Copyright (c) 2010 Rafael J. Wysocki Copyright (c) 2010 Alan Stern stern@rowland.harvard.edu *************************************************** *********** This article was translated by DroidPhone on 2011.8.5 *************************************************** *********** Most of the Linux source code belongs to the device driver code. Therefore, most of the power management (PM) code also exists in the driver. Many drivers may only do a small amount of work, while others, such as those using battery-powered hardware platforms (mobile phones, etc.), will do a lot of work in power management. This document provides a general description of how the driver interacts with the power management part of the system, especially the sharing of models and interfaces related to the driver core. It is recommended that people who are engaged in driver-related fields can learn about it through this document. background knowledge. =================================== Drivers can use one of these models to put the device into a low-power state: The driver, as part of the program, follows system-level low-power states like "suspend" (also called "suspend-to-RAM"), or for systems with hard drives, can enter "hibernation" (also called "suspend-to-RAM"). -disk"). In this case, the driver, bus, and device class driver work together to clearly shut down the hardware device and various software subsystems through various device-specific suspend and resume methods, and then reactivate the hardware without losing data. equipment. Some drivers can manage hardware wake-up events that can cause the system to leave a low-power state. This feature can be turned on and off through the corresponding /sys/devices/.../power/wakeup file (for Ethernet drivers, ethtool achieves the same purpose through the ioctl interface); enabling this feature may cause additional functionality consumption, but it gives the entire system more opportunities to enter a low-power state. This model allows the device to enter a low-power state during system operation, in principle, independently of other power management activities. However, devices generally cannot be controlled individually (for example, a parent device cannot enter suspend unless all of its child devices have entered the suspend state). In addition, depending on the bus type, some special operations may have to be done to achieve the purpose. If the device enters a low-power state during system operation, special handling must be made during system-level power state transitions (suspend or hibernation). For this reason, not only the device driver itself, but also the corresponding subsystem (bus type, device type, device class) driver and power management core will be involved in the work of rumtime power management. For example, when the system is sleeping, the above modules must cooperate with each other to implement various suspend and resume methods so that the hardware can enter a low-power state and continue to provide services after waking up without losing data. There isn't much we can say about the definition of low-power states, as they are often system-specific or even specific to a device. If enough devices enter a low-power state while the system is running, the effect is actually very similar to entering a system-level low-power state. In this way, some drivers can use rumtime power management to put the system into a state similar to deep power saving. Most devices that enter the suspend state will stop all I/O operations: there will be no DMA or IRQ requests (except those that need to wake up the system), no data reading or writing, and no requests from upper-layer drivers will be accepted. This will have different requirements for different buses and platforms. Some examples of hardware wake-up events: alarm initiated by RTC, arrival of network packets, keyboard or mouse activity, insertion or removal of media (PCMCIA, MMC/SD, USB, etc.). ================================================== = The kernel provides corresponding programming interfaces for various subsystems (bus type, device type, device class) and drivers so that they can participate in the power management of the devices they care about. These interfaces cover system-level sleep and runtime-level management. ================================================== = Device power management operations for subsystems and drivers are defined in the dev_pm_ops structure: struct dev_pm_ops { int(*prepare)(struct device *dev); void(*complete)(struct device *dev); int(*suspend)(struct device *dev); int(*resume)(struct device *dev); int(*freeze)(struct device *dev); int(*thaw)(struct device *dev); int(*poweroff)(struct device *dev); int(*restore)(struct device *dev); int(*suspend_noirq)(struct device *dev); int(*resume_noirq)(struct device *dev); int(*freeze_noirq)(struct device *dev); int(*thaw_noirq)(struct device *dev); int(*poweroff_noirq)(struct device *dev); int(*restore_noirq)(struct device *dev); int(*runtime_suspend)(struct device *dev); int(*runtime_resume)(struct device *dev); int(*runtime_idle)(struct device *dev); }; This structure is defined in include/linux/pm.h, and their functions will be described next. For now, we just have to remember that the last three methods are specifically for rumtime pm, the others are for system-level power state migration. In some subsystems, there are still so-called "outdated" or "traditional" power management operation interfaces. This method does not use the dev_pm_ops structure and is only applicable to system-level power management methods. This article will not It will be explained, if you