C2000 built-in 12-bit ADC energy measurement solution
[Copy link]
This application note introduces a solution to implement software energy metering based on the C2000 core and the on-chip 12-bit ADC. C2000 is a 32-bit high-performance real-time microcontroller produced by Texas Instruments Semiconductor Co., Ltd. It is widely used in various real-time control fields related to power control, such as motor drive and digital power supply. The calculation of energy and power often exists as the input signal of feedback control in the above applications. Therefore, integrating software energy metering algorithms into the C2000 platform is the key to realizing various real-time control SOC solutions. This article introduces the specific method of implementing software metering algorithms on C2000 and the use of TI's software metering library based on C2000, and also gives the test results.
1 Introduction to C2000 Metrology Library
1.1 Application Background
The advocacy and promotion of energy conservation and environmental protection has led to the need for energy consumption statistics in most household appliances. For this huge application market, the purpose of this design is to use the application advantages of the C2000 chip in household appliance motor power supplies to expand the energy consumption statistics function at the minimum hardware cost.
The C2000 chip focuses on motor power control, and its excellent performance has been widely recognized by the industry. This design is a software testing platform built based on the C2000 Piccolo series chips, which implements a software library that meets the energy consumption measurement of smart homes, and enriches the application field of C2000 with the minimum hardware cost.
1.2 Introduction to hardware platform and software library
The reference design hardware platform uses C2000 Piccolo Entry Line Control Pad, which is a low-cost C2000 development board provided by TI. The circuit built on this platform can be used to develop a metering library, which allows users to gradually learn C2000 development and programming while implementing a set of energy consumption metering software.
This reference design implements a single-phase electric energy metering solution. The purpose of the reference design is to implement a complete single-phase metering library. It allows users to quickly build their own single-phase metering solutions based on this reference design. The code library provides active power, reactive power, apparent power, active energy and reactive energy. It can also measure loop current RMS, voltage RMS, power factor, frequency and other parameters to meet the needs of single-phase electric energy metering. The software supports digital calibration.
2 Part II Metering Circuit and Function Description
2.1 Metering Code Base
When calling the metering library, you only need to add all the files in the emeter directory to use the metering function. The file emeter-interface.h declares all external call interfaces for program calls to implement functions such as meter calibration and metering data reading. The algorithm library defaults to configuring the ADC conversion rate to 3200 points/second, the conversion data to 12 bits, and the data range to 0-4096, to achieve single-phase metering. The function adc_interrupt() implements the operation of the original conversion data, and measurement_callback() is responsible for further processing the pre-processed data in adc_interrupt(). Therefore, adc_interrupt(adc_raw[2]) can be called in each ADC interrupt, or multiple points can be cached and then this function can be called in a loop. The function input parameter adc_raw is the signal input, adc_raw[0] is the voltage conversion data, and adc_raw[1] is the current conversion data. The implementation process of adc_interrupt() and measurement_callback() is shown in Figure 1:
