As long as you change the port yourself, you can use it, and the time is still relatively accurate.
#include#define uchar unsigned char #define uint unsigned int sbit RING1=P3^7; //P3^7 connects to buzzer sbit OPEN=P3^1; //LED lights up when alarm is on uchar idata buffer[8]={0,0,0,0,0,0,10,11}; //define two buffers, buffer is used to temporarily store and initialize the BCD code of the time during the process uchar code LED[]={0X3F,0X06,0X5B,0X4F,0X66,0X6D,0X7D,0X07,0X7F,0X6F,0X40,0X40}; //LED segment translation is stored in the program storage area uchar miao=0;fen=58;shi=10; //define global variables for miao,fen,shi for storing process time uchar _20ms; //define the global variable _20ms to count the number of interrupts, 50 for 1 second uchar hour2,minutes2,second2; //Alarm setting time storage area uchar hour1,minutes1,second1; //temporary storage area when modifying time bit hour,minutes,second; //Modify the corresponding bit flag bit add,dec; //addition and subtraction bit flags bit openring, cancelring; //Alarm on, off, cancel flag bit setring,settime; //Alarm setting, time setting flag bit ok; // confirm the variable void delay_1ms(uchar x) //delay 1ms { flying j; while(x--) { for(j=0;j<123;j++){;} } } void inital(void) //Timer initialization function { RING1=1; //Here, when RING is equal to 0, the buzzer sounds; TMOD=0x01; //Timer 0 works in mode 1, using 12M crystal oscillator, timing 20ms TH0=(65536-20000)/256; TL0=(65536-20000)%256; TR0=1; //TR0=1 starts the timer EA=1; //Open the general interrupt ET0=1; //Open timer interrupt } void timer0 (void) interrupt 1 using 1 //Timer 0 interrupt function { TH0=(65536-20000)/256; TL0=(65536-20000)%256; _20ms++; if(50==_20ms) //Judge whether it is one second { miao++; // one second has passed, seconds++ _20ms=0; if(miao==60) //Have you reached sixty seconds? { fen++; //It's time++ miao=0; if(fen==60) //Has it reached 60 minutes? { shi++; //When the time comes++ fen=0; if(shi==24) //Has 24 hours arrived? { shi=0; // clear to zero fen=0; miao=0; } } } } if(openring==1) //Is the alarm on? { if((shi==hour2)&&(fen==minutes2)&&(miao==second2)) //The alarm goes off when the set time comes RING1=0; if(cancelring==1) //Alarm off {RING1=1;cancelring=0;} } } void timebcd (uchar m,uchar f,uchar s) //Time change function, when the timing reaches 1 second, the time will be changed { buffer[0]=m%10; //shi, fen, miao are converted into BCD codes, mainly for the display of digital tubes buffer[1]=m/10; buffer[2]=f%10; buffer[3]=f/10; buffer[4]=s%10; buffer[5]=s/10; } void keyscan(void) //Key scan and determine key value function, this part is the core of various clock functions { uchar scode,recode,value; //Define row and column variables P2=0XF0; // Note the connection between keyboard and P2, 4*4 keyboard if ((P2 & 0XF0)!=0XF0) { delay_1ms(10); if ((P2&0XF0)!=0XF0) { scode=0xfe; while((scode & 0x10)!=0) { P2=scode; if ((P2&0XF0)!=0XF0) {recode=(P2 & 0XF0)|0X0f; value=((~scode)+(~recode)); switch(value) { case 0x11: hour=1;minutes=0;second=0;break;//, the key pressed is the function key for modification, and hour is the flag for modification case 0x21: hour=0;minutes=1;second=0;break;//Minutes, the key pressed is the function key for modifying minutes, and minutes is the flag for modifying minutes; case 0x41: hour=0;minutes=0;second=1;break;//Seconds are similar to above; case 0x81: add=1;dec=0;break;// 加 case 0x12: dec=1;add=0;break;//减 