want to understand please look directly at the source code of the kernel. -------------------------------------------------------- The key methods for the device to enter suspend and resume are in the pm members of the bus_type structure, device_type structure and class structure. It is a pointer to the dev_pm_ops structure. In most cases, these are the concerns of the maintainers of a specific bus architecture (such as PCI or USB or a device class and device class). Bus drivers implement these methods appropriately for the hardware and drivers to use them; because PCI and USB work in different ways. Only a few people write subsystem-level drivers; most device drivers are built on top of various bus-architecture-specific code. These calls will be described in more detail later; they will be called device by device along the parent-child device model tree. -------------------------------------------------- All devices in the device model have two flags to control wake events (which can cause the device or system to exit a low-power state). Assume that the two flag bits are initialized by the bus or device driver using device_set_wakeup_capable() and device_set_wakeup_enable(), which are defined in include/linux/pm_wakeup.h. The "can_wakeup" flag indicates that the device (or driver) physically supports wake events, and the device_set_wakeup_capable() function affects this flag. The "should_wakeup" flag controls whether the device should attempt to enable its wakeup mechanism. device_set_wakeup_enable() affects this flag. Most drivers do not actively modify their values. The initial value of should_wakeup for most devices is set to false. There are exceptions, such as the power button, keyboard, and network cards with wake-on-LAN function set by ethtool. Whether the device has the ability to issue wake-up events is a hardware issue, and the kernel is only responsible for continuously tracking the occurrence of these events. On the other hand, whether a wake-capable device should initiate a wake-up event is a policy issue, which is managed by user space through the sysfs property file (power/wakeup). User space can write "enabled" or "disabled" to set or clear the shoe_wakeup flag. Correspondingly, when reading the file, if the can_wakeup flag is true, the corresponding string is returned. If can_wakeup is false, an empty value is returned. A string indicating that the device does not support wake events. (It should be noted that although an empty string is returned, writing to the file will still affect the should_wakeup flag) The device_may_wakeup() function will return true only if both flags are true. When the system transitions to sleep, the driver should check through this function to determine whether the wake-up mechanism is enabled before putting the device into a low-power state. However, in rumtime power management mode, wake-up events will be enabled regardless of whether the device and driver support it or whether the should_wakeup flag is set. -------------------------------------------------------- Each device in the device model has a flag bit to control whether it belongs to runtime power management mode. This flag called runtime_auto is initialized by the bus type (or other subsystem) using pm_rumtime_allow() or pm_rumtime_forbid(). The default value is to allow rumtimepm. User space can modify this flag by writing "on" or "auto" to the device's sysfs file power/control. Writing "auto" is equivalent to calling pm_rumtime_allow(), allowing the device to be rumtimepmed by the driver. Writing "on" is equivalent to calling pm_rumtime_forbid(), the flag bit is cleared, the device will return to the full power state from the low power state, and prevents the device from performing runtime power management. User space can also read this file to check the current value of runtime_auto. The device's runtime_auto flag does not affect system-level power state migration. Of particular note is that even though the runtime_auto flag is cleared, the device is also brought into a low-power state when the system-level power state transitions to sleep. Two models of device power management
1. System sleep model:
2. Runtime power management model:
Interface to enter system sleep state
Device power management operations
Subsystem-Level method
/sys/devices/.../power/wakeup files
/sys/devices/.../power/control files
Previous article:Detailed explanation of Run-time PM of power management of Linux driver (4)
Next article:Changes in power management methods in the new version of Linux system device architecture of linux driver power management
Recommended ReadingLatest update time:2024-11-16 14:35
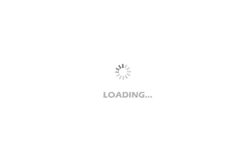
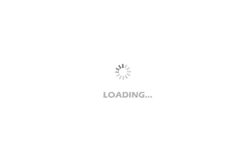
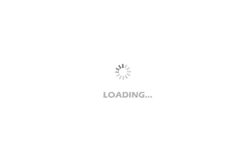
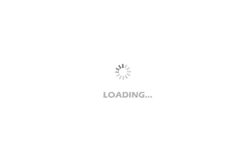
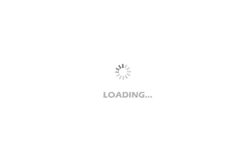
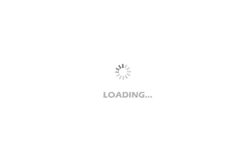
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- TI Live: Overview and application introduction of 60G millimeter wave sensors, "packaged antenna" makes building and factory detection solutions simpler
- First day of the journey in Shenzhen
- Ethernet Problems
- MicroPython precompiled firmware for TB-01 (W600)
- Is it necessary to attend Altium's offline training?
- How to prevent audio equipment from aging and make the sound better
- Discussion on BQ35100 Li-ion Battery Capacity Monitoring
- Evaluation: STM32F769I-DISCO connected to Gizwits Cloud to realize IoT development remote control and other functions
- Application of 4G solar energy monitoring
- GD32E231 analog IIC driver LPS22HH