1 Experimental Phenomenon
2 Experimental Principle
The working process of ADC0809: First input the 3-bit address and make ALE=1, and input the address into the address latch. This address is decoded to select one of the 8 analog inputs to the comparator. The rising edge of START will gradually approach the register reset. The falling edge starts the ADC conversion, and then the EOC output signal becomes low, indicating that the conversion is in progress. Until the ADC conversion is completed, EOC becomes a high level, indicating that the ADC conversion is over, and the result data has been stored in the latch. This signal can be used as an interrupt request. When the OE input is high, the output tri-state gate is opened, and the digital value of the conversion result is output to the data bus. Common software control methods for AD conversion are:
(1) Program query method
First, the microprocessor sends a start signal to the A/D converter, then reads the conversion end signal, and queries whether the conversion is completed. If it is completed, read the data, otherwise continue to query until the conversion is completed. This method is simple and reliable, but the query takes up CPU time and is less efficient.
(2) Delayed waiting method
After the microprocessor sends a start signal to the A/D converter, it delays according to the conversion time of the A/D converter. Generally, the delay time is slightly longer than the conversion time of the A/D converter. When the delay ends, the data is read in. This method is simple and does not occupy the query port, but it occupies CPU time and has low efficiency. It is suitable for situations where the microprocessor has few processing tasks.
(3) Interrupt mode
After the microprocessor starts the A/D conversion, it can handle other things. After the A/D conversion is completed, it will actively send an interrupt request signal to the CPU. The CPU responds to the interrupt and then reads the conversion result. The microprocessor can work in parallel with the A/D converter, which improves efficiency.
3 System Design
4 Hardware Design
(1) ADC0809 cannot be simulated normally in Proteus software, but ADC0808 can be simulated normally, so ADC0808 is used instead;
(2) The CLOCK of ADC0809 is typically 500KHz, which is obtained by using the ALE (2MHz) of the microcontroller through a four-frequency division circuit;
(3) Output of ADC0809, mainly the order of high and low bits;
(4) EOC, connect the microcontroller external interrupt EX0, P3.2, the trigger mode is falling edge trigger, and add an inverter between EOC and P3.2.
(5) The ALE pin does not have a 6-frequency pulse output. Setting method: Reset the microcontroller. In the Advanced Properties option, select Simulate program Fetches and select YES.
5 Software Design
5.1 Main Program
#include "DisplaySmg.h"
#include "ADC0809.h"
#include "Timer0.h"
int adc_result_show = 0;
unsigned char adc_flag = 1; // Flag signal to start ADC conversion
void disp_num() //Put the data to be displayed into the buffer
{
LedBuf[0]= 23; //Thousands, not displayed
LedBuf[1]= adc_result_show/100; //hundreds place
LedBuf[2]= adc_result_show/10%10; //ten digit
LedBuf[3]= adc_result_show%10; //unit digit
}
void main()
{
Timer0_Init(); //Timer counter T0 initialization
EX0_Init(); //External interrupt initialization
EA=1; //Interrupt main switch
DotDig1=1; //light up the decimal point of the second digital tube
while(1)
{
if(adc_flag==1) //Start ADC conversion every 500ms
{
adc_flag = 0;
Start_ADC0809(); //Filtering, cannot sample only once, multiple sampling digital filtering should be performed
adc_result_show = adc_result*1.0*100*5/255; //Data conversion processing (linear scale conversion)
disp_num(); //Display data
}
}
}
void Timer0_ISR(void) interrupt 1
{
static unsigned int timer0cnt=0;
TR0=0; //Turn off the timer
timer0cnt++;
if(timer0cnt>=500)
{
timer0cnt = 0;
adc_flag = 1; //500ms flag signal
}
DisplaySmg(); //Refresh the digital tube display function every 1ms
