1 Device resource initialization
Linux-2..6.32.2 already comes with a complete DM9000 network card driver (source code location: linux-2.6.32.2/
drivers/net/dm9000.c). It is also a platform device. Therefore, in the target platform initialization code, just fill in the corresponding
structure table. The specific steps are as follows:
First add the header file dm9000.h required by the driver:
#include
Redefine the physical base address of the DM9000 network card device for later use:
/* DM9000AEP 10/100 ethernet controller */
#define MACH_MINI2440_DM9K_BASE (S3C2410_CS4 + 0x300)
Then fill in the resource settings of the platform device to cooperate with the DM9000 network card driver interface, as follows
static struct resource mini2440_dm9k_resource[] = {
[0] = {
.start = MACH_MINI2440_DM9K_BASE,
.end = MACH_MINI2440_DM9K_BASE + 3,
.flags = IORESOURCE_MEM
},
[1] = {
.start = MACH_MINI2440_DM9K_BASE + 4,
.end = MACH_MINI2440_DM9K_BASE + 7,
.flags = IORESOURCE_MEM
},
[2] = {
.start = IRQ_EINT7,
.end = IRQ_EINT7,
.flags = IORESOURCE_IRQ | IORESOURCE_IRQ_HIGHEDGE,
}
};
/*
* * * The DM9000 has no eeprom, and it's MAC address is set by
* * * the bootloader before starting the kernel.
* * */
static struct dm9000_plat_data mini2440_dm9k_pdata = {
.flags = (DM9000_PLATF_16BITONLY | DM9000_PLATF_NO_EEPROM),
};
static struct platform_device mini2440_device_eth = {
.name = "dm9000",
.id = -1,
.num_resources = ARRAY_SIZE(mini2440_dm9k_resource),
.resource = mini2440_dm9k_resource,
.dev = {
.platform_data = &mini2440_dm9k_pdata,
},
};
; At the same time, add the network card platform device prepared above to the mini2440 device set, as shown in the red part below
static struct platform_device *mini2440_devices[] __initdata = {
&s3c_device_usb,
&s3c_device_lcd,
&s3c_device_wdt,
&s3c_device_i2c0,
&s3c_device_iis,
&mini2440_device_eth,
&s3c_device_nand,
&mini2440_device_eth
};
In this way, the interface of the DM9000 platform device is completed.
Note: Regarding this structure struct resource, the resources of the network card are defined here. Looking at the circuit diagram of the board, you can find that the network card is hung on
The bus address is in bank 4, and the interrupt is external interrupt 7.
2 Adjust the bit width register used by DM9000
Because the DM9000 network card driver of Linux-2.6.32.2 is not specially prepared for mini2440, some porting work needs to be done in its source code, as follows.
Open linux-2.6.32.2/ drivers/net/dm9000.c, add 2410 related configuration definitions in the header file, as shown in the red part below:
#include
#include
#include
#if defined(CONFIG_ARCH_S3C2410)
#include
#endif
#include "dm9000.h"
Add the following red part in the initialization function of the dm9000 device. This is the timing for configuring the chip select bus used by DM9000. Because mini2440 currently has only one device that expands through the bus, it will be easier to understand by directly modifying the relevant register configuration in this device driver. Of course, this part can also be placed in mach-mini2440.c. You can experiment on your own and I will not go into details here.
