To light up the LED on the development board, you need to first check the schematic diagram, find the corresponding pins, and understand the schematic diagram to see how the light will light up on the circuit.
1. Look at the schematic diagram JZ2440v2_sch.pdf and find the corresponding pins
nLED_1 corresponds to GPF4
nLED_2 corresponds to GPF5
nLED_4 corresponds to GPF6
2. Read the chip manual S3C2440A_UserManual_Rev13.pdf to set the corresponding I/O registers
CPFCON Control Register
GPFCON Data Register
Set the control register corresponding to the pin to output and the data register to 0 (indicating output low level) to light up the corresponding LED.
The assembly code to light up nLED_1 is as follows:
.global _start
_start:
LDR R0,=0x56000050 @ R0 is set to GPFCON register
@ This register is used to select the function of each pin of the port
@ is output, input or other
MOV R1,#0x00000100
STR R1,[R0] @ Set GPF4 as output port, bit [9:8] = 01
LDR R0,=0x56000054 @ R0 is set to GPFDAT register
@ Used to read/write data of port pins
MOV R1,#0x00000000 @ Change this value to 0x00000010 to turn off the LED
STR R1,[R0] @ GPF4 output 0, light up LED1
MAIN_LOOP:
B MAIN_LOOP
When writing bare metal programs in C language, you need to write the startup file yourself. (In conventional programs, the startup file is completed by the operating system, but there is no operating system in the logic.) The function of the startup file is to initialize the stack, call the main function, and do cleanup work when the main function returns after execution.
The startup file contains at least:
Software initialization:
1. Setting the stack: Setting the stack means pointing the stack pointer (sp=>) to the memory. When the memory is SRAM on the chip, it can be used directly, but when it is SDRAM, it needs to be initialized first.
2. Set the return address.
3. Call the main function.
4. Cleaning work. (Generally used to recycle resources, etc.)
Hardware initialization:
1. Turn off the watchdog. When the 2440 starts, the watchdog will count down. If it is not turned off within 3 seconds, it will restart the development board.
2. Initialize the clock. The 2440 can run at up to 400 MHz, but the clock frequency is only 12 MHz when powered on.
3. Initialize SDRAM.
Startup file content:
@******************************************************************************
@ File:crt0.S
@ Function: Startup file (through which the C program is transferred)
@******************************************************************************
.text
.global _start
_start:
ldr r0, =0x53000000 @ WATCHDOG register address
mov r1, #0x0
str r1, [r0] @ Write 0 to the watchdog register to disable watchdog. Otherwise the CPU will restart continuously.
ldr sp, =1024*4 @ Set the stack. Note: it cannot be larger than 4K, because only 4K of memory is available now.
@ The code in nand flash will be moved to internal ram after reset, this ram is only 4K
bl main @ Call the main function in the C program
halt_loop:
b halt_loop
C language lights up nLED_1 (implements the same function as assembly language) source code:
#define GPFCON (*(volatile unsigned long *)0x56000050)
#define GPFDAT (*(volatile unsigned long *)0x56000054)
int main(int argc,char **argv){
GPFCON |= (1 << 8); // Set GPF4 as output port, bit [9:8] = 01
GPFDAT &= (0 << 4); // GPF4 outputs 0, turns on the LED
return 0;
}
Write a running light in C language
#define GPFCON (*(volatile unsigned long *)0x56000050)
#define GPFDAT (*(volatile unsigned long *)0x56000054)
#define CPF4_OUT (1 << 8)
#define CPF5_OUT (1 << 10)
#define CPF6_OUT (1 << 12)
void delay_time(unsigned int sec){
for(;sec > 0; sec--);
}
int main(int argc, char **argv){
GPFCON = CPF4_OUT | CPF5_OUT | CPF6_OUT;
unsigned int i = 0;
while(1){
delay_time(4000);
GPFDAT = (~(i << 4));
if(++i == 8)
i = 0;
}
return 0;
}
Write a button to control the light in C language
The principle is the same as lighting, but with one more part (need to determine whether the button is pressed). Then you need to check the schematic diagram to find the pins corresponding to the button, and how to determine whether the button is pressed or released. Which registers need to be set.
