There are some differences between the NAND FLASH driver of S3C2440A and S3C2410. The main difference is that the NAND FLASH registers of these two ICs are slightly different. Please see the difference between the two below:
-
//
-
// Copyright (c) Microsoft Corporation. All rights reserved.
-
//
-
//
-
// Use of this source code is subject to the terms of the Microsoft end-user
-
// license agreement (EULA) under which you licensed this SOFTWARE PRODUCT.
-
// If you did not accept the terms of the EULA, you are not authorized to use
-
// this source code. For a copy of the EULA, please see the LICENSE.RTF on your
-
// install media.
-
//
-
//------------------------------------------------ ----------------------------------
-
//
-
// Header: s3c2410x_nand.h
-
//
-
// Defines the NAND controller CPU register layout and definitions.
-
//
-
#ifndef __S3C2410X_NAND_H
-
#define __S3C2410X_NAND_H
-
-
#if __cplusplus
-
extern "C"
-
{
-
#endif
-
-
-
//------------------------------------------------ ----------------------------------
-
// Type: S3C2410X_NAND_REG
-
//
-
// NAND Flash controller register layout. This register bank is located
-
// by the constant CPU_BASE_REG_XX_NAND in the configuration file
-
// cpu_base_reg_cfg.h.
-
//
-
-
typedef struct
-
{
-
UINT32 NFCONF; // configuration reg
-
UINT8 NFCMD; // command set reg
-
UINT8 pad1[3]; // pad
-
UINT8 NFADDR; // address set reg
-
UINT8 pad2[3]; // pad
-
UINT8 NFDATA; // data reg
-
UINT8 pad3[3]; // pad
-
UINT32 NFSTAT; // operation status reg
-
UINT32 NFECC; // error correction code 0
-
-
} S3C2410X_NAND_REG, *PS3C2410X_NAND_REG;
-
-
-
#if __cplusplus
-
}
-
#endif
-
-
#endif
The above code is the register of S3C2410A. Let's take a look at the register address description of S3C2440A:
-
//
-
// Copyright (c) Microsoft Corporation. All rights reserved.
-
//
-
//
-
// Use of this source code is subject to the terms of the Microsoft end-user
-
// license agreement (EULA) under which you licensed this SOFTWARE PRODUCT.
-
// If you did not accept the terms of the EULA, you are not authorized to use
-
// this source code. For a copy of the EULA, please see the LICENSE.RTF on your
-
// install media.
-
//
-
//------------------------------------------------ ----------------------------------
-
//
-
// Header: s3c2440a_nand.h
-
//
-
// Defines the NAND controller CPU register layout and definitions.
-
//
-
#ifndef __S3C2440A_NAND_H
-
#define __S3C2440A_NAND_H
-
-
#if __cplusplus
-
extern "C"
-
{
-
#endif
-
-
-
//------------------------------------------------ ----------------------------------
-
// Type: S3C2440A_NAND_REG
-
//
-
// NAND Flash controller register layout. This register bank is located
-
// by the constant CPU_BASE_REG_XX_NAND in the configuration file
-
// cpu_base_reg_cfg.h.
-
//
-
-
typedef struct
-
{
-
UINT32 NFCONF; // configuration reg
-
UINT32 NFCONT;
-
UINT8 NFCMD; // command set reg
-
UINT8 d0[3];
-
UINT8 NFADDR; // address set reg
-
UINT8 d1[3];
-
UINT8 NFDATA; // data reg
-
UINT8 d2[3];
-
UINT32 NFMECCD0;
-
UINT32 NFMECCD1;
-
UINT32 NFSECCD;
-
UINT32 NFSTAT; // operation status reg
-
UINT32 NFESTAT0;
-
UINT32 NFESTAT1;
-
UINT32 NFMECC0; // error correction code 0
-
UINT32 NFMECC1; // error correction code 1
-
UINT32 NFSECC;
-
UINT32 NFSBLK;
-
UINT32 NFEBLK; // error correction code 2
-
-
} S3C2440A_NAND_REG, *PS3C2440A_NAND_REG;
-
-
-
#if __cplusplus
-
}
-
#endif
-
-
#endif
The difference between the two is huge. Therefore, the difficulty in transplantation is mainly the ECC part. At the same time, the addresses of some register bits are different and need to be modified, as shown below:
-
// Use Macros here to avoid extra over head for c function calls
-
#define READ_REGISTER_BYTE(p) (*(PBYTE)(p))
-
#define WRITE_REGISTER_BYTE(p, v) (*(PBYTE)(p)) = (v)
-
#define READ_REGISTER_USHORT(p) (*(PUSHORT)(p))
-
#define WRITE_REGISTER_USHORT(p, v) (*(PUSHORT)(p)) = (v)
-
#define READ_REGISTER_ULONG(p) (*(PULONG)(p))
-
#define WRITE_REGISTER_ULONG(p, v) (*(PULONG)(p)) = (v)
The above code can be used for both S3C2410 and S3C2440. When I transplanted them, the two parts were the same. Of course, the registers of S3C2443 and S3C2450 have not changed much, but the NAND FLASH driver in the BSP of S3C2450 has changed a lot. I will analyze it later. Now I will analyze this under WINCE5.0. I haven't had time to transplant WINCE6.0 yet. I usually like to transplant it myself, so that I can have a deeper understanding of the WINCE process and become more familiar with this IC. Let's take a look at the difference between the two.
