Both the tq2440 and mini2440 are connected with EEPROMs, and their function is just to test whether the I2C bus is available.
The EEPROM model on mini2440 is AT24C08, and on tq2440 it is AT24C02A.
They have different capacities and address lines.
The S3C2440A RISC microprocessor can support a multi-master IIC bus serial interface. A serial data line (SDA) and a dedicated clock line (SCL) are connected between the bus master and the peripherals of the IIC bus. Both SDA and SCL lines are bidirectional. Both are connected to GPE14 (SCL) GPE15 (SDA).
To control multi-master IIC bus operation, values must be written to the following registers:
– Multi-master IIC bus control register, IICCON
– Multi-master IIC bus control/status register, IICSTAT
– Multi-master IIC bus Tx/Rx data shift register, IICDS
– Multi-master IIC bus address register, IICADD
Because we only use s3c2440 as the master device, and there is only one master device on the IIC bus of the system, the address register IICADD used to set the slave device address does not need to be configured.
The IIC bus interface of S3C2440A has 4 working modes:
– Host sending mode
– Host receiving mode
– Slave Transmit Mode
– Slave Receiver Mode
Start and Stop Conditions
When the IIC bus interface is inactive, it is usually in slave mode.
In other words, the interface should be in slave mode before a start condition is detected on the SDA line (a high-to-low transition of SDA while the SCL clock signal is high)
To initiate a start condition). When the interface status is changed to master mode, it can start sending data to SDA and generate SCL signal.
The start condition can transfer 1 byte of serial data to the SDA line, and the stop condition can end the
Transmission of data.
The stop condition is a low to high transition of the SDA line while SCL is high. The start and stop conditions are always generated by the master. The IIC bus becomes busy when a start condition is generated. A stop condition will make the IIC bus free.
When the host initiates a start condition, it should send a slave address to notify the slave device.
The 1-byte address field consists of a 7-bit address and a 1-bit transfer direction flag (read or write).
Assume bit [8] is 0, which indicates a write operation.
Assume that bit [8] is 1, which indicates a request to read data (receive operation).
The master will complete the transfer operation by sending a stop condition. Assuming the master wishes to continue sending data to the bus, it should generate another start condition at the same slave address. This allows various formats of read and write operations to be performed.
Note that there are several SCL clocks between the start and stop conditions, which are used to send data.
Transmission data format
Each byte placed on the SDA line should be 8 bits in length.
Each transfer byte can be sent indefinitely. The first byte following the start condition should include the address field. When the IIC bus operates in master mode, it can be sent by the master.
Send the address field.
Each byte should be followed by an acknowledge (ACK) bit.
Serial data and addresses are always sent MSB first.
Correction to the above picture: The legend here should be reversed --- the grey box represents from slave to master. It seems that the person who translated the document was not careful enough.
There are 4 working modes mentioned above. Here we only use s3c2440 as the master device of IIC bus. Therefore, we only introduce the first two operating modes.
First, let's look at the master device sending flow chart:
First, configure the IIC mode, and then write the slave device address into the receive and transmit data shift register IICDS. Then write 0xF0 into the control status register IICSTAT, and then wait for the slave device to send a response signal. If you want to continue sending data, then after receiving the response signal, write the data to be sent into the register IICDS, clear the interrupt flag, and wait for the response signal again. If you don't want to send data anymore, then
Write 0x90 into register IICSTAT, clear the interrupt flag and wait for the stop condition, and the master device has completed the transmission.
The code is as follows:
//AT24C02A page write, when sizeofdate is 1. It is byte write
//The input parameters are the device memory address, IIC data cache array and the number of data to be written.
void __attribute__((optimize("O0"))) wr24c02a(UINT8 wordAddr,UINT8 *buffer,UINT32 sizeofdate )
{
int i;
i2cflag =1; //Response flag
rIICDS = devAddr;
rIICSTAT = 0xf0; //Master device sending mode
rIICCON &= ~0x10; // Clear interrupt flag
while(i2cflag == 1) //Wait for the slave device to respond,
OSTimeDly(2); //Once entering the IIC interrupt, you can jump out of the dead loop
i2cflag = 1;
rIICDS = wordAddr; //Write to the memory address of the slave device
rIICCON &= ~0x10;
while(i2cflag)
OSTimeDly(2);
//Continuously write data
for(i=0;i
{
i2cflag = 1;
rIICDS = *(buffer+i);
rIICCON &= ~0x10;
while(i2cflag)
OSTimeDly(2);
}
rIICSTAT = 0xd0; //Issue a stop command to end the communication
rIICCON = 0xe0; //Prepare for the next IIC communication
OSTimeDly(100);
}
There are two points that need to be explained above. The first point is that the initialization of rIICCON must be assigned a value after rIICSTAT.
