ADC driver design
ADC: Analog-to-digital converter.
A device that converts analog signals into digital signals;
DAC: Digital to Analog Converter.
A device that converts digital signals into analog signals.
Steps to convert analog signal into digital signal:
1. Get the value;
2. Quantification;
3. Coding;
S3C2440ADC
The S3C2440 chip has 8 A/D conversion channels AIN0~AIN7, but there is only one converter.
The conversion precision is 10 bits, so the minimum converted value will be close to 0 and the maximum converted value will be close to 1024.
The maximum conversion rate can reach 500KSPS (5000 thousand samples per second) at a conversion clock of 2.5MHZ.
In common designs, such as the mini2440 development board, AIN4, AIN5, AIN6, and AIN7 are generally used as the YM, YP, XM, and XP channels of four-wire resistive touch; the remaining AIN0~3 are brought out, of which AIN0 is directly connected to an adjustable resistor W1.
Teacher: AIN4, AIN5, AIN6, and AIN7 are for touch screens;
AIN0 is used for adjustable resistance
(So that the voltage can be converted as an analog signal)
ADC driving process: 1. Initialization à 2. Start à 3. Conversion end à 4. Read conversion value
1. ADC initialization
A. Select the conversion channel - B. Set the conversion frequency
How to determine whether the conversion has started? Use a while loop to check whether ENABLE_START has become 0
How to determine whether the conversion is complete? Keep checking whether ECFLG is 1
Read conversion value
#define GLOBAL_CLK 1
#include
#include
#include "def.h"
#include "option.h"
#include "2440addr.h"
#include "2440lib.h"
#include "2440slib.h"
#include "mmu.h"
#include "profile.h"
#include "memtest.h"
#define ADC_FREQ 2500000
//#define ADC_FREQ 1250000
volatile U32 preScaler;
void adc_init(void);
int ReadAdc(int channel);
static void cal_cpu_bus_clk(void);
void Set_Clk(void);
void beep_init(void);
void beep_run(void);
/******************************************************
Function name: delay
Parameter : times
Description: Delay function
Return : void
Argument : void
Autor & date : Daniel
*************************************************** /
void delay(int times)
{
int i,j;
for(i=0;i
for(j=0;j<400;j++);
}
/******************************************************
Function name: Main
Parameter : void
Description: Main function
Return : void
Argument : void
Autor & date : Daniel
*************************************************** /
void Main(void)
{
int a0=0,tmp;
int Scom=0;
Set_Clk();
Uart_Init(0,115200);
Uart_Select(Scom);
adc_init();
while(1)
{
a0=ReadAdc(0);
Uart_Printf( "AIN0: %04dn", a0);
delay(1000);
}
}
/******************************************************
Function name: adc_init()
Parameter : int channel
Description: adc initialization
Return : void
Argument : void
Autor & date : Daniel
*************************************************** /
void adc_init(void)
{
int channel=0; //AIN0, corresponding to the adjustable resistor W1 on the development board
preScaler = ADC_FREQ;
Uart_Printf("ADC conv,freq. = %dHzn",preScaler);
preScaler = 50000000/ADC_FREQ - 1; //PCLK=50M We want to get ADC_FREQ=2500000
Uart_Printf("PRSCVL=PCLK/ADC_FREQ - 1=%dn",preScaler);
/*AD conversion frequency setting, maximum frequency is 2.5MHz*/
rADCCON = (1<<14)|(preScaler<<6)|(channel<<3); //setup channel 1<<14 enable prescaler (preScaler<<6) prescaler value channel<<3 analog channel selection
delay(1000);
}
/******************************************************
Function name: ReadAdc(int channel)
Parameter : int channel
Description: Get the value after AD conversion
Return : int
Argument : void
Autor & date : Daniel
*************************************************** /
int ReadAdc(int channel)
{
/*Start AD conversion*/
rADCCON |= 0x01; //start ADC
while(rADCCON & 0x1); //check if Enable_start is low
while(!(rADCCON & 0x8000)); //check if EC(End of Conversion) flag is high to determine whether the conversion is finished
return ( (int)rADCDAT0 & 0x3ff ); //Read the converted value
}
/******************************************************
Function name: Set_Clk()
Parameter : void
Description: Set the CPU clock frequency
Return : void
Argument : void
Autor & date : Daniel
*************************************************** /
void Set_Clk(void)
{
int i;
U8 key;
U32 mpll_val = 0 ;
i = 2; //don't use 100M!