1. Check the schematic diagram JZ2440v2_sch and find the pins corresponding to the buttons
s2 corresponds to GPF0
s3 corresponds to GPF2
s4 corresponds to GPG3
2. Read the chip manual S3C2440A_UserManual_Rev13.pdf to set the corresponding I/O registers
GPFCON Control Register
GPFCON Data Register
GPGCON Control Register
GPGDAT Data Register
Set the control register corresponding to the pin as input, read the value of the data register, and if it is 0 (indicating a low level is connected), the corresponding button is pressed, and the LED to be controlled is turned on. If 1 is detected, it means that the button is released, and the LED to be controlled is turned off.
Source code:
#define GPFCON (*(volatile unsigned long *)0x56000050)
#define GPFDAT (*(volatile unsigned long *)0x56000054)
#define GPGCON (*(volatile unsigned long *)0x56000060)
#define GPGDAT (*(volatile unsigned long *)0x56000064)
//Set the pin corresponding to the LED to output
#define GPF4_OUT (1 << 8)
#define GPF5_OUT (1 << 10)
#define GPF6_OUT (1 << 12)
//led control register mask
#define GPF4_MASK (3 << 8)
#define GPF5_MASK (3 << 10)
#define GPF6_MASK (3 << 12)
//Set the pin corresponding to the button to input
#define GPF0_IN (0 << 0)
#define GPF2_IN (0 << 4)
#define GPG3_IN (0 << 6)
//Key control register mask
#define GPF0_MASK (3 << 0)
#define GPF2_MASK (3 << 4)
#define GPG3_MASK (3 << 6)
int main(int argc, char **argv){
GPFCON &= ~(GPF0_MASK | GPF2_MASK | GPF4_MASK | GPF5_MASK | GPF6_MASK);
GPFCON |= (GPF4_OUT | GPF5_OUT | GPF6_OUT | GPF0_IN | GPF2_IN);
GPGCON &= ~GPG3_MASK;
GPGCON |= GPG3_IN;
unsigned long dwDat;
while(1){
// Take out the value of the data register and determine whether the response data bit is 1 or 0. If it is 0, the corresponding LED will be lit.
dwDat = GPFDAT;
if (dwDat & 1)
GPFDAT |= (1 << 4);
else
GPFDAT &= ~(1 << 4);
if (dwDat & (1 << 2))
GPFDAT |= (1 << 5);
else
GPFDAT &= ~(1 << 5);
dwDat = GPGDAT;
if (dwDat & (1 << 3))
GPFDAT |= (1 << 6);
else
GPFDAT &= ~(1 << 6);
}
return 0;
}
The above C language needs to be compiled together with the startup file when compiling. The following is a simple Makefile for the last program as an example.
key_led.bin : crt0.S key_led.c
arm-linux-gcc -g -c -o crt0.o crt0.S
arm-linux-gcc -g -c -o key_led.o key_led.c
arm-linux-ld -Ttext 0x00000000 -g crt0.o key_led.o -o key_led_elf
arm-linux-objcopy -O binary -S key_led_elf key_led.bin
clean:
rm -f key_led.bin key_led_elf *.o
Previous article:S3C2440 interrupt control register
Next article:Memory Management Unit MMU
Recommended ReadingLatest update time:2024-11-16 17:44
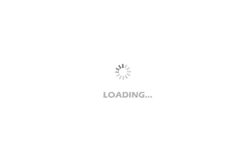
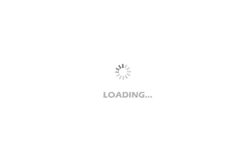
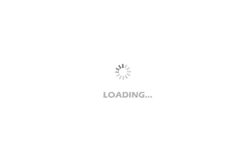
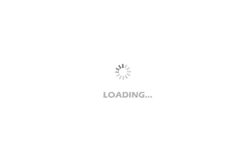
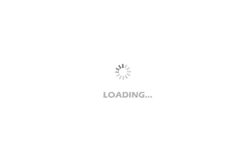
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Temperature transmitter hardware framework and schematic diagram
- Testing solutions for redundant link networks
- Key wireless technologies for 5G systems
- [NXP Rapid IoT Review] + Rapid IoT App Running Error
- How to Design an RF Power Amplifier: The Basics
- What is the principle of touch switch?
- What is jitter and phase noise?
- [Shanghai Hangxin ACM32F070 development board + touch function evaluation board evaluation] + OLED screen display driver
- Tailing Micro B91 Development Kit Burning Pitfalls Record
- Antai Testing - Sharing of Maintenance Experience of Tektronix AFG3021 Arbitrary Signal Generator