The seven-segment display can be used as a Verilog console in DE2 to output hexadecimal results.
Introduction
Usage environment: Quartus II 7.2 SP3 + DE2 (Cyclone II EP2C35F627C6)
Simply use the switch as a binary input and use an 8-digit seven-segment display to display the decimal result.
switch_seg10.v/Verilog
1/*
2 (c) OOMusou 2008
3
4 Filename : switch_seg10.v
5 Compiler : Quartus II 7.2 SP3
6 Description : Demonstrates how to use 8-bit 7-segment display decimals
7 Release : 07/20/2008 1.0
8 *
9 Module switch_seg10 (
input 10 [17:0] SW,
output 11 [6:0] HEX0,
output 12 [6:0] HEX1,
output 13 [6:0] HEX2,
output 14 [6:0] HEX3,
output 15 [6:0] HEX4,
output 16 [6:0] HEX5,
output 17 [6:0] HEX6,
output 18 [6:0] HEX7
19);
20
21 seg7_lut_8 u0 (
22 .i_dig (SW),
23 .o_seg0 (HEX0),
24 .o_seg1 (HEX1),
25 .o_seg2 (HEX2),
26 .o_seg3 (HEX3),
27 .o_seg4 (HEX4),
28 .o_seg5 (HEX5),
29 .o_seg6 (HEX6),
3 0 .o_seg7 (HEX7)
31);
32
33 endmodule
This is the top level module responsible for creating and instantiating seg7_lut8.
switch_lut.v/Verilog
1/*
2 (c) OOMusou 2008
3
4 Filename : switch_lut.v
5 Compiler : Quartus II 7.2 SP3
6 Description : Demonstrates how to use 8-bit 7-segment display with decimal
7 Release : 07/20/2008 1.0
8 *
9 module seg7_lut(
10 inputs [3:0] i_dig,
11 outputs reg [6:0] o_seg
12);
13
14 always @ (i_dig) begin
15 case (i_dig)
16 4 ' h1 : o_seg = 7 ' b111_1001; // ---t----
17 4 ' h2 : o_seg = 7 ' b010_0100; // | |
18 4 ' h3 : o_seg = 7 ' b011_0000; // Lt rt
19 4 ' h4
: o_seg = 7 ' b001_1001; // | | 20 4 ' h5 : o_seg = 7 ' b001_0010; // ---m----
21 4 ' h6
: o_seg = 7 ' b000_0010; // | seg = 7 ' b111_1000; // lb rubidium
23 4 ' h8 : o_seg = 7 ' b000_0000; // | |
24 4 ' h9 : o_seg = 7 ' b001_1000; // ---b----
25 4 ' ha : o_seg = 7 ' b000_1000;
26 4' hb: o_seg = 7' b000_0011;
27 4' hc: o_seg = 7' b100_0110;
28 4' hd: o_seg = 7' b010_0001;
29 4' he: o_seg = 7' b000_0110;
30 4' hf: o_seg = 7' b000_1110;
31 4' h0: o_seg = 7' b100_0000;
32 endcase
33 end
34
35 endmodule
36
This is a lookup table for a seven segment display.
