Because the previous bare metal program is very simple, I won’t blog about it.
Program flow:
1. Initialize C SP
2. Turn off the watchdog
3. Initialize SDRAM
4. Read the program containing pictures in NAND FLASH and put it into SDRAM.
5. Jump to SDRAM for execution
Because the 2440 automatically only reads 4K to SRAM, if you put pictures in it, it will naturally not be enough. It's just one more step to put it in SDRAM.
I didn't directly copy the program in the tutorial, that one is more complicated. C library files are used.
In the tutorial, we just draw lines, and drawing circular lines is very simple.
At the beginning, I used 24BPP to develop, so I encountered many problems.
The picture needs to be converted into a C language header file. I see that there are many people on the Internet looking for software to convert, and there is also one who uses C to convert under LINUX. I looked for a software, it was made by MFC, but it didn't support 24BPP.
I can only do it myself. Written in python. The link is here: http://www.cnblogs.com/ningci/p/5203053.html
16BPP:
//lcd controller
typedef struct{
unsigned long LCDCON1;
unsigned long LCDCON2;
unsigned long LCDCON3;
unsigned long LCDCON4;
unsigned long LCDCON5;
unsigned long LCDSADDR1;
unsigned long LCDSADDR2;
unsigned long LCDSADDR3;
unsigned long REDLUT;
unsigned long GREENLUT;
unsigned long BLUELUT;
unsigned long DITHMODE;
unsigned long TPAL;
} LCD;
LCD * lcd = (LCD *)0x4d000000;
#define GPBCON (*(volatile unsigned long *)0x56000010)
#define GPBDAT (*(volatile unsigned long *)0x56000014)
#define GPCUP (*(volatile unsigned long *)0x56000028)
#define GPCCON (*(volatile unsigned long *)0x56000020)
#define GPDUP (*(volatile unsigned long *)0x56000038)
#define GPDCON (*(volatile unsigned long *)0x56000030)
#define GPGUP (*(volatile unsigned long *)0x56000068)
#define GPGCON (*(volatile unsigned long *)0x56000060)
#define HCLK 100000000
#define LCD_WIDTH 480
#define LCD_HEIGHT 272
#define LCD_CLKVAL 4
#define LCD_TFT 3
#define LCD_24BBP 0xd
#define LCD_16BBP 0xc
#define LCD_EN_OFF 0
#define LCD_EN_ON 1
#define LCD_VBPD 1
#define LCD_LINEVAL (LCD_HEIGHT - 1)
#define LCD_VFPD 1
#define LCD_VSPW 9
#define LCD_HBPD 1
#define LCD_HOZVAL (LCD_WIDTH - 1)
#define LCD_HFPD 1
#define LCD_HSPW 40
#define LCD_INVVLINE 1
#define LCD_INVVFRAME 1
#define LCD_FRAMEBUFFER 0x30400000 //4M aligned address
void wait(s)
{
while(s--);
}
void init_lcd()
{
//LCD_PWREN
GPGUP = 0xffffffff; // Disable internal pull-up
GPGCON = 3<<8;
GPCUP = 0xffffffff; // Disable internal pull-up
GPCCON = 0xaaaaaaaa; // GPIO pins for VD[7:0], LCDVF[2:0], VM, VFRAME, VLINE, VCLK, LEND
GPDUP = 0xffffffff; // Disable internal pull-up
GPDCON = 0xaaaaaaaa; // GPIO pins for VD[23:8]
//GPB0 KEYBOARD
//backlight on
GPBCON &= ~(3);
GPBCON |= 1;
//HCLK 100M LCD CLK 9M 100/9/2-1=4
//The default is not enabled
lcd->LCDCON1 = LCD_CLKVAL<<8 | LCD_TFT<<5 | LCD_16BBP<<1 | LCD_EN_OFF;
lcd->LCDCON2 = LCD_VBPD<<24 | LCD_LINEVAL<<14 | LCD_VFPD<<6 | LCD_VSPW;
lcd->LCDCON3 = LCD_HBPD<<19 | LCD_HOZVAL<<8 | LCD_HFPD;
lcd->LCDCON4 = LCD_HSPW;
//8bpp BSWP 1 16bpp HWSWP 1 24bpp 0 0
lcd->LCDCON5 = 1<<11 | LCD_INVVLINE<<9 | LCD_INVVFRAME<<8 | 1;
//The address is stored separately and the 31:22 bits can be shifted to the right. The 21:1 bits are used & the upper 21 1s are cleared if the high bit is not 7.
lcd->LCDSADDR1 = (LCD_FRAMEBUFFER>>22)<<21 | ((LCD_FRAMEBUFFER>>1) & 0x1fffff);
//24bpp occupies 4 lengths of 16bpp, 2 lengths of 8bpp and 1 length
lcd->LCDSADDR2 = ((LCD_FRAMEBUFFER + LCD_WIDTH * LCD_HEIGHT*2)>>1) & 0x1fffff;
lcd->LCDSADDR3 = LCD_WIDTH;
lcd->TPAL = 0;
}
void lcd_on()
{
//backlight on
GPBDAT |= 1;
lcd->LCDCON1 |= LCD_EN_ON;
lcd->LCDCON5 |= 0x3<<2;
}
void lcd_off()
{
//Backlight off
GPBDAT &= 0;
lcd->LCDCON1 &= LCD_EN_OFF;
lcd->LCDCON5 &= ~(0x3<<2);
}
void show_img(unsigned short *img)
{
int i=0;
//Video memory address
unsigned short * frame_buf = (volatile unsigned short *)LCD_FRAMEBUFFER;
for(i=0;i<(480*272);i++)
{
*frame_buf = *img;
frame_buf++;
img++;
}
}
#include "img1.h"
int main()
{
init_lcd();
lcd_on();
show_img(&img1);
return 0;
}
Just write the color value directly into the memory and display it on the screen.
The following is the display rendering:
Previous article:Compile busybox-1.24.1 and create a file system
Next article:ARM WIFI AP mode uses iptables nat forwarding to access the Internet through LAN cable
Recommended ReadingLatest update time:2024-11-16 07:51
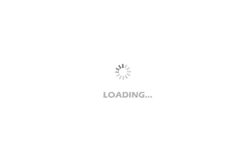
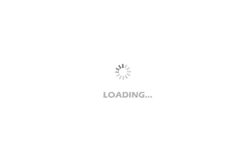
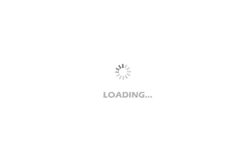
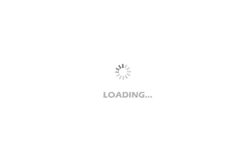
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- [ESP32-Audio-Kit Audio Development Board] - 3: Install "esp-a...
- Understand DNS basics in one article
- Application of Dacai UART screen in environmental testing equipment
- Moto 360 Wireless Charging Base Disassembly
- What is MU-MIMO and why is it important for Wi-Fi 6 and 6E?
- 【Lazy Self-Care Fish Tank Control System】Work Submission
- [Summer benefits to celebrate the Dragon Boat Festival] A large number of technical information is waiting for you to claim, and there are also gifts to help
- Please help me find which resistor in the picture is the 1.1K resistor in the formula?
- Xiaozhi Science Popularization丨How to Correctly Understand Power Supply Ripple and Noise
- Find the maximum voltage stress of the diode when it is working