TL0 = 0x66; //Set the initial timing value, timing 1ms
TH0 = 0xFC; //Set the initial timing value, timing 1ms
TR0=1; //Turn on the timer
}
5.2 ACD0809 analog-to-digital conversion module
#ifndef __ADC0809_H__
#define __ADC0809_H__
#include
#define ADC_DATA P1
sbit ADDR_A = P3^7;
sbit ADDR_B = P3^6;
sbit ADDR_C = P3^5;
sbit START = P3^4;
sbit EOC = P3^2;
sbit OE = P3^3;
extern unsigned char adc_result;
void Start_ADC0809();
void EX0_Init();
#endif
#include "ADC0809.h"
unsigned char adc_result;
void Start_ADC0809()
{
OE = 0; //Data output enable signal, high level is valid
START = 0; //ADC conversion start signal, high level is valid, connected with ALE (address latch enable signal) in the circuit
ADDR_A = 1; //3-bit address input line
ADDR_B = 1; //Used to select one of the 8 analog inputs
ADDR_C = 0;
START = 1; //Rising edge, clear the register inside the ADC
START = 0; //Generate a certain pulse, Typ = 100ns, the falling edge starts AD conversion
}
void EX0_Init() //External interrupt initialization
{
IT0 = 1; //External interrupt 0, set to falling edge trigger
EX0 = 1; //External interrupt 0 switch
}
void EX0_ISR() interrupt 0
{
OE = 1;
adc_result = ADC_DATA;
OE = 0;
}
5.3 Digital tube dynamic display module
#ifndef __DisplaySmg_H__
#define __DisplaySmg_H__
#include
#define GPIO_SEG P0 //Segment select port
#define GPIO_SEL P2 //bit select port
extern unsigned char LedBuf[]; //external variable declaration
extern unsigned char DotDig0,DotDig1,DotDig2,DotDig3;
void DisplaySmg(void);
#endif
#include "DisplaySmg.h"
unsigned char code LedData[]={ //segment code table, character, serial number of common cathode digital tube
0x3F, //"0", 0
0x06, //"1", 1
0x5B, //"2", 2
0x4F, //"3", 3
0x66, //"4", 4
0x6D, //"5", 5
0x7D, //"6", 6
0x07, //"7", 7
0x7F, //"8", 8
0x6F, //"9", 9
0x77, //"A", 10
0x7C, //"B", 11
0x39, //"C", 12
0x5E, //"D", 13
0x79, //"E", 14
0x71, //"F", 15
0x76, //"H", 16
0x38, //"L", 17
0x37, //"n", 18
0x3E, //"u", 19
0x73, //"P", 20
0x5C, //"o", 21
0x40, //"-", 22
0x00, //turn off 23
};
unsigned char DotDig0=0,DotDig1=0,DotDig2=0,DotDig3=0; //decimal point control bit
unsigned char code LedAddr[]={0xfe,0xfd,0xfb,0xf7}; //digital tube position selection
unsigned char LedBuf[]={22,22,22,22}; //Display buffer area
void DisplaySmg() //Four-digit digital tube, consider the decimal point
{
unsigned char i; // equivalent to "static unsigned char i = 0;"
unsigned char temp;
switch(i)
{
case 0:
{
GPIO_SEG = 0x00; //Eliminate shadow
if(DotDig0==1) //decimal point
{
temp = LedData[LedBuf[0]] | 0x80; //Light up the decimal point
}
else
{
temp = LedData[LedBuf[0]];
}
GPIO_SEG = temp; //segment code
GPIO_SEL = LedAddr[0]; //bit selection
i++;
break;
}
case 1:
GPIO_SEG = 0x00;
if(DotDig1==1) //decimal point
{
temp = LedData[LedBuf[1]] | 0x80;
}
else
{
temp = LedData[LedBuf[1]];
}
GPIO_SEG = temp;
GPIO_SEL = LedAddr[1];
i++;
break;
case 2:
GPIO_SEG = 0x00;
if(DotDig2==1) //decimal point
{
temp = LedData[LedBuf[2]] | 0x80;
}
else
{
temp = LedData[LedBuf[2]];
}
GPIO_SEG = temp;
GPIO_SEL = LedAddr[2];
i++;
break;
case 3:
GPIO_SEG = 0x00;
if(DotDig3==1) //decimal point
{
temp = LedData[LedBuf[3]] | 0x80;
}
else
{
temp = LedData[LedBuf[3]];
}
GPIO_SEG = temp;
GPIO_SEL = LedAddr[3];
i=0;
break;
default:break;
}
}
5.4 Timer T0 module
#ifndef __Timer0_H__
#define __Timer0_H__
#include
void Timer0_Init(void);
#endif
#include "Timer0.h"
void Timer0_Init(void) //1 millisecond @ 11.0592MHz
{
TMOD &= 0xF0; //Set timer mode
TMOD |= 0x01; //Set timer mode
TL0 = 0x66; //Set the initial value of the timing
TH0 = 0xFC; //Set the initial value of the timing
TF0 = 0; // Clear TF0 flag
TR0 = 1; //Timer 0 starts timing
ET0 = 1; //Timer 0 interrupt switch
//EA = 1; //Interrupt main switch
}
//The interrupt service function must be a function without a return value
//The interrupt service function must be a function without parameters
//Interrupt service function function name followed by the keyword interrupt
