st y+,r23
inc r17
inc r17
inc r17
rcall EERead
rcall bto
st y+,r3
st y+,r4
dec r17
dec r17
dec r17
gzcsxs: ldi r23,40
ldi r24,40
gzcsxs1: rcall display
sbis pinb,5 ; turn page
rjmp fycz ; yes, turn page
sbis pinb,6 ; change value
rjmp bzcz ; yes, change value
sbis pinb,4 ; reset
rjmp res1 ; yes, reset
rjmp gncs[page]
fycz: dec r23
brne gzcsxs1
rcall EERead
cpi r16,0
brne fycz1
inc r22
cpi r22,4
brmi fycz2
clr r22
fycz1: inc r25
cpi r25,32
brmi fycz2
clr r25
fycz2: rjmp gncs
bzcz: dec r24
brne gzcsxs1
cpi r22,0
brne bzcz2
bzcz1: rcall EERead
cpi r16,0
breq bzcz11
clr r16
rjmp bzcz12
bzcz11: ldi r16,1
bzcz12: rcall EEWrite
rjmp gncs
bzcz2: add r17,r22
rcall EERead
inc r16
cpi r22 ,1
breq bzcz21
cpi r16,60
brmi bzcz22
clr r16
rjmp bzcz22
bzcz21: cpi r16,24
brmi bzcz22
clr r16
bzcz22: rcall EEWrite
sub r17,r22
rjmp gncs
;****Display subroutine********************************************
;*
;* Function: Send the data stored in the SRAM display buffer to the digital tube display
;* Entry: DISBUF---Display buffer start address (SRAM)
;* Y--Display buffer pointer
;* DISTBL---Display character encoding table start address (FLASH)
;* LEDSXW---LED flash register bit setting
;* XSDBZ----Decimal point setting
;* Exit: Y--Display buffer pointer, pointing to the high address
;*
;****Program segment
display: nop
push temp
push temp1
push temp2
push temp3
push temp4
push r6
ldi temp1,6
ldi temp3,0b11011111
ldi yl,low(DISBUF)
ldi yh,high(DISBUF)
ldi xl,low(XSDBZ)
clr xh
ld r6,x
disp1: ld temp,y+
ldi zl,low(DISTBL)
ldi zh,high(DISTBL)
add zl,temp
lsl zl
rol zh
lpm
lsr r6
brcc disp2
ldi temp4,0b10000000
or r0,temp4
disp2: out PORTC,r0
in temp2,PORTA
ori temp2,0b00111111
and temp2,temp3
out PORTA,temp2
sec
ror temp 3
rcall delay
in temp2,PORTA
ori temp2,0b00111111
out PORTA,temp2 ;off display
dec temp1
brne disp1
pop r6
pop temp4
pop temp3
pop temp2
pop temp1
pop temp
ret
;****Clock display subroutine********************************************
colodis: push temp
ldi yl,low(DISBUF) ;Set the start address of the display buffer
clr yh
ldi xl,low(TIMEDIS) ;Set the storage unit of the clock hours, minutes and seconds
clr xh
ldi zl,low(XSDBZ)
clr zh
ldi temp,0b00001010 ;b0-5 corresponds to the 1-6 decimal points of the LED from left to right, set "1" at this decimal point.
st z,temp
rcall ram6ram
rcall display
pop temp
ret
;****Delay subroutine************************************************
delay: nop ;Delay subroutine
push temp2
push temp1
ldi temp2,$ff
lp1: ldi temp1,$f
lp2: dec temp1
brne lp2
dec temp2
brne lp1
pop temp1
pop temp2
ret ;Subroutine returns
;****Write EEPRON subroutine********************************************
;*
;*Function: Write the contents of EEDWR(r16) into the EEPRON unit with the contents of EEAWR(r18:r17) as the address.
;*
;****Program segment
EEWrite:sbic EECR,EEWE
rjmp EEWrite
out EEARH,EEawrh
out EEARL,EEawr
out EEDR,EEdwr
sbi EECR,EEMWE
sbi EECR,EEWE
ret
;****Read EEPRON subroutine*************************************
;*
;*Function: Read the contents of the EEPRON unit with the content of EEARD(r18:r17) as the address and send them to the EEDRD(r16) register.
