The principle of the 6-bit electronic clock using AT89C2051 is shown in the figure below. As long as the hardware connection is correct, success is guaranteed. In addition, the SET button in the figure is used to calibrate the time. Press and hold for more than 2 seconds to enter the calibration time state and shift and exit. Quick touch is used to adjust the time value. The triode can be 9015. It is best to use a red common anode LED digital tube for a higher brightness. Because it is a scanning display mode, the abcdefg pins of each digital tube are connected in parallel by bus. Changing the 510 ohm resistor can change the display brightness.
Electronic clock source program
MCS51 MCU assembly program
;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;; AT89C2051 clock program; ;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;; The overflow period of timers T0 and T1 is 50MS, T0 is used for counting seconds, T1 is used for flashing when adjusting, ; P3.7 is the adjustment button, P1 port is the character output port, and a common anode display tube is used. ; ;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;; ORG 0000H ;Program execution start address LJMP START ;Jump to label START and execute ORG 0003H ;External interrupt 0 interrupt program entry RETI ;External interrupt 0 interrupt return ORG 000BH ;Timer T0 interrupt program entry LJMP INTT0 ;Jump to INTTO and execute ORG 0013H ;External interrupt 1 interrupt program entry RETI ;External interrupt 1 interrupt return ORG 001BH ;Timer T1 interrupt program entry LJMP INTT1 ;Jump to INTT1 and execute ORG 0023H ;Serial interrupt program entry address RETI ;Serial interrupt program return ;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;; ;; Main program;; ;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;; START: MOV R0,#70H ;Clear 70H-7AH, a total of 11 memory units MOV R7,#0BHCLEARDISP:MOV @R0,#00HINC R0DJNZ R7,CLEARDISPMOV 20H,#00H ;Clear 20H (for flag) MOV 7AH,#0AH ;Put in "extinguisher" data MOV TMOD,#11H ;Set T0 and T1 as 16-bit timers MOV TL0,#0B0H ;50MS timing initial value (for T0 timing) MOV TH0,#3CH ;50MS timing initial value MOV TL1,#0B0H ;50MS timing initial value (for T1 flashing timing) MOV TH1,#3CH ;50MS timing initial value SETB EA ;General interrupt open
[page]
SETB ET0 ; Enable T0 interrupt
SETB TR0 ; Start T0 timer
MOV R4,#14H; Initial value for 1 second timing (50MS×20)
START1: LCALL DISPLAY ;Call display subroutine
JNB P3.7,SETMM1; When P3.7 is 0, switch to time adjustment program
SJMP START1; jump back to START1 when P3.7 is 1
SETMM1: LJMP SETMM ; Go to time adjustment program SETMM
;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;
;; 1 second timing program;;
;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;
;T0 interrupt service routine
INTT0: PUSH ACC; Accumulator stack protection
PUSH PSW; status word stack protection
CLR ET0 ; turn off T0 interrupt enable
CLR TR0 ; turn off timer T0
MOV A,#0B7H ;Interrupt response time synchronization correction
ADD A,TL0; lower 8-bit initial value correction
MOV TL0,A ; Reload initial value (lower 8 bits correction value)
MOV A,#3CH ; High 8-bit initial value correction
ADDC A, TH0
MOV TH0,A ; Reload initial value (high 8-bit correction value)
SETB TR0 ; Start timer T0
DJNZ R4, OUTT0; 20 interrupts have not been received and the interrupt exit
ADDSS: MOV R4,#14H; 20 interrupts to (1 second) re-initialize
MOV R0,#71H ; point to the second timing unit (71H-72H)
ACALL ADD1; Call the add 1 program (add 1 second operation)
MOV A,R3; put the second data into A (R3 is a 2-digit decimal combination)
CLR C ; Clear carry flag
CJNE A,#60H,ADDMM
ADDMM: JC OUTT0; Interrupt and exit when less than 60 seconds