static int __init
dm9000_init(void)
{
#if defined(CONFIG_ARCH_S3C2410)
unsigned int oldval_bwscon = *(volatile unsigned int *)S3C2410_BWSCON;
unsigned int oldval_bankcon4 = *(volatile unsigned int *)S3C2410_BANKCON4;
*((volatile unsigned int *)S3C2410_BWSCON) =
(oldval_bwscon & ~(3<<16)) | S3C2410_BWSCON_DW4_16 |
S3C2410_BWSCON_WS4 | S3C2410_BWSCON_ST4;
*((volatile unsigned int *)S3C2410_BANKCON4) = 0x1f7c;
#endif
printk(KERN_INFO "%s Ethernet Driver, V%sn", CARDNAME, DRV_VERSION);
return platform_driver_register(&dm9000_driver);
}
3 About MAC address
It should be noted that the DM9000 network card used in this development board does not have an external EEPROM to store the MAC address, so the MAC address in the system is a "soft" address, which means it can be modified by software and can be changed to other values at will. It can be seen in the static int __devinit dm9000_probe(struct platform_device *pdev) function: /* try reading the node address from the attached EEPROM */; try to read the MAC address from the EEPROM
for (i = 0; i < 6; i += 2)
dm9000_read_eeprom(db, i / 2, ndev->dev_addr+i);
if (!is_valid_ether_addr(ndev->dev_addr) && pdata != NULL) {
mac_src = "platform data";
memcpy(ndev->dev_addr, pdata->dev_addr, 6);
}
if (!is_valid_ether_addr(ndev->dev_addr)) {
/* try reading from mac */
mac_src = "chip";
for (i = 0; i < 6; i++)
ndev->dev_addr[i] = ior(db, i+DM9000_PAR);
}
;使用“软”MAC 地址: 08:90:90:90:90:90
memcpy(ndev->dev_addr, "x08x90x90x90x90x90", 6);
if (!is_valid_ether_addr(ndev->dev_addr))
dev_warn(db->dev, "%s: Invalid ethernet MAC address. Please ""set using ifconfign", ndev->name);
实际上到此为止DM9000 就已经移植结束了。
4 Configure the kernel to add DM9000, and compile and run the test. At this time, the kernel source code directory will be included. Execute:
#make menuconfig
to start configuring the network card driver in the kernel. Select the following menu items in sequence:
Device Drivers ---> Network device support ---> Ethernet (10 or 100Mbit) --->
to find the configuration items of DM9000. You can see that DM9000 has been selected. This is because the default kernel configuration of Linux-2.6.32.2 has added support for DM9000.
Then execute:
#make zImage
to generate the arch/arm/boot/zImage file. Use the "k" command to burn it to the development board and boot it using the default file system. Run the ifconfig command in the command line terminal to view the information of eth0.
Note: The above transplant process is mainly based on the manual. Here are some personal additions. About the added lines
unsigned int oldval_bwscon = *(volatile unsigned int *)S3C2410_BWSCON;
unsigned int oldval_bankcon4 = *(volatile unsigned int *)S3C2410_BANKCON4;
*((volatile unsigned int *)S3C2410_BWSCON) =
(oldval_bwscon & ~(3<<16)) | S3C2410_BWSCON_DW4_16 |
S3C2410_BWSCON_WS4 | S3C2410_BWSCON_ST4;
*((volatile unsigned int *)S3C2410_BANKCON4) = 0x1f7c;
What does it mean?
S3C2410_BWSCON, S3C2410_BANKCON4 are actually the addresses of BWSCON and BANKCON4. The former corresponds to the mapped address, and the latter corresponds to the actual physical address. For S3C2440, the virtual-real address mapping relationship is actually very simple, just adding a cheap. Take S3C2410_BWSCON as an example (or track the implementation process of this)
#define S3C2410_BWSCON S3C2410_MEMREG(0x0000)
#define S3C2410_MEMREG(x) (S3C24XX_VA_MEMCTRL + (x))
#define S3C24XX_VA_MEMCTRL S3C_VA_MEM
#define S3C_VA_MEM S3C_ADDR(0x00200000) /* memory control */
#define S3C_ADDR(x) (S3C_ADDR_BASE + (x))
#define S3C_ADDR_BASE (0xF4000000)
In fact, S3C2410_BWSCON is F4200000, which is a mapping of address 0x48000000. This relationship is to add an offset. Everyone should know this.
Let's talk about what the above program does.
Several macro definitions appear in it as follows:
#define S3C2410_BWSCON_ST4 (1<<19)
#define S3C2410_BWSCON_WS4 (1<<18)
#define S3C2410_BWSCON_DW4_16 (1<<16)
Below are bits 16 to 19 of the BWSCON control register
ST4 [19] Determines SRAM for using UB/LB for bank 4.
0 = Not using UB/LB (The pins are dedicated nWBE[3:0])
1 = Using UB/LB (The pins are dedicated nBE[3:0])
0
WS4 [18] Determines WAIT status for bank 4.
