void int1proc() interrupt IE1_VECTOR using 1
{
unsigned char i, key;
code unsigned char PS2TAB[] = {//20-key PS2 keypad key code table
0x70,//0
0x69,//1
0x72,//2
0x7a,//3
0x6b,//4
0x73,//5
0x74,//6
0x6c,//7
0x75,//8
0x7d,//9
0x05,//F1
0x06,//F2
0x04,//F3
0x0c,//F4
0x03,//F5
0x0b,//F6
0x5a,//Enter
0x76,//Esc
0x66,//Bksp
0x71//KP.
};
if (PS2Buffers.PS2KeyCount == 0){//PS2 start bit testif
(!PS2CLOCK && !PS2DATA){//Low level is the start bitif
(PS2Buffers.PS2KeyExtFlage != 0xf0){
PS2Buffers.PS2KeyTemp = 0;
PS2Buffers.PS2KeyExtFlage = 0;
PS2Buffers.PS2KeyPopError = 0;
}
PS2Buffers.PS2KeyCount ++;//Pulse count
}
else PS2Buffers.PS2KeyPopError = 0xeb;//Set key start bit error number 0xeb
}
else if (PS2Buffers.PS2KeyCount < 9){//PS2 data bit
key = PS2Buffers.PS2KeyTemp;//Get keyboard buffer shift data
key >>= 1;
if (PS2DATA) key = 0x80;
PS2Buffers.PS2KeyTemp = key;
PS2Buffers.PS2KeyCount++;//Pulse count
}
else if (PS2Buffers.PS2KeyCount == 9){//PS2 data odd parity
ACC = PS2Buffers.PS2KeyTemp;//Get keyboard buffer shift data (C51 takes even parity)
if (P != PS2DATA) PS2Buffers.PS2KeyCount ++;//Pulse count
else{
PS2Buffers.PS2KeyPopError = 0xec;////Set key odd parity error number 0xec
PS2Buffers.PS2KeyCount = 0;//Pulse count resets to zero
}
}
else if (PS2Buffers.PS2KeyCount == 10){//Stop bit
key = PS2Buffers.PS2KeyTemp;//Get keyboard buffer shift data
PS2Buffers.PS2KeyCount = 0;//Pulse count resets to zero
if (PS2DATA){//High level is stop bit
if (key == 0xe0){//This is an extended
keyPS2Buffers.PS2KeyExtFlage = 0xe0;//Set the extended key flag (the small keyboard only has the Enter key)
}
else if (key == 0xf0){//This is a key break code, key releasePS2Buffers.PS2KeyExtFlage
= 0xf0;//Set the key release flag
}
else{//This must be a key valueif
((key == 0xaa) (key == 0xfa)) PS2Buffers.PS2KeyPushCount = 0;//Long
key press counter clearselse
{
PS2CLOCK = 0;//Prevent the PS2 keyboard from sending back data immediatelyPS2Buffers.PS2KeyPopError
= 0xaa;//Error codefor
(i = 0; i < 20; i++){
if (key == (PS2TAB[i])){//Searchkey
= i + 1;
if (PS2Buffers.PS2KeyExtFlage == 0xf0){
key = 0x80; //Key release
PS2Buffers.PS2KeyPushCount = 0; //Long press key counter cleared
PS2Buffers.PS2KeyMessage = key; //Save current key value and execute command
}
else{
if (key != PS2Buffers.PS2KeyVal){//Changed a
keyPS2Buffers.PS2KeyPushCount = 0;//Reset the long-press key
counterPS2Buffers.PS2KeyMessage = key;//Save the current key value and execute the command
}
else{//The key has not been changedPS2Buffers.PS2KeyPushCount
++;//Long-press key counter countif
(PS2Buffers.PS2KeyPushCount > DEFPS2PUSHCOUNT){//Long-press time is
upPS2Buffers.PS2KeyMessage = key 0x40;//Save the current key value and execute
the
commandPS2Buffers.PS2KeyPushCount
= 0;//Reset the long-press key counter
}
}
}
PS2Buffers.PS2KeyVal = key;//Save the current key value 1~20 or 0x80+(1~20)
PS2Buffers.PS2KeyTemp = 0;//Key code shift recorder
PS2Buffers.PS2KeyExtFlage = 0;//Extended key flag
PS2Buffers.PS2KeyPopError = 0;//Key release flag or error code
break;
}
}
if (PS2Buffers.PS2KeyPopError) PS2Buffers.PS2KeyPushCount = 0;//Long press key
Counter cleared
PS2CLOCK = 1;//Release PS2 clock bus
}
}
}
else PS2Buffers.PS2KeyPopError = 0xed;//Set stop bit error number 0xed
}
else PS2Buffers.PS2KeyCount = 0;//PS2 keyboard error
}[page]
/*------------------------------------------------------------------
Run PS2 keyboard scattered transfer command using function pointer array
-------------------------------------------------------------------*/
void PS2CommandExec(unsigned char key)
{
unsigned int i;
code void *funcpushbuffers[] = {//Command transfer table (16 DWs in assembly)
/*-------------------------------------------------------------------
Keyboard c program 15 key press, key release and long key press event processing function pointer address
-------------------------------------------------------------------*/
(void *)ClrWdt + 0x0000,
/*---------------------------------------------
10 function key press event processing (independent management)
----------------------------------------------*/
(void *)PS2F1KeyPush + 0x5b7d, //Function key F1 key press event processing
(void *)PS2F2KeyPush + 0xa6ea, //Function key F2 key press event processing
(void *)PS2F3KeyPush + 0xf157, //Function key F3 key press event processing
(void *)PS2F4KeyPush + 0x4cc4, //Function key F4 key press event processing
(void *)PS2F5KeyPush + 0x9731, //Function key F5 key press event processing
(void *)PS2F6KeyPush + 0xe2ae, //Function key F6 key press event processing
(void *)PS2EnterKeyPush + 0x3d1b, //Function key Enter key press event processing
(void *)PS2EscKeyPush + 0x8888, //Function key Esc key press event processing