ACALL CLR0 ; Clear the second timer unit to 0 when it is greater than or equal to 60 seconds
MOV R0,#77H ; point to the sub-timing unit (76H-77H)
ACALL ADD1; add 1 minute to the timer unit
MOV A,R3; put the data into A
CLR C ; Clear carry flag
CJNE A,#60H,ADDHH
ADDHH: JC OUTT0; Interrupt and exit when less than 60 minutes
ACALL CLR0 ; Clear the timer unit to 0 if it is greater than or equal to 60 minutes
MOV
[page]
R0,#79H; points to the hour timing unit (78H-79H)
ACALL ADD1; hour timer unit plus 1 hour
MOV A,R3; when data is put into A
CLR C ; Clear carry flag
CJNE A,#24H,HOUR
HOUR: JC OUTT0; less than 24 hours interrupt exit
ACALL CLR0 ; greater than or equal to 24 hours hour timer unit cleared to 0
OUTT0: MOV 72H,76H; when the interrupt exits, move the minute and hour timing unit data to
MOV 73H,77H; enter the corresponding display unit
MOV 74H, 78H
MOV 75H,79H
POP PSW ; Restore status word (pop the stack)
POP ACC ; restore accumulator
SETB ET0 ; open T0 interrupt
RETI ;Interrupt return
;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;
;; Flashing timing program;;
;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;
;T1 interrupt service routine, used as a flashing indication for the adjustment unit when adjusting the time
INTT1: PUSH ACC; interrupt context protection
PUSH PSW
MOV TL1, #0B0H; install the initial value of timer T1
MOV TH1, #3CH
DJNZ R2, INTT1OUT; Exit interrupt if 0.3 seconds have not passed (50MS interrupt 6 times)
MOV R2,#06H ; Reinstall the initial value of 0.3 second timing
CPL 02H ;0.3 seconds timing to reverse the flashing mark
JB 02H,FLASH1; When 02H is 1, the display unit is "off"
MOV 72H,76H; Normal display when 02H is 0
MOV 73H,77H
MOV 74H, 78H
MOV 75H,79H
INTT1OUT:
POP PSW ;Recovery site
POP ACC
RETI ;Interrupt exit
FLASH1: JB 01H, FLASH2; When 01H is 1, the control will be turned off in the hour
MOV 72H,7AH; When 01H is 0, the "extinguishing character" data is put into the sub
MOV 73H,7AH; Display unit (72H-73H), will not display sub-data
MOV 74H, 78H
MOV 75H,79H
AJMP INTT1OUT ;Transfer to interrupt exit
FLASH2: MOV 72H,76H; When the 01H bit is 1, the "extinguishing symbol" data is placed in the hour register.
MOV 73H,77H; Display unit (74H-75H), hour data will not be displayed
MOV 74H,7AH
MOV 75H,7AH
AJMP INTT1OUT ;Transfer to interrupt exit
;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;
;; Add 1 subroutine;;
;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;
[page]
A,@R0; Get the current timing unit data to A
DEC R0 ; point to the previous address
SWAP A; the high four bits and low four bits of data in A are exchanged
ORL A,@R0; the data in the previous address is placed in the lower four bits of A
ADD A,#01H; A plus 1 operation
DA A ;Decimal Adjustment
MOV R3,A ; move to register R3
ANL A,#0FH ; high four bits become 0
MOV @R0,A ; put back to the previous address unit
MOV A,R3; retrieve the temporary data in R3
INC R0 ; points to the current address unit
SWAP A; the high four bits and low four bits of data in A are exchanged
ANL A,#0FH ; high four bits become 0
MOV @R0,A ; data is placed in the current address unit
RET ; Subroutine return
;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;
;; Clearing procedure;;
;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;
;Reset the timing unit
CLR0: CLR A; Clear accumulator
MOV @R0,A ; Clear current address unit
DEC R0 ; point to the previous address
MOV @R0,A ; clear the previous address unit to 0
RET ; Subroutine return
;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;
;; Clock adjustment procedure;;
;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;