switch_lut_8.v
1/*
2 (c) OOMusou 2008
3
4 Filename : switch_lut_8.v
5 Compiler : Quartus II 7.2 SP3
6 Description : Demonstrates how to use 8-bit 7-segment display decimal
7 Release : 07/20/2008 1.0
8 *
9 module seg7_lut_8 (
output 10 [6:0] o_seg0,
output 11 [6:0]
o_seg1, output 12 [6:0] o_seg2,
output 13 [6:0] o_seg3,
output 14 [6:0] o_seg4,
output 15 [6:0] o_seg5, output
16 [6:0] o_seg6,
output 17 [6:0] o_seg7,
input 18 [31:0] i_dig
19);
20
21 seg7_lut u0 (
22 .i_dig (i_dig%10),
23 .o_seg (o_seg0),
24);
25
26 seg7_lut u1 (
27 .i_dig ((i_dig or 10)%10),
28 .o_seg (o_seg1)
29);
30
31 seg7_lu t u2
(
32 .i_dig ((i_dig/100) %10),
33 .o_seg (o_seg2)
34);
35
36 seg7_lut u3 (
37 .i_dig ((i_dig/1000) %10),
38 .o_seg (o_seg3)
39);
40
41 seg7_lut u4
(
42 .i_dig ((i_dig/10000) %10)
,
43 .o_seg (o_seg4)
44);
45
46 seg7_lut u5
( 47 .i_dig ((i_dig/100000) %10),
48 .o_seg (o_seg5)
49);
0
51 seg7_lut u6
(
52 .i_dig ((i_dig/1000000) %10),
53 .o_seg (o_seg6)
54);
55
56 seg7_lut u7 (
57 .i_dig ((i_dig/10000000) %10),
58 .o_seg (o_seg7)
59);
60
61 endmodule
Compared with (Original) How to display 8-digit numbers in binary on a seven-segment display? (SOC) (Verilog) (DE2) and (Original) How to display 8-digit numbers in hexadecimal on a seven-segment display? (SOC) (Verilog) (DE2)
, the key difference lies in switch_lut_8.v. Since the switch input is binary, displaying binary means sending each bit to a seven-segment display, and displaying hexadecimal means sending every 4 bits to a seven-segment display, but what about decimal?
For example, if the switch input is 378 in decimal, if you want to get the tens digit 7, you can use 378/10 = 37, and then 37% 10 = 7. In this way, you can get the tens digit 7. The same goes for the other digits.
Previous article:Reshaping Ultrasound Imaging System Design
Next article:Implementation of high-speed intermediate frequency sampling signal processing platform based on FPGA+DSP
Recommended ReadingLatest update time:2024-11-16 20:53
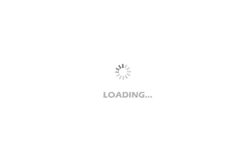
- Popular Resources
- Popular amplifiers
-
MATLAB and FPGA implementation of wireless communication
-
Learn CPLD and Verilog HDL programming technology from scratch_Let beginners easily learn CPLD system design technology through practical methods
-
Based on Altera FPGA devices & Verilog HDL language
-
Become a design expert easily Verilog HDL examples and detailed explanations with source code
- Huawei's Strategic Department Director Gai Gang: The cumulative installed base of open source Euler operating system exceeds 10 million sets
- Analysis of the application of several common contact parts in high-voltage connectors of new energy vehicles
- Wiring harness durability test and contact voltage drop test method
- Sn-doped CuO nanostructure-based ethanol gas sensor for real-time drunk driving detection in vehicles
- Design considerations for automotive battery wiring harness
- Do you know all the various motors commonly used in automotive electronics?
- What are the functions of the Internet of Vehicles? What are the uses and benefits of the Internet of Vehicles?
- Power Inverter - A critical safety system for electric vehicles
- Analysis of the information security mechanism of AUTOSAR, the automotive embedded software framework
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- DIY a USB hub shell
- STM8S001J3 uses HalfDuplex mode and uses IO mapping and cannot receive data.
- Analysis of the Causes of Operational Amplifier Oscillation and Self-excitation
- (Bonus 14) GD32L233 Review - Driving Segment Code LCD
- China's chip self-sufficiency rate was 30% last year, and the goal is to reach 70% by 2025 (attached with representative companies in 70 sub-sectors of domestic chips)
- 【New Year's Taste Competition】New Year's Eve Reunion Dinner and My Wedding
- Getting Started with the TI AWR1642BOOST-ODS EVM Development Board
- [STM32WB55 Review] A brief analysis of the execution process of the BLE demo program
- Positioning, positioning, positioning: the road to ultra-wideband
- 35-year-old programmer trades Luna and loses tens of millions of assets in three days