case 0x22: setring=1;settime=0;second1=second2;minutes1=minutes2;hour1= hour2;break;//Alarm setting, case 0x42: settime=1;setring=0;second1=miao;minutes1=fen;hour1=shi;break;//time setting case 0x82: setring=0;settime=0;break;//return case 0x14: ok=1;break;//OK case 0x24: openring=1;OPEN=0;break;//Open the alarm case 0x44: openring=0;OPEN=1;break;//Turn off the alarm case 0x84:cancelring=1;break;//Cancel the ringing default: break; } } else scode=(scode<<1)|0x01; } } } } Note: The keyboard values are encoded here. 12 keyboards are encoded. Pay special attention to the implementation methods of alarm setting and time setting. They share the function resettime, which is not difficult. void resettime (viod) { if(hour==1) { if (add==1) {hour1++;add=0;} if( hour1==24) hour1=0; if (dec==1) {hour1--;dec=0;} if (hour1==-1) hour1=23; } if(minutes==1) { if (add==1) {minutes1++;add=0;} if (minutes1==60) minutes1=0; if(dec==1) { minutes1--;dec=0;} if (minutes1==-1) minutes1=59; } if(second==1) { if (add==1) {second1++;add=0;} if(second1==60) second1=0; if (dec==1) {second1--;dec=0;} if(second1==-1) second1=59; } } display (void) //display function subroutine { flying j,n; for(n=0,j=0XFE;n<8;j=((j<<1)|0X01),n++) {P1=j; //P1 connects to digital tube position selection P0=LED[buffer[n]]; //P0 is connected to the digital tube segment selection delay_1ms(5); } } void main (void) { inital(); // Call timer initialization for(;;) { timebcd(miao,fen,shi); keyscan(); //Call key scan and confirm function if(settime==1) //When the key pressed is time setting { resettime(); timebcd ( second1, minutes1,hour1); if(ok==1) //When the OK button is pressed, it indicates that the reset time will overwrite the original time, so reset miao, fen, shi, and clear _20ms to zero { shi=hour1; fen=minutes1; miao=second1; _20ms=0; } } if(setring==1) { resettime(); timebcd( second1, minutes1,hour1); if(ok==1) { hour2=hour1; minutes2=minutes1; second2=second1; } } display(); //Call display function } }
Previous article:MCU decoding 315M pt2262 encoded c51 program
Next article:Design of electronic clock based on stc51 single chip microcomputer
Recommended ReadingLatest update time:2024-11-16 16:02
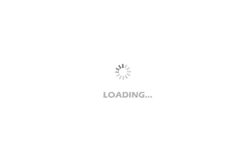
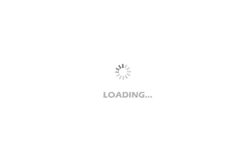
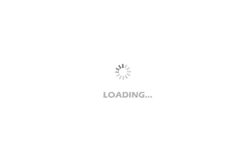
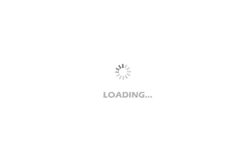
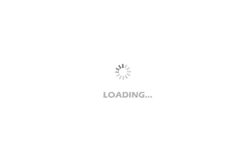
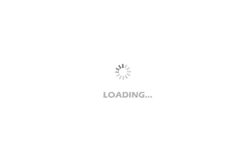
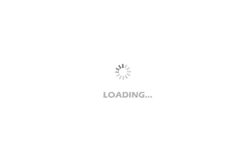
- Popular Resources
- Popular amplifiers
-
Wireless Sensor Network Technology and Applications (Edited by Mou Si, Yin Hong, and Su Xing)
-
Modern Electronic Technology Training Course (Edited by Yao Youfeng)
-
Modern arc welding power supply and its control
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- [SAMR21 New Gameplay] 10. Serial Port Communication-3
- Can you give me an example of how to read the development board data in real time via TCP protocol on PC?
- AC7801 chip serial port printing problem
- I would like to ask you about the closed-loop control of the motor encoder
- How to protect privacy using Bluetooth
- Tesla Robot Optimus Prime Conference Uncut 4K HD First Episode
- O-RAN development trends, reference architecture, and interoperability testing
- Qorvo PAC series highly integrated motor control chips and applications
- Five skills required for RF test engineers
- 【GD32L233C-START Review】Display driver for color OLED screen