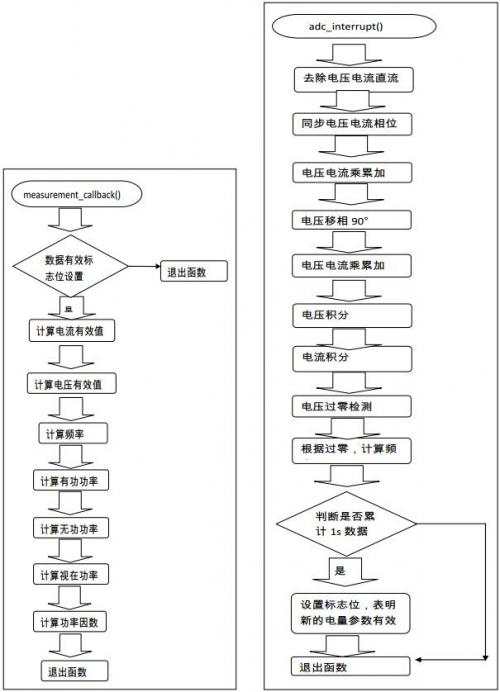
Figure 1 Flowchart of measurement software
The metering front-end circuit consists of a voltage signal conditioning circuit and a current signal conditioning circuit. The voltage signal conditioning circuit uses a resistor to divide the input power frequency signal, and sends it to the MCU ADC input pin after being buffered by an operational amplifier. The current transformer output current signal is converted into a voltage signal through a load resistor, and is amplified by an operational amplifier and sent to the MCU ADC input pin. The recommended circuit is shown in Figure 2:
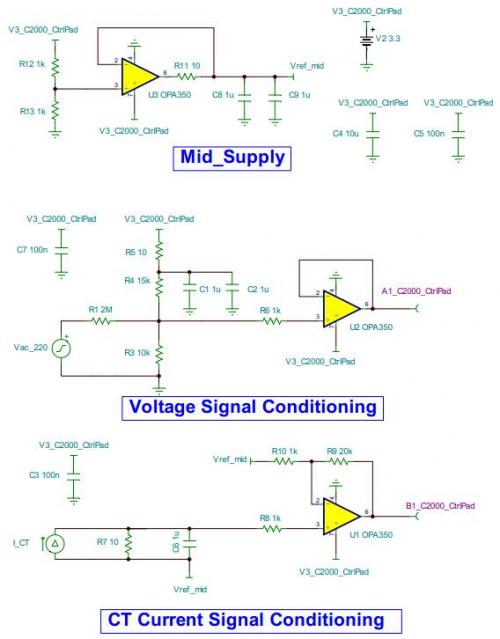
Figure 2 CT measurement front-end reference circuit
The voltage input analysis in the above figure is as follows:
The mains power is 220V AC input, and the input AC voltage is 220V*(6/(2000+6))=0.66V after 10k and 15k are connected in parallel and then connected in series with 2M resistors. The DC voltage is 3.3*10/25=1.32V. The total input voltage amplitude is 0.66*1.414+1.32=2.25V, and the input signal is within 3.3V. The current transformer input is 5A/2.5mA (depending on the specific model of the transformer), the sampling resistor voltage is 2.5*10=25mV, and the operational amplifier is amplified 20 times (the specific amplification factor is adjusted by R9, R10), the input AC signal is 25*20=0.5V, the total input current amplitude (10A) is 1*1.414+1.6=3.014V, and the input signal is within 3.3V. When using manganese copper resistors to obtain current signals, the current signal conditioning circuit is as follows:
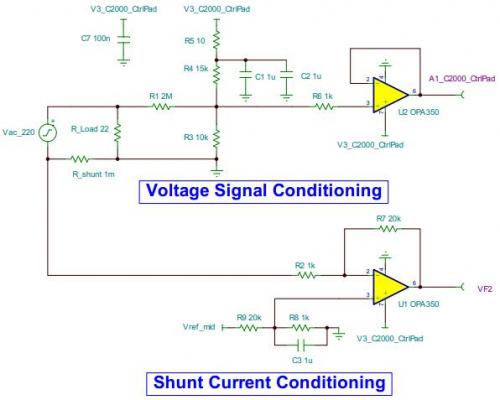
Figure 3 Manganese copper metering front-end reference circuit
2.2 Function Description
The main functions and macro definitions declared in the emeter-interface.h file are as follows:
int32_t get_parameter(int address)
Description: Get various measurement parameters from address address.
Input parameter: 16-bit integer address
Output parameter: Return the data at this address.
Interruptible and reentrant, can be used in interruption.
uint8_t set_parameter(int address, int32_t value)
Description: Set various calibration parameters and table parameters to address address.
Input parameter: 16-bit integer, address address 32-bit signed integer value value
Output parameter: Return 1 for successful operation, return 0 for failed operation.
Cannot be interrupted and reentrant, can not be used in interruption.
void measurement_setup (void)
Description: Initialize the code library, called at the beginning of power-on.
Input parameter: None
Output parameter: None
void measurement_callback (void)
Description: This function is called periodically (1s) to update real-time data.
Input parameter: None
Output parameter: None
Cannot be interrupted and reentrant, can not be used in interruption.
void adc_interrupt(int16_t * adc_raw)
Application Report
ZHCA501–Jan 2013
Introduction: You can call adc_interrupt(adc_raw[2]) in each ADC interrupt, or you can cache multiple points and then call this function in a loop.