(void *)PS2BkspKeyPush + 0xd3f5, //Function key Bksp key press event processing
(void *)PS2KpKeyPush + 0x2e62, //Function key Kp key press event processing
/*---------------------------------------------
2 key release event processing (centralized management)
----------------------------------------------*/
(void *)PS2NumberKeyPop + 0x79df, //Number key release event processing
(void *)PS2FuncKeyPop + 0xc44c, //Function key release event processing
/*---------------------------------------------
2 long key press event processing (centralized management)
------------------------------*/
(void *)PS2NumberKeyPushL + 0x1fb9, //Number key long press event processing
(void *)PS2FuncKeyPushL + 0x6a26, //Function key long press event processing
/*---------------------------------------------
1 number key press event processing (centralized management)
----------------------------------------------*/
(void *)PS2NumberKeyPush + 0xb593 //Number key 0~9 press event processing
};
/*--------------------------------------------*/
i = key;
key &= 0x3f;//Remove the key release and long press flags, and get the true key codekey
--;
if (key < 20){//Only 20 keysif
(key < 10){//Number keys 0~9
if (i <= 10) key = 15;//(scattered transfer number 0) press number keys 0~9
else{
if (i & 0x80) key = 11;//(scattered transfer number 11) release number keys 0~9
else key = 13;//(scattered transfer number 13) long press number keys 0~9
}
}
else{//Press function keys F1~F6, Enter..KP
if ((i & 0xc0) == 0) key -= 9;//(scattered transfer number 1~10) press function keys F1~F6, Enter..KP
else{
if (i & 0x80) key = 12;//(scattered transfer number 12) release function key
else key = 14;//(scattered transfer number 14) long press function key
}
}
ClrWdt(); //Feed the dog (who knows how long the keyboard program will run, just feed it first)
i = ((key * 53 & 0xf) * 0x1000)+ ((key * 43 & 0xf) * 0x100) + ((key * 23 &
0xf) * 0x10) + (key * 13 & 0xf);
_icall_((void *)funcpushbuffers[key] - i); //Get the keyboard transfer table and execute the keyboard command
}
}
This keyboard program is very different from the general ones on the Internet. It makes full use of external interrupts (0 words) hotpower[1 time]
****The following is a version of the single-chip keyboard C program. Welcome readers to experiment. http://www.51hei.com to compile **************************************
unsigned CHAR key,key_h,kpush;
unsigned int key_l;
//Buttons connected to p1.0, p1.1, p1.2
void int_t0(void) interrupt 1 {
unsigned CHAR dd,i;
TL0=TL0+30;TH0=0xfb; //800
/* key identification */
if ((P1&0x7)==0x7) {
if ((key_l>30)&&(key_l<800)&&(key_h>30)) { //Release the key. If the previous key press time is less than 1 second, read the key value
key=kpush;
}
if ((++key_h)>200) key_h=200;
key_l=0;
if (key>=0x80) key=0; //If the previous key was pressed for 1 second, clear the key value
} else {
kpush=P1&0x7;
key_l++;
if ((key_l>800)&&(key_h>30)) { //If the key is pressed for more than 1 second, add 0x80 to the key value to indicate a long key
key=kpush|0x80;
key_h=0;
key_l=0;
}
}
}
void main(void) {
TMOD=0x1;TR0=1;ET0=1;EA=1;
while (1) {
while (!key) {}
SWITCH (key) {
case 1:break;
case 2:break;
}
}
}
Previous article:Notes on single chip microcomputer programming and stepper motor control
Next article:Design of air pressure altimeter based on MCU
Recommended ReadingLatest update time:2024-11-16 17:53
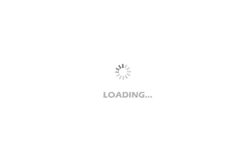
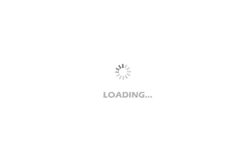
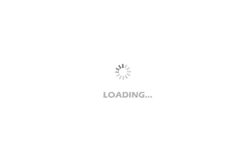
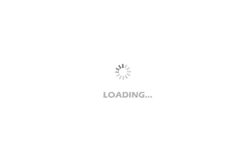
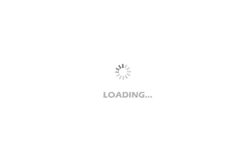
- Popular Resources
- Popular amplifiers
-
Wireless Sensor Network Technology and Applications (Edited by Mou Si, Yin Hong, and Su Xing)
-
Modern Electronic Technology Training Course (Edited by Yao Youfeng)
-
Modern arc welding power supply and its control
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- [Original] I recently heard that ST's chips have started to increase in price. So can domestic chips rise through this opportunity?
- Free review: Domestic RISC-V Linux board Fang Xingguang VisionFive
- How to configure DM368 to make the output image horizontally mirrored when using sensor image acquisition
- Chat: How does your company control technical documents?
- [ModelSim FAQ] Can't launch the ModelSim-Altera software
- Please give me some advice!!!
- Can the vehicle gateway be integrated into other modules?
- How to select MOS tubes for three-phase full-bridge inverter drive circuits?
- [RT-Thread Reading Notes] Part 2 (4) Events, Software Timers, Memory Management
- Apply for free | STM32F7508-DK Discovery Kit and have a chance to win a prize of 10,000 yuan!