//interrupt n 0~4 5 interrupt sources, 8*n+0003H
// 0003H INT0, 00BH T0, 0013H INT1, 001BH T1, 0023H ES
//The interrupt service function cannot be called by the main program or other programs
//n is followed by using m (0~3) working register group
//void Timer0_ISR(void) interrupt 1
//{
// TL0 = 0x66; //Set the initial timing value
// TH0 = 0xFC; //Set the initial value of the timing
//}
6 References
(1) Single chip microcomputer application - ADC0809 interrupt mode to realize A/D conversion of one analog signal (digital tube display)_bilibili_bilibili;
Previous article:Simple digital voltmeter + ADC0809 + bus mode to achieve one-way data conversion
Next article:Simple digital voltmeter + ADC0809 + program query (delay waiting) method
Recommended ReadingLatest update time:2024-11-23 08:26
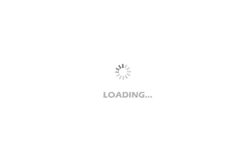
- Popular Resources
- Popular amplifiers
-
MCU C language programming and Proteus simulation technology (Xu Aijun)
-
Principles and Applications of Single Chip Microcomputers and C51 Programming (3rd Edition) (Xie Weicheng, Yang Jiaguo)
-
MCU Principles and Interface Technology C51 Programming (Edited by Zhang Yigang)
-
Principles and Applications of Single Chip Microcomputers (Sun Baofa)
- Naxin Micro and Xinxian jointly launched the NS800RT series of real-time control MCUs
- How to learn embedded systems based on ARM platform
- Summary of jffs2_scan_eraseblock issues
- Application of SPCOMM Control in Serial Communication of Delphi7.0
- Using TComm component to realize serial communication in Delphi environment
- Bar chart code for embedded development practices
- Embedded Development Learning (10)
- Embedded Development Learning (8)
- Embedded Development Learning (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Intel promotes AI with multi-dimensional efforts in technology, application, and ecology
- ChinaJoy Qualcomm Snapdragon Theme Pavilion takes you to experience the new changes in digital entertainment in the 5G era
- Infineon's latest generation IGBT technology platform enables precise control of speed and position
- Two test methods for LED lighting life
- Don't Let Lightning Induced Surges Scare You
- Application of brushless motor controller ML4425/4426
- Easy identification of LED power supply quality
- World's first integrated photovoltaic solar system completed in Israel
- Sliding window mean filter for avr microcontroller AD conversion
- What does call mean in the detailed explanation of ABB robot programming instructions?
- STMicroelectronics discloses its 2027-2028 financial model and path to achieve its 2030 goals
- 2024 China Automotive Charging and Battery Swapping Ecosystem Conference held in Taiyuan
- State-owned enterprises team up to invest in solid-state battery giant
- The evolution of electronic and electrical architecture is accelerating
- The first! National Automotive Chip Quality Inspection Center established
- BYD releases self-developed automotive chip using 4nm process, with a running score of up to 1.15 million
- GEODNET launches GEO-PULSE, a car GPS navigation device
- Should Chinese car companies develop their own high-computing chips?
- Infineon and Siemens combine embedded automotive software platform with microcontrollers to provide the necessary functions for next-generation SDVs
- Continental launches invisible biometric sensor display to monitor passengers' vital signs
- 【Development Kit for nRF52840】+ Review 2: Pathfinder
- ZIGBEE power management POWER_SAVING related functions
- PCB wiring
- Principle and Application of Single Chip Microcomputer
- Children's Toy "Black Box"-- BabyCareAssistant
- Crazy Shell AI open source drone SPI (six-axis sensor data acquisition)
- TE's latest trend report | "How does temperature monitoring affect the generator market"
- 【NXP Rapid IoT Review】+7. Understand what each icon means
- The challenges of power tools brought about changes, and the three-phase brushless DC motor was born.
- 【bk7231N】Tuya product line learning