The function input parameter adc_raw is the signal input, adc_raw[0] is the voltage conversion data, and adc_raw[1] is the current conversion data.
Input parameter: voltage and current input ADC value, adc_raw [0] voltage, adc_raw [1] current.
Output parameter: None
2.3 Introduction to addresses and corresponding functions
Considering the future modification or expansion of the code, the address is not specifically defined. Use an enumeration variable to let the compiler automatically generate it. The user can include this variable definition and use the enumeration address to set and get data.
The int32_t get_parameter (int address) function address enumeration variable is defined as follows :
Get real-time data structure
enum get_parameter_table
{
AFE_BASE_A = 0x0000,
AFE_GET_ACTIVE_POWER,
AFE_GET_REACTIVE_POWER,
AFE_GET_APPARENT_POWER,
AFE_GET_ACTIVE_ENERGY, AFE_GET_REACTIVE_ENERGY
,
AFE_GET_VRMS,
AFE_GET_IRMS , AFE_GET_POWER_FACTOR
,
AFE_GET _FREQUENCY,
AFE_GET_ENERGY_MODE, AFE_GET_STARTUP_I
,
AFE_GET_PULSE_CONST,
AFE_GET_POWER_GAINA0,
AFE_GET_PHASEOFFSET_A0,
AFE_GET_VGAINA,
AFE_GET_IGAINA,
AFE_GET_IOFFSETA,
};
The following parses each address:
AFE_GET_ACTIVE_POWER
Description: Active power
Unit: 10mW
AFE_GET_REACTIVE_POWER
Description: Reactive power
Unit: 10mVar
AFE_GET_APPARENT_POWER
Description: Apparent power
Unit: 10mVA
AFE_GET_VRMS
Description: Voltage RMS
Unit: 10mV
AFE_GET_IRMS
Description: Current RMS
Unit: 1mA
AFE_GET_POWER_FACTOR
Description: Power factor
Unit: 0.0001
AFE_GET_ACTIVE_ENERGY
Description: Maximum number of active energy pulses in the two circuits
Unit: Dependent on pulse constant
AFE_GET_REACTIVE_ENERGY
Description: Maximum number of reactive energy pulses in the two circuits
Unit: Dependent on pulse constant
AFE_GET_FREQUENCY
Description: System frequency
Unit: 0.01Hz
AFE_GET_POWER_GAINA0
Description: Power gain
AFE_GET_PHASEOFFSET_A0
Description: Voltage and current angle difference compensation
AFE_GET_VGAINA
Description: Voltage gain
AFE_GET_IGAINA Description
: Current gain
AFE_GET_IOFFSETA
Description: Current offset
Unit: 1mA
AFE_GET_POFFSETA
Description: Power offset
Unit: 10mW
AFE_GET_ENERGY_MODE
Description: Energy accumulation mode
Unit: 0 absolute value accumulation 1 positive energy accumulation
ZHCA501–Jan2013
Description: Startup current
Unit: 1mA
AFE_SET_PULSE_CONST
Description: Pulse constant
Get real-time data structure
enum set_parameter_table
{
AFE_SET_BASE = 0x00,
AFE_SET_POWER_GAINA0,
AFE_SET_PHASEOFFSET_A0,
AFE_SET_VGAINA,
AFE_SET_STARTUP_I,
AFE_SET_FREQUENCY,
AFE_SET_SAMPLES_10S,
AFE_SET_IGAINA,
AFE_SET_IOFFSETA,
AFE_SET_POFFSETA,
AFE_SET_ENERGY_MODE,
AFE_SET_PULSE_CONST,
AFE_SET_CAL_INIT,
};
AFE_SET_POWER_GAINA0
Description: Power gain
AFE_SET_PHASEOFFSET_A0
Description: Voltage and current angle difference compensation
AFE_SET_VGAINA
Description: Voltage gain
AFE_SET_STARTUP_I
Description: Startup current
Unit: 1mA
AFE_SET_IGAINA
Description: Current gain
AFE_SET_IOFFSETA
Description: Current offset
Unit: 1mA
AFE_SET_POFFSETA
Description: Power offset
Unit: 10mW
AFE_SET_PULSE_CONST
Description: Pulse constant
AFE_SET_CAL_INIT
Description: Calibration initialization
AFE_SET_CAL_END
Description: Calibration end
AFE_SET_SAVE_FUNC
Description: User set calibration data save function
3 Calibration Introduction
3.1 Calibration Register
The calibration register treats the live and neutral wires equally.
Power Gain Register

