Recently, due to work needs, I am preparing to make an offline downloader for AT89S5X. The initial consideration is to use AT89S52 as the host and write the target code into AT24C64 through the serial port (AT24C64 has 8K space, just enough for 52). After arriving at the site, the code in the EEPROM can be written into the target microcontroller through the host through the simulated ISP timing to achieve offline download.
Before drawing the schematic, I planned to figure out the ISP timing of S5X. When I first started reading the English document, I was so confused... I mistook the high-bit transmission for the low-bit transmission selection... I spent two days without realizing it... Because the AT document was not clear about ISP (at least in my opinion), I searched the source code of USBASP on the Internet and referred to the ISP timing of S5X in it, and finally understood it... Here are some key points about the ISP timing programming of S5X:
1: About reset timing
RST = 1;
SCK = 0;
DELAY(1);
RST = 0; //Note that there is a pull-down process
DELAY(1);
RST = 1;
DELAY(1);
2: Connect to the computer to check whether to enter the ISP programming mode
ISP_WR(0XAC);
ISP_WR(0X53);
ISP_WR(0X00);
TempData[3]=ISP_WR(0X00); //The fourth byte is written and read at the same time. If the data is 0X69, it means that the
In ISP mode
3: Regarding the read identification word, before the Erase command was tested, the data just read out was normal (1E 52 06). Later, when testing the Erase command, I suspected that
the Erase delay time was too short. Unfortunately, the read value was always 1F 7F 1F.
ISP_WR(0X28);
ISP_WR(0X00);
ISP_WR(0X00);
TempData[0] = ISP_WR(0X00); //1E
ISP_WR(0X28);
ISP_WR(0X01);
ISP_WR(0X00);
TempData[1] = ISP_WR(0X00); //52
ISP_WR(0X28);
ISP_WR(0X02);
ISP_WR(0X00);
TempData[2] = ISP_WR(0X00); //06
4: Regarding the Erase command, the delay I found on the Internet is about 500MS. I haven't tested it specifically yet. I will test and determine the delay
parameters after the entire program function is complete.
5: About ISP pin connections:
MOSI: Master out, slave in
MISO: Master in, slave out
SCK/RST: This should be self-explanatory
==================================================
The following is my test program.
=============================================
/**********************************************************
S5X ISP test program
**********************************************************/
#include
#include "1602.h"
sbit RST = P2^3;
sbit MISO = P2^2;
sbit MOSI = P2^1;
sbit SCK = P2^0;
ISP_WR(uchar command);
ISP_RD();
void DELAY(uint temp);
void main()
{
uchar TempData[4];
P0 = 0XFF;
P1 = 0XFF;
P2 = 0XFE;
P3 = 0XFF;
Lcd_Init();
MOSI = 1;
MISO = 1;
RST = 1;
SCK = 0;
DELAY(1);
RST = 0; //Note
DELAY(1) here;
RST = 1;
DELAY(1);
ISP_WR(0XAC);
ISP_WR(0X53);
ISP_WR(0X00);
TempData[3]=ISP_WR(0X00); //Connection test
/* //Write test
ISP_WR(0X40
);
ISP_WR(0X00);
ISP_WR(0X00); ISP_WR(0XA5);
DELAY(5000);
*/
/*
ISP_WR(0XAC); //Erase test
ISP_WR(0X80);
ISP_WR(0X00);
ISP_WR(0X00);
DELAY(5000);
*/
ISP_WR(0X28);
ISP_WR(0X00);
ISP_WR(0X00);
TempData[0] = ISP_WR(0X00); //1E
ISP_WR(0X28);
ISP_WR(0X01);
ISP_WR(0X00);
TempData[1] = ISP_WR( 0X00); //52
ISP_WR(0X28);
ISP_WR(0X02);
ISP_WR(0X00
);
TempData[2] = ISP_WR(0X00); //06
/*
ISP_WR(0X20); //Read test
ISP_WR(0X00);
ISP_WR(0X00);
TempData[3] = ISP_WR(0X00);
*/
//******************************************************The following is the data sent to LCD1602
for displayif((TempData[0] >>4) >9) Lcd_Out(Data,(TempData[0]>>4)+0x37);
else Lcd_Out(Data,(TempData[0]>>4)+0x30);
if((TempData[0] & 0x0f) >9) Lcd_Out(Data,(TempData[0] & 0x0f)+0x37);
else Lcd_Out(Data,(TempData[0] &0x0f)+0x30);
if((TempData[1] >>4) >9) Lcd_Out(Data,(TempData[1]>>4)+0x37);
else Lcd_Out(Data,(TempData[1]>>4)+0x30);
if ((TempData[1] & 0x0f) >9) Lcd_Out(Data,(TempData[1] & 0x0f)+0x37);
else Lcd_Out(Data,(TempData[1] &0x0f)+0x30);
if((TempData[2] >>4) >9) Lcd_Out(Data,(TempData[2]>>4)+0x37);
else Lcd_Out(Data,(TempData[2]>>4)+0x30);
if ((TempData[2] & 0x0f) >9) Lcd_Out(Data,(TempData[2] & 0x0f)+0x37);
else Lcd_Out(Data,(TempData[2] &0x0f)+0x30);
if((TempData[3] >>4) >9) Lcd_Out(Data,(TempData[3]>>4)+0x37);
else Lcd_Out(Data,(TempData[3]>>4)+0x30);
if ((TempData[3] & 0x0f) >9) Lcd_Out(Data,(TempData[3] & 0x0f)+0x37);
else Lcd_Out(Data,(TempData[3] &0x0f)+0x30);
while(1);
}
ISP_WR(uchar DATA)
{
uchar i,Rec_Data;
for(i=0;i<8;i++)
{
MOSI = DATA& 0x80;
DATA= DATA<<1;
Rec_Data = Rec_Data << 1;
if(MISO == 1) Rec_Data |= 0x01;
SCK = 1;
DELAY(1);
SCK = 0;
DELAY(1);
}
return(Rec_Data);
}
void DELAY(uint temp)
{
uint i,j;
for(i=0;i
}
Previous article:ds18b20 temperature measurement system based on single chip microcomputer
Next article:51 MCU controls K9K8G08U0C NAND Flash read and write program
Recommended ReadingLatest update time:2024-11-16 18:07
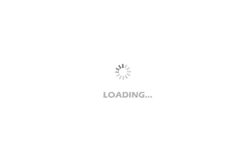
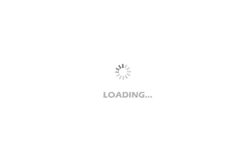
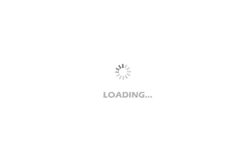
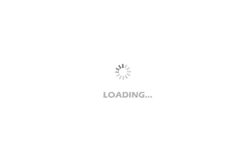
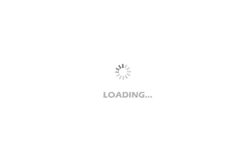
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Play with smart speaker design: Hold TI system solutions and look forward to unlimited possibilities in the future!
- Things you don’t know about Qorvo’s acquisition of Active-Semi!
- The problem of program running away
- Raspberry Pi Speaker Pirate Audio
- Will a short circuit between 3.3V and GND in a microcontroller system burn the microcontroller?
- Summary of DIY Bing Dwen Dwen works
- [Shanghai Hangxin ACM32F070 development board + touch function evaluation board evaluation] + development environment construction and download test
- Do you know the future star material in the RF field?
- There is no virtual serial port when the STM32F0discovery development board is plugged into the computer?
- Please advise everyone!