;*
;****Program segment
EERead: sbic EECR,EEWE
rjmp EERead
out EEARH,EEardh
out EEARL,EEard
sbi EECR,EERE
sbi EECR,EERE
in EEdrd,EEDR
ret
;****6-byte SRAM content transfer subroutine********************************************************
;*
;* Function: transfer 6 consecutive bytes in SRAM from BLOCK1 to BLOCK2
;* x---pointer to the starting address (SRAM) of the 6 bytes to be transferred
;* y---pointer to the starting address (SRAM) of the 6 bytes to be placed
;*
;****Program segment
ram6ram:push temp1
push temp2
ldi temp1,$06
ramd1: ld temp2,x+
st y+,temp2
dec temp1
brne ramd1
pop temp2
pop temp1
ret
;****8-bit binary number to 3-bit BCD number subroutine********************************************************
;*
;*Function: Convert an 8-bit unsigned binary number to a 3-bit BCD number.
;*Entry: r16---8-bit unsigned binary number.
;*Exit: BCD code is placed in r2:r3:r4, r2 stores the hundreds digit.
;*
;****Program segment
bt push r16
clr r2
clr r3
clr r4
rjmp bto2
bto1: inc r2
bto2: subi r16,100
brpl bto1
ldi temp,100
add r16,temp
rjmp bto4
bto3: inc r3
bto4: subi r16,10
brpl bto3
ldi temp,10
add r16,temp
mov r4,r16
pop r16
ret
;****Clock 0 interrupt service routine******************************************************
.org $0bf0
TIM0_OVF: push temp
ldi temp,256-195
out tcnt0,temp ;reinstall T0 time constant
inc cntms
mov temp,cntms
cpi temp,40
brne timecunt
clr cntms
inc second
mov temp,second
cpi temp,60
brne timecunt
clr second
ldi TIM0INT,1
inc minute
mov temp,minute
cpi temp,60
brne timecunt
clr minute
inc hour
mov temp,hour
cpi temp,24
brne timecunt
clr hour
timecunt: ldi xl, low(TIMEDIS)
clr xh
mov r16,hour
rcall bto
st x+,r3
st x+,r4
mov r16,minute
rcall bto
st x+,r3
st x+,r4
mov r16,second
rcall bto
st x+,r3
st x+,r4
pop temp
reti
;****Glyph table********************************************
.cseg
.org DISTBL
.dw 0x003f,0x0006,0x005b,0x004f,0x0066,0x006d,0x007d,0x0007,0x007f,0x006f
.dw 0x0077,0x007c,0x0039,0x005e,0x0079,0x0071,0x0073,0x0076,0x0058,0x0038
.dw 0x005c,0x0067,0x0050,0x006e,0x0078,0x0000,0x0054,0x0040,0x0074,0x0000
Previous article:Single chip stopwatch course design
Next article:8×8 dot matrix LED driven by single chip microcomputer
Recommended ReadingLatest update time:2024-11-16 19:32
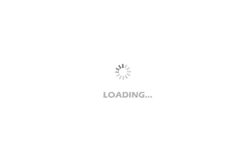
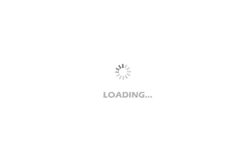
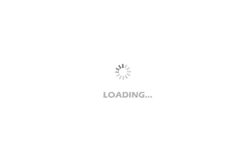
- Popular Resources
- Popular amplifiers
-
Wireless Sensor Network Technology and Applications (Edited by Mou Si, Yin Hong, and Su Xing)
-
Modern Electronic Technology Training Course (Edited by Yao Youfeng)
-
Modern arc welding power supply and its control
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- RF Circuit Engineering Design
- What is the role of the pull-up resistor? How to choose the value of the pull-up resistor
- How to obtain additional information of Pingtouge scenario-based Bluetooth MESH
- I have encountered a problem with the iTOP4418 development board. Is there any big guy who can help me?
- FLASH cannot be downloaded
- [GD32E231 DIY Contest] 3. Timer + button (supports long press and short press) + LED
- New findings from Nature's sub-journal: Do we need to work 10% more per week after working from home?
- Harbin in my eyes in 2020
- RISC-V IDE MRS usage notes (I): Target mode doesn't match
- How to distinguish live wire, neutral wire and ground wire? Is it reliable to distinguish them by color?