Voltage and current angle difference compensation register
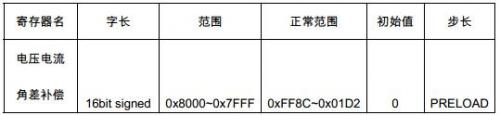
Active bias register

Current Gain Register

Current Bias Register

Voltage Gain Register

3.2 Calibration method
This metering library provides a special interface to calibrate the metering parameters. The calibration software runs on the PC and sets the parameters through serial communication. For specific operations, please refer to the sample code.
3.2.1 Power Gain and Offset Calibration
Power gain calibration can be performed in single point or two point. When single point calibration is performed, the power offset defaults to 0. When two point calibration is performed, the power offset is the intercept of the power relative to the 0 point. The calibration can be performed by pulse or by averaging the power values read multiple times. The two point calibration formula is as follows: (It is best to take 100%Ib and 5%Ib)
Power gain verification formula:

EH and EL are the power errors at high and low currents. (For example, the power error at 100%Ib and 5%Ib) NH2L is the ratio difference between high and low currents. (For example, NH2L=100%Ib/5%Ib=20)
Power bias calibration formula:

PGEN is the standard power value at low current.
The gain and offset of reactive power are similar to those of active power, and can be referred to as active power calibration. The following is an example of a single-point calibration to illustrate how to write calibration software:
1. EH = 0.5%
2. Original P1_GAINn=10000
3. New P1_GAINn+1=10000/(1+0.5%)=9950
3.2.2 Voltage and current angle difference calibration
The angle compensation of voltage and current is synchronized by software.
The angular difference compensation uses the following formula
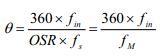
fM is the grid frequency. If fM=50Hz, fm=256×4096=1MHz, each step is 0.017°. After the power gain and bias are calibrated, the angle difference is calibrated. The steps are as follows:
1. At 100% Ib point, set the power factor to 0.5L.
2. Get the error E of the current pulse. Substitute E into the following formula:

The calculation formula is as follows:
1. E = 0.3%
2. Original P1_PHASEn = 6
3. New P1_PHASEn+1 =10+6=16
3.2.3 Voltage Gain Calibration
The voltage gain calibration steps are as follows:
1. Set the voltage to a fixed value, such as: 220V
2. The following formula