;When the time adjustment button is pressed, enter this program
SETMM: CLR ET0 ; turn off timer T0 interrupt
CLR TR0 ; turn off timer T0
LCALL DL1S ;Call 1 second delay program
JB P3.7, CLOSEDIS; If the key is pressed for less than 1 second, the display will be turned off (power saving)
MOV R2,#06H; Enter the timing state and assign the initial value of the flashing timing
SETB ET1 ; Enable T1 interrupt
SETB TR1 ; Start timer T1
SET2: JNB P3.7, SET1; P3.7 port is 0 (key not released), wait
SETB 00H ;Key release, minute adjustment flashing flag set to 1
SET4: JB P3.7, SET3; Wait for the key to be pressed
LCALL DL05S ;When a key is pressed, the delay is 0.5 seconds
JNB P3.7,SETHH
[page]
; Press for more than 0.5 seconds to switch to hour status
MOV R0,#77H ; Press for less than 0.5 seconds plus 1 minute of operation
LCALL ADD1 ; call the add 1 subroutine
MOV A,R3; Get adjustment unit data
CLR C ; Clear carry flag
CJNE A,#60H,HHH ; Adjust unit data to compare with 60
HHH: JC SET4; adjust the unit data to less than 60 turns SET4 cycle
LCALL CLR0 ; Clear to 0 when the adjustment unit data is greater than or equal to 60
CLR C ; Clear carry flag
AJMP SET4 ; Jump to SET4 loop
CLOSEDIS:
SETB ET0 ; Power saving (LED not displayed) state. Open T0 interrupt
SETB TR0; Start T0 timer (turn on clock)
CLOSE: JB P3.7,CLOSE; No button is pressed, wait.
LCALL DISPLAY ;When a key is pressed, the display subroutine is called to delay and reduce jitter
JB P3.7, CLOSE; Interference return CLOSE wait
WAITH: JNB P3.7, WAITH; Wait for key release
LJMP START1 ; Return to the main program (LED data display is bright)
SETHH: CLR 00H; Clear the minute flashing mark (enter the hour adjustment state)
SETHH1: JNB P3.7,SET5 ; Wait for key release
SETB 01H ; Hour adjustment flag set to 1
SET6: JB P3.7, SET7; Wait for the button to be pressed
LCALL DL05S; 0.5 second delay when a key is pressed
JNB P3.7, SETOUT; Press for more than 0.5 seconds to exit time adjustment
MOV R0,#79H ; Press time less than 0.5 seconds plus 1 hour operation
LCALL ADD1 ; add 1 subroutine
MOV A,R3
CLR C
CJNE A,#24H,HOUU ; Timing unit data compared with 24
HOUU: JC SET6; less than 24 turns SET6 cycle
LCALL CLR0 ; Clear to 0 when greater than or equal to 24
AJMP SET6 ; Jump to SET6 loop
SETOUT: JNB P3.7, SETOUT1; Exit the program after adjusting the time. Wait for the key to be released
LCALL DISPLAY; delay and jitter reduction
JNB P3.7, SETOUT; if it is jitter, return to SETOUT and wait
CLR 01H ; Clear hour mark
CLR 00H ; Clearing and adjustment mark
[page]
CLR 02H ; Clear flashing flag
CLR TR1 ; Close timer T1
CLR ET1 ; turn off timer T1 interrupt
SETB TR0 ; Start timer T0
SETB ET0; Open timer T0 interrupt (timing starts)
LJMP START1 ; Jump back to the main program
SET1: LCALL DISPLAY; Call the display program (adjustment) when the key is released
AJMP SET2 ; Prevent no clock display when key is pressed
SET3: LCALL DISPLAY ; Clock display while waiting for the adjustment button
AJMP SET4
SET5: LCALL DISPLAY; Call the display program (adjust hours) when the key is released and waiting
AJMP SETHH1 ; Prevent no clock display when key is pressed
SET7: LCALL DISPLAY; Clock display when waiting for the hour adjustment button
AJMP-SET6
SETOUT1:LCALL DISPLAY ; Wait for key release when exiting clock adjustment
AJMP SETOUT ; Prevent no clock display when key is pressed
;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;
;; Show program;;
;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;
; Display data in 70H-75H unit, using six-digit LED common anode digital tube display, P1 port output segment code data, P3 port as
; Scan control, each LED digital tube lights up for 1MS and then cycles bit by bit.