The second point is because this is executed in a multi-tasking environment, and the OSTimeDly following while has a delay, that is, assuming that OSTimeDly (2) is delayed by 10ms, and the interrupt occurs at 1ms.
It still takes 10ms to continue running. You can consider using a semaphore instead. In this way, once an interrupt occurs, it will continue running immediately after coming out of the interrupt.
Then there is the main device receiving flow chart:
First, configure the IIC mode, then write the slave device address into the receive and transmit data shift register IICDS, and then write 0xB0 into the control status register IICSTAT, and then wait for the slave device to send a response signal. If you want to receive data, then after the response signal, read the register IICDS and clear the interrupt flag; if you do not want to receive data, then write 0x90 to the register IICSTAT. After clearing the interrupt flag and waiting for the stop condition, the master device has completed the reception.
//AT24C02A serial read, when sizeofdate is 1, it is random read
//The input parameters are the device memory address, IIC data cache array and the number of data to be read.
void rd24c02a(UINT8 wordAddr,UINT8 *buffer,UINT32 sizeofdate)
{
int i;
unsigned char temp;
i2cflag =1;
rIICDS = devAddr; //
rIICCON &= ~0x10; // Clear interrupt flag
rIICSTAT = 0xf0; //Master device sending mode
while(i2cflag)
OSTimeDly(2);
i2cflag = 1;
rIICDS = wordAddr;
rIICCON &= ~0x10;
while(i2cflag)
OSTimeDly(2);
i2cflag = 1;
rIICDS = devAddr; //
rIICCON &= ~0x10;
rIICSTAT = 0xb0; //Master device receiving mode
while (i2cflag)
OSTimeDly(2);
i2cflag = 1;
temp = rIICDS; //Read slave device address
rIICCON &= ~0x10;
while(i2cflag)
OSTimeDly(2);
//Continuous reading
for(i=0;i
{
i2cflag = 1;
if(i==sizeofdate-1) //Assume it is the last data
rIICCON &= ~0x80; //No longer respond
*(buffer+i) = rIICDS;
rIICCON &= ~0x10;
while(i2cflag)
OSTimeDly(2);
}
rIICSTAT = 0x90; //End the communication
rIICCON = 0xe0; //
OSTimeDly(100);
}
The IIC clock source of s3c2440 is PCLK. When the system PCLK is 50MHz and the slave device requires a maximum of 100kHz, the IICCON register needs to be configured as shown in the following figure:
When the system is initialized, the iic register is configured as follows:
void init_i2c(void)
{
rGPEUP |= 0xc000; //Pull-up disable
rGPECON |= 0xa0000000; //GPE15:IICSDA, GPE14:IICSCL
rINTMSK &= ~(1<<27); /// enable i2c
rIICCON = 0xe0; //Set the IIC clock frequency, enable the response signal, and enable the interrupt
rIICSTAT = 0x10;
pIRQ_IIC = (UINT32)i2c_isr;
}
In i2c_isr, just set i2cflag to 0:
void i2c_isr(void)
{
i2cflag = 0;
}
The detailed code can be cloned from my github.
Previous article:Analysis of start.S of ARM920T in u-boot
Next article:s3c2440 network card interface expansion DM9000
Recommended ReadingLatest update time:2024-11-16 13:54
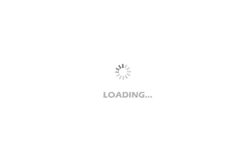
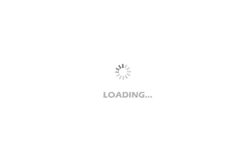
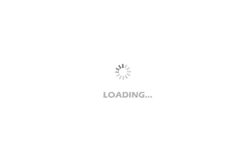
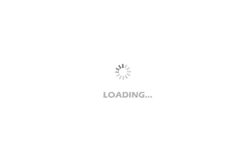
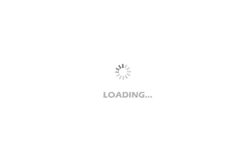
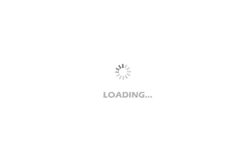
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- [Review of Arteli Development Board AT32F421] 2. Use of peripheral ERTC
- [Review of SGP40] + Unboxing & Basic Use
- How to choose between different chips in TI's Sub-1GHz product line?
- CircuitPython Electronic Chain Bracelet
- "C Programming Magic Book: Based on C11 Standard"
- [Project Source Code] 3 methods to display state machine names in Modelsim simulation based on FPGA
- Temperature sensor + I2C + serial port + PC host (pyserial) example
- MSP430G2553 internal ADC principle and routine description
- Types of PCB substrates
- What is the difference between an AD schematic and a schematic library?