3. Set VRMS_FACTORn+1 to the power gain register. The steps are as follows:
1) VRMSGEN = 220V and VRMSmeasure = 219V
2) Original VRMS_FACTORn = 2000
3) New VRMS_FACTORn+1 = 2000*220/219 = 2009
3.2.4 Current Gain Calibration
This calibration is similar to voltage gain calibration.
3.3 Use of calibration software
3.3.1 Introduction to the calibration software interface
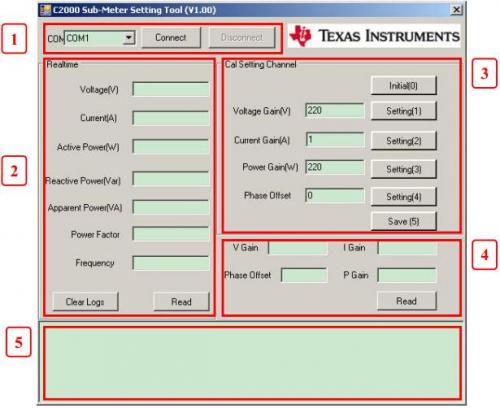
Figure 4 Calibration software interface
The calibration software interface of Figure 4 is divided into five modules: 1, serial port connection module; 2, real-time data module; 3, calibration data setting module; 4, calibration data readout module; 5, communication data module.
3.3.2 Use of real-time data module
The real-time data module reads the data measured by the meter in real time through the serial port and displays it in the calibration software. The real-time display data includes: Voltage (V) effective voltage value (unit: volt), Current (A) effective current (unit: ampere), Active Power (W) active power (unit: watt), Reactive Power (Var) reactive power (unit: var), Apparent Power (VA) apparent power (unit: volt-ampere), Power Factor power factor Frequency (Hz) frequency (unit: Hertz). Click the Read button to display the data measured by the meter in real time.
3.3.3 Use of the calibration data setting module
The calibration software currently only supports single-point calibration of Voltage Gain, Current Gain, Power Gain, and Phase Offset. The default voltage calibration point is 220V, the current calibration point is 1A, the active power calibration point is 220W, and the phase offset calibration point is 220V, 1A, 0.5L.
Calibration steps:
1. Use the calibration instrument to output a signal of 220V effective voltage, 1A effective current, and power factor 1.0, and send it to the voltage and current input terminals of the meter;
2. Enter the default effective voltage correction value 220 in Voltage Gain and click Setting;
3. Enter the default effective current correction value 1 in Current Gain and click Setting;
4. Enter the default active power correction value 220 in Power Gain and click Setting;
5. Use the calibration instrument to input the effective voltage, 1A effective current, and 0.5 power factor signal, and send it to the voltage and current input terminals of the meter;
6. Enter the offset value in Phase Offset. One unit corresponds to 0.02°. Enter a value, then read the new power factor through the real-time data window. Repeat the calibration until the power factor is close to 0.5.
7. After the calibration is completed, click Save to save the data. The meter will save the final calibration data to the MCU FLASH.
3.3.4 Use of calibration data readout module
The correction data readout module can read out the V Gain, I Gain, Phase Offset, and P Gain correction values stored in the meter FLASH through the serial port.
3.3.5 Communication data module usage
The communication data module displays the data sent and received by the serial port in real time. If the prompt "Time out!" appears during the setting process, it means that the connection has timed out. You should check the communication line connection and then resend the command. After the calibration data is set successfully, the communication data module prompts "Set successful!". By pressing the Clear Logs button, you can clear the historical data in the communication data window.
4 Conclusion
4.1 Hardware Platform
This solution built a test platform on the C2000F280270 Control Pad and performed accuracy tests on standard electric energy meter test equipment. Figure 5 shows the actual test prototype:
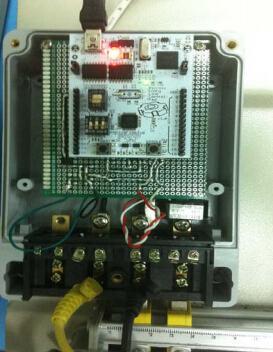
Figure 5 Test prototype
4.2 Software Resource Occupancy
The C2000 resources occupied by this solution are shown in the following table.

4.3 Test Data
The test accuracy of this solution is as follows:
Voltage Current Frequency
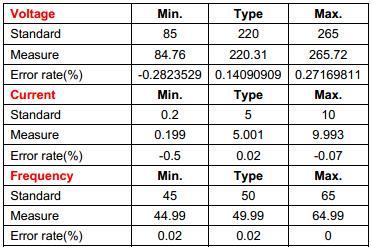
Active power and reactive power:
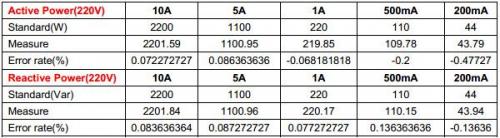
Power Factor:
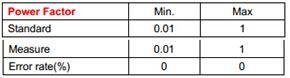
|