//boot_params.cpu_clk.val = 3;
switch ( i ) {
case 0: //200
key = 12;
mpll_val = (92<<12)|(4<<4)|(1);
break;
case 1: //300
key = 13;
mpll_val = (67<<12)|(1<<4)|(1);
break;
case 2: //400
key = 14;
mpll_val = (92<<12)|(1<<4)|(1);
break;
case 3: //440!!!
key = 14;
mpll_val = (102<<12)|(1<<4)|(1);
break;
default:
key = 14;
mpll_val = (92<<12)|(1<<4)|(1);
break;
}
//init FCLK=400M, so change MPLL first
ChangeMPllValue((mpll_val>>12)&0xff, (mpll_val>>4)&0x3f, mpll_val&3); //set the register--rMPLLCON
ChangeClockDivider(key, 12); //the result of rCLKDIVN [0:1:0:1] 3-0 bit
cal_cpu_bus_clk(); //HCLK=100M PCLK=50M
}
/******************************************************
Function name: cal_cpu_bus_clk
Parameter : void
Description: Set the frequency of PCLKHCLKFCLK
Return : void
Argument : void
Autor & date : Daniel
*************************************************** /
static void cal_cpu_bus_clk(void)
{
static U32 cpu_freq;
static U32 UPLL;
U32 val;
U8 m, p, s;
val = rMPLLCON;
m = (val>>12)&0xff;
p = (val>>4)&0x3f;
s = val&3;
//(m+8)*FIN*2 Do not exceed 32 digits!
FCLK = ((m+8)*(FIN/100)*2)/((p+2)*(1<
val = rCLKDIVN;
m = (val>>1)&3;
p = val&1;
val = rCAMDIVN;
s = val>>8;
switch (m) {
case 0:
HCLK = FCLK;
break;
case 1:
HCLK = FCLK>>1;
break;
case 2:
if(s&2)
HCLK = FCLK>>3;
else
HCLK = FCLK>>2;
break;
case 3:
if(s&1)
HCLK = FCLK/6;
else
HCLK = FCLK/3;
break;
}
if(p)
PCLK = HCLK>>1;
else
PCLK = HCLK;
if(s&0x10)
cpu_freq = HCLK;
else
cpu_freq = FCLK;
val = rUPLLCON;
m = (val>>12)&0xff;
p = (val>>4)&0x3f;
s = val&3;
UPLL = ((m+8)*FIN)/((p+2)*(1<
UCLK = (rCLKDIVN&8)?(UPLL>>1):UPLL;
}
Previous article:s3c2440 bare metal development and debugging environment (MDK4.72, Jlink v8, mini2440)
Next article:Porting openssh to embedded ARM development board
Recommended ReadingLatest update time:2024-11-16 09:37
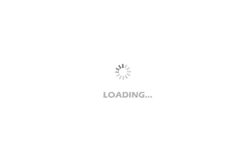
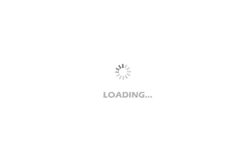
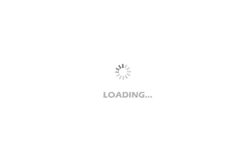
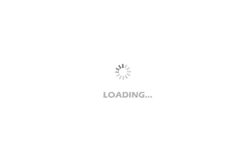
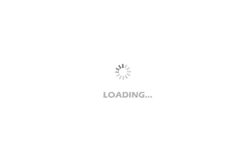
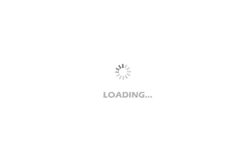
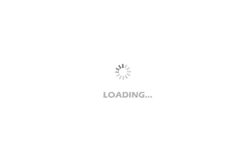
- Popular Resources
- Popular amplifiers
-
Practical Deep Neural Networks on Mobile Platforms: Principles, Architecture, and Optimization
-
ARM Embedded System Principles and Applications (Wang Xiaofeng)
-
ARM Cortex-M4+Wi-Fi MCU Application Guide (Embedded Technology and Application Series) (Guo Shujun)
-
osk5912 evaluation board example source code
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- 3-wire spi (cs clk sda), LCD driver for HX8369
- 8 DDR3 surface-to-bottom mounting solution
- Where are the options for PCB material/PCB copper thickness/PCB spray color/milling edge in the Gerber file of Altium Designer?
- Basic knowledge of SCI serial port programming of TMS320F28035
- 【BLE 5.3 wireless MCU CH582】14. BLE serial port transparent transmission test
- Use 1117 solar panels
- Implementation of asynchronous serial port.zip
- 【Badminton Training Monitor Project】-- Additional function test of badminton training monitoring module
- In the 28035EPWM module up-down counting mode, how can I generate ADC trigger pulses at CMPA when both increasing and decreasing?
- DSP startup loading principle