0 = WAIT disable 1 = WAIT enable
0
DW4 [17:16] Determine data bus width for bank 4.
00 = 8-bit 01 = 16-bit, 10 = 32-bit 11 = reserved
Below is the meaning of each bit of the BANK4CON register.
Tacs [14:13] Address set-up time before nGCSn
00 = 0 clock 01 = 1 clock
10 = 2 clocks 11 = 4 clocks
00
Tcos [12:11] Chip selection set-up time before nOE
00 = 0 clock 01 = 1 clock
10 = 2 clocks 11 = 4 clocks
00
Tacc [10:8] Access cycle
000 = 1 clock 001 = 2 clocks
010 = 3 clocks 011 = 4 clocks
100 = 6 clocks 101 = 8 clocks
110 = 10 clocks 111 = 14 clocks
Note: When nWAIT signal is used, Tacc ³ 4 clocks.
111
Tcoh [7:6] Chip selection hold time after nOE
00 = 0 clock 01 = 1 clock
10 = 2 clocks 11 = 4 clocks
000
Tcah [5:4] Address hold time after nGCSn
00 = 0 clock 01 = 1 clock
10 = 2 clocks 11 = 4 clocks
00
Tacp [3:2] Page mode access cycle @ Page mode
00 = 2 clocks 01 = 3 clocks
10 = 4 clocks 11 = 6 clocks
00
PMC [1:0] Page mode configuration
00 = normal (1 data) 01 = 4 data
10 = 8 times 11 = 16 times
Convert the data to be assigned above into binary bits, and check one by one to see what function is set. In general, it is to set the timing, so I won't go into details.
Previous article:Linux-2.6.32 ported to mini2440 development board - ported to yaffs2
Next article:Linux-2.6.32 transplanted on mini2440 development board - RTC transplanted
Recommended ReadingLatest update time:2024-11-15 14:49
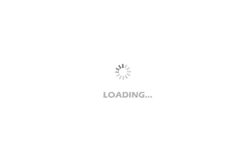
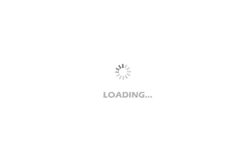
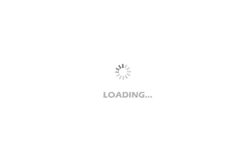
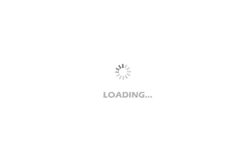
- Popular Resources
- Popular amplifiers
- Learn ARM development(16)
- Learn ARM development(17)
- Learn ARM development(18)
- Embedded system debugging simulation tool
- A small question that has been bothering me recently has finally been solved~~
- Learn ARM development (1)
- Learn ARM development (2)
- Learn ARM development (4)
- Learn ARM development (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- Analysis of the application of several common contact parts in high-voltage connectors of new energy vehicles
- Wiring harness durability test and contact voltage drop test method
- From probes to power supplies, Tektronix is leading the way in comprehensive innovation in power electronics testing
- From probes to power supplies, Tektronix is leading the way in comprehensive innovation in power electronics testing
- Sn-doped CuO nanostructure-based ethanol gas sensor for real-time drunk driving detection in vehicles
- Design considerations for automotive battery wiring harness
- Do you know all the various motors commonly used in automotive electronics?
- What are the functions of the Internet of Vehicles? What are the uses and benefits of the Internet of Vehicles?
- Power Inverter - A critical safety system for electric vehicles
- Analysis of the information security mechanism of AUTOSAR, the automotive embedded software framework
- Chinese mobile phones continue to sell well in India, Vivo surpasses Samsung to become the second largest brand
- Share CC2541 Bluetooth learning about ADC
- Summary of frequently asked questions about oscilloscopes (Part 2)
- MSP430 LCD5110 driver programming example
- A huge reward! Looking for someone who can crack the RSA2048 and factory protocol in the ECU!
- Family Pet Health Maintenance System
- [Sipeed LicheeRV 86 Panel Review] 2. Data Links
- TI DSP CAN online program upgrade question
- List of instruments, equipment and main components for the 2019 National Undergraduate Electronic Design Competition
- Has this condition reached the level of shock (moderate)?