DISPLAY:MOV R1,#70H ; point to the display data first address
MOV R5,#0FEH ; Scan control word initial value
PLAY: MOV A,R5 ; scan word and put it into A
MOV P3,A ; output from P3 port
MOV A,@R1; Get display data to A
MOV DPTR,#TAB ; Get the segment code table address
MOVC A,@A+DPTR; check the corresponding segment code of the displayed data
MOV P1,A; put the segment code into P1 port
LCALL DL1MS ;Display 1MS
INC R1 ; point to the next address
MOV A,R5; scan control word into A
JNB ACC.5,ENDOUT ;When ACC.5=0, the display ends once
RL A; data in A is circularly shifted to the left
MOV R5,A ; put back into R5
AJMP PLAY ; Jump back to the PLAY loop
ENDOUT: SETB P3.5; one display ends, P3 port reset
MOV
[page]
P1,#0FFH; P1 port reset
RET ; Subroutine return
TAB: DB 0C0H,0F9H,0A4H,0B0H,99H,92H,82H,0F8H,80H,90H,0FFH
;共阳段码表 "0""1""2" "3""4""5""6""7" "8""9""不亮"
;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;
;; Delay program;;
;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;
;1MS delay program, LED display program
DL1MS: MOV R6,#14H
DL1: MOV R7,#19H
DL2: DJNZ R7,DL2
DJNZ R6,DL1
RIGHT
;20MS delay program, using the display subroutine to improve the LED display flickering phenomenon
DS20MS: ACALL DISPLAY
ACALL DISPLAY
ACALL DISPLAY
RIGHT
; Delay program, used to judge the length of time the key is pressed
DL1S: LCALL DL05S
LCALL DL05S
RIGHT
DL05S: MOV R3,#20H; 8 milliseconds*32=0.196 seconds
DL05S1: LCALL DISPLAY
DJNZ R3,DL05S1
RIGHT
END
Previous article:Environmental Noise Measuring Instrument Based on 89C51 Single Chip Microcomputer
Next article:A brief analysis of the design method and application of rehabilitation equipment based on single chip microcomputer
Recommended ReadingLatest update time:2024-11-16 15:37
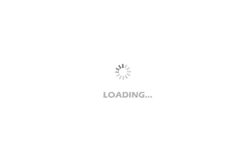
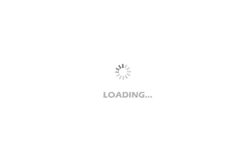
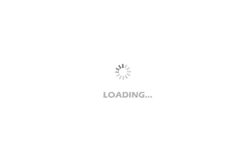
- Popular Resources
- Popular amplifiers
-
Learning MCU Technology from Scratch (Liu Jianqing)
-
Wireless doorbell alarm based on AT89C2051 single chip microcomputer
-
Infrared remote control LED electronic clock using real-time clock chip DS1302+AT89C2051
-
Infrared remote control LED electronic clock using real-time clock chip DS1302+AT89C2051.rar
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- TMS320DM642 Bootmode
- Single chip microcomputer drives 9013 transistor to realize infrared emission circuit diagram
- What are the characteristics of such a zero-pole system?
- SDR-Hackrf one
- SHT31 Review + Unboxing Test
- Matlab and Modelsim co-simulation error
- ST NUCLEO-G071RB evaluation application - Come and experience the new generation of entry-level 32-bit STM32, giving you the best cost-effective experience
- Classification of RFID readers and their advantages
- CC2640R2F supports Alibaba Cloud Link IoT platform
- Some understanding of MSP430 usage