Register macro definition of S3C2440A:
-
//MACROS
-
#define NF_CE_L() WRITE_REGISTER_USHORT(pNFCONT, (USHORT) (READ_REGISTER_USHORT(pNFCONT) & ~(1<<1)))
-
#define NF_CE_H() WRITE_REGISTER_USHORT(pNFCONT, (USHORT) (READ_REGISTER_USHORT(pNFCONT) | (1<<1)))
-
#define NF_CMD(cmd) WRITE_REGISTER_USHORT(pNFCMD, (USHORT) (cmd))
-
#define NF_ADDR(addr) WRITE_REGISTER_USHORT(pNFADDR, (USHORT) (addr))
-
#define NF_DATA_R() READ_REGISTER_BYTE(pNFDATA)
-
#define NF_DATA_W(val) WRITE_REGISTER_BYTE(pNFDATA, (BYTE) (val))
-
#define NF_DATA_R4() READ_REGISTER_ULONG(pNFDATA)
-
#define NF_DATA_W4(val) WRITE_REGISTER_ULONG(pNFDATA, (ULONG) (val))
-
#define NF_STAT() READ_REGISTER_USHORT(pNFSTAT)
-
#define NF_MECC_UnLock() WRITE_REGISTER_USHORT(pNFCONT, (USHORT) (READ_REGISTER_USHORT(pNFCONT) & ~(1<<5)))
-
#define NF_MECC_Lock() WRITE_REGISTER_USHORT(pNFCONT, (USHORT) (READ_REGISTER_USHORT(pNFCONT) | (1<<5)))
-
#define NF_RSTECC() WRITE_REGISTER_USHORT(pNFCONT, (USHORT) (READ_REGISTER_USHORT(pNFCONT) | (1<<4)))
-
#define NF_WAITRB() {while(!(NF_STAT() & (1<<1))) ;}
-
#define NF_CLEAR_RB() WRITE_REGISTER_USHORT(pNFSTAT, (USHORT) (READ_REGISTER_USHORT(pNFSTAT) | (1<<2)))
-
#define NF_DETECT_RB() {while(!(NF_STAT() & (1<<2)));}
-
#define NF_ECC() READ_REGISTER_ULONG(pNFECC)
Register macro definition of S3C2410A:
-
//MACROS
-
#define NF_CE_L() WRITE_REGISTER_USHORT(pNFCONF, (USHORT) (READ_REGISTER_USHORT(pNFCONF) & ~(1 << 11)))
-
#define NF_CE_H() WRITE_REGISTER_USHORT(pNFCONF, (USHORT) (READ_REGISTER_USHORT(pNFCONF) | (1 << 11)))
-
#define NF_CMD(cmd) WRITE_REGISTER_USHORT(pNFCMD, (USHORT) (cmd))
-
#define NF_ADDR(addr) WRITE_REGISTER_USHORT(pNFADDR, (USHORT) (addr))
-
#define NF_DATA_R() READ_REGISTER_USHORT(pNFDATA)
-
#define NF_DATA_W(val) WRITE_REGISTER_BYTE(pNFDATA, (BYTE)(val))
-
-
#define NF_STAT() READ_REGISTER_USHORT(pNFSTAT)
-
-
#define NF_RSTECC() WRITE_REGISTER_USHORT(pNFCONF, (USHORT) (READ_REGISTER_USHORT(pNFCONF) | (1 << 12)))
-
#define NF_WAITRB() {while(!(NF_STAT() & (1<<0))) ;}
-
#define NF_DETECT_RB() {while(!(NF_STAT() & 0x01));}
-
#define NF_ECC() READ_REGISTER_ULONG(pNFECC)
The registers of S3C2410 are the register definitions that I have modified. The main differences are: NF_CE_L(), NF_CE_H(), NF_RSTECC(), NF_WAITRB(), NF_DETECT_RB(). These registers can be distinguished by the detailed descriptions on the datasheets of the two. I will not explain them in detail here! Modifying the registers is the first and most important step in porting the NAND FLASH driver.
Previous article:ADC driver implementation for ARM Linux S3C2440
Next article:S3C2440 Hardware Programming Example
Recommended ReadingLatest update time:2024-11-16 09:24
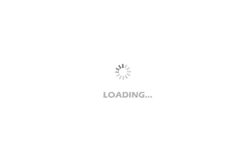
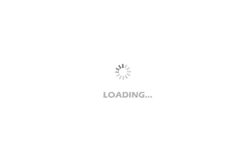
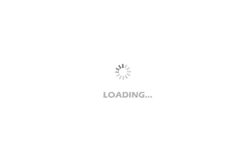
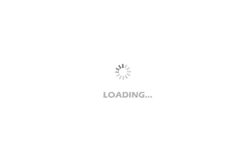
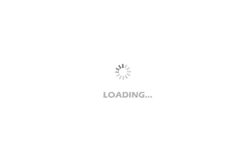
- Popular Resources
- Popular amplifiers
-
Automotive Electronics S32K Series Microcontrollers: Based on ARM Cortex-M4F Core
-
EDA Technology Practical Tutorial--Verilog HDL Edition (Sixth Edition) (Pan Song, Huang Jiye)
-
ARM Embedded System Principles and Applications (Wang Xiaofeng)
-
Deep Understanding of Linux Driver Design (Tsinghua Developer Library) (Wu Guowei, Yao Lin, Bi Chenglong)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- TGF4042 Function Signal Generator Review_General Parameter Measurement
- GitHub Annual Report: TypeScript surpasses C++ to become the fourth most popular language
- Remote emission management terminal involves diesel engine purification and non-exhaust pollutant control technology
- IAR FOR 430 Failed to re-intialize A possible solution
- Design information for temperature monitoring system
- Speed, frequency, chip instruction set
- The MPU9250 magnetometer ID reading does not get the correct value for the following reasons:
- CircuitPython 6.0.0 Beta 1 released
- Controlling LED brightness under ZSTACK
- [Analog Electronics Course Selection Test] + Input and Output Limitation